Overcoming Common RabbitMQ Configuration Issues in Spring Boot
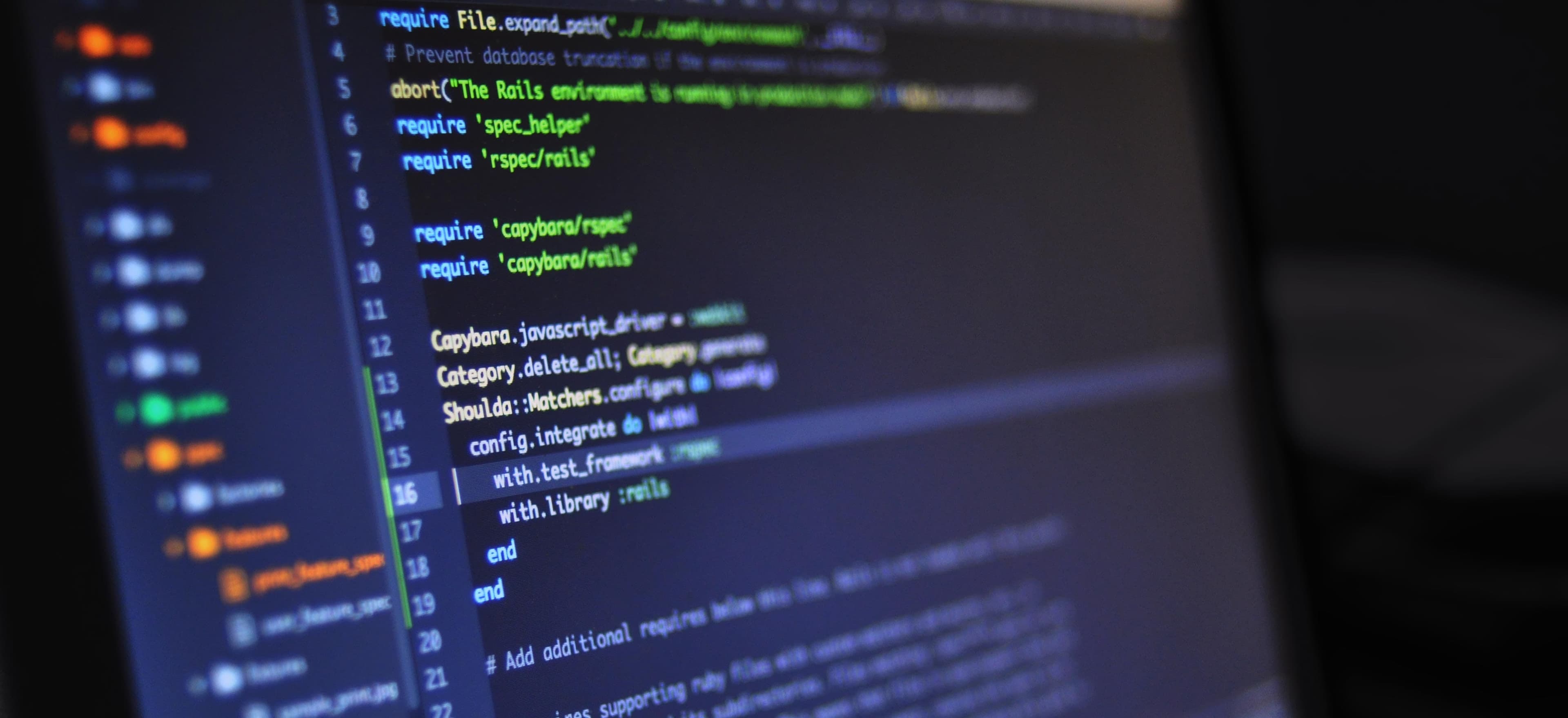
- Published on
Overcoming Common RabbitMQ Configuration Issues in Spring Boot
As microservices architectures gain traction, message brokers like RabbitMQ have become essential for ensuring seamless communication between services. Spring Boot provides robust and easy-to-use support for RabbitMQ, allowing developers to quickly integrate messaging functionality into their applications. However, configuration issues can arise that may hinder performance or result in runtime errors. In this post, we'll explore common RabbitMQ configuration challenges in Spring Boot and discuss strategies to overcome them.
Table of Contents
- Understanding RabbitMQ and Spring Boot
- Common Configuration Issues
- 2.1 Connection Errors
- 2.2 Message Conversion Issues
- 2.3 Queue and Exchange Configuration Problems
- Tips for Successful Integration
- Code Snippets with Commentary
- Conclusion and Further Reading
Understanding RabbitMQ and Spring Boot
RabbitMQ is an open-source message broker that implements the Advanced Message Queuing Protocol (AMQP). It facilitates communication between applications or services through message queues. Spring Boot simplifies the use of RabbitMQ by providing auto-configuration and support for various messaging operations.
To get started with RabbitMQ in Spring Boot, you need to include the necessary dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
This dependency allows you to leverage Spring AMQP, offering a powerful and flexible way to work with RabbitMQ.
Common Configuration Issues
While integrating RabbitMQ with Spring Boot, developers often encounter a range of configuration problems. Let's delve into some of the most prevalent issues and explore their solutions.
2.1 Connection Errors
Connection issues are quite frequent when working with RabbitMQ. They can be caused by incorrect username/password, wrong host/port configurations, or network constraints.
Solution Steps:
-
Check your application properties:
The RabbitMQ connection details should be specified in your
application.properties
orapplication.yml
file. Here’s a sample configuration:spring.rabbitmq.host=localhost spring.rabbitmq.port=5672 spring.rabbitmq.username=user spring.rabbitmq.password=secret
-
Test connectivity:
Use telnet or similar tools to test if you can connect to the RabbitMQ service.
telnet localhost 5672
If you cannot connect, verify that RabbitMQ is running and accessible.
-
Check RabbitMQ logs:
Investigate the RabbitMQ logs to obtain detailed error messages. They can usually be found in the RabbitMQ data directory.
2.2 Message Conversion Issues
Another common problem is related to message conversion, where the application can’t serialize or deserialize messages correctly.
Solution Steps:
-
Define custom message converters:
By default, Spring AMQP uses a simple, string-based message conversion. If you're sending complex objects, consider creating a custom message converter that can handle your object types.
Below is a simple example of how to configure a
Jackson2JsonMessageConverter
for JSON serialization:@Bean public Jackson2JsonMessageConverter jsonMessageConverter() { return new Jackson2JsonMessageConverter(); }
Use this converter in your configuration:
@Bean public RabbitTemplate rabbitTemplate(ConnectionFactory connectionFactory) { RabbitTemplate template = new RabbitTemplate(connectionFactory); template.setMessageConverter(jsonMessageConverter()); return template; }
-
Check
@JsonProperty
annotations:If you are using custom classes, verify that all properties are correctly annotated with
@JsonProperty
if necessary.
2.3 Queue and Exchange Configuration Problems
Queues and exchanges might not be configured correctly, leading to issues like messages not being routed as expected.
Solution Steps:
-
Explicitly declare queues and exchanges:
Leverage Spring's configuration capabilities to declare queues and exchanges programmatically. This ensures that your infrastructure is set up before messaging takes place.
@Bean public Queue myQueue() { return new Queue("myQueue", true); } @Bean public TopicExchange myExchange() { return new TopicExchange("myExchange"); } @Bean public Binding binding(Queue myQueue, TopicExchange myExchange) { return BindingBuilder.bind(myQueue).to(myExchange).with("my.routing.key"); }
-
Use RabbitMQ Management UI:
The RabbitMQ Management Plugin provides a web interface that allows you to view queues, exchanges, and bindings in real-time. Ensure that your queues are bound to the respective exchanges.
-
Check messages in unacked state:
Sometimes messages may get stuck in an unacknowledged state. Investigate if the RabbitMQ configurations are set correctly regarding acknowledgment modes.
Tips for Successful Integration
-
Enable Debug Logs: Set the logging level to DEBUG for better insights during troubleshooting. This can reveal hidden issues during the application's startup sequence.
logging.level.org.springframework.amqp=DEBUG
-
Use Try-Catch Blocks: When sending messages, implement error handling mechanisms. This is particularly important in production applications to ensure that failures can be logged and managed effectively.
-
Health Check: Implement health checks to monitor the application’s connection to RabbitMQ. Spring Boot Actuator can aid in providing endpoint details for health status.
-
Keep Dependencies Up to Date: Regularly update your Spring Boot and RabbitMQ client libraries. New versions can include fixes for known issues.
Code Snippets with Commentary
A practical example can clarify how to send and receive messages. Below is a simplified producer and consumer implementation.
Producer Example
@Service
public class MessageProducer {
private final RabbitTemplate rabbitTemplate;
@Autowired
public MessageProducer(RabbitTemplate rabbitTemplate) {
this.rabbitTemplate = rabbitTemplate;
}
public void sendMessage(String message) {
// Use logging to track sent messages
System.out.println("Sending message: " + message);
rabbitTemplate.convertAndSend("myExchange", "my.routing.key", message);
}
}
In this code, the sendMessage
method sends a message to a specified exchange with a defined routing key.
Consumer Example
@Service
public class MessageConsumer {
@RabbitListener(queues = "myQueue")
public void receiveMessage(String message) {
// Handle the received message
System.out.println("Received message: " + message);
}
}
The @RabbitListener
annotation sets up the method to listen for messages arriving on "myQueue".
To Wrap Things Up and Further Reading
Configuring RabbitMQ within a Spring Boot application can present challenges that require careful consideration and troubleshooting. Armed with the right knowledge and practices, developers can seamlessly integrate RabbitMQ into their architectures while overcoming common pitfalls.
For further reading, consider exploring the following resources:
By deploying the solutions discussed, you can ensure a robust configuration of RabbitMQ in your Spring Boot application, paving the way for efficient and reliable message handling in your microservices ecosystem. Happy coding!