Common Pitfalls in Certificate Transparency Verification in Java
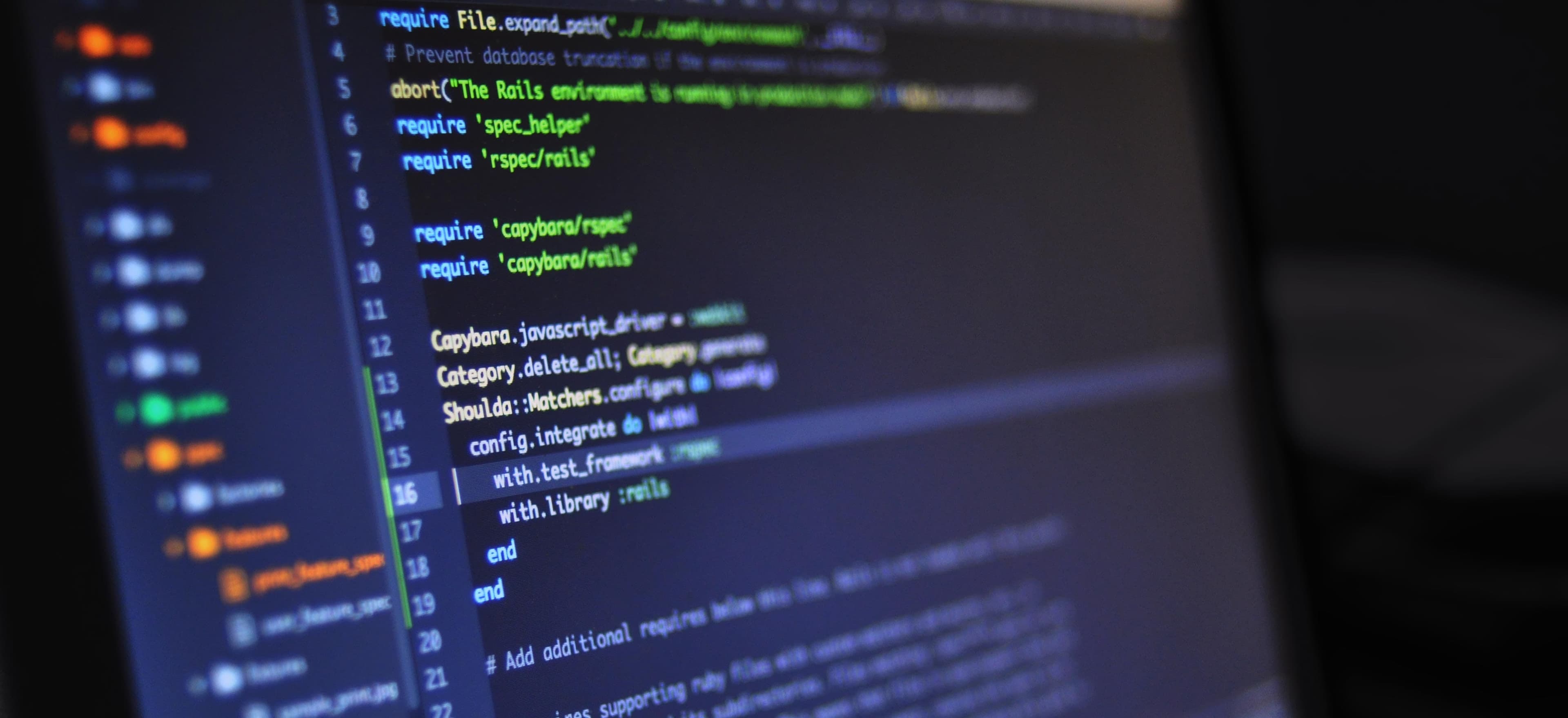
- Published on
Common Pitfalls in Certificate Transparency Verification in Java
Certificate Transparency (CT) is a security mechanism that aims to reduce the chances of fraudulent SSL/TLS certificates being issued by Certificate Authorities (CAs). In Java, implementing CT verification correctly is crucial for maintaining security and trust. However, there are some common pitfalls that developers encounter while working with CT. This blog post discusses these pitfalls, explains how to avoid them, and provides code snippets to illustrate best practices.
Understanding Certificate Transparency
Before diving into common pitfalls, let's briefly revisit what Certificate Transparency is and why it's important. Certificate Transparency works by keeping a public log of all issued certificates, allowing anyone to check if a certificate was issued legitimately. This helps prevent man-in-the-middle attacks and other malicious activities involving fraudulent certificates.
When you verify a certificate against a CT log, you're essentially checking whether it has been logged correctly. It involves fetching logs, validating signatures, and ensuring that the issued certificate is not present in the logs. Let's explore some common challenges Java developers face while implementing these verifications.
1. Ignoring Log Consistency
The Pitfall
One common mistake developers make is ignoring the importance of consistency among multiple CT logs. Each log must provide a consistent view of the certificate data. If different logs show different states for the same certificate, it could suggest a compromise or error in one or more logs.
Solution
Always verify the consistency of logs before you perform further checks on the certificate. This can be done by creating a function to fetch the latest entries from multiple logs and comparing them.
Example Code
import java.util.List;
import java.util.Map;
import java.util.HashMap;
// A simple method to check log consistency
public boolean checkLogConsistency(List<String> logEntries) {
Map<String, Integer> logCount = new HashMap<>();
for (String entry : logEntries) {
logCount.put(entry, logCount.getOrDefault(entry, 0) + 1);
}
return logCount.size() == 1; // All entries must match
}
Why?
In the example above, we iterate through a list of log entries and count occurrences of each entry. If all entries are the same, the size of the map will be 1. This approach prevents you from proceeding with verification if inconsistencies are detected, safeguarding your app against potential security issues.
2. Neglecting to Validate Signatures
The Pitfall
Another frequent oversight is overlooking the validation of signatures in CT logs and certificates. Validating signatures ensures the integrity of the log and the authenticity of the certificate. If a certificate's signature is not validated, an attacker could pose as a trusted authority.
Solution
Ensure that your verification routine includes checks for the signatures associated with both the certificate and the CT log. You can implement signature validation using Java's built-in security libraries.
Example Code
import java.security.Signature;
import java.security.PublicKey;
public boolean validateSignature(PublicKey publicKey, byte[] data, byte[] signatureBytes) {
try {
Signature signature = Signature.getInstance("SHA256withRSA"); // Use the appropriate algorithm
signature.initVerify(publicKey);
signature.update(data);
return signature.verify(signatureBytes);
} catch (Exception e) {
// Handle error gracefully
e.printStackTrace();
return false;
}
}
Why?
This code snippet demonstrates signature validation in Java. It initializes the Signature
object with a specified algorithm, updates it with the certificate data, and verifies it against the signature. Failing to perform this step could leave your application vulnerable to fake certificates.
3. Lack of Error Handling and Logging
The Pitfall
Improper error handling and insufficient logging are often neglected, which can lead to difficulty troubleshooting and understanding failures during CT verification. Without proper logging, you may be left in the dark about why certain verifications fail.
Solution
Implement comprehensive error handling and logging mechanisms in your CT verification process to capture significant events and exceptions.
Example Code
import java.util.logging.Logger;
public void verifyCertificate(String certificate) {
Logger logger = Logger.getLogger("CTVerification");
try {
// Performing verification steps...
if (/* verification fails */) {
logger.warning("Certificate verification failed for: " + certificate);
return;
}
logger.info("Certificate verification succeeded for: " + certificate);
} catch (Exception e) {
logger.severe("An error occurred during certificate verification: " + e.getMessage());
// Optionally rethrow or handle more gracefully
}
}
Why?
Using a logging framework helps gather insights into your application’s behavior, making it easier to identify and fix issues. Logging messages at various levels—info, warning, severe—enables you to monitor the verification process comprehensively.
4. Failing to Check for Expired Logs
The Pitfall
Developers sometimes forget that CT logs can expire or may become unavailable due to various reasons (like server downtime). If you attempt to verify a certificate against an expired log, you could be missing pertinent information.
Solution
Implement a logic to periodically verify the availability and expiration of CT logs before using them in verification.
Example Code
import java.net.HttpURLConnection;
import java.net.URL;
public boolean isLogAvailable(String logUrl) {
try {
HttpURLConnection connection = (HttpURLConnection) new URL(logUrl).openConnection();
connection.setRequestMethod("HEAD");
connection.connect();
return connection.getResponseCode() == 200; // Log is available
} catch (Exception e) {
// Log the error and return false
return false;
}
}
Why?
The isLogAvailable
method provides a way to check log availability before performing any verification operations. Using an HTTP HEAD request allows you to check the status without downloading the entire log, improving efficiency.
5. Confusing Certificate Chain Validation with CT Verification
The Pitfall
Often, developers confuse the process of certificate chain validation with CT verification. They might think that passing standard certificate checks is enough to ensure that a certificate is trustworthy while overlooking CT logs entirely.
Solution
Clearly separate the processes of chain validation and CT verification in your architecture. Both routines serve different functions, and both must be executed for a full verification process.
Example Code
public boolean validateCertificateChain(X509Certificate certificate, List<X509Certificate> chain) {
// Implement chain validation using the appropriate Java methods
// Placeholder for successful validation
return true;
}
public boolean verifyCertificateWithCT(X509Certificate certificate) {
// Perform CT verification separately
return true; // Placeholder
}
Why?
By separating these functions, you maintain a clearer architecture and ensure that both paths of verification are thoroughly addressed. Ignoring one could lead to vulnerabilities that attackers can exploit.
Final Thoughts
Implementing Certificate Transparency verification in Java is an important step in ensuring secure communication. However, it comes with challenges that can easily trip up even experienced developers. By addressing the common pitfalls outlined in this post—log consistency, signature validation, error handling, log expiry checks, and separating validation processes—you can build a robust CT verification system.
For further reading, consider checking out the Java Security documentation, which provides great resources for implementing cryptographic operations. By being aware of these pitfalls and implementing the recommendations, you can improve the security posture of your Java applications significantly.
Feel free to share your experiences with Certificate Transparency in the comments below, and let us know what other topics you would like to see discussed!
By following the guidelines and best practices outlined in this blog post, you should be well-equipped to navigate the complexities of Certificate Transparency verification in Java. Happy coding!