Boosting Java Performance: Tips for JDK 21's New Features
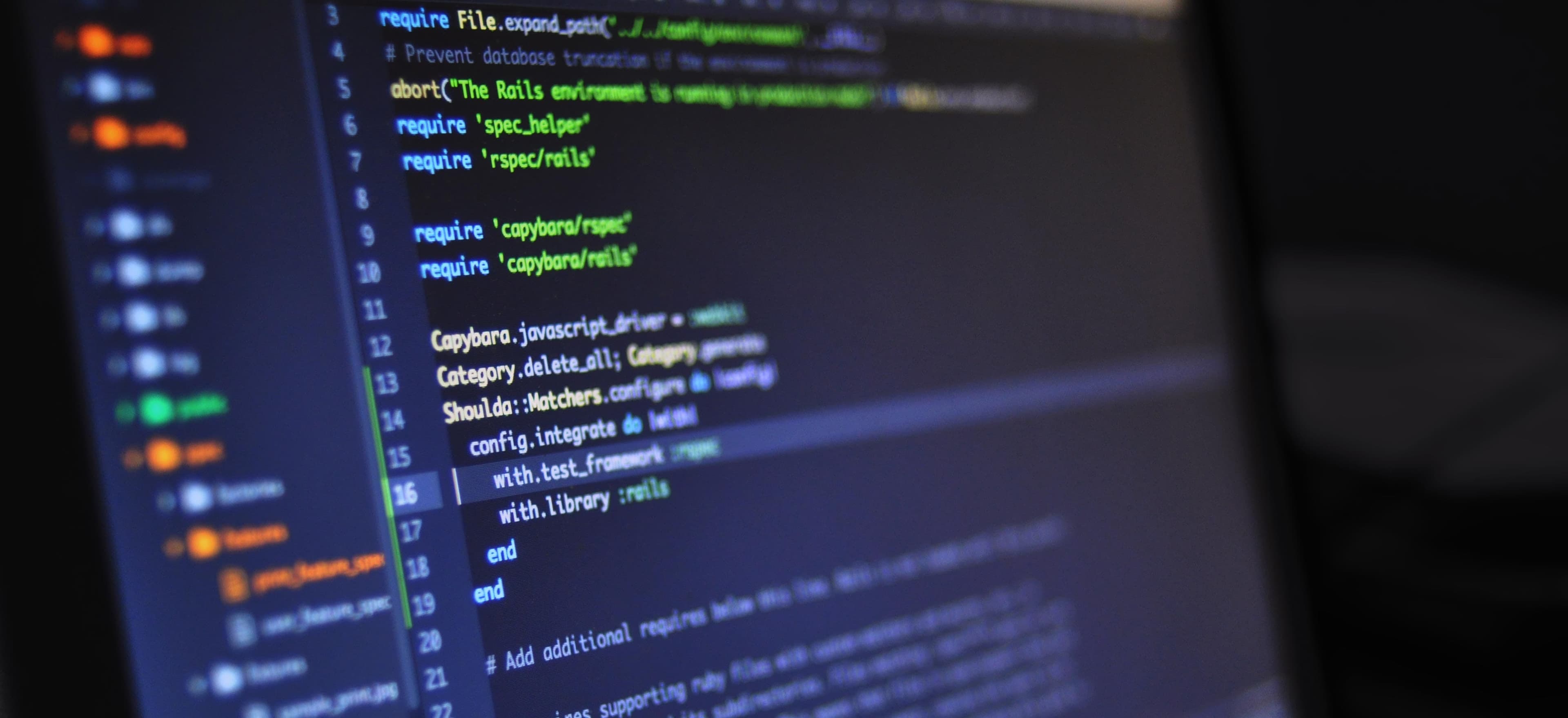
- Published on
Boosting Java Performance: Tips for JDK 21's New Features
Java is a powerful programming language, constantly evolving to meet the demands of developers and the complexity of applications. With the recent release of JDK 21, several new features have been introduced that promise to enhance performance and streamline development. This blog post will delve into those features, providing examples and insights into how they can help you boost the performance of your Java applications.
What’s New in JDK 21?
JDK 21 is packed with enhancements and new features that can significantly improve application performance. Here are some highlights:
- Virtual Threads
- Pattern Matching for Switch
- Generational ZGC
- Scoped Values
Let’s explore each of these features in detail.
1. Virtual Threads
Virtual threads are designed to simplify concurrency in Java. They allow you to create lightweight threads without the overhead associated with traditional Java threads.
Why Use Virtual Threads?
Traditional threads can be resource-intensive, limiting the number of concurrent tasks your application can handle. Virtual threads, on the other hand, dramatically reduce this overhead, enabling thousands or even millions of concurrent threads.
import java.util.concurrent.Executors;
public class VirtualThreadsExample {
public static void main(String[] args) {
var executor = Executors.newVirtualThreadPerTaskExecutor();
for (int i = 0; i < 100_000; i++) {
executor.submit(() -> {
// Simulate some work
try {
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
executor.close();
}
}
Explanation: In this example, we create a virtual thread for each task. The Executors.newVirtualThreadPerTaskExecutor()
method does the heavy lifting, allowing us to scale up the number of threads without consuming excessive resources.
Learn more about Virtual Threads.
2. Pattern Matching for Switch
Pattern matching simplifies coding by allowing you to use a more streamlined syntax when dealing with conditional statements.
Benefits of Pattern Matching
This feature improves readability and reduces boilerplate while also optimizing execution time.
public class Shape {
public static void main(String[] args) {
Object shape = getShape();
switch (shape) {
case Circle c -> System.out.println("Circle with radius: " + c.radius());
case Rectangle r -> System.out.println("Rectangle with area: " + r.area());
default -> System.out.println("Unknown shape");
}
}
public static Object getShape() {
return new Circle(5);
}
}
record Circle(int radius) {}
record Rectangle(int length, int width) {
int area() {
return length * width;
}
}
Explanation: This code snippet demonstrates how to use pattern matching in a switch statement. By directly accessing properties of the objects (like radius
and area()
), we eliminate the need for extensive type checking and casting.
3. Generational ZGC
Z Garbage Collector (ZGC) is designed for low-latency applications. The generational enhancement in JDK 21, ZGC can reclaim memory in even fewer pauses.
Why It Matters?
Garbage collection can be a significant performance bottleneck. With generational ZGC, young and old generations are handled efficiently, thus minimizing the collection time.
public class ZGCExample {
public static void main(String[] args) {
System.out.println("Starting application with ZGC");
// Simulate long-running application
for (int i = 0; i < 50_000; i++) {
String dummy = "Memory allocation " + i;
}
}
}
Explanation: This simple example simulates memory allocation. Running the application with ZGC provides reduced pause times, leading to a smoother performance profile, especially under heavy loads.
To enable ZGC, add the following JVM option:
-XX:+UseZGC
4. Scoped Values
Scoped values are a new feature in JDK 21 that enhance the management of contextual data. They provide a way to pass data within a specific scope without the need for passing context explicitly.
Context Management
Scoped values help avoid boilerplate context management code, improving performance by reducing overhead.
import java.util.concurrent.Executors;
public class ScopedValuesExample {
static class UserContext {
static final ThreadLocal<String> userId = ThreadLocal.withInitial(() -> "Guest");
public static void set(String id) {
userId.set(id);
}
public static String get() {
return userId.get();
}
}
public static void main(String[] args) {
Executors.newVirtualThreadPerTaskExecutor().submit(() -> {
UserContext.set("User-123");
System.out.println("Current User: " + UserContext.get());
});
}
}
Explanation: Here, we use a ThreadLocal
variable to manage user context. This approach allows each virtual thread to handle its context, reducing the need for global state management and minimizing the risk of data corruption.
Final Considerations
JDK 21 brings exciting new features that can dramatically improve the performance and maintainability of your Java applications. By leveraging virtual threads, pattern matching, generational ZGC, and scoped values, you can boost concurrency, readability, and memory management in your projects.
Staying Updated
To keep abreast of Java developments, always refer to the official Java SE Documentation and check out the latest OpenJDK Community updates.
Incorporating these new features into your applications is not only beneficial for current needs but also prepares your projects for future demands. With JDK 21, your Java applications can be faster, more efficient, and easier to maintain than ever before.
Happy coding!
Checkout our other articles