Fixing Common Spring Boot and React Integration Issues
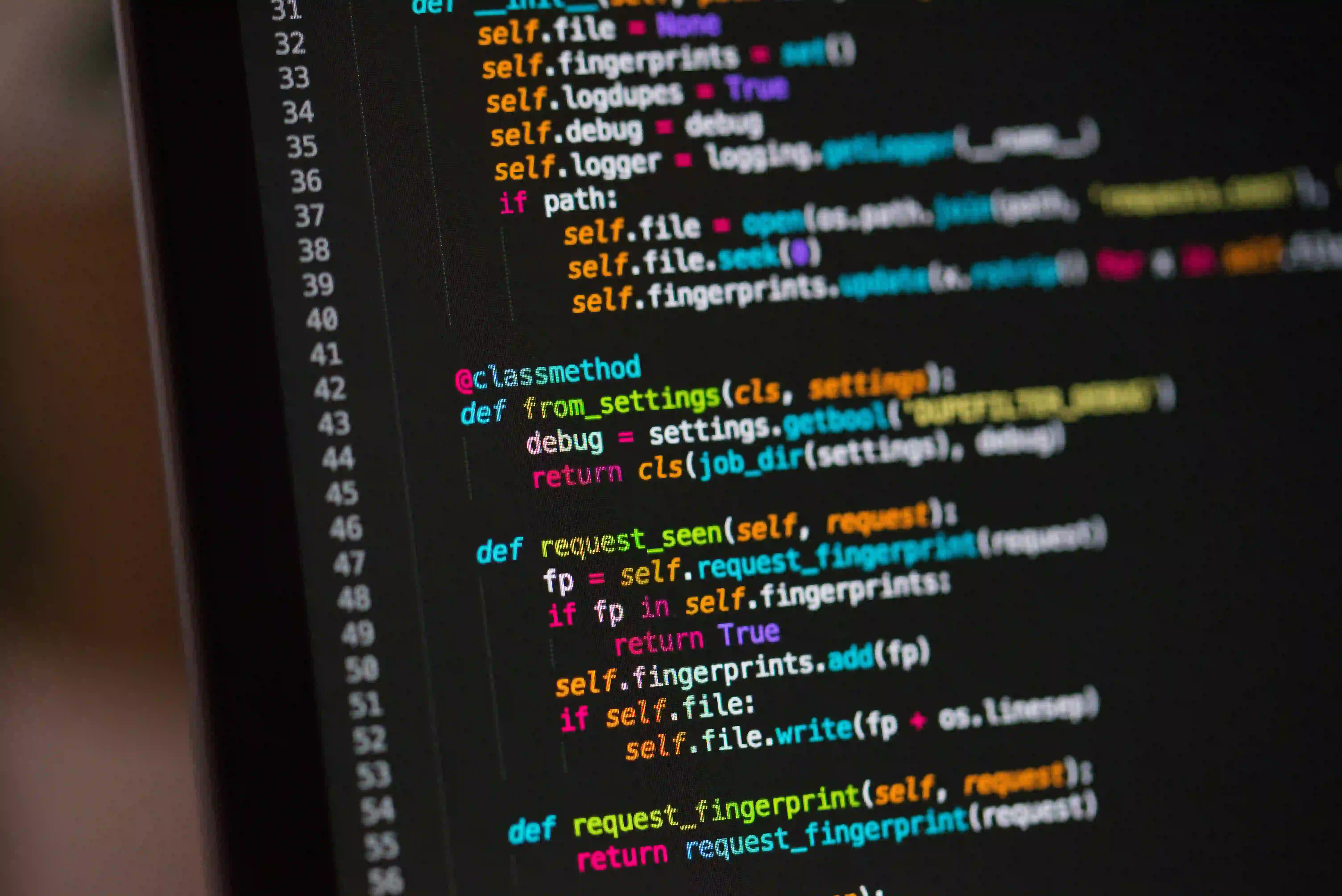
Fixing Common Spring Boot and React Integration Issues
In the world of web development, the synergy between Spring Boot and React has gained immense popularity. Spring Boot, a robust framework for building Java applications, meshes seamlessly with React, a JavaScript library for building user interfaces. Together, they enable developers to create powerful and dynamic web applications. However, this integration is not without its challenges. In this blog post, we will cover common integration issues and how to fix them.
Table of Contents
- Understanding the Stack
- CORS Issues
- Mismatched API Endpoints
- Error Handling
- State Management in React
- Dependency Management
- Conclusion
- Additional Resources
1. Understanding the Stack
Before diving into troubleshooting, let's clarify the tech stack. Typically, Spring Boot will act as your backend API provider, while React serves as the frontend consumer. This separation allows for a clean and modular architecture, but it also requires clear communication between the two ends.
Why Use Spring Boot with React?
- Microservices Architecture: Spring Boot supports building microservices, making it easy to scale your applications.
- CORS Support: It can handle Cross-Origin Resource Sharing (CORS) out of the box.
- Rapid Development: Spring Boot simplifies configuration and setup, allowing for rapid development cycles.
2. CORS Issues
CORS, or Cross-Origin Resource Sharing, is a security feature implemented in web browsers to prevent malicious websites from accessing resources from another domain that the user is currently visiting. When integrating React with Spring Boot, CORS issues may arise if both are hosted on different ports during development.
Solution: Configure CORS in Spring Boot
To allow your React application to communicate with your Spring Boot application, you need to configure CORS. Here’s a simple approach to do it:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // Allow all endpoints
.allowedOrigins("http://localhost:3000") // Your React app's URL
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS") // Allowed HTTP methods
.allowCredentials(true); // Allow credentials
}
}
Why This Matters
This configuration ensures that all requests from your React app to the Spring Boot backend are allowed. Not only does this permit access, but it also enforces security by restricting it to only specified origins and allowed HTTP methods.
3. Mismatched API Endpoints
Another common issue arises when the API endpoints defined in your Spring Boot application do not match what your React frontend is calling. This usually results in 404 errors.
Solution: Verify Correct API Endpoints
Inspect your React service calls. For instance:
import axios from 'axios';
const API_URL = 'http://localhost:8080/api/v1/products';
export const fetchProducts = async () => {
try {
const response = await axios.get(API_URL);
return response.data;
} catch (error) {
console.error('Error fetching products:', error);
throw error;
}
};
Why This Matters
Clearly defining the API URL in your service helps prevent mismatches. Ensure that the path in API_URL
matches the controller mappings in your Spring Boot application.
4. Error Handling
Both Spring Boot and React have their own error handling mechanisms. Commonly, errors in the backend may need to be gracefully communicated to the frontend.
Solution: Global Exception Handling in Spring Boot
Implement a global exception handler in your Spring Boot application:
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
@ControllerAdvice
@RestController
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public String handleGlobalException(Exception ex) {
return ex.getMessage(); // You can customize this response
}
}
Why This Matters
By implementing a global exception handler, you provide a clear response for any errors that occur. This is critical for debugging and user feedback.
5. State Management in React
State management can become complex, especially in larger applications. It’s essential to manage state efficiently to ensure that your React app remains responsive and performs well.
Solution: Use Context API or Libraries
If your application starts to grow in complexity, consider using the Context API or libraries like Redux:
import React, { createContext, useReducer } from 'react';
const StateContext = createContext();
const initialState = { products: [] };
const reducer = (state, action) => {
switch (action.type) {
case 'SET_PRODUCTS':
return { ...state, products: action.payload };
default:
return state;
}
};
export const StateProvider = ({ children }) => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<StateContext.Provider value={{ state, dispatch }}>
{children}
</StateContext.Provider>
);
};
Why This Matters
A well-structured state management solution ensures that the application remains easy to maintain and enhance over time, improving your development experience and end-user performance.
6. Dependency Management
Dependency issues can also arise when your React front end or Spring Boot back end is not properly configured to handle packages or libraries.
Solution: Properly Manage Versions
Keep an eye on the version compatibility between your backend and frontend libraries. For instance, if you are using Spring Boot’s web starter, you might also want to ensure your React version is compatible with other libraries you are using.
In your pom.xml
for Spring Boot:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Other dependencies -->
</dependencies>
And for React, manage your dependencies in package.json
:
{
"dependencies": {
"react": "^17.0.0",
"axios": "^0.21.1",
// Other dependencies
}
}
Why This Matters
Maintaining consistent versions across your tech stack reduces conflicts and errors during development and production.
7. Conclusion
Integrating Spring Boot with React presents a modern approach to building web applications. By addressing CORS issues, ensuring API endpoints are correct, implementing proper error handling, managing state efficiently, and keeping your dependencies in check, you will create a smoother development experience.
As you continue to build and expand your applications, remember these best practices and solutions to encounter fewer integration issues and focus on what you do best—code!
8. Additional Resources
By keeping this playbook in mind, you'll avoid the common pitfalls associated with Spring Boot and React integration, paving the way for a successful project ahead!