Struggling with Swagger Annotations in Play Framework?
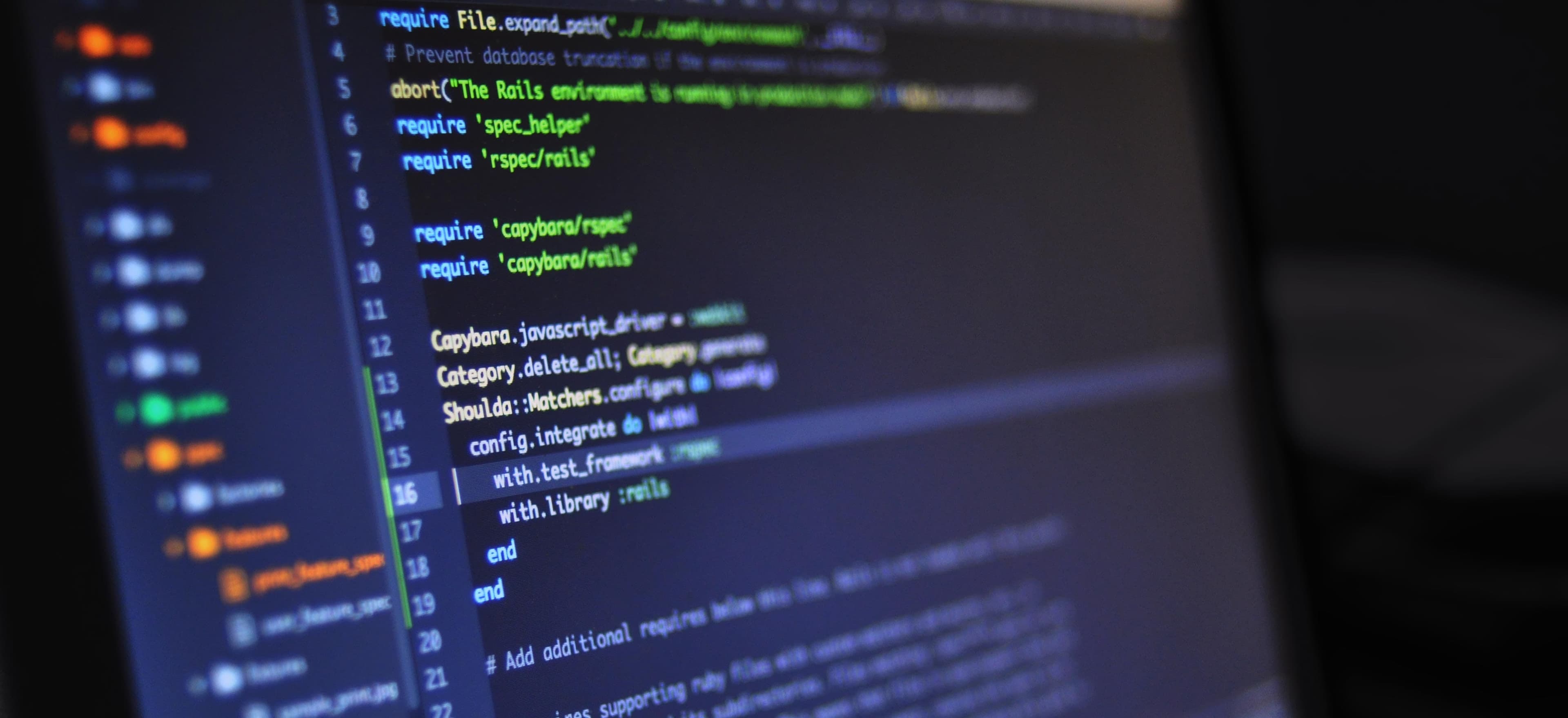
- Published on
Struggling with Swagger Annotations in Play Framework?
If you're developing RESTful APIs with the Play Framework, you may have heard about Swagger. Swagger is a powerful tool that simplifies API documentation, making it easy for developers to understand the endpoints available. It can also help you conduct automated testing of your API. However, integrating Swagger annotations can sometimes be daunting.
In this blog post, we will delve into how to efficiently use Swagger annotations in Play Framework applications. We will also provide code snippets with detailed explanations, and discuss common pitfalls to avoid along the way.
What Is Swagger?
Swagger, now known as OpenAPI, is an open-source project that helps document REST APIs. With Swagger, you can take advantage of:
- Visual Documentation: Automatically generates an interactive documentation page.
- Code Generation: Generates client SDKs in various programming languages.
- Validation: Allows for consistent request and response validation.
Before we dive into the annotations, ensure you have the Swagger Play library in your dependencies. If you're using sbt
, add the following in your build.sbt
:
libraryDependencies += "com.iheart" %% "play-swagger" % "0.9.1"
Setting Up Swagger With Play Framework
To begin with Swagger in Play Framework, follow these steps:
- Install Swagger Play
- Create a Controller
- Annotate Your Endpoints
Let’s break these down step by step.
Step 1: Install Swagger Play
Ensure that you have added the dependency for Swagger Play in your build.sbt
. Once you've done that, you can create your Swagger configuration file.
Create a new Scala file named SwaggerConfig.scala
and place it in your application:
package com.example.swagger
import com.iheart.playSwagger.SwaggerComponents
import play.api.Mode
import play.api.routing.Router
import play.api.{Configuration, Environment}
import play.api.mvc.{ControllerComponents, AbstractController}
class SwaggerConfig @javax.inject.Inject() (
val config: Configuration,
val environment: Environment,
val controllerComponents: ControllerComponents,
val router: Router
) extends SwaggerComponents {
// Additional config settings can be defined here
}
Step 2: Create a Controller
Next up, create a simple controller for demonstration. Below is an example of a UserController that provides basic CRUD operations.
package controllers
import javax.inject._
import play.api.mvc._
import play.api.libs.json._
import scala.collection.mutable
case class User(id: Long, name: String)
object User {
implicit val userFormat = Json.format[User]
}
@Singleton
class UserController @Inject()(cc: ControllerComponents) extends AbstractController(cc) {
private val users: mutable.Map[Long, User] = mutable.Map()
def addUser() = Action(parse.json) { request =>
val user = request.body.validate[User].get
users += (user.id -> user)
Created(Json.toJson(user))
}
def getUser(userId: Long) = Action {
users.get(userId) match {
case Some(user) => Ok(Json.toJson(user))
case None => NotFound
}
}
}
Step 3: Annotate Your Endpoints
Now, let's add Swagger annotations to your controller methods. This will enhance the auto-generated documentation for your API.
import io.swagger.annotations._
@SwaggerDefinition(
info = @Info(
title = "User API",
version = "1.0",
description = "API for user management"
)
)
class UserController @Inject()(cc: ControllerComponents) extends AbstractController(cc) {
@ApiOperation(value = "Add a user", httpMethod = "POST")
@ApiResponses(Array(
new ApiResponse(code = 201, message = "User created"),
new ApiResponse(code = 400, message = "Invalid input")
))
def addUser() = Action(parse.json) { request =>
// Method implementation...
}
@ApiOperation(value = "Get a user", httpMethod = "GET")
@ApiResponses(Array(
new ApiResponse(code = 200, message = "User found"),
new ApiResponse(code = 404, message = "User not found")
))
def getUser(userId: Long) = Action {
// Method implementation...
}
}
Explanation of Swagger Annotations
-
@SwaggerDefinition: It provides a foundation for your API documentation. You specify details like title, version, and description.
-
@ApiOperation: This annotation describes an operation or endpoint. It includes information like the HTTP method used, which helps clients know how to interact with it.
-
@ApiResponse: This annotation outlines possible outcomes of the API call, telling clients what to expect. Each response code can have a message providing clarity.
These annotations not only improve the readability of the code but also serve as an essential reference for API consumers.
Generating Swagger UI
With the controller set up, you can now generate the Swagger UI for your API. When your Play application is running, navigate to /swagger-ui/
. You should see the interactive API documentation that Swagger provides.
Common Pitfalls and How to Avoid Them
1. Not Validating Input
Validation of input is crucial. Ensure API consumer requests are properly validated to avoid errors down the line. Use Json.validate
to ensure the request body conforms to your data model.
2. Missing Annotations
If you don’t annotate your endpoints properly, you will end up with incomplete API documentation. Be thorough in your documentation efforts.
3. Avoid Over-Complicating Routes
Keep your API routes RESTful and simple. The more complex your routes are, the harder it will be for API users to understand.
To Wrap Things Up
Integrating Swagger annotations into your Play Framework applications can significantly improve your API's usability and developer experience.
We've explored how to set it up, annotate your endpoints, and provided tips on avoiding common pitfalls.
For further reading and deeper insights, explore:
With these tips and code snippets, you should be well on your way to crafting well-documented APIs that both you and your users can benefit from. Happy coding!