How Automatic Resource Management Can Lead to Memory Leaks
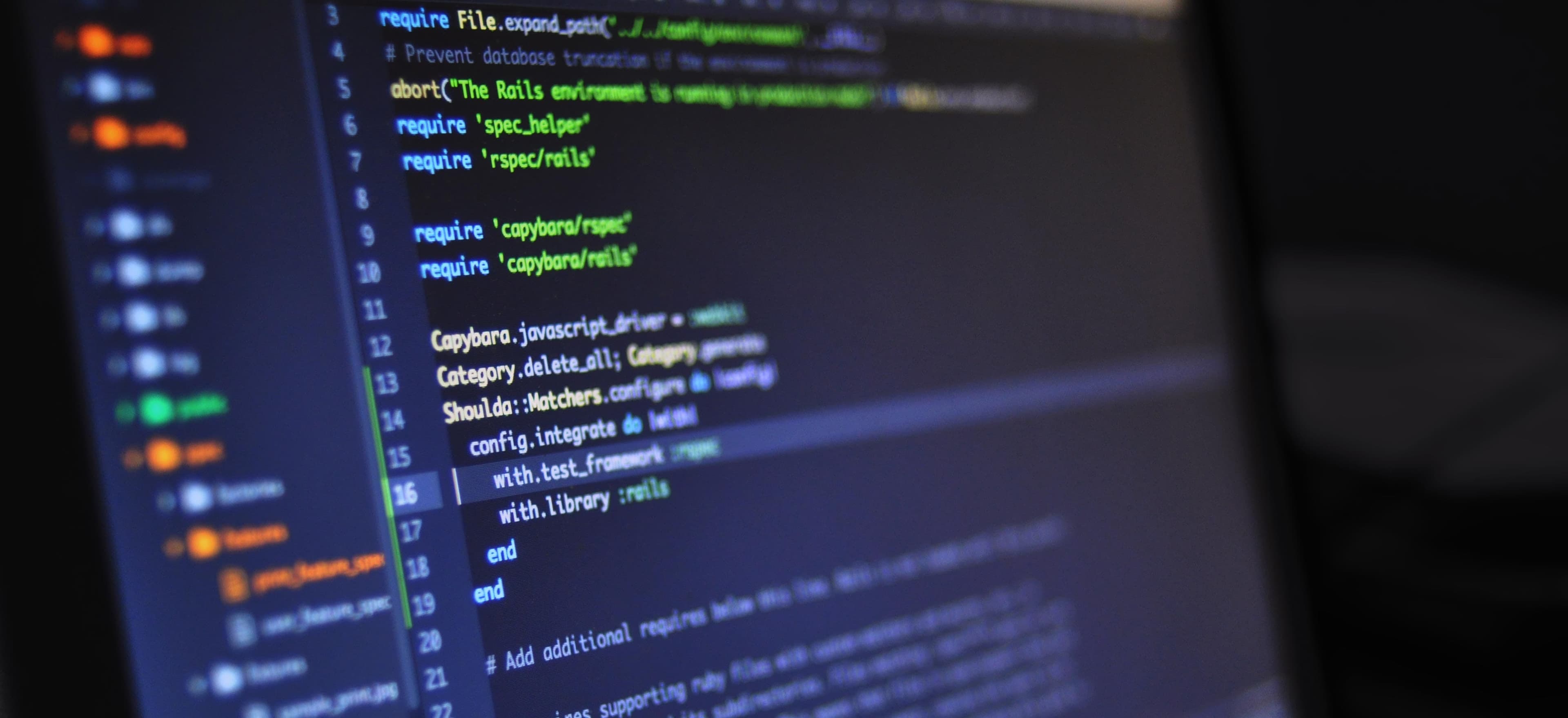
- Published on
How Automatic Resource Management Can Lead to Memory Leaks
In Java, automatic resource management is placed at the forefront of effective programming. The idea is simple—Java’s garbage collector is designed to free up memory by automatically reclaiming objects that are no longer in use. However, the truth is a bit more complicated. Despite Java's built-in mechanisms, it is entirely possible for developers to inadvertently introduce memory leaks. In this post, we will explore how this can happen and what you can do to prevent it.
Understanding Resource Management in Java
Java uses a garbage collection system, relying on several algorithms to manage memory automatically. The most commonly used mechanisms for resource management are the following:
-
Automatic Garbage Collection: Java uses garbage collectors to reclaim memory from objects that are no longer referenced. The Java Virtual Machine (JVM) manages heap memory where objects reside.
-
try-with-resources Statement: Introduced in Java 7, this framework helps manage resources like files and sockets. When the try block is complete, it automatically closes the resources.
Here's an example:
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
In this snippet:
- Why Use This?: Automatic resource management ensures that the BufferedReader is closed automatically, even if the read operation throws an exception.
What Causes Memory Leaks in Java?
Despite these automatic features, memory leaks can occur through careless coding practices. Here are some common scenarios that lead to memory leaks in Java:
1. Unintentional Object References
When objects are referenced unintentionally, they remain in memory even if they are no longer needed. A classic example is using collections like Lists or Maps that hold references to objects.
List<MyObject> objects = new ArrayList<>();
objects.add(new MyObject());
- Why This Matters: If you do not clear the list when it's no longer needed, the
MyObject
instances will stay in memory.
2. Static References
Static fields can lead to memory leaks when they reference objects that should be garbage-collected. Static fields persist for the lifetime of the application.
public class MemoryLeak {
private static final List<MyObject> leakedObjects = new ArrayList<>();
public static void addObject(MyObject obj) {
leakedObjects.add(obj);
}
}
- Why Is This Problematic?: The
leakedObjects
list never gets cleared, and even if theMyObject
instances become unused, they will remain in memory as long as the class is loaded.
3. Unclosed Resources
Using resources like database connections or file streams improperly can cause memory leaks as well. If you fail to close resources after usage, they might not be eligible for garbage collection.
Connection conn = DriverManager.getConnection(url);
Statement stmt = conn.createStatement();
stmt.executeQuery("SELECT * FROM table");
// Missing conn.close() and stmt.close()
- Why Should You Care?: If the application repeatedly creates connections without closing them, it could lead to exhausting the database connection pool.
Best Practices to Prevent Memory Leaks
To mitigate the risk of memory leaks, practitioners should adhere to the following best practices:
1. Use Weak References
Java provides the WeakReference
class, which allows an object to be garbage-collected when it is strongly referenced elsewhere. This is particularly useful in cache scenarios.
WeakReference<MyObject> weakRef = new WeakReference<>(new MyObject());
MyObject myObject = weakRef.get(); // Returns null if MyObject is no longer reachable.
- Why Use This?: Weak references allow memory to be reclaimed without needing the developer to specify when to dereference an object.
2. Implement Proper Clean-Up
Always ensure that any resource opened within an application is closed properly. The best approach is to use the try-with-resources statement.
3. Monitor Memory Usage
Use tools like Java VisualVM and Eclipse Memory Analyzer to monitor your application’s memory usage. These tools can help identify potential leaks.
4. Avoid Long-Lived Static References
As pointed out earlier, avoid using static fields to hold references to objects that can vary in size or number. If necessary, make sure these references are cleared when they are no longer needed.
Lessons Learned
Automatic resource management is a powerful boon in Java, but it shouldn't lead developers to complacency. Understanding how resources are managed can help you avoid the pitfalls that lead to memory leaks. By being aware of your coding practices and following the best practices discussed here, you can effectively manage memory and ensure your Java applications run efficiently.
For further reading on garbage collection and memory management, you might find these resources useful:
- Understanding the Java Garbage Collection Process
- Effective Java - Cleaning Up Resources
Incorporating these practices into your programming workflow will significantly reduce the likelihood of memory leaks, ultimately resulting in more robust Java applications.