Troubleshooting Dockerized Java Apps with Solr and MongoDB
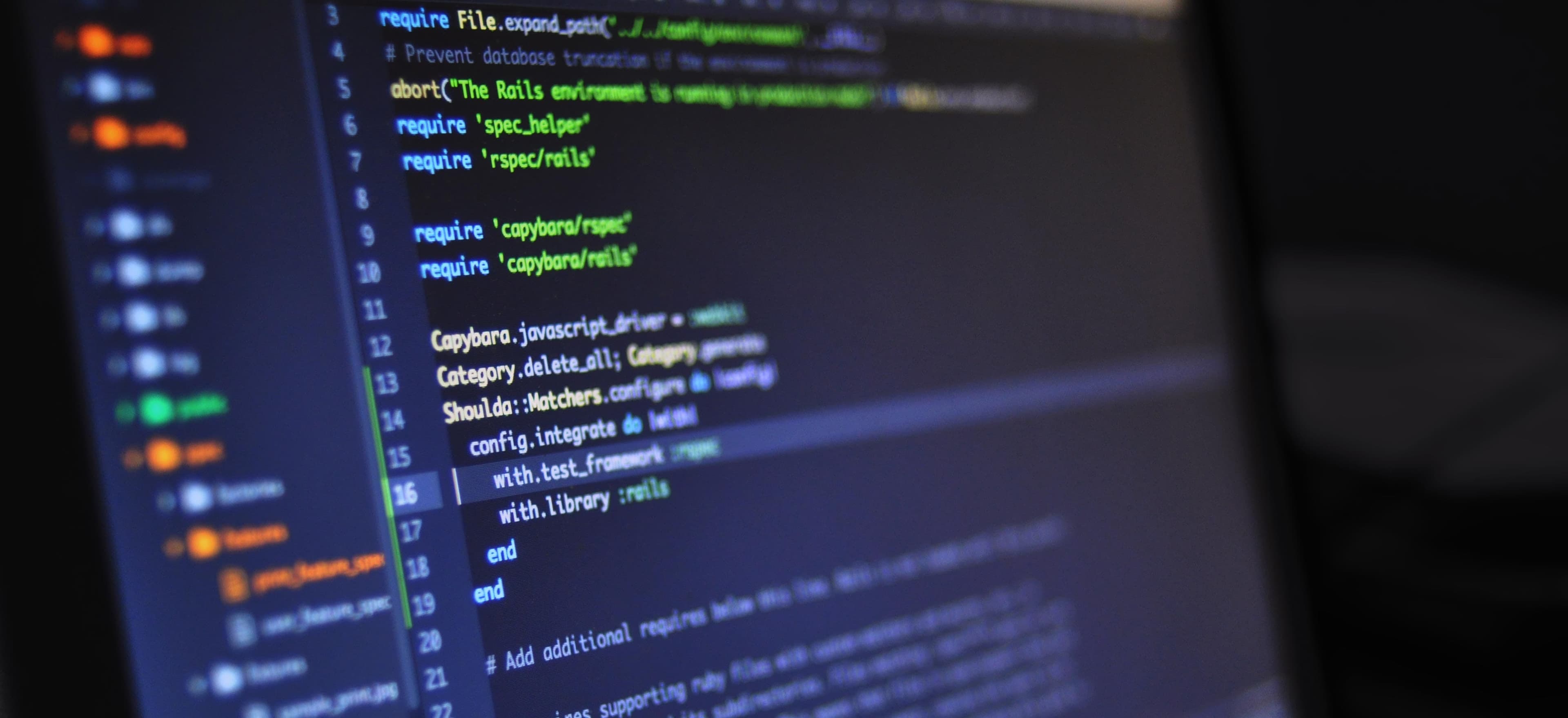
- Published on
Troubleshooting Dockerized Java Apps with Solr and MongoDB
In today's fast-paced development environment, Java developers often turn to containerization for deploying applications. Docker enables seamless deployment and scaling, but troubleshooting can become daunting, especially when multiple services like Solr and MongoDB are involved. This guide will help you navigate common issues, utilizing Docker effectively to troubleshoot your Java applications.
Why Use Docker with Java, Solr, and MongoDB
Docker provides consistent environments that reduce the "it works on my machine" syndrome. By containerizing Java applications along with their dependencies like Solr and MongoDB, you ensure that your app runs smoothly across all environments—development, staging, and production.
Benefits of This Approach
- Isolation: Each service runs in its container.
- Scalability: Services can be scaled horizontally by deploying more containers.
- Reproducibility: Docker images can be version-controlled, allowing easy rollback if needed.
Setting the Stage
In this tutorial, we will consider a simple scenario where a Dockerized Java application interacts with Solr for search capabilities and MongoDB for data storage. Below is the basic structure of our docker-compose.yml
file:
version: '3.8'
services:
java-app:
build: ./java-app
ports:
- "8080:8080"
depends_on:
- mongo
- solr
mongo:
image: mongo:latest
ports:
- "27017:27017"
solr:
image: solr:latest
ports:
- "8983:8983"
In this configuration, we define three services: a Java application, MongoDB, and Solr. The depends_on
directive ensures that MongoDB and Solr are up before the Java application starts.
Common Issues and Troubleshooting Steps
Issue 1: App Fails to Connect to MongoDB
One of the most frequent issues encountered is the inability of the Java app to connect to MongoDB.
Possible Causes
- MongoDB is not ready when the Java application starts.
- Incorrect connection string or host configuration.
Fixes
To ensure MongoDB is up before the Java service starts, consider adding a wait mechanism in your Java code or configuring a retry strategy. You can modify your application to keep retrying the connection until it succeeds:
import com.mongodb.MongoClient;
import com.mongodb.MongoClientURI;
public class MongoConnection {
private static final String uri = "mongodb://mongo:27017";
public static void main(String[] args) {
connectWithRetry();
}
public static void connectWithRetry() {
while (true) {
try (MongoClient mongoClient = new MongoClient(new MongoClientURI(uri))) {
// Your code to access database
System.out.println("Connected to MongoDB");
break; // Exit loop on success
} catch (Exception e) {
System.out.println("Failed to connect to MongoDB. Retrying...");
try {
Thread.sleep(2000); // Wait before retrying
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
}
}
This code attempts to connect to MongoDB, and if it fails, it waits for 2 seconds before trying again.
Issue 2: Solr Is Not Accepting Requests
Another common trouble is when Solr fails to accept requests due to improper setup or configuration.
Possible Causes
- Solr collection not created.
- Incorrect API endpoint or core selection.
Fixes
Before you start your Java application, ensure you've created a Solr collection. You can create a collection using the following command:
docker exec -it <solr_container_id> solr create -c example_collection
Ensure that the collection is named correctly in your Java application code and update any API requests accordingly.
You can follow Solr's official documentation for setting up and managing collections.
Issue 3: Performance Bottlenecks
Performance issues can arise when interacting with databases or when the application logic isn't optimized.
Causes
- Inefficient queries.
- Non-optimal indexing in Solr and MongoDB.
Solutions
- Use proper indices in both MongoDB and Solr to speed up queries.
- Profile your Java application using tools like VisualVM or YourKit.
In MongoDB, ensure that you create indexes as follows:
MongoCollection<Document> collection = database.getCollection("example_collection");
collection.createIndex(Indexes.ascending("field_name"));
In Solr, you can optimize your schema to make indexing faster. Follow best practices by referring to the Apache Solr documentation.
Debugging Tips
-
Logs: Always check logs for all services. Use Docker's logging commands to access logs quickly:
docker-compose logs <service_name>
-
Networking: Ensure that containers communicate correctly. Since services share the same network by default, check firewall and IP settings.
-
Service Health: Use health checks in your Docker Compose file to ensure services are running as expected before other containers start.
Example for MongoDB with health checks:
mongo:
image: mongo:latest
ports:
- "27017:27017"
healthcheck:
test: ["CMD", "mongo", "--eval", "db.runCommand({ ping: 1 })"]
interval: 30s
timeout: 10s
retries: 5
The Closing Argument
Dockerizing your Java applications with Solr and MongoDB can streamline development and deployment. However, troubleshooting is critical for maintaining performance and reliability. Always ensure that your containers are properly configured and communicate effectively.
Remember to utilize logging and monitoring tools to catch issues early on, and don't hesitate to consult the respective documentation for Solr and MongoDB. For further reading, consider the following links:
By adhering to these practices, you can effectively troubleshoot your Dockerized Java app and maintain a robust application. Happy coding!