Common Mistakes to Avoid When Building Map Applications
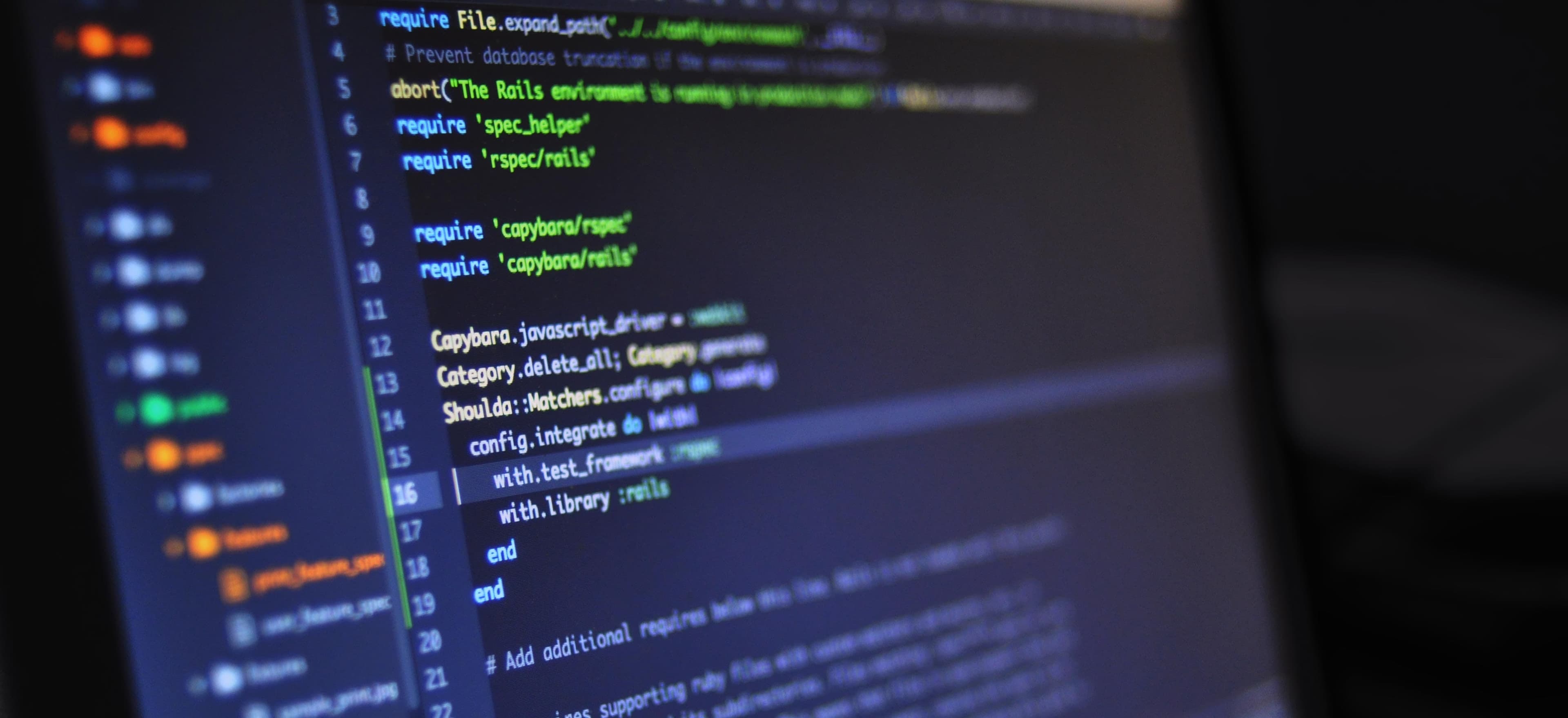
- Published on
Common Mistakes to Avoid When Building Map Applications
Building a map application can be a challenging task, filled with various complexities. As open-source technologies, frameworks, and APIs have evolved, developers now have a myriad of tools at their disposal. However, despite this wealth of resources, some developers still fall into common traps. In this blog post, we will discuss key mistakes to avoid when creating map applications, specifically focusing on Java-based solutions.
1. Neglecting User Experience
Importance of UX in Mapping
User experience (UX) is the cornerstone of any successful application, especially for map applications where clarity and usability are paramount. Focusing solely on functionality without considering the end-user may lead to a muddled interface.
Best Practices for UX
- Simplified Design: Ensure that the interface is uncluttered and intuitive.
- Responsive Layouts: Your application must perform well on both mobile and desktop platforms.
- Contextual Menus: Providing options in context will help users navigate easier.
Example of a Simplified Interface
JFrame frame = new JFrame("Simplified Map Application");
frame.setSize(800, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
MapView mapView = new MapView(); // A hypothetical class handling map view
mapView.setZoomLevel(10); // Setting a reasonable default zoom
frame.add(mapView); // Adding mapView to the frame
frame.setVisible(true); // Making the frame visible
This code demonstrates the creation of a simple window featuring a map view. It focuses solely on presenting the map without extra clutter to distract users.
2. Ignoring Performance Optimization
Why Performance Matters
A sluggish map application can alienate users quickly. If your application takes too long to load or responds slowly to interactions, it may lead to increased bounce rates.
Techniques for Enhancing Performance
- Asynchronous Loading: Load heavy components (like map tiles) asynchronously.
- Caching: Implement caching strategies to avoid redundant data requests.
- Optimized Rendering: Use techniques such as vector graphics for improved performance.
Example of Asynchronous Loading
SwingWorker<MapData, Void> worker = new SwingWorker<MapData, Void>() {
@Override
protected MapData doInBackground() throws Exception {
// Simulating the loading of map data
return loadMapData();
}
@Override
protected void done() {
try {
MapData mapData = get();
updateMapView(mapData); // Hypothetical method to refresh the view
} catch (Exception e) {
e.printStackTrace();
}
}
};
worker.execute(); // Start the worker thread
In this example, we use a SwingWorker
to load map data in the background so that the UI remains responsive while the data is fetched.
3. Misusing API Calls
The API Dilemma
Most map applications rely on third-party APIs for map tiles, locations, and other data. Developers often misuse these APIs, leading to unnecessary expenses and rate-limits being reached.
Strategies for Proper API Usage
- Understand Rate Limits: Always check the API's limits and adjust your application's behavior accordingly.
- Batch Requests: If your application needs multiple data points, batch those requests instead of making numerous individual ones.
- Use Efficient Queries: Make sure that your API queries are optimized to return only the necessary data.
API Request Example
URL url = new URL("https://maps.googleapis.com/maps/api/geocode/json?address=YOUR_ADDRESS&key=YOUR_API_KEY");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
try (BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()))) {
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
parseResponse(response.toString()); // Hypothetical method to handle response
}
This snippet demonstrates how to make an API call to Google's Geocoding API. It is important to manage connections properly to prevent memory leaks.
4. Overworking Data Layers
Complexity of Data Layers
Using multiple data layers is one way to enrich your maps with information. However, overloading your map can confuse users and decrease performance.
Guidelines for Managing Data Layers
- Prioritize Essential Data: Limit the layers based on user needs.
- Toggle Options: Provide users with the ability to toggle layers on and off for clarity.
- Load Layers on Demand: Instead of loading all layers at startup, consider loading them as needed.
Example of Layer Management
public void toggleLayer(Layer layer) {
if (layer.isVisible()) {
layer.setVisible(false);
} else {
layer.setVisible(true);
}
}
This method toggles the visibility of a map layer. It's essential to keep the interface clean and provide users control over the data displayed.
5. Failing to Handle Failures Gracefully
The Importance of Failures
No application is perfect, and network failures are common in map applications due to varying connectivity levels. Failing to handle these gracefully can frustrate users.
Handling Failures
- Provide Feedback: Inform users about issues clearly.
- Retry Logic: Implement simple retry mechanisms for transient errors.
- Graceful Fallbacks: Use cached data as a fallback when live data is unavailable.
Example of Handling Failures
try {
fetchMapData();
} catch (IOException e) {
showErrorMessage("Unable to fetch map data. Please try again later.");
// Optionally load cached data
}
Here, we gracefully handle exceptions by showing an informative message to the user, improving the overall user experience.
A Final Look
Building an effective map application involves more than just implementing a few features. It requires a keen understanding of user experience, performance optimization, and proper error handling, among other factors.
By avoiding these common mistakes—negligent user experience, performance issues, misusing APIs, overcrowding with data layers, and handling failures poorly—you can build a map application that not only serves its purpose but also delights users.
For further reading on this topic, you may find these resources helpful:
- Google Maps API Documentation
- Best Practices for UI/UX Design
- Asynchronous Programming in Java
Happy coding, and may your map applications be both powerful and user-friendly!
Checkout our other articles