Avoiding Pitfalls with Java Future and Empty Value Types
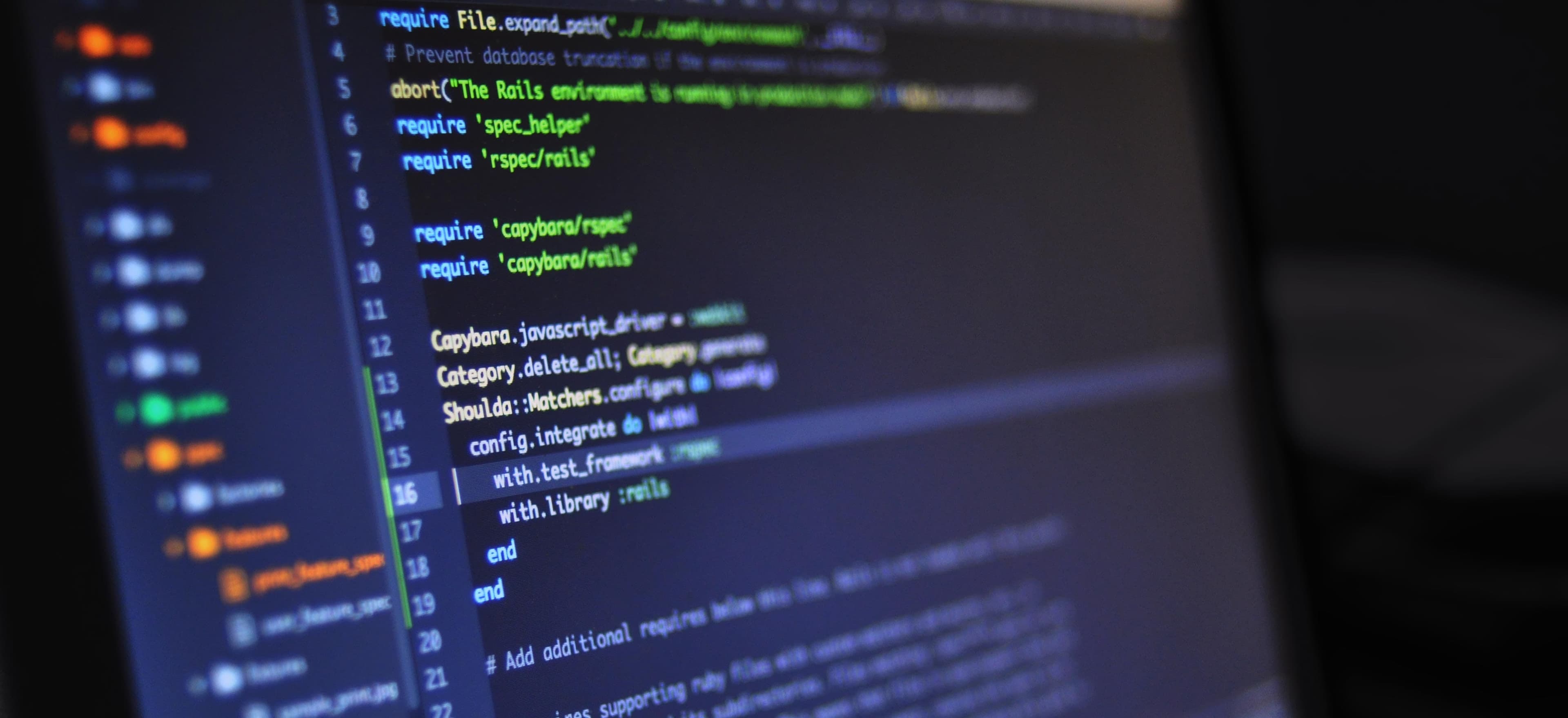
- Published on
Avoiding Pitfalls with Java Future and Empty Value Types
Java is a powerful language widely used in enterprise applications. Its concurrency utilities, specifically the Future
interface, provide a way to manage asynchronous tasks. However, when dealing with Future
and empty value types, developers often encounter common pitfalls. In this post, we will explore how to effectively manage Future
objects in Java while avoiding these pitfalls.
Understanding Java Future
The Future
interface in Java, part of the java.util.concurrent
package, represents the result of an asynchronous computation. This object allows you to fetch the result of a computation once it completes, whether successfully or with an error.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class FutureExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(2);
Future<Integer> future = executorService.submit(new Callable<Integer>() {
@Override
public Integer call() {
// Simulating long-running task
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return 42; // Returning a result
}
});
// Fetching result
try {
Integer result = future.get(); // This will block until the result is available
System.out.println("Result: " + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
} finally {
executorService.shutdown();
}
}
}
In the example above, we create an ExecutorService
with a fixed thread pool and submit a Callable
task. The call
method simulates a long-running computation. We use future.get()
to block the main thread until the task is complete.
The Problem of Empty Value Types
A Future
can also represent a computation that returns an "empty" result, such as when it completes with no outcome or returns an unexpected value. Handling such cases can lead to confusion, particularly if the developer expects a non-null return value. Let’s take a look at how to handle empty value types properly.
Using Optional to Handle Empty Values
Java 8 introduced the Optional
class, which provides a way to express nullable return types more clearly. By using Optional
, you can avoid NullPointerExceptions
and signal the absence of a value explicitly.
import java.util.Optional;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class FutureWithOptional {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(2);
Future<Optional<Integer>> future = executorService.submit(new Callable<Optional<Integer>>() {
@Override
public Optional<Integer> call() {
// Simulating long-running task
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
// Returning Optional.empty() to indicate no value
return Optional.empty();
}
});
// Fetching result
try {
Optional<Integer> result = future.get(); // This will block until the result is available
result.ifPresentOrElse(
value -> System.out.println("Result: " + value),
() -> System.out.println("No result available")
);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
} finally {
executorService.shutdown();
}
}
}
In this modified example, the task returns an Optional<Integer>
. When we call future.get()
, we can handle the absence of a result using ifPresentOrElse
, providing more robust error handling.
Common Pitfalls with Java Future
1. Ignoring Exception Handling
Many developers neglect exception handling when working with Future
. If a task fails, calling future.get()
will throw an ExecutionException
. Always wrap your get
calls in try-catch blocks.
2. Blocking the Calling Thread
Whenever you call future.get()
, the current thread may block until the operation completes. Be careful when designing your application to ensure that you're not inadvertently causing UI freezes or performance issues.
3. Unchecked Exceptions in Callable
Unchecked exceptions thrown inside a Callable
will also be wrapped in an ExecutionException
. It's crucial to either handle unchecked exceptions inside call
or propagate them appropriately.
Best Practices
-
Always Handle Exceptions: Use proper exception handling when dealing with
Future
. -
Leverage Optional: Use
Optional
for computations that can return empty values. This will improve code clarity and maintainability. -
Understand Blocking Behavior: Be cautious of blocking calls in UI applications.
-
Use Timeouts: When calling
future.get()
, consider using a timeout to prevent indefinite blocking.
try {
Integer result = future.get(2, TimeUnit.SECONDS); // Blocks only for 2 seconds
} catch (TimeoutException e) {
System.out.println("Task timed out");
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
Key Takeaways
Java's Future
provides powerful capabilities for managing asynchronous tasks. However, it comes with pitfalls that can trip up even seasoned developers. By understanding empty value types, properly handling exceptions, and employing best practices like Optional
, you can avoid these pitfalls and write more resilient Java code.
To further explore Java Concurrency, check out the official Java Concurrency documentation.
By taking a step back and analyzing how we handle Future
and empty values in Java, we can create applications that are not just efficient but also robust and maintainable.
This blog post is a guideline for developers seeking to enhance their understanding of Java's Future
and handling empty values effectively and responsibly. Happy coding!
Checkout our other articles