Understanding JDK 9's Module System: Common Pitfalls
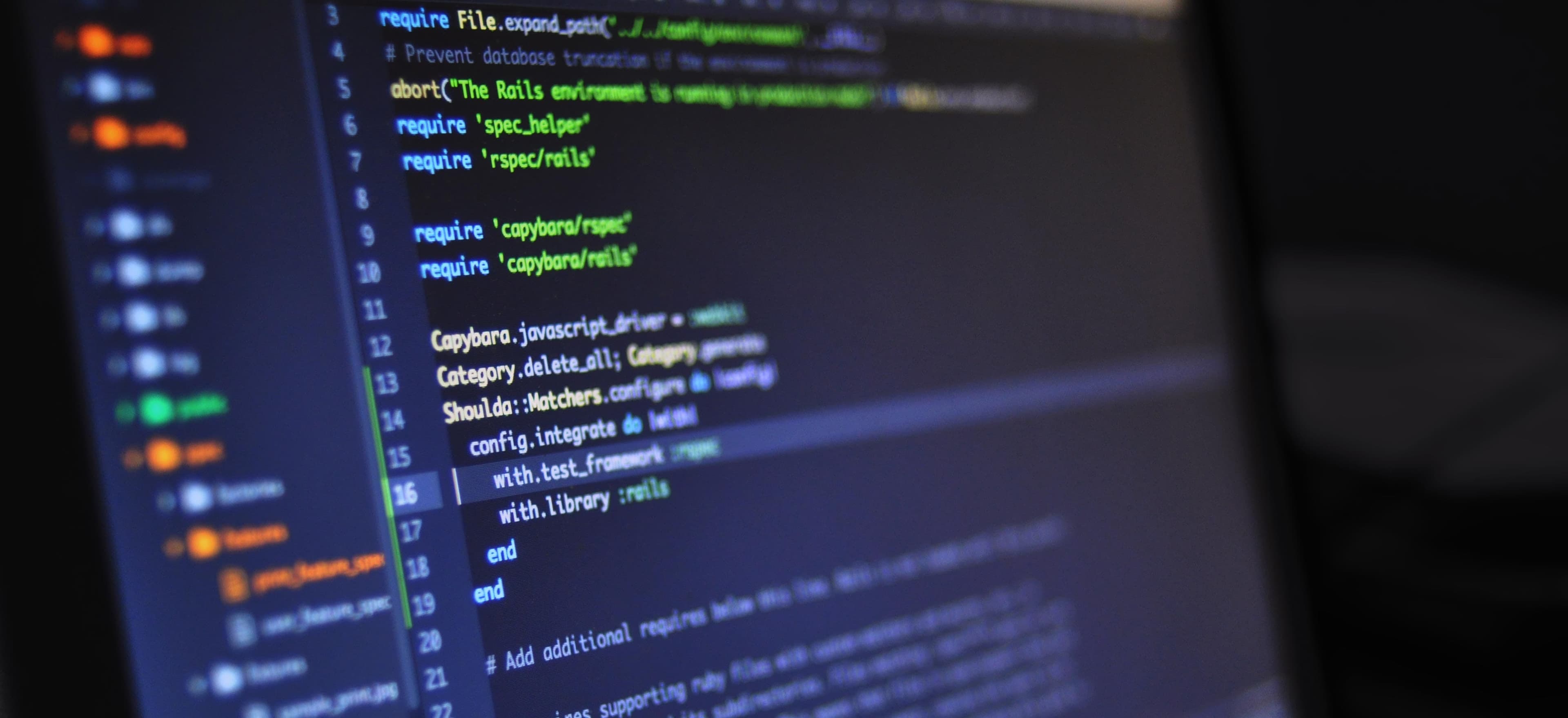
- Published on
Understanding JDK 9's Module System: Common Pitfalls
Java Development Kit (JDK) 9 was a game-changer for Java developers, introducing the long-awaited module system, also known as Project Jigsaw. This significant update aims to improve the structure and maintainability of large codebases. While the module system offers numerous advantages, such as better encapsulation and reduced footprint, it also introduces complexity. Understanding the common pitfalls developers encounter when transitioning to JDK 9's module system can save you both time and frustration.
In this blog post, we will delve into the key elements of the JDK 9 module system, highlight common pitfalls that you may encounter, and provide practical solutions with illustrative code snippets that clarify the 'why' behind them. Along the way, we will ensure that our writing remains engaging, informative, and easily digestible.
What is the JDK 9 Module System?
Before diving into common pitfalls, it's crucial to understand what the module system is and how it works. The module system enables developers to create modular applications, allowing for better organization, encapsulation, and ease of maintenance.
Key Concepts
- Module: A module is a named, self-describing unit of code that specifies which packages it contains and which other modules it depends on.
- Module Descriptor: Every module has a
module-info.java
file that declares its name, the packages it exports, and the modules it requires.
Here's a simple example of what a module descriptor might look like:
module com.example.myapp {
requires java.logging; // This module depends on the java.logging module
exports com.example.myapp.services; // Expose this package to other modules
}
In this snippet, the module com.example.myapp
declares a dependency on java.logging
and exposes the package com.example.myapp.services
. This not only clarifies dependencies but also promotes encapsulation.
Common Pitfalls
With a solid foundation laid, let’s explore some common pitfalls developers face when using JDK 9's module system.
1. Forgetting to Declare Dependencies
One of the most frequent issues developers encounter is forgetting to declare module dependencies in the module-info.java
. If a module does not explicitly declare that it requires another module, it won't be able to access its public types.
Example
Consider a scenario where you have a module com.example.myapp
that needs to utilize classes from java.sql
.
You might be tempted to access java.sql
directly without declaring it in your module-info.java
:
// Incorrect module descriptor
module com.example.myapp {
// Missing dependency on java.sql
}
This will lead to compilation errors. The correct approach is:
// Correct module descriptor
module com.example.myapp {
requires java.sql; // Declare the dependency
}
2. Misunderstanding Package Visibility
Another area where developers commonly stumble is the confusion surrounding package visibility. In a modular system, only the packages that are explicitly exported are accessible to other modules. This can lead to RuntimeException
if a required package is not exported properly.
Example
If your module relates to a service layer, you might think that sharing all classes in a specific package is straightforward. However, to share a package, you must export it:
// Incorrect module descriptor
module com.example.myapp {
requires java.logging;
// Forgetting to export the service package
}
To resolve the visibility issue:
// Correct module descriptor
module com.example.myapp {
requires java.logging;
exports com.example.myapp.services; // Expose the services package
}
3. Classpath and Modulepath Confusion
With the introduction of modules, there are two distinct paths for your Java application: the classpath and the modulepath. Confusing these two can lead to unexpected behavior at runtime.
When compiling or running your Go code, ensure you are specifying the modulepath using the --module-path
option instead of the traditional classpath:
javac --module-path mods -d out -sourcepath src src/com.example.myapp/module-info.java src/com.example.myapp/...
java --module-path mods -m com.example.myapp/com.example.myapp.Main
Using classpath instead of modulepath will not invoke the modular system and can lead you to unintended results.
4. Improper Handling of Automatic Modules
Automatic modules are created when you place non-modular JARs on the module path. These modules can introduce problems if their names conflict with existing modules or if they don't behave as expected.
Example
If you have a JAR file log4j-core
that doesn’t have a module-info.class
, its name will be derived automatically. Conflicts can arise if another module shares a similar name.
To explicitly acknowledge that this is an automatic module, you should inspect and understand the behavior of these modules, even if they simplify module creation.
5. Modularizing Legacy Code
Approaching modularization can be challenging, particularly when dealing with legacy codebases that weren't designed with modular principles in mind. A common mistake is attempting to modularize too quickly, leading to a tangled mess.
To ease this transition, consider the following steps:
- Start small: Begin by identifying core components and modularizing them.
- Incremental changes: Take an incremental approach, refactoring one module at a time.
- Keep testing: Ensure that you have robust tests for every step in the modularization journey to catch potential issues early.
Wrapping Up
Transitioning to JDK 9's module system offers immense benefits, such as improved encapsulation and easier maintenance. Nevertheless, developers must remain vigilant and aware of common pitfalls that may arise during implementation. By acknowledging issues such as dependency declarations, understanding package visibility, and properly managing the classpath vs. modulepath, you can pave a smoother path toward modularization.
For further reading on JDK 9's module system, you may find the following resources helpful:
- Oracle's Official JDK Documentation
- Understanding the Java Platform Module System
With careful consideration and implementation of best practices, you can fully leverage the potential of JDK 9's module system, contributing to cleaner, more maintainable Java code. Happy coding!