Common GWT Pitfalls for Beginners: Avoid These Mistakes!
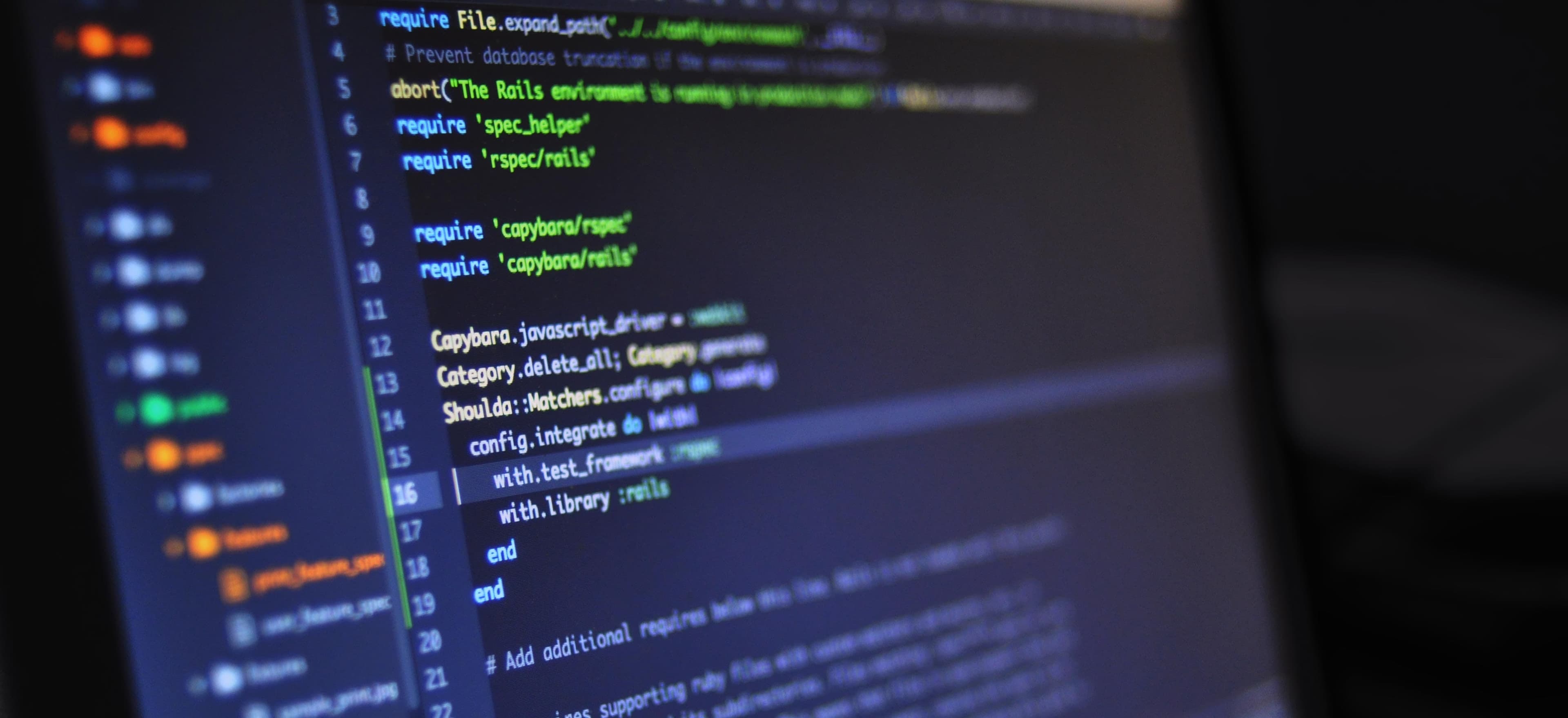
- Published on
Common GWT Pitfalls for Beginners: Avoid These Mistakes!
Google Web Toolkit (GWT) is a powerful framework that allows developers to write client-side applications in Java, which are then compiled into JavaScript. While GWT offers significant benefits, especially for Java developers venturing into web development, beginners often encounter a set of common pitfalls. This blog post outlines these pitfalls and provides guidance on how to avoid them, assisting new developers in navigating the GWT landscape more effectively.
Understanding GWT: A Brief Overview
Before diving into the pitfalls, let's clarify what GWT is and why it matters. GWT enables developers to build complex web applications with a robust Java-based backend. The framework abstracts Java from JavaScript intricacies, making it easier for Java developers to create rich internet applications (RIAs).
However, this abstraction can lead to misconceptions, particularly for beginners. Here are the most common pitfalls and how to navigate them.
Pitfall 1: Ignoring the Compile Process
What Is It?
One of the first things GWT newcomers overlook is the compilation process that transforms Java code into JavaScript. This process can be complex and sometimes leads to confusion.
Why It Matters
Understanding the compilation process is crucial because:
- Debugging: Errors or misconfigurations may arise during compilation that may not be evident in the Java code initially.
- Performance: Properly balanced GWT compilation settings can significantly affect application performance.
Solution
Be diligent in learning about and utilizing the GWT compiler. Below is a simple example to illustrate how to set up a GWT module:
// YourModule.gwt.xml
<module>
<inherits name="com.google.gwt.core.Core"/>
<entry-point class="com.example.client.YourEntryPoint"/>
</module>
In this example, YourModule.gwt.xml
defines your GWT module. Make sure to include all necessary inherited modules to prevent runtime errors.
Pitfall 2: Misunderstanding Asynchronous Calls
What Is It?
GWT applications often require asynchronous processes to retrieve data from the server without freezing the UI. Beginners frequently mishandle these calls, resulting in poor user experiences.
Why It Matters
Improperly managing asynchronous calls can lead to:
- Unresponsive Interfaces: Users might think the application is broken due to delays in data retrieval.
- State Management Issues: Failing to handle callbacks correctly can lead to data inconsistency.
Solution
Make use of GWT's asynchronous RPC (Remote Procedure Call) patterns. Here is an exemplary code snippet demonstrating how this can be effectively handled:
public interface MyServiceAsync {
void getData(AsyncCallback<List<Data>> callback);
}
public class MyServiceImpl extends RemoteServiceServlet implements MyService {
public List<Data> getData() {
// Actual implementation of data retrieval
}
}
In this snippet, the AsyncCallback<List<Data>>
provides a method for safely receiving results. Ensure that your implementation handles both success and failure states of asynchronous calls effectively.
Pitfall 3: Overcomplicating the UI
What Is It?
GWT allows for creating complex UIs, but beginners often go overboard. Cluttered or overly complex UIs can confuse users and impair usability.
Why It Matters
Clarity and simplicity should be your top priorities. An effective UI should be intuitive; if users struggle to navigate, they are unlikely to return.
Solution
Utilize GWT's built-in widgets sensibly. Here's a basic example of how to create a simple UI:
public class SimpleForm implements EntryPoint {
public void onModuleLoad() {
VerticalPanel panel = new VerticalPanel();
TextBox nameField = new TextBox();
Button submitButton = new Button("Submit");
panel.add(nameField);
panel.add(submitButton);
RootPanel.get().add(panel);
}
}
This example shows a clean layout with a single input and action button. Strive to prioritize clear and straightforward UX design principles in your applications.
Pitfall 4: Not Leveraging GWT’s Built-in Features
What Is It?
GWT comes equipped with a plethora of extensive libraries and tools that can simplify development. Beginners often overlook these features, opting to implement functionalities from scratch.
Why It Matters
Reinventing the wheel can lead to inefficient code that doesn't utilize tested solutions. Doing this may result in increased development time and lower application performance.
Solution
Take advantage of GWT's extensive libraries, such as the GWT UI library and Cell API, which provide a framework for efficient rendering of lists and tables. Here's how you can implement a simple grid using the Cell API:
public class DataGridExample implements EntryPoint {
public void onModuleLoad() {
DataGrid<MyData> dataGrid = new DataGrid<>();
// Apply data provider to the grid
ListDataProvider<MyData> dataProvider = new ListDataProvider<>();
dataProvider.addDataDisplay(dataGrid);
// Add some data
dataProvider.getList().add(new MyData(...));
RootPanel.get().add(dataGrid);
}
}
This code represents a basic Grid setup utilizing GWT's built-in functionalities, thus improving performance and reducing potential errors.
Pitfall 5: Overlooking Browser Compatibility
What Is It?
GWT may handle JavaScript generation, but browser compatibility is still a concern. Beginners often assume their application behaves consistently across different browsers.
Why It Matters
Different browsers render applications, scripts, and styles differently. Not ensuring cross-browser compatibility can alienate users on various devices.
Solution
Test your application across multiple browsers and devices. Utilize GWT's built-in testing utilities and consider using services like BrowserStack for further verification.
A Final Look
While GWT is an excellent tool for Java developers, avoiding these common pitfalls is essential for building successful web applications. Be diligent in recognizing the importance of the compilation process, managing asynchronous calls, maintaining a clean UI, leveraging built-in features, and ensuring browser compatibility.
By following these guidelines, beginners can navigate the complexities of GWT with greater confidence. For further reading, you may explore the Google Web Toolkit documentation and GWT RPC documentation.
Equip yourself with this knowledge, and watch your GWT applications flourish! Happy coding!
Checkout our other articles