Mastering Type Safety: Common Java Generics Pitfalls
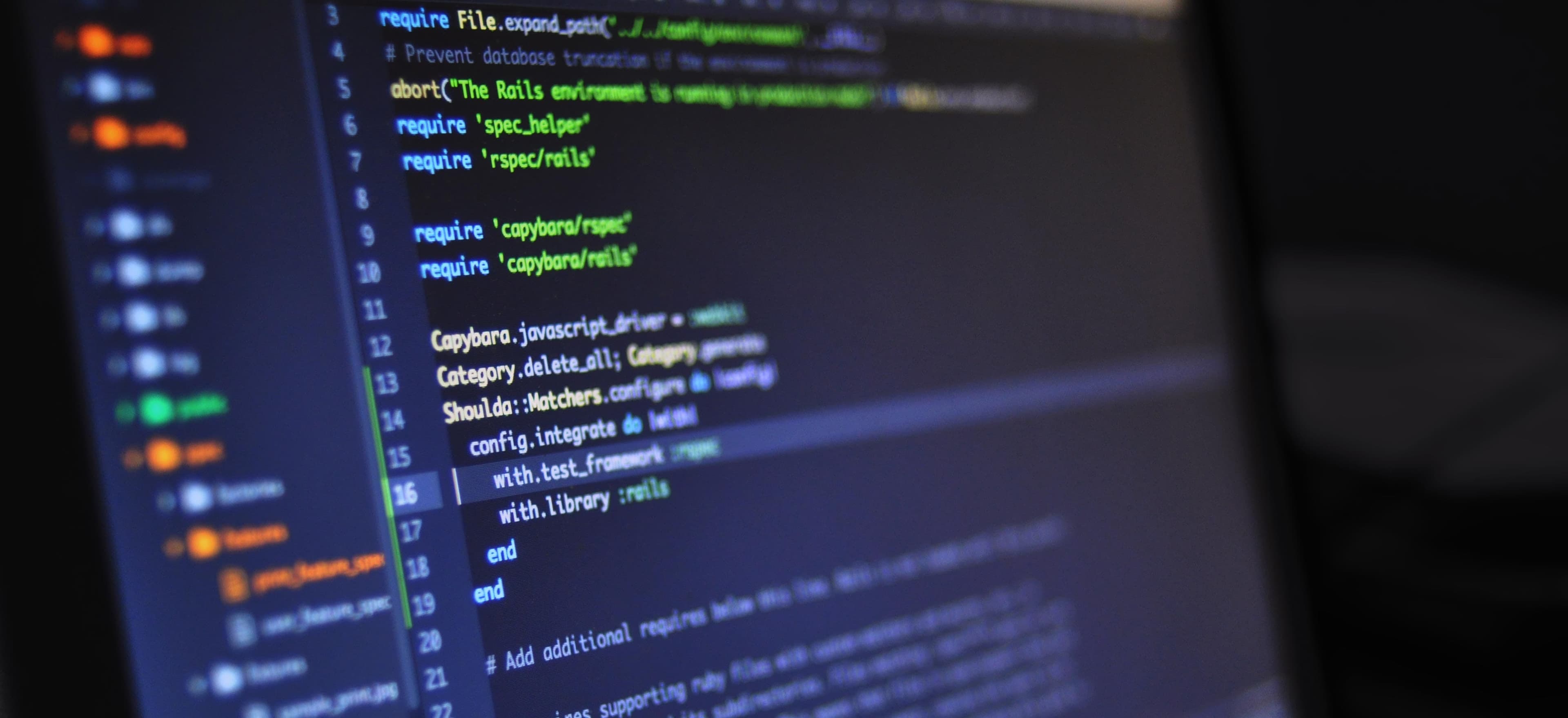
- Published on
Mastering Type Safety: Common Java Generics Pitfalls
Java has long been celebrated for its strong type system, which can prevent a wide array of programming errors. Central to this robustness are generics, introduced in Java 5, which enable developers to define classes, interfaces, and methods with type parameters. While generics promote code reusability and type safety, they also come with their set of challenges. In this post, we will explore some common pitfalls associated with Java generics and how to effectively navigate them.
What are Generics?
Before diving into pitfalls, let's clarify what generics are. Generics allow developers to specify a type (or types) as a parameter when creating classes, interfaces, or methods. For instance, the List<T>
class can store elements of any type, where T
is a placeholder for the data type (like Integer
, String
, etc.).
Here's a simple example:
import java.util.ArrayList;
import java.util.List;
public class GenericExample {
public static void main(String[] args) {
List<String> stringList = new ArrayList<>();
stringList.add("Hello");
stringList.add("Generics");
System.out.println(stringList);
}
}
In this code snippet, we create a List
that can only hold String
items. This restrictiveness is what generics are all about—type safety.
Common Pitfalls of Java Generics
1. Raw Types
One of the most common pitfalls is the use of raw types. A raw type is a generic class or interface without its type parameters, and it can lead to type safety violations. For example:
List rawList = new ArrayList(); // Raw type
rawList.add("Hello");
rawList.add(123); // No compile-time error, can lead to ClassCastException at runtime
String item = (String) rawList.get(0); // Safe to cast but can throw error if expected type is different
Why is this a problem?
Using raw types circumvents Java's type checking. The compiler cannot enforce type safety, leading to potential runtime exceptions. Always use generics to enhance type safety:
List<String> typedList = new ArrayList<>();
typedList.add("Hello");
// typedList.add(123); // Compile-time error
2. Unchecked Warnings
When you mix generics with legacy code, you may encounter unchecked warnings. These warnings appear when the compiler cannot guarantee that a generic type is used correctly.
List<String> myList = new ArrayList();
myList.add("Hello");
String item = myList.get(0); // Unchecked cast warning
Why is this a problem?
Unchecked warnings lead to uncertainty about data types. While your code may run without errors, it could fail unpredictably. To avoid this, always specify type parameters clearly. If you're transitioning legacy code, consider using @SuppressWarnings("unchecked")
judiciously, though it's preferable to refactor legacy code when possible.
3. Generic Arrays
Arrays in Java are covariant, meaning you can assign a subclass array to a superclass variable. However, this doesn’t translate seamlessly with generics.
List<String>[] stringLists = new List<String>[10]; // Compile-time error
Why is this a problem?
Creating arrays of generic types leads to type erasure issues and can create unsafe operations. Instead, you should use collections like ArrayList
:
List<List<String>> stringList = new ArrayList<>();
4. Type Erasure
Type erasure is a process by which the compiler removes all generic type information during compilation. The generics are replaced with their bounds or Object
if there are no bounds.
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
// After type erasure
public class Box {
private Object item; // T is replaced with Object
}
Why is this a problem?
Due to type erasure, information about type parameters is lost at runtime. This means you can't instantiate a generic type or create arrays of a parameterized type. Keep this in mind while designing API interfaces that use generics.
5. Wildcard Usage
Java supports wildcards (?
), which can generally be broken down into three categories:
- Unbounded Wildcards: Represent any type.
- Upper Bounded Wildcards: Restrict the unknown type to be a specific type or its subclasses.
- Lower Bounded Wildcards: Restrict the unknown type to be a specific type or its superclasses.
Why is this a problem?
In complex scenarios, the use of wildcards can become confusing, leading to clarity issues in the code. For instance:
public void processList(List<?> list) {
// Can read but cannot modify
Object item = list.get(0);
// list.add("test"); // Compile-time error
}
To alleviate confusion, use wildcards when necessary, but prefer explicit types wherever possible.
6. Type Inference Problems
Java uses type inference to deduce generics when instantiating objects based on the context. However, this can lead to errors if the context is not clear.
List<String> stringList = new ArrayList<>(); // Type inferred
var anotherList = new ArrayList<>(); // Type inferred but can lead to confusion
Why is this a problem?
Misunderstanding inferred types can create issues down the line. Be explicit about types, especially in public or complex APIs.
7. Mixing Generics with Other Types
While Java allows mixing generics with other raw types or collections, it can lead to incongruences.
List<String> stringList = new ArrayList<>();
List<Integer> integerList = (List<Integer>)(List<?>)stringList; // Raw-type casting
Why is this a problem?
This kind of casting can easily introduce ClassCastException at runtime. Always ensure that generics are kept homogeneous to prevent invalid data manipulations.
Best Practices for Using Generics
- Always Use Parameterized Types: Avoid raw types to maintain type safety.
- Prefer Lists over Arrays: Use collections instead of arrays for generic types.
- Be Explicit in Wildcard Usage: Avoid ambiguity by clearly defining when to use wildcards.
- Avoid Unchecked Operations: Minimize unchecked warnings by checking compatibility upfront.
A Final Look
Mastering generics in Java is pivotal for writing robust, maintainable, and type-safe code. While generics provide an excellent mechanism for type safety and code reusability, they can also introduce complexities if not carefully managed. By being mindful of the pitfalls discussed and adhering to best practices, you can effectively harness the power of generics in your Java applications.
For more information on generics, you can refer to the official Java documentation.
By learning and avoiding common pitfalls in generics, Java developers can create safer and cleaner code that stands the test of time. Have you encountered any other issues with generics in your Java projects? Feel free to share your experiences in the comments below!