Struggling with ReadWriteLocks? Simplify with Java 8 Lambdas!
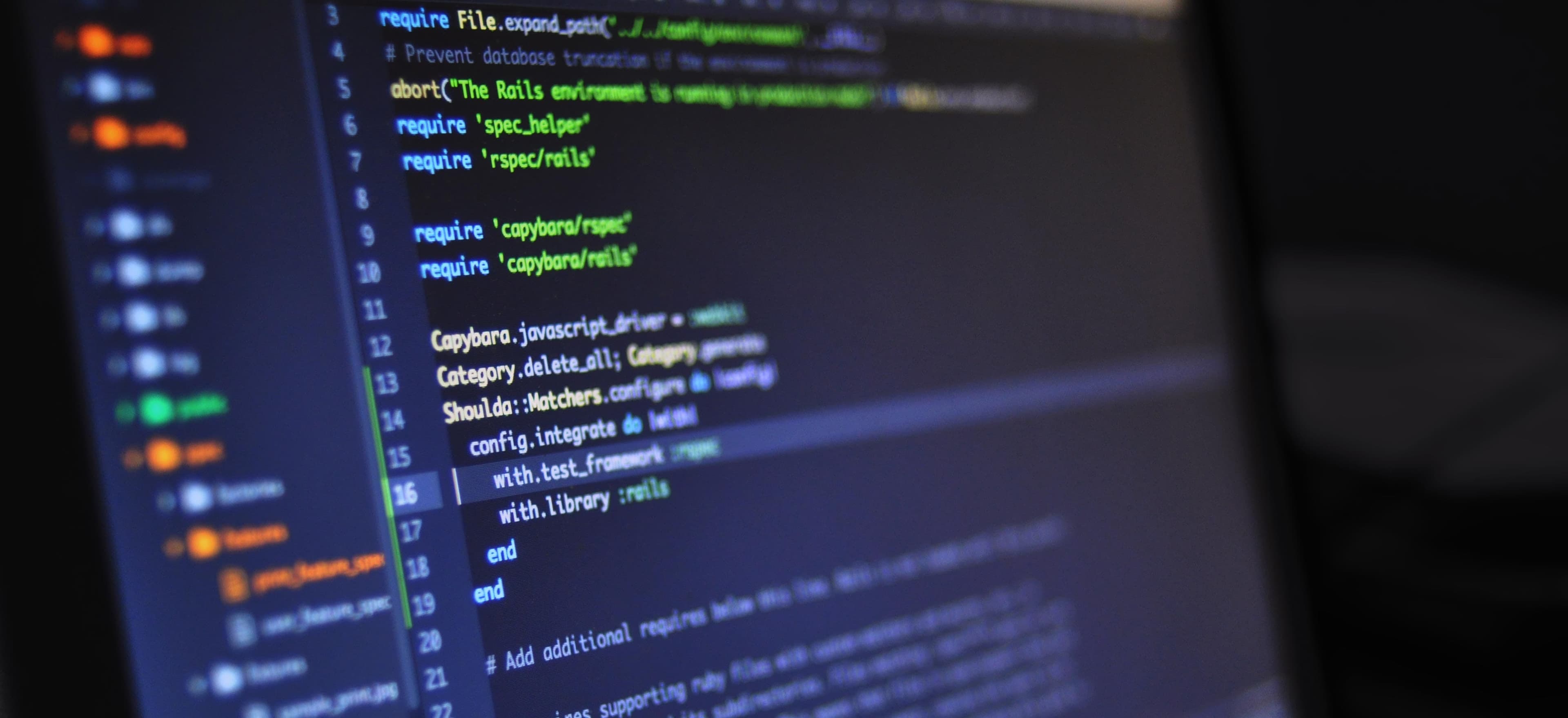
- Published on
Struggling with ReadWriteLocks? Simplify with Java 8 Lambdas!
The world of multithreading in Java can be a daunting landscape, especially when working with synchronization constructs such as ReadWriteLocks
. But fear not! This blog post will explore how to effectively leverage Java 8's Lambda expressions to simplify the usage of ReadWriteLocks.
What are ReadWriteLocks?
Before we dive into the practical implementations, let's clarify what ReadWriteLocks are. ReadWriteLock
is an interface that provides a pair of associated locks: a ReadLock
and a WriteLock
.
- A
ReadLock
allows multiple threads to read data concurrently. - A
WriteLock
is exclusive, allowing only one thread to write at a time.
This mechanism increases concurrency in scenarios where read operations vastly outnumber write operations. If you're curious to explore more about the inner workings of Java concurrency, you can check Java's official documentation on concurrency.
Benefits of Using ReadWriteLocks
- Improved Performance: When you use a
ReadWriteLock
, multiple threads can read without being blocked by write operations, significantly improving the performance for read-heavy workloads. - Data Consistency: The WriteLock ensures that when a write operation is happening, no other thread can read or write, maintaining data integrity.
The Traditional Way
To use a ReadWriteLock
, one often ends up writing verbose and complex code. Let’s look at a traditional example:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteLockExample {
private final ReadWriteLock lock = new ReentrantReadWriteLock();
private String sharedResource = "Initial Value";
public String read() {
lock.readLock().lock();
try {
return sharedResource;
} finally {
lock.readLock().unlock();
}
}
public void write(String value) {
lock.writeLock().lock();
try {
sharedResource = value;
} finally {
lock.writeLock().unlock();
}
}
}
This approach, while effective, can result in boilerplate code that obscures the primary logic. Fortunately, Java 8's lambda expressions enable a more elegant solution.
Simplifying with Java 8 Lambdas
Java 8 introduced a host of features, notably Lambda expressions, that help simplify coding patterns. The ReadWriteLock
can benefit from this functionality, allowing us to encapsulate the locking logic in reusable utility methods.
Creating a Lock Utility Class
We can create a LockUtils
class that provides methods to handle locking using Lambdas.
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import java.util.function.Supplier;
public class LockUtils {
private final ReadWriteLock lock = new ReentrantReadWriteLock();
public <T> T read(Supplier<T> action) {
lock.readLock().lock();
try {
return action.get();
} finally {
lock.readLock().unlock();
}
}
public void write(Runnable action) {
lock.writeLock().lock();
try {
action.run();
} finally {
lock.writeLock().unlock();
}
}
}
Using the Lock Utility Class
With our LockUtils
class set up, our code can now be streamlined. Let's keep working with our ReadWriteLockExample
class:
public class ReadWriteLockExample {
private final LockUtils lockUtils = new LockUtils();
private String sharedResource = "Initial Value";
public String read() {
return lockUtils.read(() -> sharedResource);
}
public void write(String value) {
lockUtils.write(() -> sharedResource = value);
}
}
Commentary on the Code
- Separation of Concerns: We extracted the locking logic into a dedicated utility class (
LockUtils
), promoting code reuse. - Lambda Expressions: The
Supplier<T>
andRunnable
functional interfaces enable us to encapsulate any read or write action in a compact form. Instead of boilerplate locking and unlocking logic, we now focus on the core functionalities. - Readability: Code becomes cleaner and significantly easier to read and maintain.
Handling Exceptions and Failures
In a multithreaded environment, failure handling is crucial. Consider modifying the LockUtils
class to handle exceptions gracefully.
import java.util.function.Consumer;
public class LockUtils {
private final ReadWriteLock lock = new ReentrantReadWriteLock();
public <T> T read(Supplier<T> action) {
lock.readLock().lock();
try {
return action.get();
} catch (Exception e) {
// Handle exceptions if necessary
System.err.println("Exception in read: " + e.getMessage());
return null;
} finally {
lock.readLock().unlock();
}
}
public void write(Consumer<Throwable> action) {
lock.writeLock().lock();
try {
action.accept(null);
} catch (Throwable t) {
// Handle exceptions if necessary
System.err.println("Exception in write: " + t.getMessage());
} finally {
lock.writeLock().unlock();
}
}
}
A Final Look
The powerful combination of ReadWriteLocks
and Java 8 Lambda expressions can greatly simplify your multithreading code. By encapsulating locking mechanisms and creating reusable utilities, you can significantly enhance the clarity and maintainability of your code.
Leveraging the fundamentals of concurrency with modern programming constructs opens a valuable pathway for developing efficient, clean, and robust applications. For further reading on improving concurrency in Java, check out Java Concurrency in Practice.
As you dive deeper into using ReadWriteLocks
in Java, remember that simplification is often just a Lambda expression away! Happy coding!
Checkout our other articles