Streamline Your Java 11: Converting Collections to Arrays
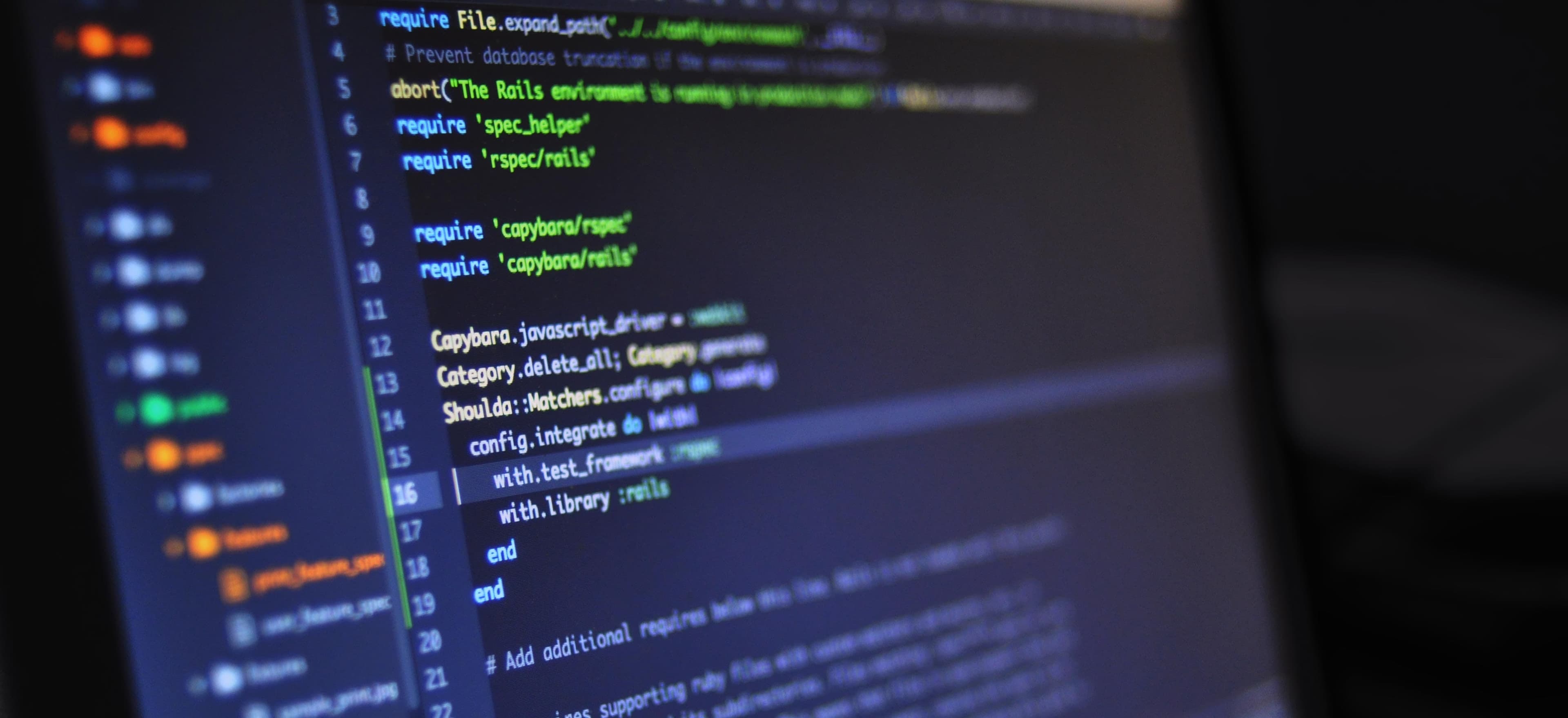
- Published on
Streamline Your Java 11: Converting Collections to Arrays
In the world of Java programming, collections are a key feature of the language. They provide a versatile way to store groups of objects. However, at times, you may need to convert these collections into arrays for specific operations or library functions that require an array as input. In this blog post, we'll explore how to effectively convert collections to arrays in Java 11.
Understanding Collections and Arrays
Before diving into the conversion process, let's clarify what collections and arrays are:
-
Collections: These are data structures provided by Java's Collections Framework, such as
List
,Set
, andMap
. They can dynamically grow or shrink and offer numerous useful methods for managing groups of objects. -
Arrays: These are fixed-size data structures. Once declared, the size of an array cannot change. They offer better performance in terms of speed but lack the flexibility of collections.
Converting between these two structures can be advantageous when you want to leverage the features of both.
When to Convert
You might need to convert collections to arrays for several reasons:
- To interact with legacy APIs that expect arrays.
- To improve performance in certain scenarios.
- To use built-in array algorithms for sorting or searching.
Now, let’s dive into how to accomplish this task effectively in Java 11.
Converting a List to an Array
The most common scenario is converting a List
to an array. The Java Collections Framework provides a convenient method for this: toArray()
.
Here is a simple example demonstrating this process:
import java.util.ArrayList;
import java.util.List;
public class ConvertListToArray {
public static void main(String[] args) {
List<String> stringList = new ArrayList<>();
stringList.add("Java");
stringList.add("Python");
stringList.add("C++");
// Convert List to Array
String[] stringArray = stringList.toArray(new String[0]);
// Output the Array
for (String str : stringArray) {
System.out.println(str);
}
}
}
Explanation of the Code
-
Create a List: We start by creating a
List
of strings and adding three programming language names. -
Convert using toArray(): The
toArray()
method accepts an array as an argument. Passing a new array of the desired type (in this case,new String[0]
) ensures that Java creates an array with the right type and size. Passing zero length triggers thetoArray()
method to allocate a new array of the same size as the collection. -
Print the Array: Finally, we loop through the new array to print each element.
Why Use New String[0]?
Using new String[0]
is a common practice in Java. If you use new String[stringList.size()]
, it could potentially create an unnecessary object if the size of the list is small or usage patterns change. By passing an empty array, Java can handle the right size internally.
Converting a Set to an Array
Similarly, converting a Set
to an array can be done using the toArray()
method. Here’s how:
import java.util.HashSet;
import java.util.Set;
public class ConvertSetToArray {
public static void main(String[] args) {
Set<Integer> numberSet = new HashSet<>();
numberSet.add(1);
numberSet.add(2);
numberSet.add(3);
// Convert Set to Array
Integer[] numberArray = numberSet.toArray(new Integer[0]);
// Output the Array
for (Integer num : numberArray) {
System.out.println(num);
}
}
}
Key Points
-
Order is not guaranteed: When converting a
Set
to an array, note that the order of elements may not follow the insertion order, since sets do not maintain a specific sequence. -
Using Integer[0]: Similar to the list example, using an empty array helps Java manage memory effectively.
Converting a Map to an Array
Maps are a little different since they represent key-value pairs. You can convert a map to an array in two primary ways: extracting keys or values.
Example: Extracting Keys
import java.util.HashMap;
import java.util.Map;
public class ConvertMapKeysToArray {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Java", 1);
map.put("Python", 2);
map.put("C++", 3);
// Convert Map keys to Array
String[] keysArray = map.keySet().toArray(new String[0]);
// Output the Array
for (String key : keysArray) {
System.out.println(key);
}
}
}
Explanation
- Create a Map: A map with keys as strings and values as integers is created.
- Extract Keys: We use the
keySet()
method to get all the keys and then convert it to an array usingtoArray()
.
Example: Extracting Values
import java.util.HashMap;
import java.util.Map;
public class ConvertMapValuesToArray {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Java", 1);
map.put("Python", 2);
map.put("C++", 3);
// Convert Map values to Array
Integer[] valuesArray = map.values().toArray(new Integer[0]);
// Output the Array
for (Integer value : valuesArray) {
System.out.println(value);
}
}
}
Key Takeaways
- Use
keySet()
to convert keys andvalues()
for values. - The approach remains consistent across different collection types;
toArray()
handles the conversion efficiently.
Closing Remarks
Converting collections to arrays in Java 11 has become a streamlined process thanks to the robustness of the Collections Framework. Familiarity with this process not only enhances your coding efficiency but also expands your ability to integrate Java applications with various libraries or existing legacy codebases.
Whether working with List
, Set
, or Map
, understanding the conversion methods helps to better manage data in Java applications. For further reading on collections, the official Java Documentation offers comprehensive guides and advanced features.
As you continue your journey with Java, remember that mastering these foundational tasks enhances your development skill set and prepares you for more complex programming challenges in the future.
Happy coding!
Checkout our other articles