Common Pitfalls in Spark Streaming Unit Testing
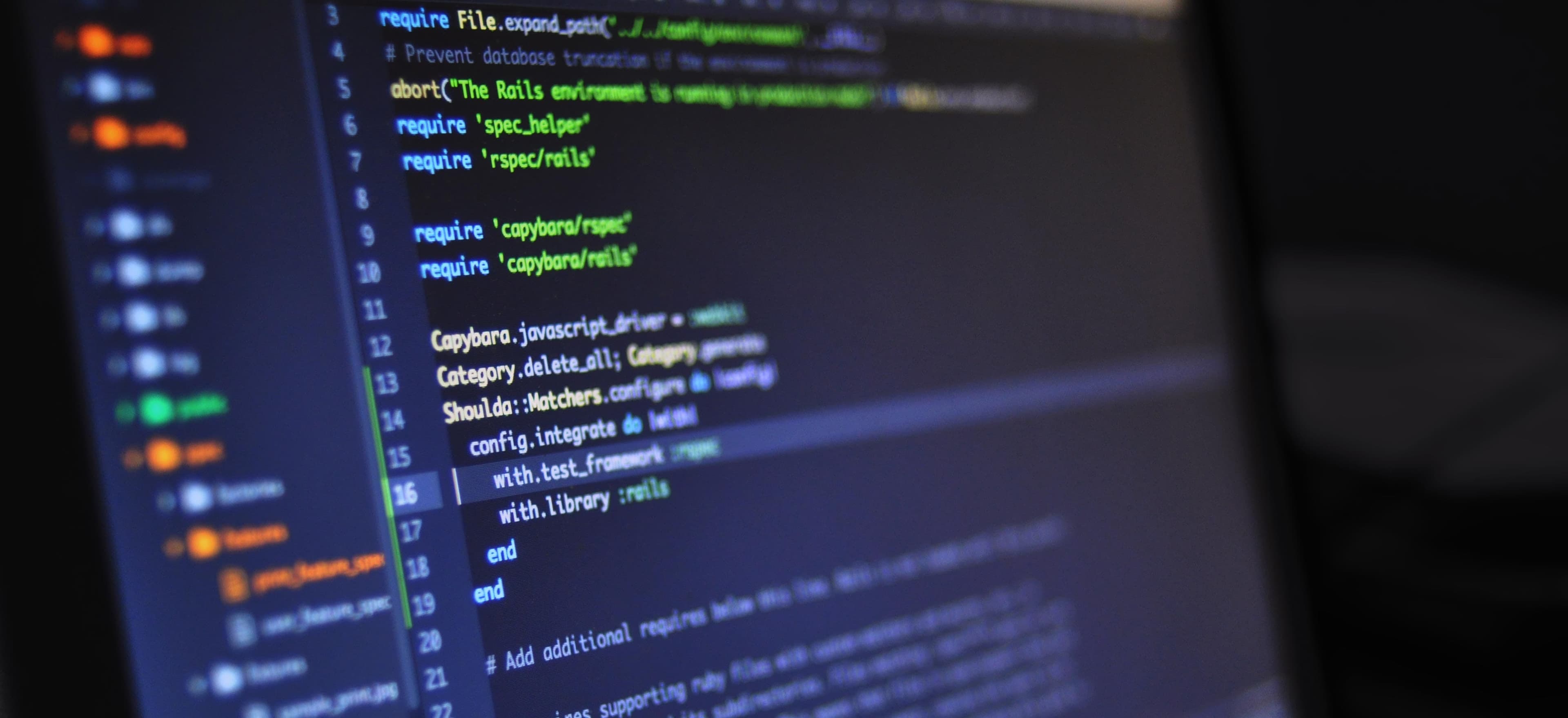
- Published on
Common Pitfalls in Spark Streaming Unit Testing
Apache Spark is a powerful open-source unified analytics engine for large-scale data processing, with built-in modules for SQL, streaming, machine learning, and graph processing. One of its most popular features is Spark Streaming, which allows users to process live data streams. However, testing Spark Streaming applications can be notoriously tricky, and many developers run into common pitfalls. In this blog post, we will discuss these pitfalls and provide effective strategies for overcoming them while optimizing your unit tests.
Understanding Spark Streaming
Before diving into the testing specifics, let’s understand what Spark Streaming does. Spark Streaming enables real-time processing of live data streams. It provides the ability to ingest data from sources like Kafka, Flume, Twitter, and others in batches. New data is processed in small intervals, making it suitable for applications requiring real-time analytics.
Why Unit Testing Is Important
Unit testing is crucial for ensuring that individual components of your application work as expected. This is especially important in data processing pipelines, where issues can propagate, leading to incorrect data analysis or interpretations.
Common Pitfalls in Spark Streaming Unit Testing
1. Insufficient Mocking of External Dependencies
One of the most common mistakes is not adequately mocking external services, such as Kafka or databases. When running unit tests, you should avoid making calls to these external services because it can lead to flaky tests and unpredictable behavior.
import org.apache.spark.streaming.api.java.JavaStreamingContext;
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mockito;
public class StreamingTest {
private JavaStreamingContext jssc;
@Before
public void setUp() {
jssc = Mockito.mock(JavaStreamingContext.class);
}
@Test
public void testStreamingProcess() {
// Your test logic here
}
}
Why it matters: Using mocking frameworks like Mockito allows you to simulate the behavior of external dependencies. This leads to more reliable, deterministic tests.
2. Not Using the StreamingContext Properly
Spark Streaming requires a specific lifecycle for the StreamingContext
, which may be overlooked in unit tests. Incorrect initialization or teardown can lead to resource leaks and inaccurate results.
import org.apache.spark.streaming.Durations;
import org.apache.spark.streaming.api.java.JavaStreamingContext;
public class StreamingContextExample {
public void setupStreamingContext() {
// Create a local streaming context with two working threads
JavaStreamingContext jssc = new JavaStreamingContext("local[2]", "NetworkWordCount", Durations.seconds(1));
// Setup other streaming operations
}
}
Why it matters: Using local[2]
allows parallel processing, which is crucial for simulating the actual streaming context. Make sure to shut down the context properly to release resources.
3. Not Using Accumulators or Broadcast Variables
Understand the differences between accumulators and broadcast variables, as this can affect the distribution and sharing of state across tasks. Failing to implement these correctly can lead to inconsistent state during tests.
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.api.java.JavaRDD;
public class AccumulatorExample {
public int sumValues(JavaSparkContext sc, int[] values) {
final org.apache.spark.util.LongAccumulator accumulator = sc.longAccumulator("Sum Accumulator");
JavaRDD<Integer> rdd = sc.parallelize(Arrays.asList(values));
rdd.foreach(value -> accumulator.add(value));
return (int) accumulator.value();
}
}
Why it matters: This code demonstrates how to use an accumulator to aggregate values across the nodes, ensuring accurate and efficient data processing.
4. Ignoring Windowing Logic
When implementing windowed operations in Spark Streaming, it is essential to understand how time and batch interval settings affect your results. Failure to properly configure window durations can lead to misleading test results.
import org.apache.spark.streaming.api.java.JavaPairDStream;
import org.apache.spark.streaming.api.java.JavaDStream;
import org.apache.spark.streaming.api.java.JavaPairRDD;
JavaPairDStream<String, Integer> windowedStream = inputDStream
.window(Durations.minutes(5), Durations.seconds(30))
.reduceByKey((x, y) -> x + y);
Why it matters: Understand that in this windowed operation, each batch can influence the result for the entire window. The delays in processing and late data can skew test outputs if not correctly understood.
5. Testing Full Application Instead of Unit Components
Some developers attempt to test entire Spark applications rather than focusing on individual components. This often results in lengthy tests that are hard to maintain.
Instead, adopt the strategy of testing each transformation and action in isolation. Use a mock environment to simulate data input, ensuring that the focus remains on the unit being tested.
6. Neglecting Resource Configurations
When using Spark, resource configurations (like executor memory, parallelism, etc.) can significantly impact testing and performance. Ensure your tests mimic production settings closely.
For example:
SparkConf conf = new SparkConf()
.setMaster("local[*]")
.setAppName("MyApp")
.set("spark.executor.memory", "512m")
.set("spark.executor.instances", "1");
Why it matters: This configuration closely resembles a "real" Spark environment and helps identify potential pitfalls before deployment.
7. Lack of Proper Assertions and Verifications
Finally, insufficient verification of outputs may lead to incorrect conclusions about your streaming logic. Always implement assertions that compare the processed output to expected results.
import org.junit.Assert;
import org.junit.Test;
public class VerifyingOutputs {
@Test
public void testOutput() {
// Simulate input and triggers processing
// Assert results here
Assert.assertEquals(expectedOutput, actualOutput);
}
}
Why it matters: Assertions help ensure that your logic is functioning correctly and that any changes in the pipeline do not unexpectedly affect your results.
In Conclusion, Here is What Matters
Unit testing in Spark Streaming might seem daunting, but by avoiding common pitfalls, you can create a robust testing strategy. Mock external dependencies, utilize accumulators, understand your lifecycle, and make sure to conduct unit tests rather than testing entire applications. For more in-depth knowledge on testing frameworks for Spark applications, you can refer to Apache Spark's official documentation and discuss best practices in community forums like Stack Overflow.
By following these guidelines, you’ll improve the reliability of your Spark Streaming applications while enhancing your workflow efficiency. Happy testing!