Mastering Spring Cloud Gateway: Common Routing Mistakes
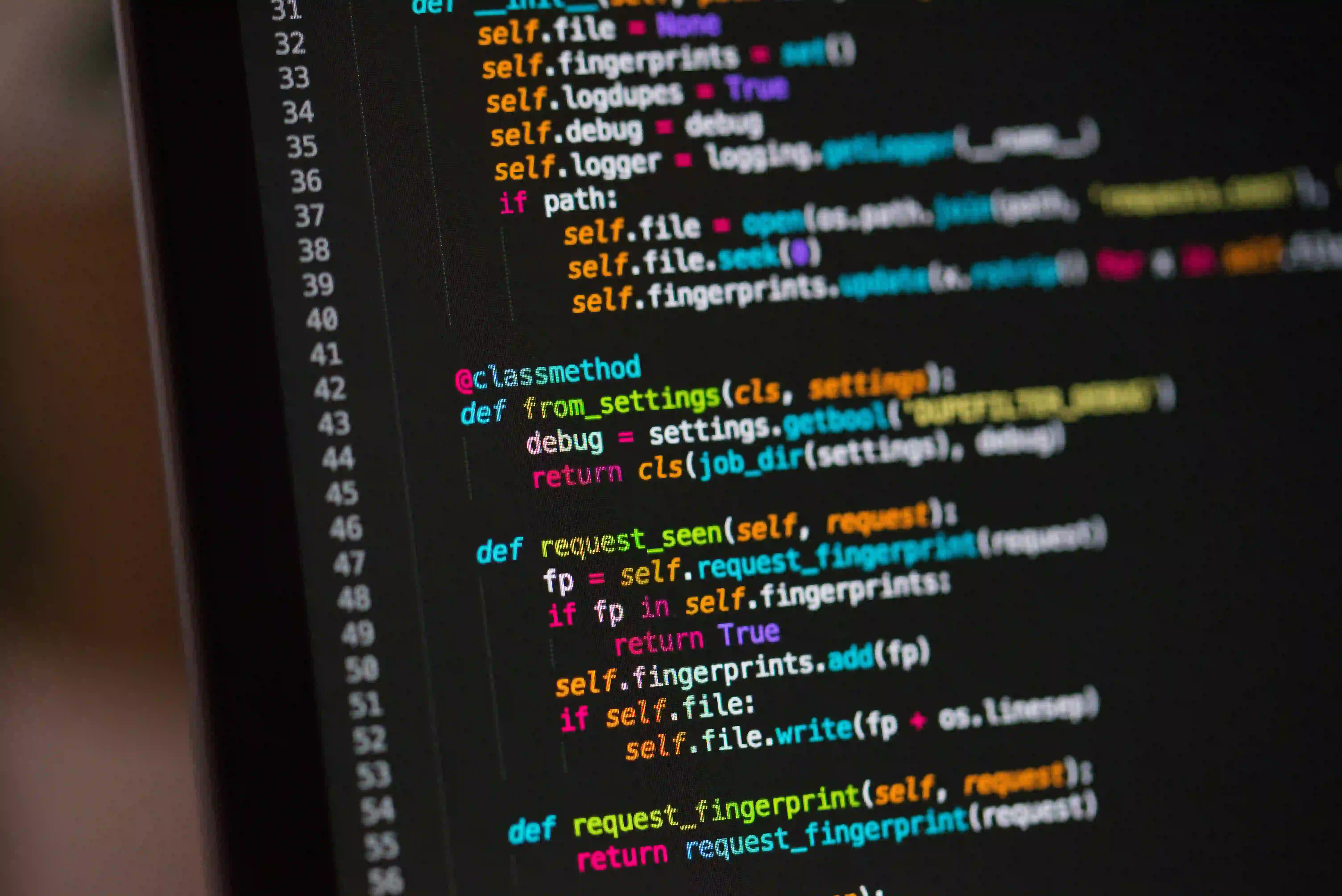
Mastering Spring Cloud Gateway: Common Routing Mistakes
Spring Cloud Gateway is a powerful library for building API gateways on top of Spring Framework. It simplifies routing, providing dynamic routing, and offering resilient patterns like load balancing, retries, and circuit breakers. However, the journey towards an efficient and effective API Gateway can be hindered by common routing mistakes. In this blog post, we will explore some of those pitfalls, offer solutions, and ensure you master Spring Cloud Gateway.
Understanding Spring Cloud Gateway
Before diving into common routing mistakes, it’s important to understand what Spring Cloud Gateway is and why it’s useful. Spring Cloud Gateway provides a simple, powerful way to route requests based on various criteria, such as the request URL, HTTP headers, or query parameters.
The key features include:
- Dynamic Routing: Change routes without requiring redeployment.
- Filters: Alter requests and responses.
- Load Balancing: Direct requests to instance replicas.
For more detailed insights, you can explore the official Spring Cloud Gateway documentation.
Common Routing Mistakes
1. Incorrectly Specifying Route Predicates
When creating routes in Spring Cloud Gateway, predicates play a crucial role in determining which routes to handle incoming requests. A common mistake is to improperly define these predicates.
Example
import org.springframework.cloud.gateway.route.RouteLocator;
import org.springframework.cloud.gateway.route.builder.RouteLocatorBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class GatewayConfig {
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("path_route", r -> r.path("/somepath/**")
.uri("http://example.com"))
.build();
}
}
In this snippet, if the path predicate is not specified correctly (e.g., using /somepath/*
instead of /somepath/**
), requests to /somepath/1
or /somepath/xyz
will not be routed as expected.
Solution
Always verify the use of wildcards. *
matches one segment, while **
matches multiple segments.
2. Hardcoding URIs
Another frequent mistake is the hardcoding of URIs in the route configuration. While it might seem convenient, this can lead to maintenance issues later.
Example
return builder.routes()
.route("static_route", r -> r.path("/service")
.uri("http://localhost:8080/service"))
.build();
When the service address changes, every instance of the hardcoded URI must be updated.
Solution
Use properties files or environment variables to externalize configuration. This approach greatly enhances flexibility.
# application.yml
service:
uri: http://localhost:8080/service
# Gateway Config
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("static_route", r -> r.path("/service")
.uri("${service.uri}"))
.build();
}
3. Not Utilizing Filters
Filters are designed to modify incoming requests or outgoing responses. Neglecting to use them can lead to missed opportunities for logging, security, or request alteration.
Example
Here is a simple filter for logging:
return builder.routes()
.route("logs_route", r -> r.path("/logs/**")
.filters(f -> f.addRequestHeader("custom-header", "value"))
.uri("http://example.com"))
.build();
In this case, we add a custom header, but if you don’t leverage filters, you miss out on altering requests.
Solution
Implement filters to ensure requests and responses are properly logged, modified, or manipulated according to your application’s needs.
4. Ignoring Timeouts and Circuit Breakers
In microservices architecture, latency issues are common. Failing to implement service timeouts and circuit breakers can lead to cascading failures in your application.
Example of Timeout Configuration
return builder.routes()
.route("timeout_route", r -> r.path("/timeout/**")
.uri("http://example.com")
.metadata("timeout", 5000)) // Timeout in milliseconds
.build();
Solution
Leverage the timeout feature, and incorporate circuit breakers to manage failures gracefully. For instance, you can integrate Resilience4j to achieve this.
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("circuit_breaker_route", r -> r.path("/service")
.filters(f -> f.circuitBreaker(c -> c.setName("myCircuitBreaker")
.setFallbackUri("forward:/fallback")))
.uri("http://service:8080"))
.build();
}
5. Misconfiguring Load Balancing
Failing to configure load balancing correctly can lead to reduced performance and uneven traffic distribution.
Example of Load Balanced Route
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("load_balanced_route", r -> r.path("/service/**")
.uri("lb://SERVICE-NAME")) // Load-balanced URI
.build();
}
Solution
Utilize a load balancer like Spring Cloud LoadBalancer to ensure distributed traffic across your microservices properly.
6. Failing to Test Routes
One of the most critical mistakes developers make is not thoroughly testing the defined routes. Issues that seem minor during development can become major problems in production.
Best Practice
Implement unit and integration tests for your routes to catch any issues early. You can use tools like MockMvc to test your routes effectively.
@Test
public void testGatewayRoute() throws Exception {
mockMvc.perform(get("/somepath/test"))
.andExpect(status().isOk());
}
To Wrap Things Up
Mastering Spring Cloud Gateway requires a thorough understanding of routing configurations and common mistakes to avoid. As we discussed, proper use of predicates, avoiding hardcoded URIs, leveraging filters, implementing timeouts and circuit breakers, effectively managing load balancing, and testing routes are crucial to ensuring a robust API gateway.
If you're interested in further enhancing your Spring Cloud Gateway knowledge, consider exploring these additional resources:
- Spring Cloud Gateway Documentation
- Resilience4j Documentation
- Understanding Spring Cloud LoadBalancer
By adhering to best practices and avoiding these common pitfalls, you'll be on your way to building an efficient and resilient API gateway. Happy coding!