Overcoming Challenges in Student Web Service Integration
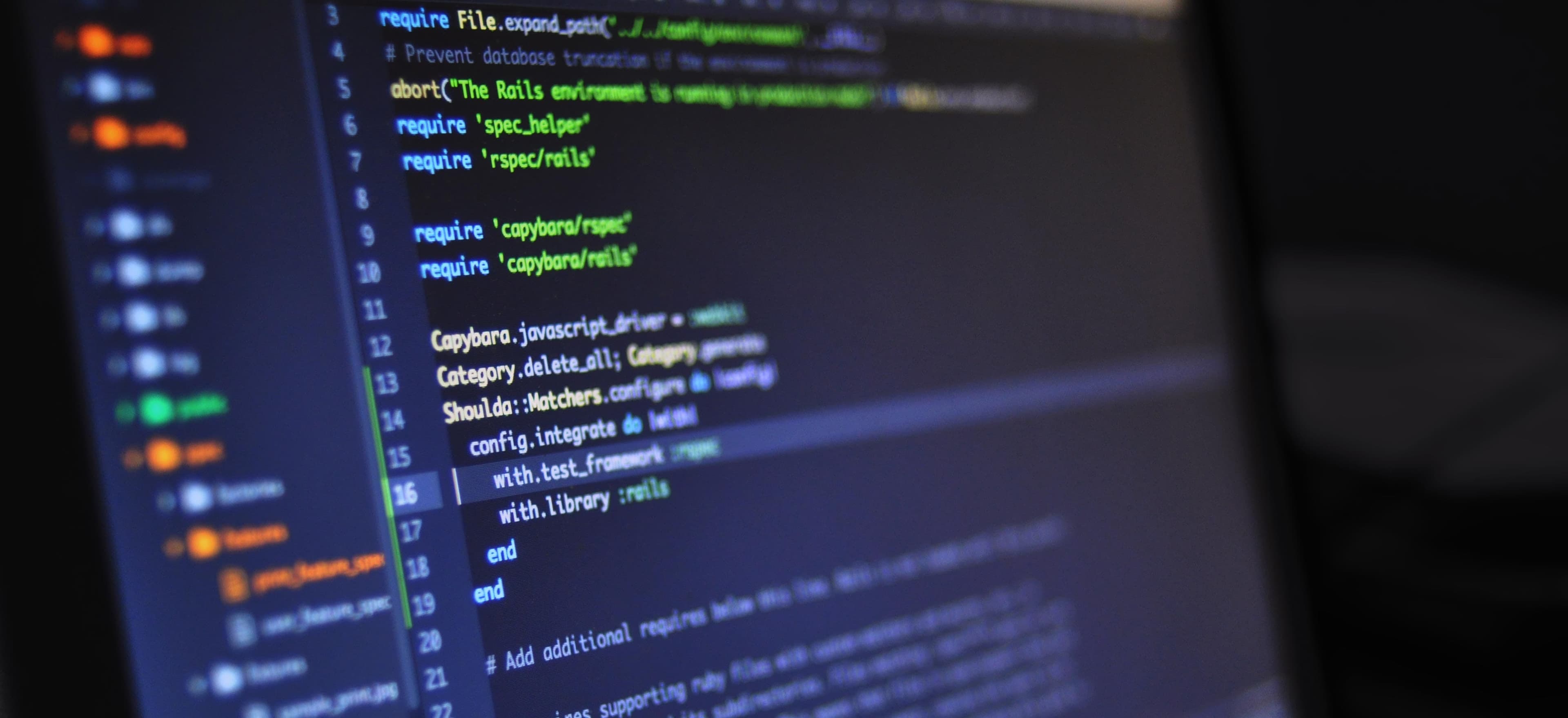
- Published on
Overcoming Challenges in Student Web Service Integration
In today's rapidly evolving educational landscape, the integration of web services—particularly for student management systems—has become paramount. Web services facilitate communication between different applications, ensuring seamless data exchange and improving the overall user experience. However, integrating these services can present various challenges. In this blog post, we'll delve into the common obstacles faced during student web service integration and explore solutions to overcome them.
Understanding Web Services
Web services are software systems designed to support interoperable machine-to-machine interaction over a network. They utilize standard protocols such as HTTP, XML, SOAP, and REST. In a student web service context, they can be used for functions such as fetching student data, handling course registration, and managing grades.
Key Types of Web Services
- SOAP (Simple Object Access Protocol): A protocol for exchanging structured information in web services. It relies on XML and is typically used in enterprise-level solutions.
- REST (Representational State Transfer): A more modern approach leveraging HTTP requests. REST APIs are generally easier to use and are more straightforward than SOAP-based services.
Common Challenges in Student Web Service Integration
1. Data Format Compatibility
One significant challenge in web service integration is ensuring that data formats are compatible across different platforms. Educational institutions often use multiple software systems with varying data formats, which can lead to inconsistency and errors.
Solution
Adopting a universal data format such as JSON or XML can help mitigate compatibility issues. Here is an example of using JSON to exchange student information:
{
"student": {
"id": "12345",
"name": "John Doe",
"courses": [
{"course_id": "CS101", "grade": "A"},
{"course_id": "MATH101", "grade": "B"}
]
}
}
Commentary
Why use JSON? It's lightweight and easier for humans to read and write compared to XML. It also integrates well with JavaScript, making it a preferred choice for modern web services.
2. Authentication and Security
Safeguarding student data is crucial. Many institutions are vulnerable to data breaches, which can undermine the integrity of the entire academic infrastructure.
Solution
Implementing OAuth 2.0 for authentication can enhance security. OAuth provides a robust framework for token-based authorization. Here’s a simplified Java example for implementing OAuth:
import java.net.HttpURLConnection;
import java.net.URL;
public class OAuthExample {
private static final String CLIENT_ID = "your_client_id";
private static final String CLIENT_SECRET = "your_client_secret";
private static final String TOKEN_URL = "https://example.com/oauth/token";
public static String getAccessToken() throws Exception {
URL url = new URL(TOKEN_URL);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
String data = "grant_type=client_credentials&client_id=" + CLIENT_ID + "&client_secret=" + CLIENT_SECRET;
// send the request
// handle response etc.
}
}
Commentary
By utilizing OAuth, institutions can protect sensitive student data effectively. This token-based authentication grants access without exposing user credentials, thereby enhancing security.
3. Versioning of Web Services
As educational needs change, updates to the web service can lead to compatibility issues with existing applications. Changes in the API might disrupt normal operation.
Solution
Implementing versioning in your API structure can help maintain backward compatibility. For instance:
GET /api/v1/student/12345
Commentary
Using versioned endpoints allows clients to specify which version of the API they wish to interact with, enabling smooth transitions during updates. For more details, API versioning strategies are worth exploring.
4. Performance Issues
Integrating multiple web services can lead to performance degradation, particularly under high loads, resulting in slow response times.
Solution
To counter performance issues, implement caching strategies and asynchronous processing. For example, using an in-memory data grid like Redis can store frequently requested data and reduce load times. Here's a simple implementation using Redis with Java:
import redis.clients.jedis.Jedis;
public class CacheExample {
public void cacheStudentData(String studentId) {
try (Jedis jedis = new Jedis("localhost")) {
jedis.set(studentId, fetchStudentData(studentId)); // Assume fetchStudentData fetches student data from the web service
}
}
}
Commentary
Caching responses from web services reduces the number of requests made, significantly improving system performance. By fetching data directly from Redis, you can provide nearly instant responses to users.
5. Lack of Standardization
Different web services may operate under various standards, leading to inconsistencies and integration hurdles.
Solution
Implementing standardized protocols like WSDL for SOAP or OpenAPI (formerly Swagger) for REST can help in defining clear contracts for your web services. Here’s a brief example of an OpenAPI definition for a student service:
openapi: 3.0.1
info:
title: Student Service API
version: 1.0.0
paths:
/students/{id}:
get:
summary: Get student by ID
parameters:
- in: path
name: id
required: true
description: ID of the student
schema:
type: string
responses:
'200':
description: A student object
Commentary
Standardized API documentation enhances developer experience and facilitates faster integration. Familiarity with standardized formats can dramatically reduce onboarding times for new developers.
In Conclusion, Here is What Matters
Integrating web services for student management can pose significant challenges. However, by adopting best practices such as standardizing data formats, implementing robust security measures, and managing versioning effectively, educational institutions can significantly improve integration outcomes.
The demand for a cohesive, efficient student management ecosystem will only grow. Understanding and overcoming these challenges will equip institutions for future advancements in education technology.
For further reading, consider exploring these resources: REST vs. SOAP for a deeper understanding of web services and API Design Best Practices for practical guidance on creating scalable APIs.
By addressing these challenges head-on, institutions can ensure a more integrated, efficient, and secure student management process in the long run.
Checkout our other articles