Struggling to Use Private Methods in Java 8 Interfaces?
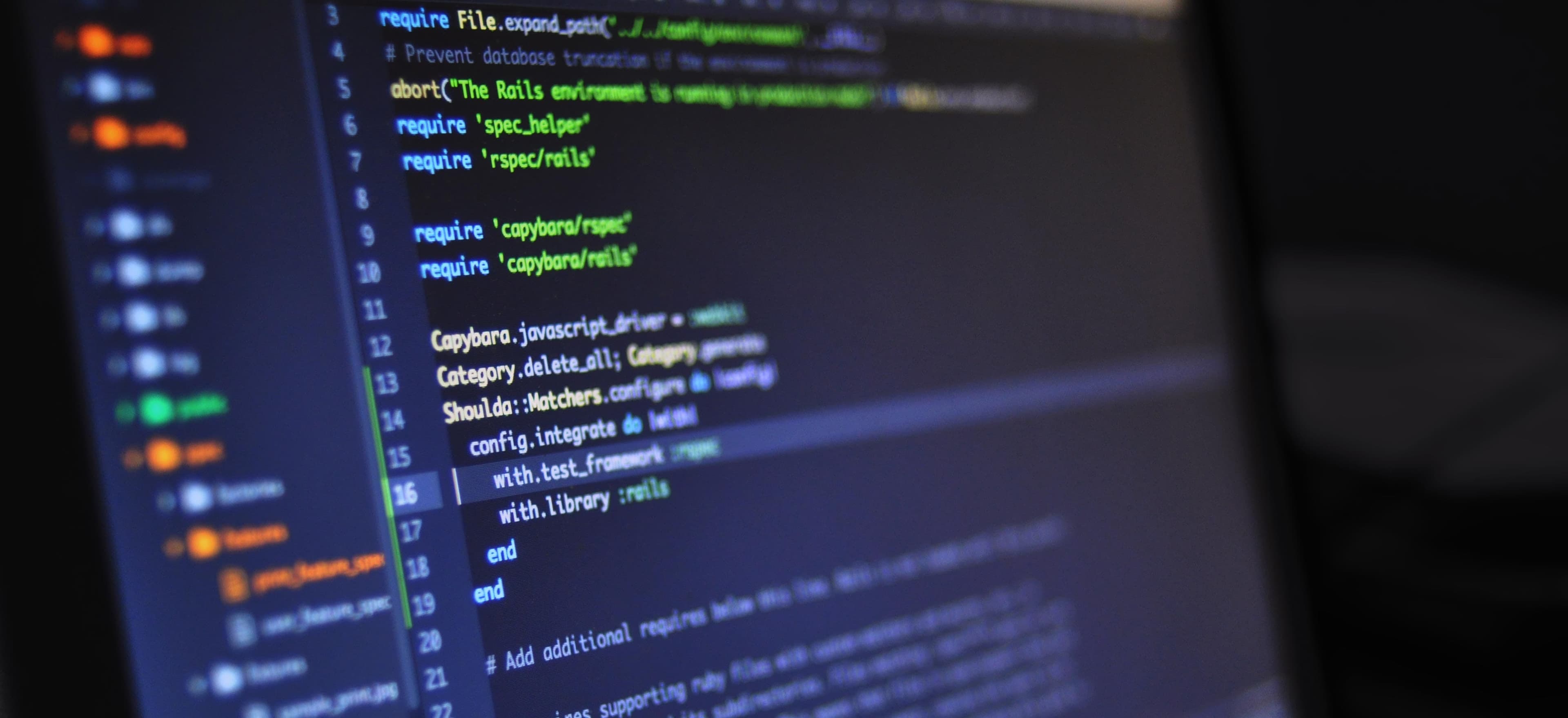
- Published on
Struggling to Use Private Methods in Java 8 Interfaces?
As developers working in Java, we often face the limitation of what we can achieve with interfaces. Traditionally, interfaces were a way to define a contract for classes to implement without any specific behavior. However, with the introduction of Java 8, we witnessed a significant enhancement to interfaces through the inclusion of default methods. These default methods allowed some level of implementation within the interface itself.
But the introduction of private methods in Java 9 truly revolutionized how we could leverage interfaces. If you are struggling to utilize private methods within interfaces, you're not alone. This post aims to simplify the concepts and provide concrete examples to clarify their applications.
What Are Private Methods in Interfaces?
Private methods in interfaces serve to share code between default methods. They allow you to encapsulate common functionality without exposing it to external classes. This means you can write cleaner and more maintainable code, eliminating redundancy.
Example: Understanding Private Methods
Let’s scratch the surface with an example. We will define an interface with default and private methods.
public interface MyInterface {
// Default method
default void greet(String name) {
String greetingMessage = createGreeting(name);
System.out.println(greetingMessage);
}
// Private method shared among default methods
private String createGreeting(String name) {
return "Hello, " + name + "!";
}
}
Explanation of the Code
-
Default Method: The
greet
method is a default method that any class implementing this interface can override. It has a simple role—greeting a user. -
Private Method:
createGreeting
is a private method. Its sole purpose is to generate a greeting message. Notice how it is not accessible directly from outside the interface, which encapsulates the functionality away from the user. This promotes code reuse within the interface itself.
By adopting the private method, you avoid duplicating the greeting logic if you have multiple default methods within the same interface.
Why Use Private Methods?
-
Code Reusability: You write once, and it’s available everywhere within the interface, enhancing the DRY (Don’t Repeat Yourself) principle.
-
Encapsulation: By keeping shared functionality private, you provide only the necessary methods to implementors, avoiding confusion and potential misuse.
-
Clean Interfaces: It allows interfaces to be more straightforward and focused, improving code readability.
Another Practical Use Case: Calculating Tax
Let’s take a more practical example where private methods help us calculate tax in multiple default implementations.
public interface TaxCalculator {
// Default method for calculating income tax
default double calculateIncomeTax(double income) {
return calculateTax(income, 0.2); // 20% tax rate
}
// Default method for calculating capital gains tax
default double calculateCapitalGainsTax(double gains) {
return calculateTax(gains, 0.15); // 15% tax rate
}
// Private method to avoid code duplication
private double calculateTax(double amount, double rate) {
return amount * rate;
}
}
Explanation of the Tax Example
-
Default Tax Calculation: The
calculateIncomeTax
andcalculateCapitalGainsTax
are both default methods that provide specialization for different kinds of tax calculations but share the underlying calculation logic. -
Shared Logic: The private method
calculateTax
encapsulates the logic for calculating the tax based on the provided rate. This prevents duplication of logic for tax calculation across different methods.
Implementing the Interface
Let’s see a concrete class implementing this interface:
public class TaxService implements TaxCalculator {
public static void main(String[] args) {
TaxService service = new TaxService();
System.out.println("Income Tax: " + service.calculateIncomeTax(50000));
System.out.println("Capital Gains Tax: " + service.calculateCapitalGainsTax(15000));
}
}
Output
Income Tax: 10000.0
Capital Gains Tax: 2250.0
Key Takeaways from this Implementation
-
Instantiating the Class: The
TaxService
class implements theTaxCalculator
interface and can use the defined default methods seamlessly. -
Clean Usage: The external user of
TaxService
does not need to know how the tax calculation is implemented; they just invoke the appropriate method.
Closing Remarks: Mastering Private Methods in Java Interfaces
In summary, private methods in interfaces introduced in Java 9 offer an excellent way to encapsulate shared logic without exposing it to the outside world. By using private methods, you can:
- Enhance code reusability and maintainability.
- Keep your interfaces cleaner and clearer.
- Promote encapsulation principles in your code.
If you are interested in understanding more about interfaces and their features, consider exploring the Java Documentation for more details.
Additional Resources
Also, if you're looking to deepen your understanding, you may find these resources helpful:
- Effective Java by Joshua Bloch
- Java's Interface Documentation
- Understanding Java Default Methods.
This exploration into private methods within interfaces indicates that Java is evolving to maintain good design principles in the ever-changing landscape of application development. If you have any further questions or would like deeper discussions on this topic, feel free to leave a comment!
Checkout our other articles