Maximizing JVM Performance: Tackling JStat's Limitations
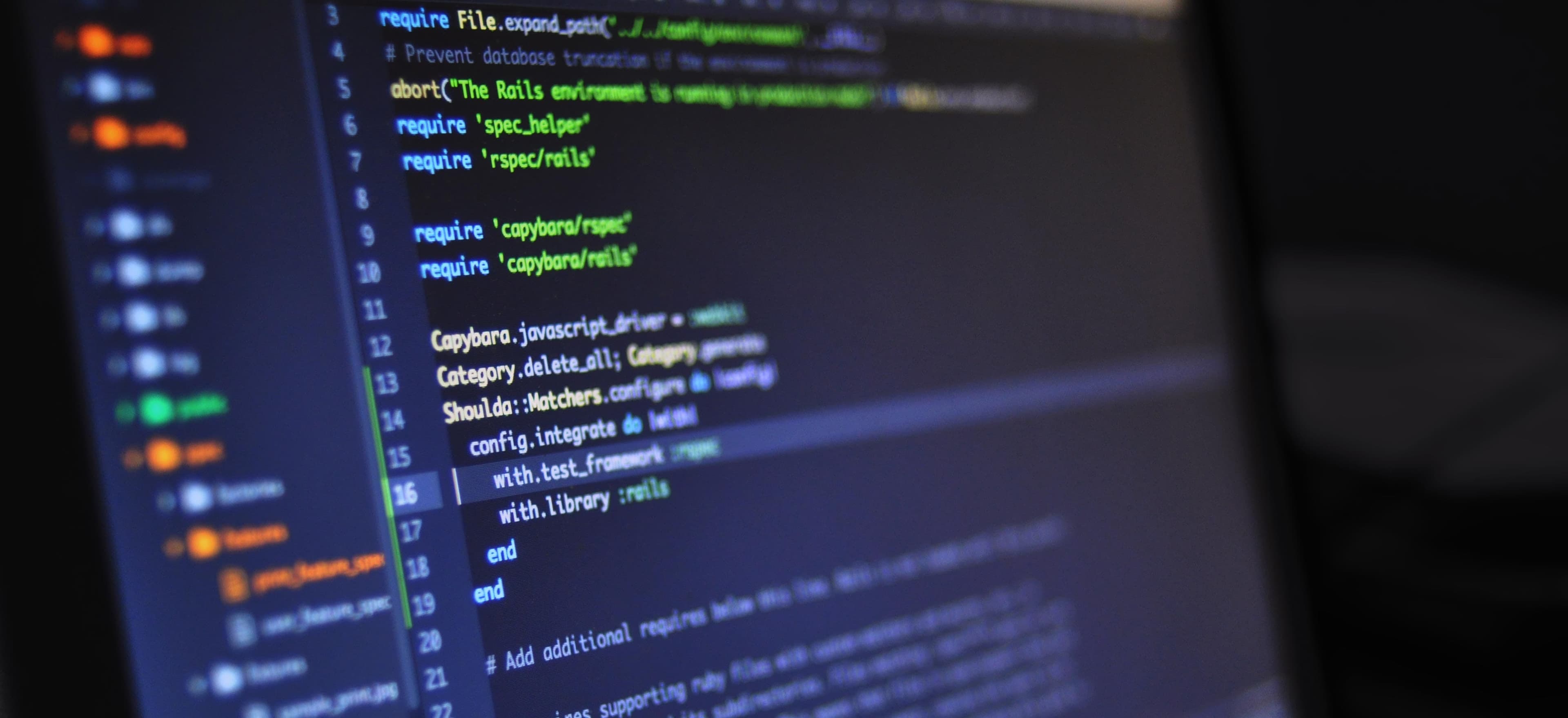
- Published on
Maximizing JVM Performance: Tackling JStat's Limitations
Java is widely recognized for its speed, scalability, and reliability. However, even the most optimized Java application can stumble if not monitored properly. This is where Java Virtual Machine (JVM) performance tools become vital. One such tool is JStat, which provides valuable statistics about the JVM. In this blog post, we will explore how to maximize JVM performance while addressing the limitations of JStat.
Understanding JStat and Its Role in JVM Monitoring
JStat is a command-line tool that comes with the Java Development Kit (JDK) and is used to monitor the performance of an application running on the JVM. It helps in gathering runtime statistics about memory, garbage collection, and the performance of Java applications. With real-time metrics, you can make informed decisions, allowing you to fine-tune your applications effectively.
Key Features of JStat:
- Memory Usage Metrics: Monitor heap memory usage, garbage collection statistics, and class loading performance.
- Performance Metrics: Keep an eye on compilation statistics and CPU utilization.
Here’s a simple command to start monitoring your Java application with JStat:
jstat -gc <pid> 1000
In this command:
-gc
indicates that you want garbage collection statistics.<pid>
should be replaced with your Java process ID.1000
is the delay in milliseconds between each output.
Why JStat Has Its Limitations
Despite its utility, JStat has its limitations. Here are a few constraints you may encounter when using it:
- Real-Time Monitoring: JStat collects data at set intervals, which may not provide a complete picture of transient issues.
- Lack of Detail: JStat presents aggregate data but lacks in-depth information concerning individual thread performance and resource utilization.
- Requires JMX: For some functionalities, you may need to enable Java Management Extensions (JMX), which adds complexity.
Alternative JVM Monitoring Tools
To complement JStat's capabilities and overcome its limitations, consider utilizing other monitoring tools.
- VisualVM: A powerful tool for monitoring and troubleshooting Java applications. It provides real-time profiling data, including thread management and heap dumps.
- Java Mission Control (JMC): Another advanced monitoring and profiling tool that offers insights based on JMX.
Choosing the right monitoring tool depends on your specific needs. Using multiple tools can give you a comprehensive view of your application’s performance.
Maximizing JVM Performance: Key Strategies
To ensure that your Java application runs smoothly and efficiently, consider the following strategies to maximize JVM performance:
1. Tuning JVM Parameters
Understanding and tuning JVM parameters can significantly affect performance. Here are common options:
- Heap Size: Set the maximum and initial sizes of the heap according to the application's memory consumption patterns.
java -Xms512m -Xmx2g -jar yourapp.jar
Here’s why you set these parameters:
-Xms
sets the initial heap size, allowing you to avoid allocation delays.-Xmx
sets the maximum heap size, preventing the application from consuming all system memory, which can lead to crashes.
2. Java Garbage Collection (GC) Optimization
Garbage Collection is a critical process in JVM management. Optimizing it can yield better performance, particularly in long-running applications. Different GC algorithms, such as the G1GC or ZGC, may suit various applications.
For example, enabling G1GC can improve performance for applications with large heaps:
java -XX:+UseG1GC -jar yourapp.jar
Choosing the Right Garbage Collector
It's crucial to choose the right garbage collector based on the application requirements:
- Serial GC: Best for small applications with low memory requirements.
- Parallel GC: Suitable for applications that need high throughput.
- Concurrent Mark-Sweep (CMS): A good choice if you need shorter pause times.
- G1GC: Recommended for applications with large heaps.
3. Profiling and Benchmarking
Before making changes, it’s essential to profile and benchmark your application. This allows you to understand bottlenecks and areas that need improvement. Tools like JMH (Java Microbenchmark Harness) can help in this regard.
Here’s a basic example of using JMH to benchmark a function:
import org.openjdk.jmh.annotations.*;
import java.util.concurrent.TimeUnit;
public class MyBenchmark {
@Benchmark
@OutputTimeUnit(TimeUnit.MILLISECONDS)
public void testMethod() {
// Code you want to benchmark
}
}
By running this benchmark, you'll find out how long the method takes to execute, providing a baseline for optimization efforts.
4. Effective Use of Threads
Java applications often rely heavily on multi-threading. Properly managing threads can lead to better performance:
- Thread Pools: Utilize thread pools instead of creating new threads for each task. This reduces overhead and improves resource utilization.
Example of using an ExecutorService for thread management:
ExecutorService executor = Executors.newFixedThreadPool(10); // Create a pool of 10 threads
executor.submit(() -> {
// Code to execute
});
executor.shutdown();
5. Optimize I/O Operations
Input/Output operations can be a significant bottleneck. Using buffered I/O and asynchronous mechanisms can help.
Example of using BufferedReader for efficient reading:
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
// Process the line
}
}
This code leverages buffering, allowing for more efficient reads compared to standard I/O operations.
6. Avoiding Memory Leaks
Memory leaks can drain valuable resources and hurt performance. Use memory profiling tools like VisualVM to detect leaks early. The following practices can help:
- Remove unnecessary references.
- Use weak references where applicable.
The Closing Argument: Mastering JVM Performance
Performance tuning is both an art and a science. Understanding JStat's limitations, coupled with the strategies listed above, can significantly enhance JVM performance. Remember that optimizing one aspect of your application may inadvertently affect others.
Regular profiling, continuous monitoring, and adjusting JVM parameters are the keys to maintaining a high-performing Java application.
For further reading, consider:
- Java Performance Tuning: The Definitive Guide by Rajesh Nadkarni
- Effective Java by Joshua Bloch
By proactively managing your application's performance, you ensure a smoother and more efficient operation, paving the way for excellent user experiences. Happy coding!
Checkout our other articles