Overcoming Backpressure Challenges in MicroProfile Bulkheads
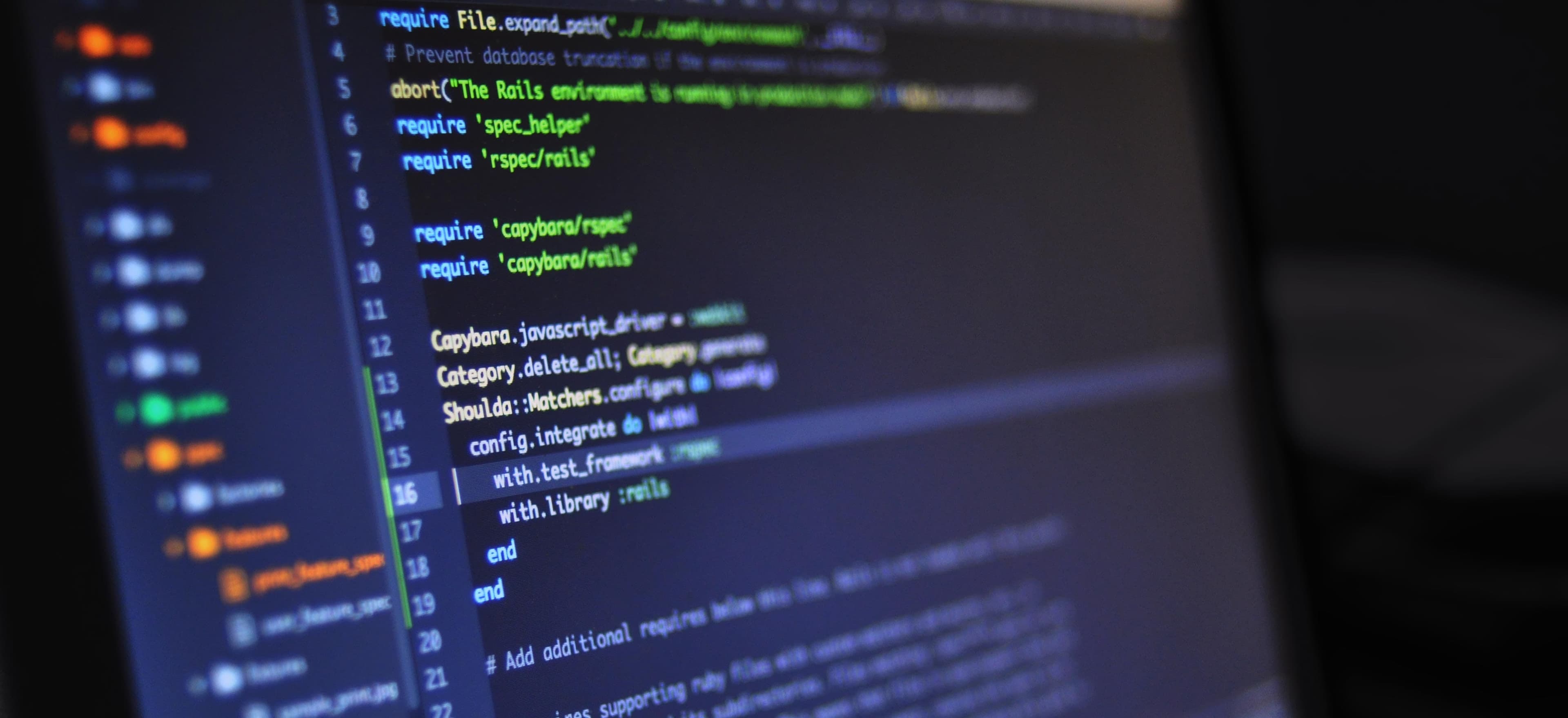
- Published on
Overcoming Backpressure Challenges in MicroProfile Bulkheads
In microservices architecture, where resilience and scalability are paramount, handling resource constraints gracefully is a key concern for developers. One innovative solution that has surfaced in this domain is the use of Bulkheads. Adopted from maritime engineering, bulkheads help manage the flow of resources in a way that maximizes service availability. However, this approach often invites backpressure challenges that can affect a microservice's performance. In this blog, we will explore how to implement MicroProfile Bulkheads effectively and how to overcome the ensuing backpressure challenges.
What is Backpressure?
Simply put, backpressure is a signal to a system that it is overwhelmed by incoming data or requests. In the context of microservices, this indicates that one service cannot handle the volume of requests it receives and needs to slow down or perhaps deny incoming requests to maintain overall system stability. This can lead to increased latency, request failures, and a poor user experience.
Why Use Bulkheads?
Bulkheads in microservices help isolate problems so that one failing component doesn’t cascade and bring down the entire system. By partitioning different functionality into separate pool-like structures, microservices can maintain resilience. The added advantage of Bulkheads comes from the MicroProfile specification, which allows developers to implement patterns like timeouts, retries, and circuit breakers in a coherent manner.
Setting Up MicroProfile Bulkheads
Implementing MicroProfile Bulkheads is straightforward. Below is a simple demonstration of how to set up Bulkheads in a Java application.
Step 1: Adding Dependencies
Ensure that you include the required MicroProfile libraries in your pom.xml
or build.gradle file. Here is an example for Maven:
<dependency>
<groupId>org.eclipse.microprofile.fault-tolerance</groupId>
<artifactId>microprofile-fault-tolerance-api</artifactId>
<version>3.0</version>
</dependency>
Step 2: Implementing a Bulkhead
Here’s how you might use a Bulkhead to isolate an operation in your Java EE service:
import javax.enterprise.context.ApplicationScoped;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import org.eclipse.microprofile.faulttolerance.Bulkhead;
@Path("/service")
@ApplicationScoped
public class MyService {
@GET
@Path("/process")
@Bulkhead(value = 5, waitingTaskQueue = 10)
public String process() {
// Simulate processing time
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Processed Successfully";
}
}
Explanation of the Code
- @Bulkhead: This annotation configures the number of concurrent threads permitted and the task queue size. Here, up to 5 requests can be processed simultaneously, and a queue can accommodate 10 waiting tasks.
- Thread.sleep(2000): This simulates a delayed operation, thereby allowing you to test how your service behaves under bulkhead conditions.
Step 3: Testing the Bulkhead Implementation
To test your Bulkhead implementation, you can send several requests to /service/process
. You will observe how additional requests are queued and how some may ultimately fail or timeout if the queue also fills up. This approach renders insight into how bulkheads manage backpressure scenarios.
Addressing Backpressure Challenges
While Bulkheads minimize the risk of cascading failures, they may introduce challenges, particularly backpressure. There are several strategies to effectively deal with these challenges:
1. Rate Limiting
Implement rate limiting to control the number of incoming requests. This can be done using a combination of APIs such as Resilience4j or built-in rate-limiting features in your server configuration.
import org.eclipse.microprofile.faulttolerance.RateLimit;
@GET
@Path("/rateLimitedProcess")
@Bulkhead(value = 5, waitingTaskQueue = 10)
@RateLimit(value = 5, period = 1, limit = 10)
public String rateLimitedProcess() {
// Simulate task processing
return "Processed with Rate Limit";
}
2. Fallback Mechanisms
Use fallback mechanisms to provide alternative responses in cases where backpressure leads to timeouts or failures. This is particularly critical when ensuring user experience remains intact.
import org.eclipse.microprofile.faulttolerance.Fallback;
@GET
@Path("/fallbackProcess")
@Bulkhead(value = 5, waitingTaskQueue = 10)
@Fallback(fallbackMethod = "fallbackResponse")
public String fallbackProcess() {
// Simulate a process that might fail
throw new RuntimeException("Intentional Failure");
}
public String fallbackResponse() {
return "Fallback Response - Service is busy. Please try again later.";
}
3. Metrics and Monitoring
Implementing metrics and monitoring allows you to track failures, queued requests, and processing times. This insight helps in adjusting Bulkhead sizes and parameters, ensuring they reflect the current load.
Best Practices When Implementing Bulkheads
- Isolate Critical Services: Identify which services most require isolation and implement bulkheads accordingly to prevent failures from affecting other services.
- Adjust Configuration Dynamically: Based on observed metrics, consider fine-tuning the values for concurrent requests and waiting tasks.
- Test Extensively: Before deploying changes, test extensively in your staging environment to gauge system responses under different load scenarios.
Closing the Chapter
Overcoming backpressure challenges in MicroProfile bulkheads is no small feat but is entirely manageable with the right strategies. By leveraging Bulkheads, you can enhance the resilience of your microservice-based applications while carefully managing the impacts of backpressure.
Utilizing techniques like rate limiting, implementing fallback methods, and continually monitoring metrics empowers developers to maintain optimal user experiences, even under strain.
For further reading on MicroProfile concepts, check out MicroProfile Fault Tolerance and Java Microservices to gain a broader understanding of how these technologies can work together for robust applications.
With a comprehensive grasp on bulkheads and backpressure management, you can build resilient microservices that not only function effectively but also scale gracefully under load. Happy coding!