Common Pitfalls When Dockerizing Spring Boot Apps
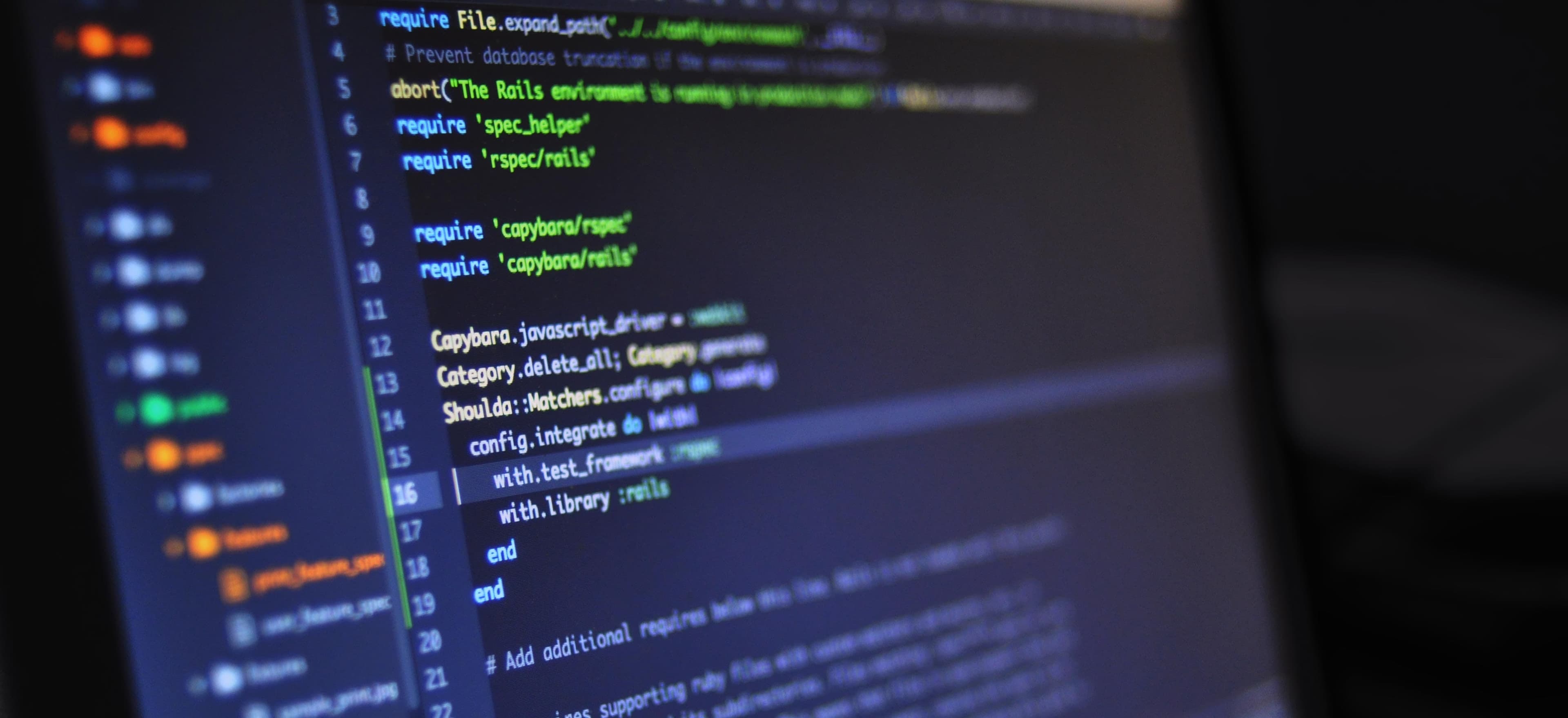
- Published on
Common Pitfalls When Dockerizing Spring Boot Apps
Docker has revolutionized the world of application deployment, allowing developers to package applications with their dependencies into containers. This approach not only streamlines the deployment process but also ensures consistent environments across development, testing, and production. However, when Dockerizing Spring Boot applications, developers often encounter several common pitfalls that can hinder performance and functionality. In this blog post, we will explore these pitfalls, how to recognize them, and offer solutions to ensure a smooth Docker experience.
Pitfall 1: Ignoring the .dockerignore
File
Just as a .gitignore
file prevents unnecessary files from being tracked in Git, the .dockerignore
file prevents unwanted files from being included in your Docker image. Failing to create this file can lead to bloated images and longer build times.
Why It Matters
Including unnecessary files increases the image size, leading to longer deployment times and wasted resources.
Solution
Create a .dockerignore
file in your project root and ensure it contains the following entries:
target/
.git/
.idea/
*.iml
*.log
*.md
Example
If your Spring Boot application is structured like this:
my-spring-boot-app/
├── .gitignore
├── .dockerignore
├── pom.xml
└── src/
Your .dockerignore
should prevent files that do not need to be in the final image from being built.
Pitfall 2: Not Using Multi-Stage Builds
Docker allows you to create multi-stage builds, which help produce smaller and more efficient images. A common mistake is to build the application along with all development dependencies in a single stage.
Why It Matters
Single-stage builds lead to unnecessarily large images due to development dependencies staying in the final image, which may not be required to run the application.
Solution
Utilize multi-stage builds to separate your build environment from your production environment.
# Use Maven to build the application
FROM maven:3.8.1-openjdk-17 AS build
WORKDIR /app
COPY pom.xml .
COPY src/ ./src
RUN mvn clean package -DskipTests
# Create a smaller production image
FROM openjdk:17-jdk-slim
WORKDIR /app
COPY --from=build /app/target/my-spring-boot-app.jar ./my-spring-boot-app.jar
ENTRYPOINT ["java", "-jar", "my-spring-boot-app.jar"]
Explanation
- The first stage (
build
) uses the Maven image to compile the Spring Boot app. - The second stage (
production
) uses a lighter JDK image, copying only the necessary jar file.
Pitfall 3: Overlooking HEALTHCHECK
Ensuring that your application is running correctly is critical, especially in production environments. Failing to implement a HEALTHCHECK directive can lead to running instances that fail without detection.
Why It Matters
Without a proper health check, orchestration tools like Kubernetes may not be aware of an unhealthy application, leading to downtime.
Solution
Implement a HEALTHCHECK instruction in your Dockerfile as follows:
HEALTHCHECK --interval=30s --timeout=10s --retries=3 CMD curl -f http://localhost:8080/actuator/health || exit 1
Explanation
--interval=30s
: Run the check every 30 seconds.--timeout=10s
: Give it 10 seconds to respond.--retries=3
: Consider the container unhealthy after 3 failed attempts.
This setup effectively uses the Spring Boot Actuator to check the application's health.
Pitfall 4: Hardcoding Configuration Values
Hardcoding configuration values in your application can make it challenging to maintain and scale.
Why It Matters
When your application runs in different environments (development, testing, production), configurations may differ. Hardcoded values can lead to errors during deployment or runtime.
Solution
Externalize configuration using environment variables or Spring profiles.
ENV SPRING_DATASOURCE_URL=jdbc:mysql://localhost:3306/mydb
ENV SPRING_DATASOURCE_USERNAME=your-username
ENV SPRING_DATASOURCE_PASSWORD=your-password
Example
You can modify your application.properties
to use environment variables for database configuration:
spring.datasource.url=${SPRING_DATASOURCE_URL}
spring.datasource.username=${SPRING_DATASOURCE_USERNAME}
spring.datasource.password=${SPRING_DATASOURCE_PASSWORD}
By doing so, you can easily change configurations without modifying your codebase.
Pitfall 5: Forgetting to Optimize the JVM
Another common mistake is not optimizing the Java Virtual Machine (JVM) settings for a containerized environment.
Why It Matters
The default JVM settings might not be suited for container environments. For instance, the memory settings may not reflect the limits imposed by the container.
Solution
Add JVM options tailored for Docker containers to the ENTRYPOINT
.
ENTRYPOINT ["java", "-XX:+UseContainerSupport", "-XX:MaxRAMPercentage=80.0", "-jar", "my-spring-boot-app.jar"]
Explanation
-XX:+UseContainerSupport
: Instructs the JVM to use container settings.-XX:MaxRAMPercentage=80.0
: Allocates 80% of the available container memory to the JVM.
Pitfall 6: Inefficient Logging Configuration
When running Spring Boot in a container, the logs should be sent to the standard output instead of files.
Why It Matters
Containerized applications often require centralized logging solutions. Writing logs to files hinders this, complicating log management.
Solution
Make sure your logging configuration directs output to the console.
Example
In your application.properties
, you can set your logging configuration as follows:
logging.pattern.console=%d{yyyy-MM-dd HH:mm:ss} - %msg%n
This setup routes logs to the console, allowing containerized environments to capture and aggregate logs efficiently.
The Last Word
Dockerizing Spring Boot applications can provide scalability and ease of deployment, but it comes with its own set of challenges. By understanding and avoiding these common pitfalls, developers can create efficient, maintainable, and scalable Docker images. With the right practices, you empower your applications to thrive in containerized environments.
For more practical insights on Docker and Spring Boot integration, check out Spring's official documentation and Docker's getting started guide.
Happy Dockering! 🚢
Checkout our other articles