Unlocking Constructor Parameters with Reflection in JDK 8
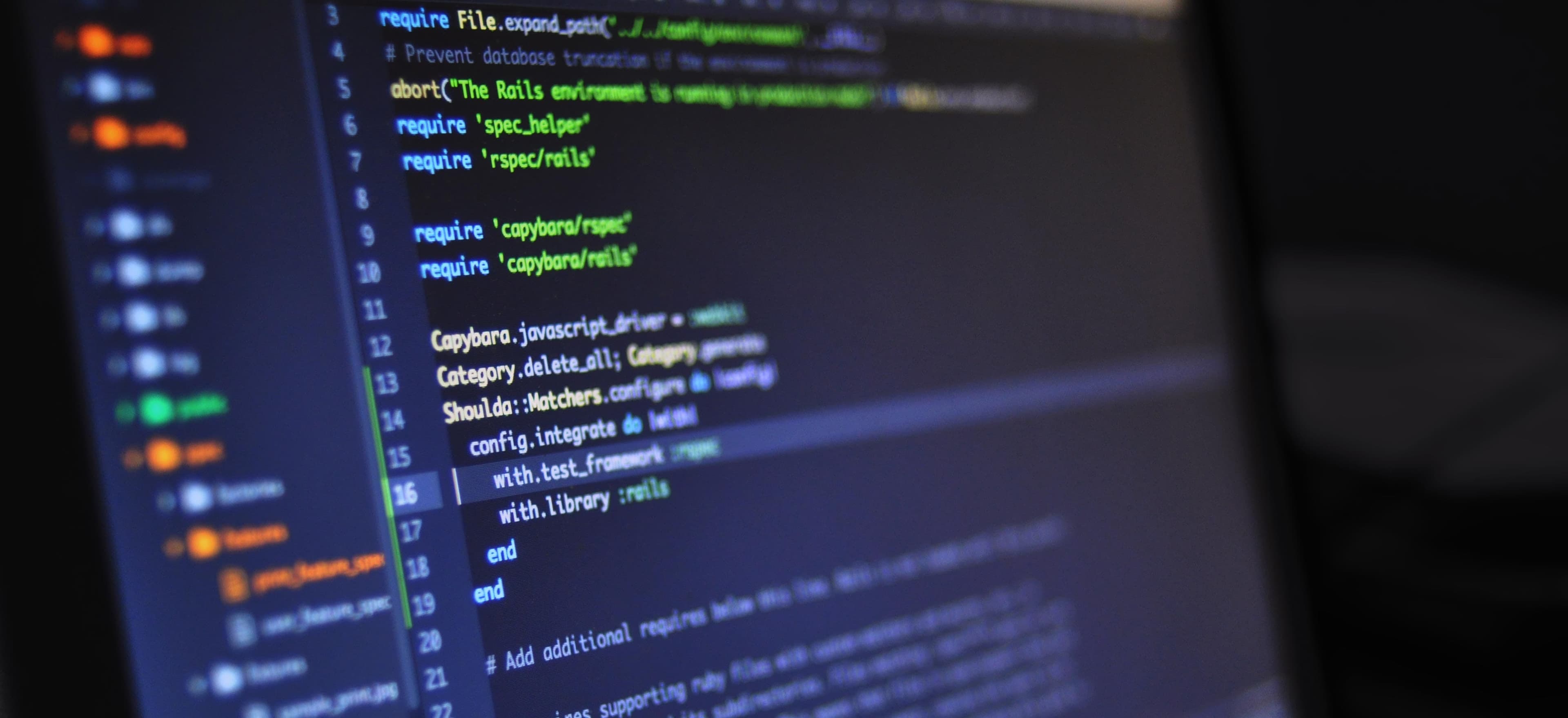
- Published on
Unlocking Constructor Parameters with Reflection in JDK 8
Reflection in Java is a powerful feature that allows developers to inspect classes, interfaces, fields, methods, and constructors at runtime. One of the intriguing aspects of reflection is its ability to unlock constructor parameters dynamically. In this blog post, we'll delve deep into how you can achieve this using Java Reflection in JDK 8, why it's useful, and some practical examples.
Understanding Reflection in Java
Before diving into constructors, it's crucial to grasp the basics of reflection. Reflection is located in the java.lang.reflect
package and enables you to:
- Retrieve metadata about classes and their members.
- Create instances of classes dynamically.
- Invoke methods and access fields at runtime.
Here’s a quick example to demonstrate basic reflection:
Class<?> clazz = Class.forName("java.util.ArrayList");
System.out.println("Class Name: " + clazz.getName());
Method[] methods = clazz.getMethods();
for (Method method : methods) {
System.out.println("Method: " + method.getName());
}
In this snippet, we load the ArrayList
class and output its methods. This should give you a primer on what reflection can accomplish.
Why Constructor Parameters Matter
When creating objects through reflection, knowing the constructor parameters is essential. Constructors may have multiple overloads, and determining the appropriate one can be tricky without proper insight. This is especially useful in frameworks, libraries, or dynamic applications where you may not have compile-time knowledge of classes.
Using Reflection to Access Constructor Parameters
In JDK 8, we can leverage reflection to inspect constructor parameters easily. Here’s how to do it:
- Get the Class Object - Start by loading the class for which you want to inspect constructors.
- Retrieve Constructors - Use
Class.getConstructors()
orClass.getDeclaredConstructors()
to list all available constructors. - Access Parameter Types - For each constructor, get its parameter types using
Constructor.getParameterTypes()
.
Step-by-Step Implementation
Consider the below example where we have a class named User
:
public class User {
private String name;
private int age;
public User(String name) {
this.name = name;
}
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
We need to unlock the constructor parameters for the User
class. Here’s how we can do that using reflection:
import java.lang.reflect.Constructor;
public class ConstructorInspector {
public static void main(String[] args) {
try {
Class<?> clazz = Class.forName("User");
Constructor<?>[] constructors = clazz.getDeclaredConstructors();
for (Constructor<?> constructor : constructors) {
System.out.println("Constructor: " + constructor);
Class<?>[] parameterTypes = constructor.getParameterTypes();
System.out.print("Parameters: ");
for (Class<?> parameterType : parameterTypes) {
System.out.print(parameterType.getSimpleName() + " ");
}
System.out.println("\n");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Code Breakdown:
- Class.forName("User"): Loads the
User
class dynamically. - getDeclaredConstructors(): Fetches all constructors, including private ones.
- getParameterTypes(): Returns an array of
Class
objects representing the types of parameters accepted by each constructor.
Running the Code
When you execute the above code, you should see the following output:
Constructor: public User(java.lang.String)
Parameters: String
Constructor: public User(java.lang.String,int)
Parameters: String int
This output provides clear visibility into the constructors of the User
class and their parameters.
Practical Applications
Using reflection to unlock constructor parameters can be especially useful in scenarios like:
- Dependency Injection Frameworks: Automatically instantiate classes with required dependencies.
- Serialization/Deserialization: When converting objects to/from their JSON/XML representations.
- Testing Frameworks: Create test instances dynamically without knowing their constructors beforehand.
Handling Exceptions
Reflection can throw several exceptions, including ClassNotFoundException
, IllegalAccessException
, and more. You should handle exceptions gracefully, as shown in the previous examples, to ensure your application remains robust.
Reflection Performance Considerations
It is important to note that reflection can be slower than direct code access because of its dynamic nature. Thus, you should:
- Use reflection judiciously, primarily when necessary.
- Cache the results if you often need to access metadata.
- Avoid using reflection in performance-critical paths, unless there's no alternative.
Lessons Learned
Understanding how to unlock constructor parameters with reflection in JDK 8 opens up numerous possibilities for dynamically managing classes and objects. If used wisely, it enhances the flexibility and capability of your Java applications.
Reflection is a double-edged sword; while powerful, it requires careful consideration regarding performance and maintainability. For a deeper dive into Java reflection, consider checking official documentation or previous discussions like Java Reflection Tutorial.
Final Thoughts
Now that you have the tools to unlock constructor parameters, experiment with different classes and constructors. The world of reflection is vast and can significantly enhance your Java programming prowess. Happy coding!