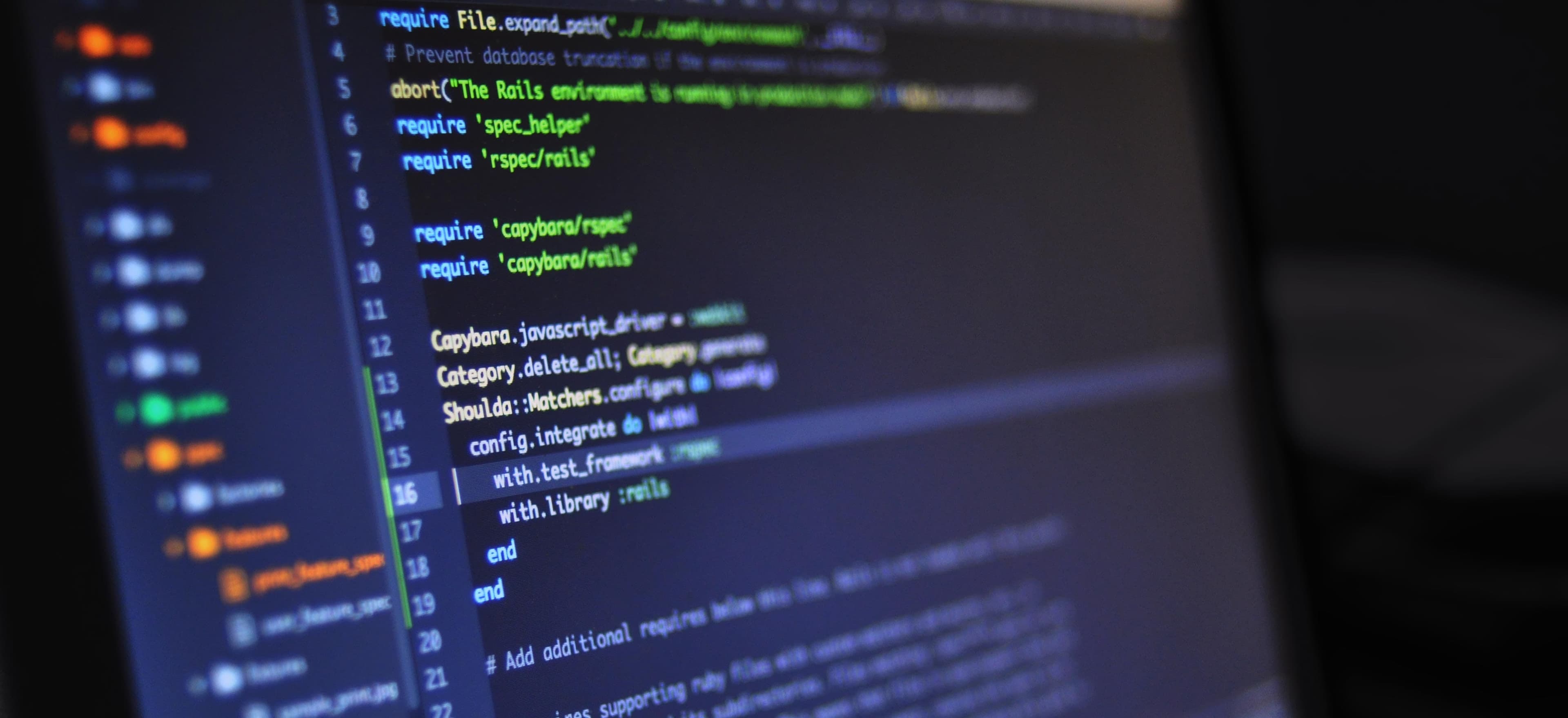
- Published on
Mastering Numeric Range Formatting in Choice Formats
When developing desktop or web applications, one often encounters scenarios where numeric values need to be formatted consistently. Abiding by formatting standards can enhance user experience while ensuring that data is both accurate and adheres to cultural expectations. In this article, we will delve into best practices for numeric range formatting in Choice Formats using Java, providing examples to illustrate key points along the way.
Understanding Numeric Ranges
Before we dive into specifics, it’s crucial to understand what we mean by numeric ranges. Numeric ranges indicate a span of values between a minimum and maximum. For example, "Age: 0-100" defines a range of acceptable values from 0 to 100.
Numeric ranges become particularly important when:
- Collecting User Input: Gather input that falls within specified limits.
- Displaying Data: Presenting values within a meaningful context, such as beauty products that may cater to a certain range of skin types.
- Validation: Ensuring that inputted values do not exceed expected parameters.
Why Use Java for Numeric Range Formatting?
Java is a robust, widely-used programming language known for its versatility and strong support for enterprise-level applications. Its rich set of libraries and frameworks makes it an ideal choice for handling numeric data, particularly when it involves formatting and validation.
Java's Formatting Capabilities
Java provides several mechanisms to format numbers effectively. The NumberFormat
class is part of the java.text
package and is remarkably useful for formatting currencies, percentages, and other numeric ranges.
Example: Basic Numeric Formatting
Let’s consider a simple example where we want to format a range of numbers that represent scores:
import java.text.NumberFormat;
public class NumericFormatter {
public static void main(String[] args) {
double minScore = 75.5;
double maxScore = 95.25;
NumberFormat formatter = NumberFormat.getNumberInstance();
formatter.setMaximumFractionDigits(2);
String formattedRange = "Scores: " + formatter.format(minScore) + " - " + formatter.format(maxScore);
System.out.println(formattedRange);
}
}
Commentary on the Code
-
Importing Required Classes: We start by importing the
NumberFormat
class, which provides the functionality for formatting. -
Declaring Variables: The
minScore
andmaxScore
variables hold the score data. -
Creating a Formatter Instance: We create an instance of
NumberFormat
to handle formatting numbers and set the maximum fraction digits to 2 to limit decimal points. -
Formatting the Range: By concatenating the formatted minimum and maximum scores, we create a user-friendly string that represents the score range.
This simple yet effective example showcases how to format numeric ranges using Java.
Numeric Range in Choice Formats
In UI applications, numeric ranges often translate into selectors or input fields. Real-world use cases include forms where users choose a range for age, prices, or other criteria. Ensuring that the user selects values within a defined range enhances usability and input integrity.
Let’s look at how we can create a function to validate a user's selection against these ranges, coupled with a choice format.
public class RangeValidator {
public static boolean isValidAge(int age) {
int minAge = 0;
int maxAge = 100;
return age >= minAge && age <= maxAge;
}
public static void main(String[] args) {
int testAge1 = 25; // Valid age
int testAge2 = 120; // Invalid age
System.out.println("Is " + testAge1 + " valid? " + isValidAge(testAge1));
System.out.println("Is " + testAge2 + " valid? " + isValidAge(testAge2));
}
}
Commentary on the Validation Code
-
Function Purpose: The
isValidAge
function checks whether a given age falls within the accepted range (0 to 100 inclusive). -
Logical Expression: The return statement applies a straightforward logical condition to verify the age. If the age is equal to or greater than the lower limit and less than or equal to the upper limit, the function returns
true
. -
Testing Validity: In the
main
method, we test the function with both a valid and invalid age, validating our logic.
This example demonstrates how to ensure that user inputs meet established criteria and provides a smooth and guided experience when selecting numeric ranges.
Real-World Application of Numeric Ranges in Java
Studying numeric ranges in Java leads to practical applications across various fields. For example, online marketplaces may require price ranges for product searches, while educational platforms might analyze student performance metrics based on grade ranges.
Advanced Formatting Techniques
With basic formatting and validation in place, we can explore more advanced techniques to enhance our applications. The Formatter
class offers a more flexible, albeit complex, means of formatting numbers.
Example: Custom Formatting with the Formatter Class
import java.util.Formatter;
public class CustomFormatter {
public static void main(String[] args) {
double price = 1234.5678;
StringBuilder sb = new StringBuilder();
Formatter formatter = new Formatter(sb);
formatter.format("Formatted Price: $%,.2f", price);
System.out.println(sb.toString());
}
}
Commentary on Custom Formatting
-
StringBuilder for Performance: By using a
StringBuilder
, we optimize memory use when concatenating strings. -
Formatter to Structure Output: The
Formatter
lets us define a custom pattern. In this situation, we format a number as currency, including thousands separators and limiting it to two decimal places. -
Output: The result is a neatly formatted price that is easy to read and understand.
To Wrap Things Up
Mastering numeric range formatting in choice formats using Java is a vital skill for developers looking to enhance user inputs and presentation of numerical data. We examined the basics through validation functions, simple formatting, and custom formatting techniques, while emphasizing the importance of user experience when dealing with numeric ranges.
As a professional, your ability to apply these techniques can greatly improve the fidelity of the applications you build. By ensuring that numeric ranges are formatted correctly, developers can maintain integrity and usability across systems.
The distinct examples provided in this guide serve to equip you with the necessary tools to handle numeric ranges effectively. While the world of Java is vast, understanding these principles forms a strong foundation for further exploration.
Stay curious, and keep developing!
This blog post provides essential information about numeric range formatting in a clear and concise manner, suitable for a Java developer audience seeking to refine their skills.
Checkout our other articles