Overcoming GC Issues in Java: The Exchanger Challenge
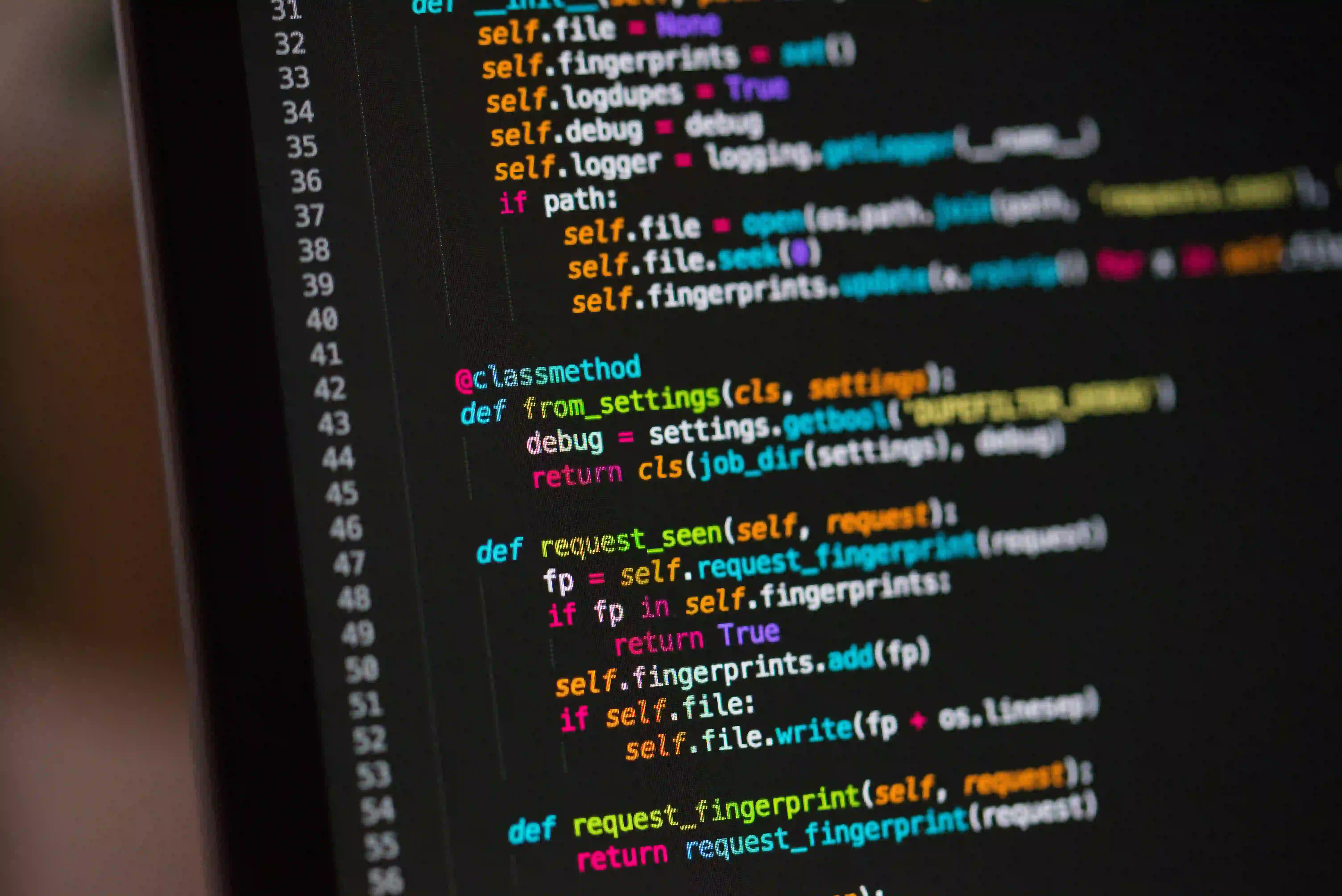
Overcoming GC Issues in Java: The Exchanger Challenge
Garbage Collection (GC) is a powerful feature in Java that manages memory automatically. However, it can sometimes lead to performance issues, particularly in high-load applications. In this blog post, we will explore GC issues associated with Java's Exchanger
class, a thread synchronization mechanism. We’ll discuss how to identify GC-related problems and potential strategies to mitigate them.
Understanding Garbage Collection in Java
Before diving into the specifics of the Exchanger
class, we must briefly understand how garbage collection works in Java. Java's garbage collector automatically frees memory by removing objects that are no longer in use. However, during this process, the Java Virtual Machine (JVM) might pause application threads, resulting in performance hiccups or "stop-the-world" events.
Why GC Matters
Java applications frequently run in environments where optimal performance is crucial. Excessive GC pauses can lead to noticeable slowdowns or even application unavailability. This is particularly problematic for real-time applications such as gaming, trading systems, or large-scale web services that need low-latency responses.
The Role of the Exchanger Class
The Exchanger
class facilitates a rendezvous point where two threads can swap data. It is often used in scenarios where a pair of threads need to exchange information concurrently without significant overhead.
Key Features of Exchanger
- Thread Blocking: If one thread calls
exchange()
, it blocks until another thread also callsexchange()
with the same target. - Data Passing: Objects are exchanged between threads, which can lead to complex memory management and GC implications, especially with frequent use.
Here is a basic implementation of Exchanger
:
import java.util.concurrent.Exchanger;
public class ExchangerExample {
public static void main(String[] args) {
Exchanger<String> exchanger = new Exchanger<>();
Thread thread1 = new Thread(() -> {
try {
String str1 = "Data from Thread 1";
String receivedData = exchanger.exchange(str1);
System.out.println("Thread 1 received: " + receivedData);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
Thread thread2 = new Thread(() -> {
try {
String str2 = "Data from Thread 2";
String receivedData = exchanger.exchange(str2);
System.out.println("Thread 2 received: " + receivedData);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
thread1.start();
thread2.start();
}
}
Commentary on the Code
In the above example, we create an Exchanger
object that enables two threads to exchange strings. Each thread waits for the other to reach the exchange()
method.
The blocking behavior is essential; however, if many threads frequently use Exchanger
, each call can lead to a buildup of objects waiting for disposal, thus triggering GC cycles more often.
Common GC Issues with Exchanger
As stated, the Exchanger
creates objects that might not recycle cleanly, depending on usage patterns. Here are some common GC-related challenges:
1. Increased Object Creation
Every time you call exchange()
, you may end up creating new objects, leading to increased memory pressure.
2. Thread Contention
If multiple threads are trying to execute exchanges simultaneously, this can lead to contention, causing unnecessary object retention in memory.
3. Frequent Full GCs
When the system is under memory pressure or when objects become unreachable, the GC tries to reclaim space. A highly active Exchanger
can increase the frequency of Full GCs, resulting in slower application performance.
Identifying GC Issues
To overcome GC challenges related to Exchanger
, we first need to identify them. Here are some tools and approaches:
Heap Dump Analysis
Using tools like VisualVM or Eclipse MAT (Memory Analyzer Tool) allows you to visualize memory consumption and check for excessive object creation patterns.
GC Logs
You can enable GC logging in your JVM by adding options like the following at startup:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -XX:+PrintGCTimeStamps -Xloggc:gc.log
This will generate logs that can provide insight into how often GC occurs and how much time is spent on it.
Strategies to Mitigate GC Issues
Here we will discuss several strategies to mitigate GC issues encountered with the Exchanger
.
1. Object Pooling
Instead of continuously creating new objects for data transfer, an object pool can be utilized to recycle objects:
import java.util.concurrent.Exchanger;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.TimeUnit;
public class ExchangerWithPooling {
private final LinkedBlockingQueue<String> pool = new LinkedBlockingQueue<>(10);
private final Exchanger<String> exchanger = new Exchanger<>();
public String lease() {
return pool.poll();
}
public void release(String obj) {
pool.offer(obj);
}
// Example operation goes here...
}
By using an object pool, we effectively minimize the number of new allocations, resulting in fewer objects for the GC to manage.
2. Reduce Contention with Fine-Grained Locking
If possible, minimize contention by reducing the critical sections in which threads wait. Consider using multiple Exchanger
instances or leveraging other concurrency constructs such as semaphores or countdown latches.
3. Tune JVM Options
Tuning the garbage collector via JVM options can also provide relief. For example, increasing the heap size can reduce the frequency of GCs:
-Xmx2g -Xms2g
In addition, selecting a different GC algorithm such as G1 or ZGC may sometimes yield better performance.
4. Assess Thread Usage Patterns
By analyzing thread usage, you can determine whether you can optimize operations to reduce repetitive calls to exchange()
. More efficient usage patterns will inherently lead to lower object creation rates.
Bringing It All Together
In this blog post, we explored the challenges presented by garbage collection when using the Exchanger
class in Java. While garbage collection significantly simplifies memory management, it is crucial to stay vigilant regarding its implications on performance, especially in multi-threaded environments.
By implementing the strategies discussed—object pooling, contention reduction, JVM tuning, and assessing thread usage—you can effectively manage GC-related issues, ensuring smoother and more responsive applications. Always remember that proactive monitoring and analysis using tools can be invaluable in identifying potential performance bottlenecks.
For more detailed insights on GC strategies and best practices, check out the Java Concurrency documentation and the Java Garbage Collection Basics.
Happy coding!