Struggling with Spring Session Scope? Here's How to Test It!
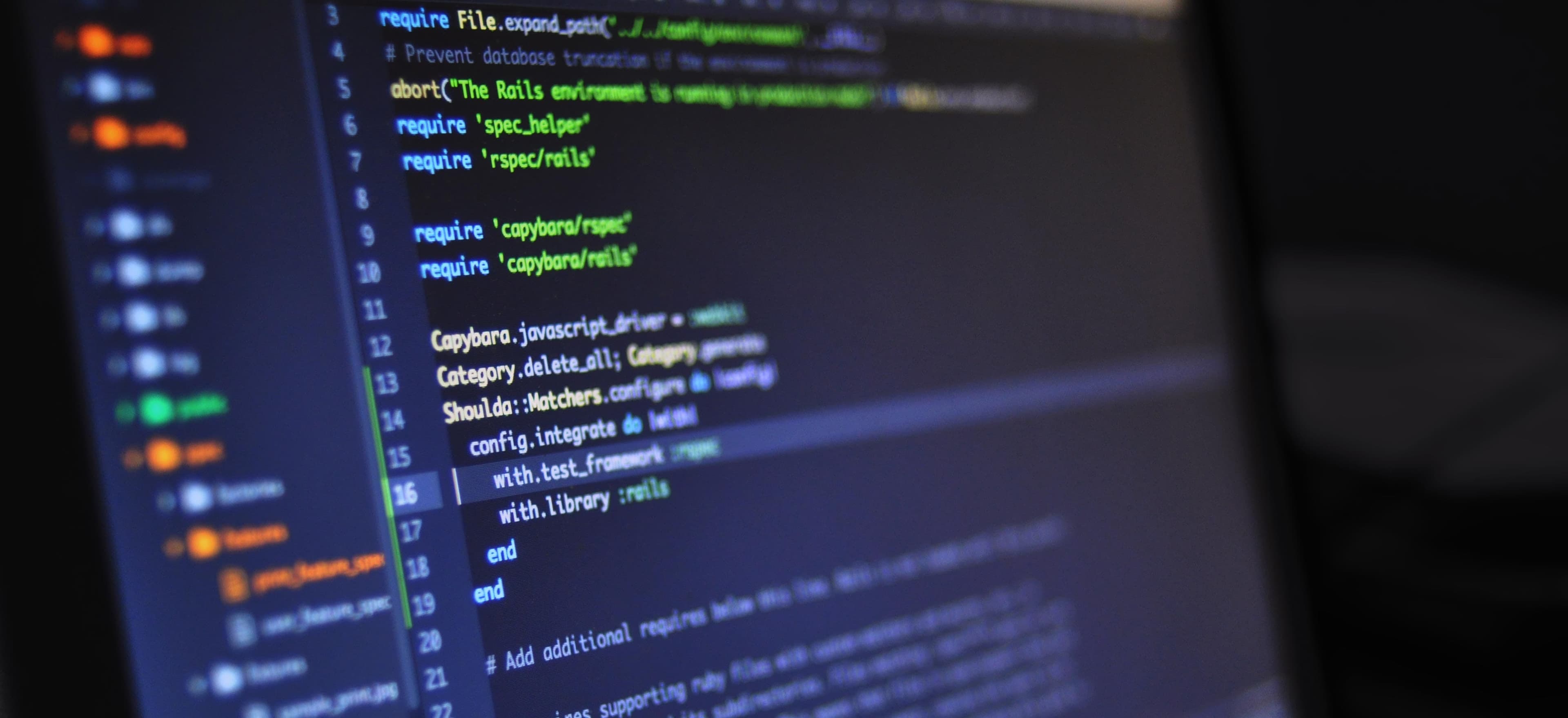
- Published on
Struggling with Spring Session Scope? Here's How to Test It!
Spring Framework offers a robust, powerful set of tools for building Java applications. Among these tools is the Spring Session module, which provides a way to manage session data in an easy and scalable manner. Whether you're building a web application or a RESTful service, understanding how to work with Session Scopes in Spring can be crucial. This blog post will focus on Spring Session Scope, why it's essential, and how to effectively test your session-scoped beans.
Understanding Spring Session Scope
Before we dive into testing, let’s clarify what session scope is. In Spring, a bean defined as session-scoped (@Scope(value = WebApplicationContext.SCOPE_SESSION)
) lives as long as the user's session. Every user gets their instance, allowing for personalization and state management over the session's lifespan.
Why Use Session Scope?
- User-specific data: Store any information pertinent to a single user session, like login status or preferences.
- Thread safety: Different users won’t share the same instance of the bean, eliminating concurrency issues and enabling safe manipulation of the session's state.
- Easier to manage: Given that session-scoped beans automatically terminate at the end of a session, you don’t have to worry about cleaning up resources manually.
With this understanding, let’s break down how to test session-scoped beans.
Setting Up a Basic Spring Application
First, ensure you have a Spring setup. Below is an example of a Spring Boot application that uses session-scoped beans.
SpringBootSessionApplication.java
package com.example.sessionscope;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootSessionApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootSessionApplication.class, args);
}
}
UserSessionBean.java
package com.example.sessionscope;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Scope(value = "session")
@Component
public class UserSessionBean {
private String username;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
}
Explanation
-
SpringBootSessionApplication: This is your main application file where the Spring application is initiated.
-
UserSessionBean: This is a simple session-scoped bean that stores username for the duration of the user's session. Notice how the class is annotated with
@Scope("session")
, telling Spring to create a new instance per user session.
Testing Session-scoped Beans
Testing session-scoped beans in Spring is crucial to ensure that your logic holds up when integrated into the application. Here's how to perform unit testing on a session-scoped bean using Spring Boot and JUnit.
Setting Up the Test
Create a test class named UserSessionBeanTest.java
.
package com.example.sessionscope;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.http.ResponseEntity;
import org.springframework.web.context.request.RequestAttributes;
import org.springframework.web.context.request.RequestContextHolder;
import static org.assertj.core.api.Assertions.assertThat;
@SpringBootTest
public class UserSessionBeanTest {
@Autowired
private UserSessionBean userSessionBean;
@Test
public void testSessionScope() {
// Simulate a user session
RequestAttributes requestAttributes = RequestContextHolder.getRequestAttributes();
assertThat(requestAttributes).isNotNull();
userSessionBean.setUsername("testUser");
// Validate that the data persists within the same session
assertThat(userSessionBean.getUsername()).isEqualTo("testUser");
}
}
Explanation
-
RequestContextHolder: This holds the current HTTP request attributes. By using this in tests, we can simulate a user session.
-
Assertions: The test sets a value for
username
and verifies if it persists within that session. If you run this test, you should see it pass if everything is set up correctly.
Mocking the HTTP Context in Tests
Sometimes, you might want to test your session-scoped beans without relying on the existing HTTP context. You can achieve this by mocking the request context in your tests.
Here's how you can enhance the given test using Mockito to simulate session attributes.
Mocking with Mockito
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import static org.assertj.core.api.Assertions.assertThat;
@SpringBootTest
public class UserSessionBeanTest {
@Autowired
private UserSessionBean userSessionBean;
private HttpSession httpSession;
@BeforeEach
public void setUp() {
HttpServletRequest request = Mockito.mock(HttpServletRequest.class);
httpSession = Mockito.mock(HttpSession.class);
Mockito.when(request.getSession()).thenReturn(httpSession);
RequestContextHolder.setRequestAttributes(new ServletRequestAttributes(request));
}
@Test
public void testSessionScopeWithMocking() {
userSessionBean.setUsername("mockedUser");
assertThat(userSessionBean.getUsername()).isEqualTo("mockedUser");
}
}
Explanation of Mocking
-
Mocking Requests and Sessions: We use Mockito to create mock instances of
HttpServletRequest
andHttpSession
. By doing so, you simulate the request context without needing an actual web-server. -
RequestContextHolder: This is set up with the mocked session before running any tests. This allows for clean testing in isolation.
My Closing Thoughts on the Matter
Testing session-scoped beans in Spring is essential for creating web applications that manage user-specific data effectively. By understanding and utilizing features like RequestContextHolder
and mocking HTTP sessions with Mockito, you can create thorough tests that ensure your application behaves as expected.
To further enhance your Spring knowledge, consider diving into the official Spring documentation here.
Happy Coding! For more practical insights on Java and Spring, you may also want to check an insightful article on testing strategies here, which covers additional testing techniques.
With this knowledge, you should feel more equipped to handle testing with Session Scope in your Spring applications. Whether you're building simple projects or complex enterprise applications, mastering these skills will undoubtedly serve you well!