Common Pitfalls in Integration Testing with Gradle
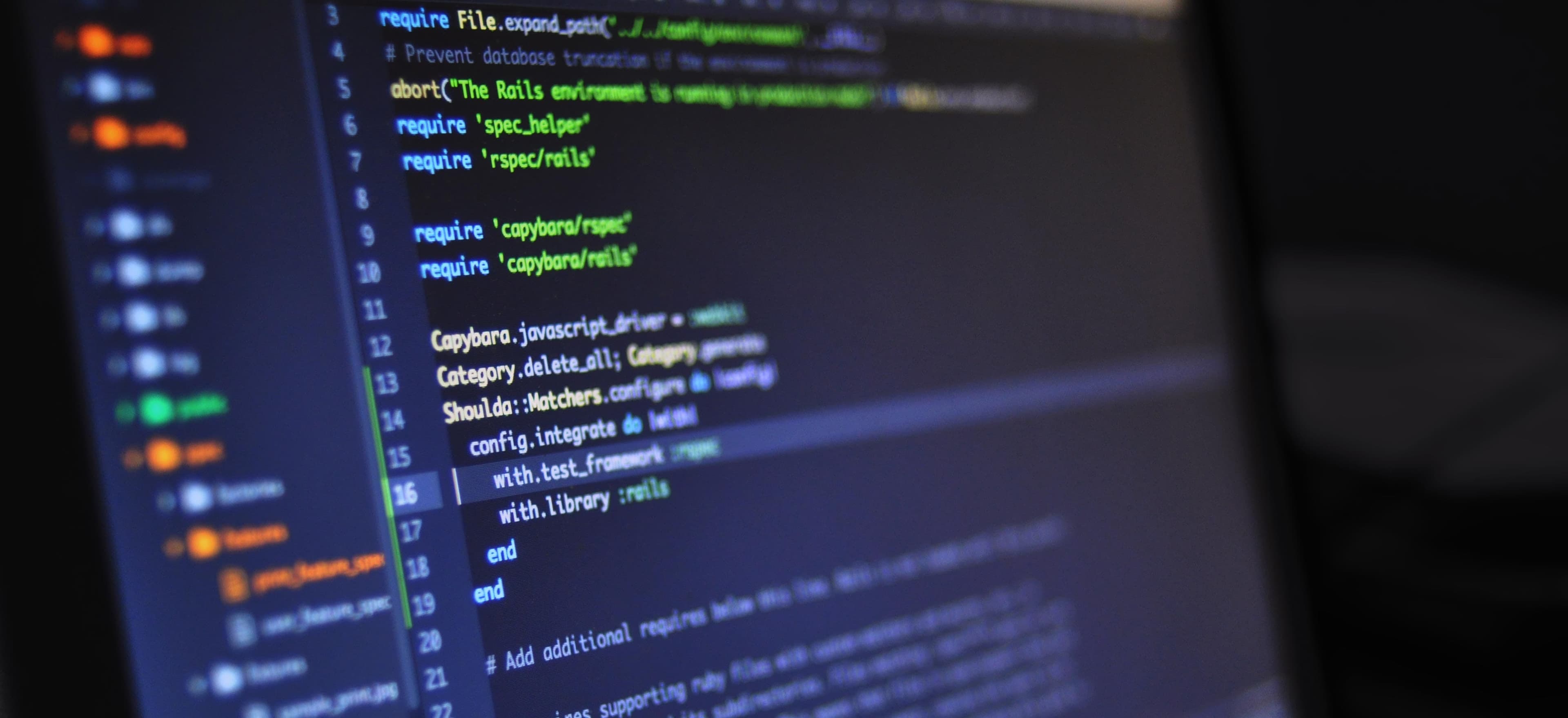
- Published on
Common Pitfalls in Integration Testing with Gradle
Integration testing is a crucial phase in the software development lifecycle. It ensures that various components of an application work together seamlessly. However, when conducted incorrectly, integration tests can lead to misleading results, wasted resources, and increased debugging times. Gradle, a powerful build automation tool, is widely used for managing dependencies, compiling code, and running tests. In this post, we will explore some common pitfalls in integration testing with Gradle and how to avoid them.
1. Improper Test Configuration
The Importance of Configuration
One of the most common mistakes developers make is improper test configuration. Gradle allows developers to configure test tasks through various means, but without proper setup, the tests may not run as expected.
Example
task integrationTest(type: Test) {
description = 'Runs integration tests.'
// Ensure integration tests are in a specific source set
testClassesDirs = sourceSets.integrationTest.output.classesDirs
classpath = sourceSets.integrationTest.runtimeClasspath
}
Commentary
In the code above, we create a integrationTest
task that is intended to run integration tests. Setting testClassesDirs
and classpath
correctly is crucial. Failing to do so can result in the task not locating the test classes or the necessary dependencies, leading to runtime failures.
Solution
Always ensure that your tests are correctly referenced:
- Check Source Sets: Make sure you define an appropriate source set for your integration tests.
- Use Gradle's Built-in Features: Utilize Gradle's built-in capabilities for task configuration, allowing clearer organization of test types.
2. Ignoring Dependency Management
The Role of Dependencies
Managing dependencies correctly is vital for executing integration tests without issues. Some tests may rely on specific libraries or frameworks, and of course, application dependencies must also be present.
Example
dependencies {
integrationTestImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.1'
integrationTestRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.8.1'
// Add any other necessary dependencies
}
Commentary
By maintaining our dependency setup for integration tests, we ensure all required libraries are available when tests execute. Neglecting to add test implementation dependencies may result in NoClassDefFoundError or similar problems.
Solution
- Group Dependencies: Always group your integration test dependencies under a designated configuration.
- Scope Management: Use
runtimeOnly
,implementation
, ortestImplementation
wisely, reflecting the role of each dependency.
3. Neglecting Environment Variability
The Impact of Environment Changes
Integration tests may behave differently in various environments, such as local machines versus CI/CD pipelines. Failing to account for this variability can cause tests to pass locally while failing in production-like environments.
Example
task integrationTest(type: Test) {
// ...
systemProperty 'DB_URL', project.hasProperty('DB_URL') ? project.DB_URL : 'jdbc:h2:mem:testdb'
}
Commentary
In the example, we set a system property for the test environment. This property allows us to define a database connection only if DB_URL
is provided, ensuring tests have the right configuration based on the environment.
Solution
- Use Environment Variables: Always pull configuration settings, like database URLs or API keys, from environment variables to accommodate different testing environments.
- Consistent Setup: Document the setup commands or scripts for configuring environments to guarantee consistent testing conditions.
4. Insufficient Test Cleanup
The Need for Cleanup
When running integration tests, residual data can lead to false positives or negatives. It is imperative to reset shared resources or configuration states after each test run.
Example
test {
finalizedBy cleanUp
doLast {
// Cleanup actions here
println 'Cleaning up resources...'
}
}
task cleanUp {
doLast {
// Perform database cleanup or reset states
println 'Finished cleanup.'
}
}
Commentary
In the above scenario, we ensure that after each test run, we perform necessary cleanup to restore the test environment to its original state. This step is crucial in avoiding interference between test runs.
Solution
- Implement Cleanup Tasks: Always create separate tasks for cleanup to ensure test environments are reset, and resources are released.
- Use Database Transactions: Consider using database transactions that roll back after tests to maintain a clean state.
5. Lack of Parallel Execution
The Benefits of Parallel Execution
Running tests in parallel can significantly reduce the time needed for execution. However, if tests are not designed to run concurrently, issues can arise, leading to flaky tests and intermittent failures.
Example
test {
maxParallelForks = Runtime.runtime.availableProcessors().intdiv(2)
}
Commentary
Here, we leverage Gradle's capability to run tests in parallel based on the available processor count. This will help speed up the execution time, but it also requires tests to be designed without side effects.
Solution
- Isolate Tests: Ensure that tests are isolated from shared states or resources to prevent conflicts.
- Monitor Resource Usage: Be aware that paralleling tests can consume more resources, so might require resource allocation adjustments.
6. Inadequate Logging and Reporting
The Importance of Visibility
In integration testing, having visibility into test execution can help diagnose failures quickly. Many developers overlook proper logging, leading to prolonged debugging times.
Example
test {
testLogging {
events 'passed', 'skipped', 'failed'
showStandardStreams = true
}
}
Commentary
This configuration enhances the test logging output, allowing us to see which tests passed or failed at runtime. Visible logs can make diagnosing issues faster and more efficient.
Solution
- Configure Logging: Always ensure adequate logging configuration for your tests.
- Integration with CI Tools: If using CI/CD tools, configure integration logging so that it captures and displays relevant information.
The Last Word
Integration testing is essential for delivering high-quality software. By avoiding these common pitfalls in your Gradle setup, you can create more reliable and efficient tests. Remember to configure your tests correctly, manage dependencies effectively, account for environmental variability, ensure proper cleanup, utilize parallel execution where possible, and maintain clear logging practices.
Learning from these pitfalls not only enhances your testing performance but also elevates your overall development practices. For a deeper dive into Gradle testing practices, you may refer to the official Gradle documentation.
Embrace these strategies, improve your integration testing, and enjoy a smoother development workflow!