Understanding Proxy Patterns: Overcoming Common Pitfalls
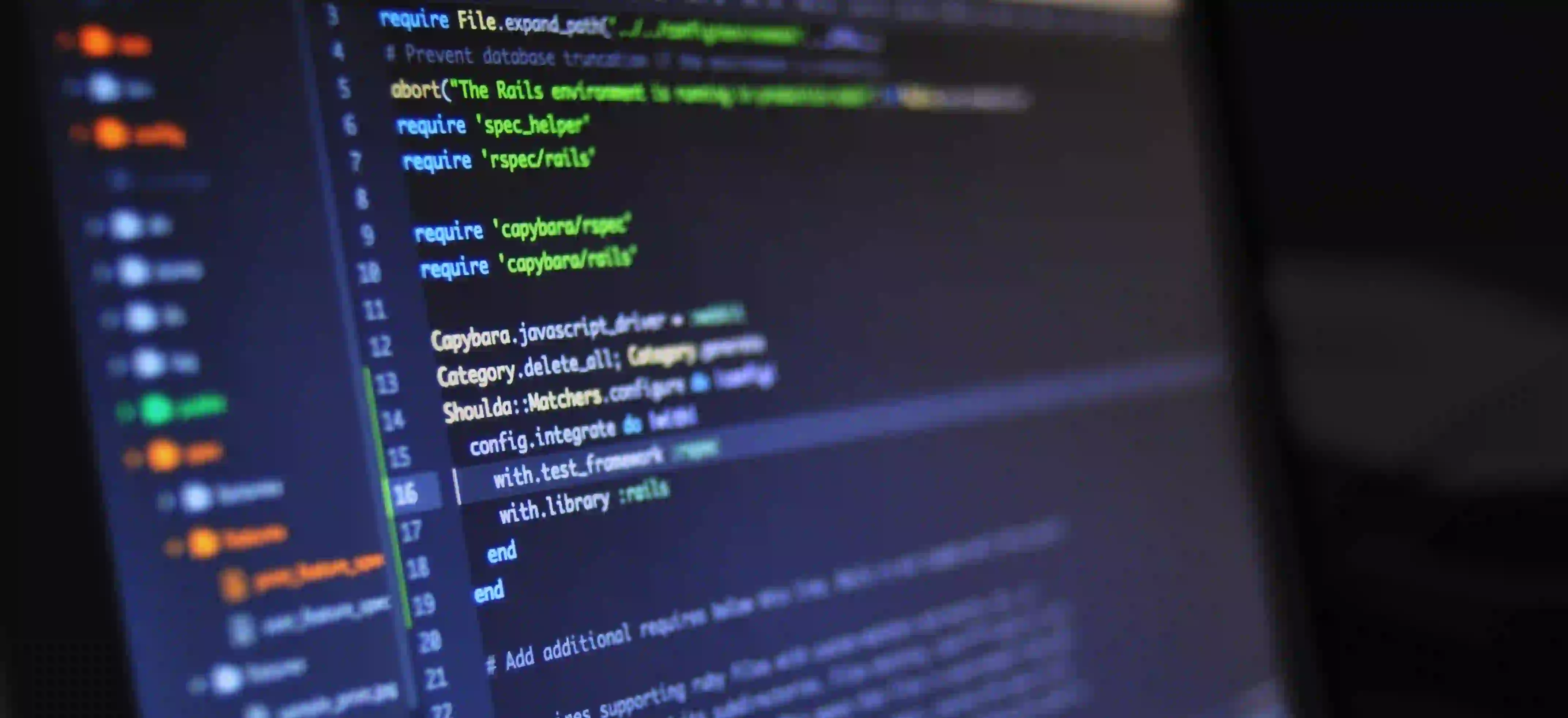
Understanding Proxy Patterns: Overcoming Common Pitfalls
In the world of software design, patterns are fundamental building blocks that help developers tackle common problems in a structured way. One such pattern that has garnered significant attention is the Proxy Pattern. This pattern serves not only to enhance flexibility and efficiency in software applications but also addresses specific issues tied to system architecture.
In this blog post, we will explore the Proxy Pattern in Java, its use cases, and common pitfalls you may encounter while implementing it. By the end, you will have a clearer understanding of how to effectively employ this pattern to your advantage.
What is a Proxy Pattern?
The Proxy Pattern is a structural design pattern that provides a surrogate or placeholder for another object to control access to it. It acts as an intermediary, allowing you to implement additional functionality without altering the original object. In Java, you can implement a Proxy Pattern in various forms, including virtual proxies, remote proxies, and protection proxies.
Key Components
- Subject Interface: This interface defines the contract that both the Real Subject and Proxy will adhere to.
- Real Subject: This is the actual object that the proxy is representing.
- Proxy: This class holds a reference to the Real Subject and delegates requests or adds additional logic.
How to Implement the Proxy Pattern in Java
Let's walk through a basic example of the Proxy Pattern in Java. For this illustration, consider a scenario where we want to control access to a resource-intensive object, such as an image loader.
// Step 1: Create the Subject Interface
public interface Image {
void display();
}
// Step 2: Create the Real Subject
public class RealImage implements Image {
private String filename;
public RealImage(String filename) {
this.filename = filename;
loadImageFromDisk();
}
private void loadImageFromDisk() {
System.out.println("Loading " + filename);
}
public void display() {
System.out.println("Displaying " + filename);
}
}
// Step 3: Create the Proxy Class
public class ProxyImage implements Image {
private RealImage realImage;
private String filename;
public ProxyImage(String filename) {
this.filename = filename;
}
public void display() {
if (realImage == null) {
realImage = new RealImage(filename);
}
realImage.display();
}
}
Commentary on the Code Snippet
-
Subject Interface: This interface,
Image
, enforces a contract for any implementing classes, ensuring they provide the methoddisplay()
. -
Real Subject: The
RealImage
class implements theImage
interface. It handles the actual loading and displaying of the image. Notice how the image is loaded from disk only when needed. -
Proxy Class: The
ProxyImage
class implements the sameImage
interface. It stores a reference to aRealImage
object and controls its instantiation. This is effective for managing resources, for instance, when dealing with large images that you may not want to load until absolutely necessary.
Use Cases for Proxy Pattern
The Proxy Pattern is particularly useful in the following scenarios:
- Lazy Initialization: As shown above, defer resource-intensive operations until they are absolutely necessary.
- Access Control: A proxy can control access to sensitive objects based on user permissions.
- Network Proxies: When resources are allocated over a network, a proxy can represent the remote object, handling communication efficiently.
- Logging and Monitoring: Proxies can intercept communication to add logging, monitoring, or even access control, giving developers insight into how objects are used.
Common Pitfalls of the Proxy Pattern
Understanding the Proxy Pattern isn't just about knowing how to implement it; it’s also crucial to recognize potential pitfalls. Below are common challenges developers may face:
1. Performance Overhead
Though proxy objects are designed to enhance performance through lazy initialization, they can inadvertently introduce latency, especially if there is significant logic in the proxy that complicates the request.
Solution: Optimize the proxy's logic to ensure it does not become a bottleneck itself. Always measure performance to ensure the benefits of using a proxy outweigh any introduced overhead.
2. Complexity in Implementation
While the Proxy Pattern simplifies object access, it can also complicate relationships between objects, especially in intricate systems with numerous proxies.
Solution: Limit the use of proxies to clear cases where they improve efficiency or resource management. Use design documentation to keep track of responsibilities among classes.
3. Over-using Proxies
It’s tempting to use the Proxy Pattern in every situation involving resource access. This can lead to unnecessary complexity in your codebase.
Solution: Assess whether a proxy is genuinely needed. If direct access suffices, abstraction may not add value and might cloud the readability of the code.
4. Serialization Issues
When dealing with remote proxies, consider serialization carefully. Failing to serialize proxy objects correctly can lead to runtime errors or inconsistencies.
Solution: Test serialization thoroughly when using remote proxies. Make sure that both the proxy and the real subject are serializable, and be mindful of transient fields.
A Real-World Example: Caching with Proxy Pattern
One practical application of the Proxy Pattern is in caching. By leveraging a proxy, developers can cache results of method calls, minimizing expensive operations like database queries.
public class CachedImage implements Image {
private ProxyImage proxyImage;
public CachedImage(String filename) {
this.proxyImage = new ProxyImage(filename);
loadFromCache(filename);
}
private void loadFromCache(String filename) {
// Suppose here we check if the image is already loaded
System.out.println("Checking cache for " + filename);
}
public void display() {
proxyImage.display(); // Delegates to the Proxy
}
}
Why This Example Matters
This example emphasizes the ability of the proxy to not just control access but also to provide caching functionality, enhancing performance especially in applications that frequently access the same resources. This can drastically reduce load times and improve UX.
The Closing Argument
The Proxy Pattern is a powerful tool in Java's design pattern arsenal, facilitating control, efficiency, and flexibility. However, like any strategy, it comes with its own set of challenges. By understanding both the implementation and the common pitfalls, developers can better utilize the Proxy Pattern to create clean, efficient, and maintainable code.
If you're keen to learn more about design patterns, consider checking out resources like Design Patterns in Java or exploring more of Head First Design Patterns for an engaging introduction.
By keeping these principles in mind, you will be better prepared to implement proxies in your projects effectively. Happy coding!