Maximize Performance: Troubleshooting ChronicleMap Issues
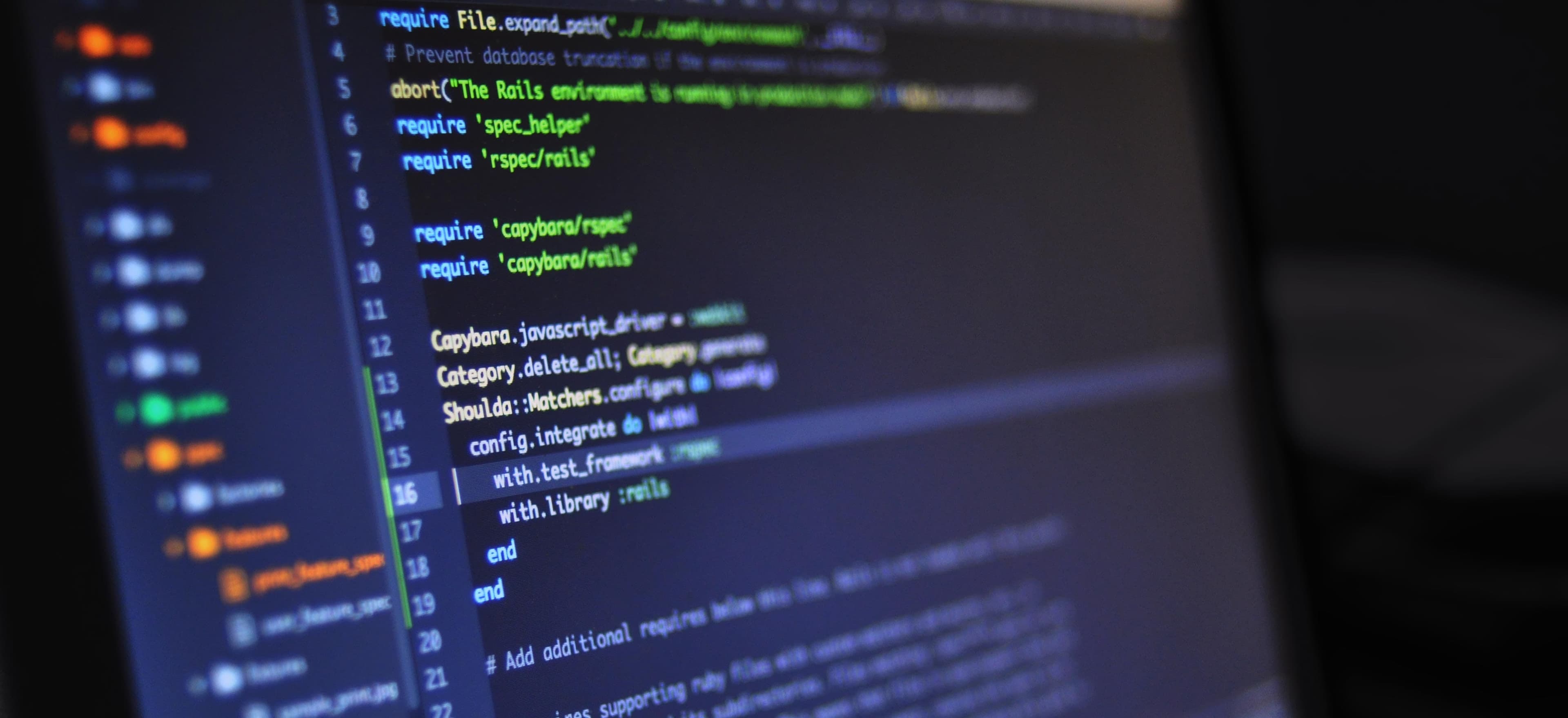
- Published on
Maximize Performance: Troubleshooting ChronicleMap Issues
In the world of high-performance computing, efficient data storage and retrieval can make or break application performance. One of the tools that developers often turn to is ChronicleMap, a high-performance, memory-mapped key-value store designed specifically for low-latency access to data structures. However, like any powerful technology, it can come with its own set of challenges. This blog post will highlight common issues when working with ChronicleMap and provide insightful solutions to maximize performance.
Understanding ChronicleMap
Before diving into troubleshooting, let’s establish what ChronicleMap is. It is an in-memory key-value store built for concurrent access, typically used in situations demanding minimal latency and exceptional throughput. Its efficiency stems from memory mapping the data, which allows the system to access data structures directly from disk when required, instead of relying heavily on garbage collection.
Here's a simple illustration of creating a ChronicleMap:
import net.openhft.chronicle.map.ChronicleMap;
ChronicleMap<Integer, String> map = ChronicleMap.of(Integer.class, String.class)
.name("example-map")
.entries(1000)
.averageKey(1)
.averageValue("example")
.create();
In this snippet, we are creating a ChronicleMap instance. The entries(1000)
method establishes an initial capacity, while averageKey(1)
and averageValue("example")
help in optimizing memory usage from the get-go.
Common ChronicleMap Issues and Solutions
1. Performance Degradation
As your application grows or the volume of data increases, you might notice a performance slowdown. This can be attributed to multiple factors including improper configuration or resource limitations.
Solution: Optimize Configuration
Adjust the configuration settings based on your use case. Consider the following:
- Max Entries: Ensure you set a sufficient number of entries when creating your map. If the setting is too low, the map can overrun, leading to increased latencies.
ChronicleMap<Integer, String> map = ChronicleMap.of(Integer.class, String.class)
.name("optimized-map")
.entries(5000) // A higher number of entries for scalability
.averageKey(1)
.averageValue("example")
.create();
- Memory Mapped File: Use a dedicated memory segment for the ChronicleMap. The path should point to SSD rather than HDD for significant latency reduction.
2. Key Collisions
Key collisions can create serious performance bottlenecks, especially in a concurrent scenario. When multiple threads try to read or write the same key, it can lead to contention and reduced throughput.
Solution: Use Unique Keys and Hashing
Ensure you're using unique keys. Employ hashing strategically to minimize the likelihood of collisions:
import java.util.Objects;
public class CustomKey {
private final String identifier;
public CustomKey(String identifier) {
this.identifier = identifier;
}
@Override
public int hashCode() {
return Objects.hash(identifier);
}
}
Using a custom key will reduce the chances of colliding with other entries and improve the overall performance.
3. Memory Consumption
While ChronicleMap is built for efficiency, misconfigurations can lead to increased memory consumption, resulting in a decrease in performance.
Solution: Regular Monitoring and Adjustment
Regularly monitor your application’s memory utilization with tools like JVisualVM or Java Mission Control. Adjust your ChronicleMap settings based on runtime conditions.
You can also implement size limits for entries needing frequent updates:
map.put(key, value);
if (map.size() > 5000) {
// Implement eviction strategy or resizing logic
}
Consider implementing an eviction strategy to discard stale entries and free up memory.
4. Serialization Issues
When storing objects in ChronicleMap, improper serialization can lead to performance issues due to increased overhead.
Solution: Use Efficient Serialization
Make sure to utilize efficient serialization techniques. Instead of standard Java serialization, consider using libraries like Kryo or Protobuf for better performance.
For instance, using Kryo:
import com.esotericsoftware.kryo.Kryo;
Kryo kryo = new Kryo();
byte[] serialized = new byte[256];
// Use Kryo to serialize your data
kryo.writeObject(new Output(serialized), yourObject);
5. Thread Safety
ChronicleMap is designed for concurrent access, but improper usage patterns can lead to thread safety issues. Ensure that access patterns do not interfere with each other, leading to unexpected behaviors.
Solution: Proper Synchronization
Even though ChronicleMap manages concurrency, it's wise to implement your level of synchronization where necessary, especially in read-modify-write scenarios.
synchronized (map) {
String existingValue = map.get(key);
map.put(key, existingValue + "_updated");
}
Additional Resources
For more detailed insights into ChronicleMap, I highly recommend the official Chronicle Documentation and Chronicle GitHub Repository. These resources provide in-depth examples and advanced configurations that are invaluable for developers looking to optimize their implementation.
Final Thoughts
ChronicleMap is a robust tool for managing key-value pairs in high-performance Java applications. While you may encounter issues like performance degradation, key collisions, memory consumption, serialization challenges, or thread safety, understanding these problems and applying the suggested solutions can significantly enhance your application's performance.
Stay vigilant and continually monitor your configuration and usage patterns to leverage the full potential of ChronicleMap. With the right strategies, you can ensure efficient and speedy data retrieval, leading to a seamless experience for your users.
Happy coding!
Checkout our other articles