Mastering Complex Objects in URL Parameters: A How-To Guide
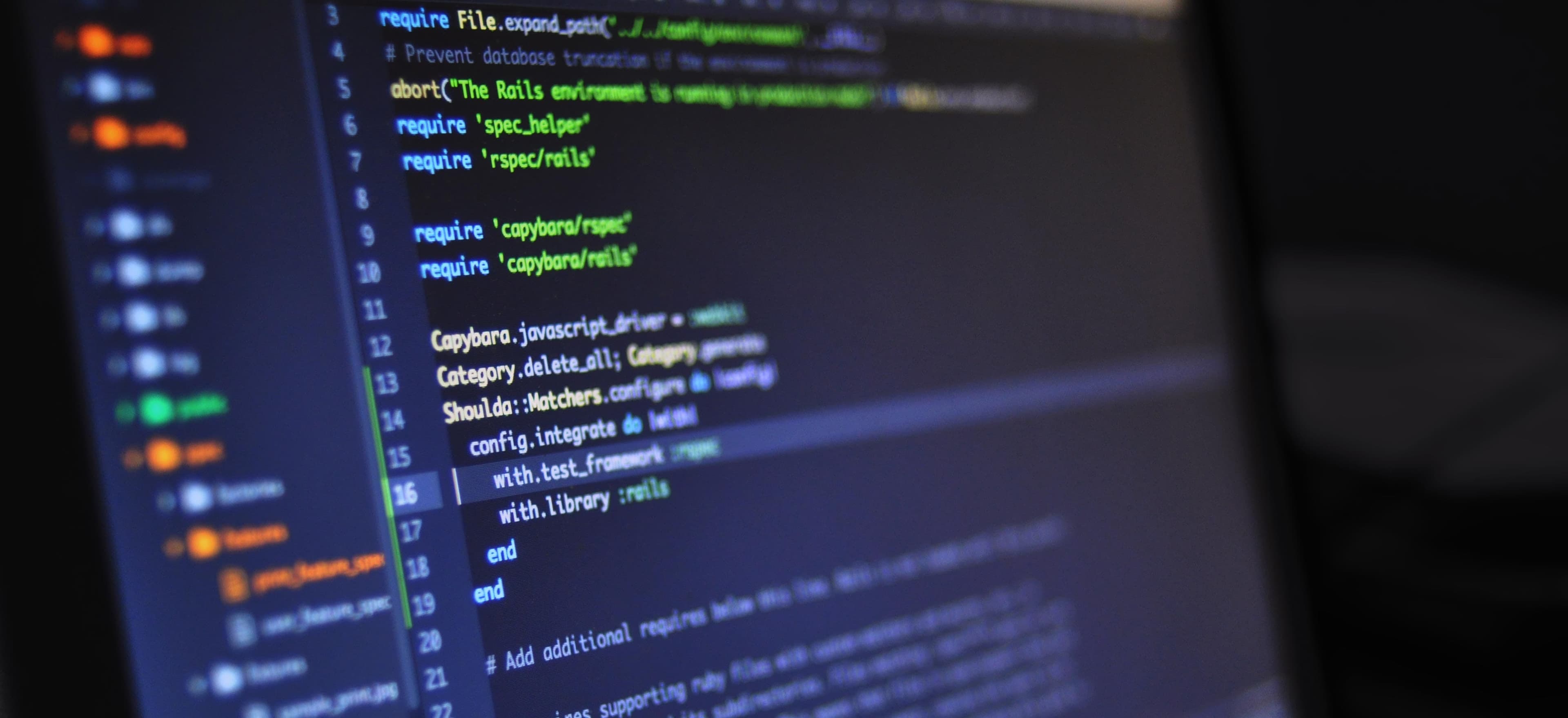
- Published on
Mastering Complex Objects in URL Parameters: A How-To Guide
In the world of web development, managing and transmitting data efficiently is crucial. One common data transmission method is through URL parameters. As applications grow more complex, you'll often find yourself needing to send structured data via these parameters, like arrays or complex objects. In this guide, we will delve into the intricacies of passing complex objects in URL parameters, offering clear examples and best practices—ensuring your applications remain robust and user-friendly.
Understanding URL Parameters
URL parameters (or query strings) are appended to a URL after a question mark (?) and consist of key-value pairs, typically structured as key=value
. Here's a basic example:
https://example.com/?userId=123&sessionId=abc
Why Use URL Parameters?
Using URL parameters is advantageous for several reasons:
- Bookmarkability: The state of an application can be saved through a simple URL.
- Shareability: URLs can be shared easily with encoded data.
- Statelessness: They enable stateless interactions, making your web applications more scalable.
Encoding Complex Objects
When dealing with complex objects—like nested objects or arrays—multiple key-value pairs can quickly clutter your URLs. Let's explore how you can effectively encode and decode these structures.
Example of a Complex Object
Suppose you have the following JavaScript object that you want to encode as URL parameters:
const userSettings = {
theme: 'dark',
notifications: {
email: true,
sms: false
},
preferences: ['news', 'updates']
};
To pass this object in a URL parameter, we need to convert it into a string format that retains the structure.
Converting Objects to Query Strings
You can use a library like qs or the native URLSearchParams
API to accomplish this.
Method 1: Using qs
Install qs
:
npm install qs
Then, you can encode the object like this:
const qs = require('qs');
const userSettings = {
theme: 'dark',
notifications: {
email: true,
sms: false
},
preferences: ['news', 'updates']
};
// Convert the object to a query string
const queryString = qs.stringify(userSettings);
console.log(queryString);
// Output: theme=dark¬ifications[email]=true¬ifications[sms]=false&preferences[]=news&preferences[]=updates
Why Use qs
?
The qs
library offers a clear, concise way to structure your URL parameters, preserving the relationship of nested objects and arrays. This approach not only enhances readability but also ensures that your backend can interpret the incoming request correctly.
Method 2: Using URLSearchParams
If you prefer not to use an external library, the URLSearchParams
built-in API is another option, though it has limitations with complex objects.
Here's how you can do it:
const userSettings = {
theme: 'dark',
notifications: {
email: true,
sms: false
},
preferences: ['news', 'updates']
};
const params = new URLSearchParams();
params.append('theme', userSettings.theme);
params.append('notifications', JSON.stringify(userSettings.notifications)); // Serialize the notifications object
params.append('preferences', JSON.stringify(userSettings.preferences)); // Serialize the preferences array
console.log(params.toString());
// Output: theme=dark¬ifications={"email":true,"sms":false}&preferences=["news","updates"]
Decoding URL Parameters
Once you receive a URL with complex objects encoded, decoding it is an essential function of your application.
If you're using the qs
library:
const receivedQuery = 'theme=dark¬ifications[email]=true¬ifications[sms]=false&preferences[]=news&preferences[]=updates';
const decodedSettings = qs.parse(receivedQuery);
console.log(decodedSettings);
Output:
{
theme: 'dark',
notifications: { email: true, sms: false },
preferences: ['news', 'updates']
}
If using URLSearchParams
:
const url = new URL('https://example.com/?theme=dark¬ifications=%7B%22email%22%3Atrue%2C%22sms%22%3Afalse%7D&preferences=%5B%22news%22%2C%22updates%22%5D');
const theme = url.searchParams.get('theme');
const notifications = JSON.parse(url.searchParams.get('notifications'));
const preferences = JSON.parse(url.searchParams.get('preferences'));
console.log({ theme, notifications, preferences });
Best Practices
-
Keep It Simple: Only include data in your URLs that needs to be shared or bookmarked.
-
Limit Length: URLs have a maximum length (generally around 2000 characters for most browsers). Ensure your parameters do not exceed this.
-
Use URL Encoding: Always encode values in URLs to avoid issues with special characters. JavaScript's
encodeURIComponent()
can be indispensable for this. -
Check with Back-End: Ensure your back-end can handle and correctly decode the complex objects you send. Consistency between front-end encoding and back-end decoding is pivotal.
-
Secure Sensitive Data: Avoid passing sensitive information through URL parameters, as URLs can be logged and cached. Use POST requests for sensitive data instead.
The Closing Argument
Mastering how to send complex objects in URL parameters is essential for developing modern web applications. By utilizing either native JavaScript features or libraries like qs
, you can efficiently encode and transmit structured data, thereby maintaining a clean, efficient codebase.
For further reading, consider exploring related topics:
By applying these techniques and best practices, you can enhance data management within your applications while ensuring a user-friendly experience. Happy coding!
Checkout our other articles