Mastering the Elvis Operator in Java 8: A Common Pitfall
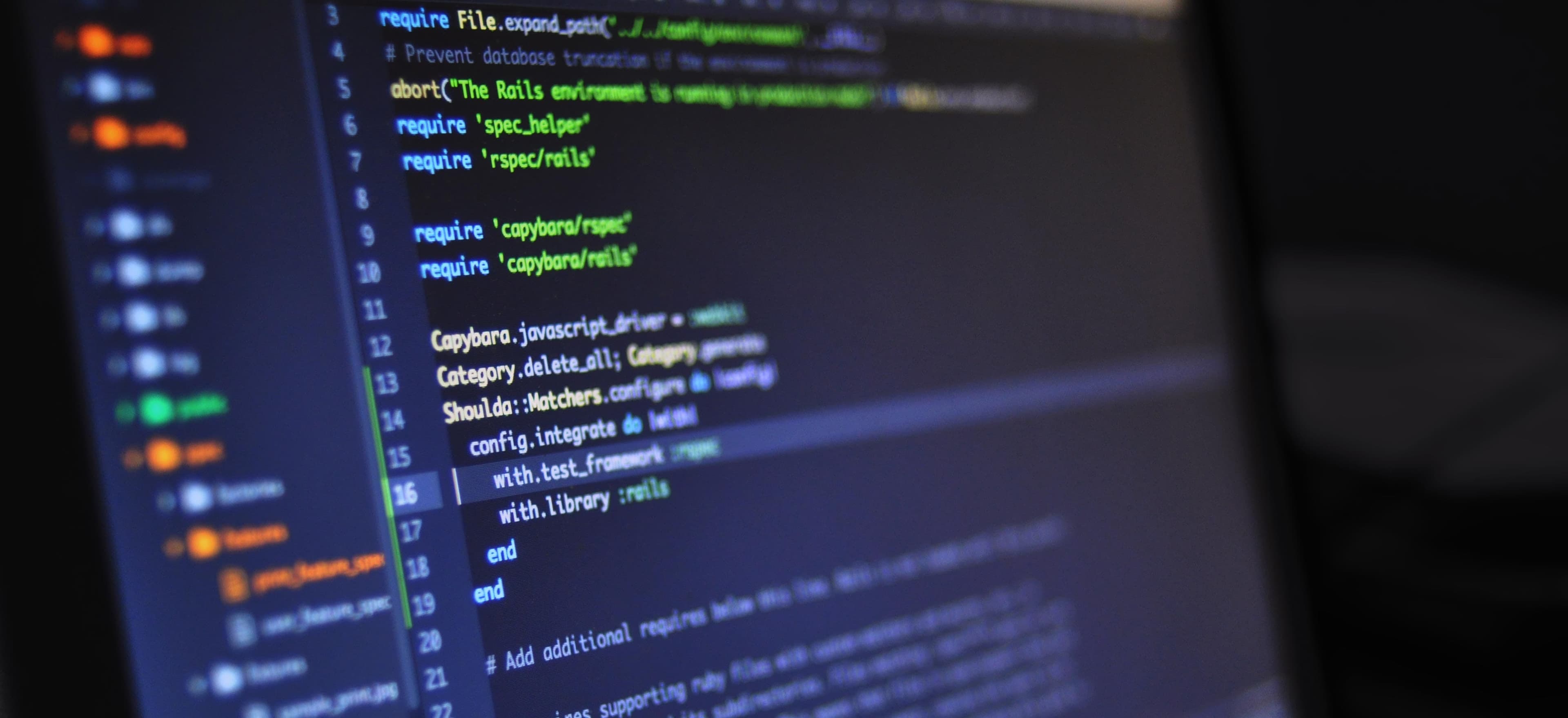
- Published on
Mastering the Elvis Operator in Java 8: A Common Pitfall
Java 8 introduced a variety of new features that streamlined coding practices and enhanced capabilities. Among the most interesting additions is the Elvis operator, a shorthand for null-checking. However, developers often misunderstand or misuse this neat feature. This post aims to clarify the Elvis operator's usage and help you leverage it effectively in your Java code.
What is the Elvis Operator?
The Elvis operator, denoted as ?:
, is a shorthand for the ternary conditional operator. It is primarily used to handle null
values in a more elegant manner. The syntax is simple:
result = (condition) ? valueIfTrue : valueIfFalse;
However, the Elvis operator simplifies this when the left-hand side of the expression is expected to be checked against null
:
result = value != null ? value : defaultValue;
This can be succinctly expressed using the Elvis operator:
result = value ?: defaultValue;
Common Pitfall: Misunderstanding Elvis
Many developers incorrectly assume the Elvis operator can directly replace multiple uses of the ternary operator and should be used in all scenarios involving null checks. This assumption leads to code that is not only confusing but also hard to maintain.
Example of Misuse
Consider the following example:
String username = getUserName(); // This could return null
String displayName = username ?: "Guest"; // Incorrect: works only in Groovy
In Java, this won't compile since Java does not support the Elvis operator in that form. Instead, you should properly use the ternary operator or the Optional class.
Using Optional to Avoid Null Values
A more modern approach recommended in Java 8 is utilizing the Optional
class. It helps alleviate the issues surrounding null checks and provides a more readable and maintainable code structure. Here's how it works:
Creating an Optional
You can create an Optional
instance using the Optional.ofNullable()
method:
Optional<String> optionalUsername = Optional.ofNullable(getUserName());
Accessing Values with Default
You can provide a default value using orElse()
:
String displayName = optionalUsername.orElse("Guest");
This is cleaner and more expressive than using the Elvis operator. It scopes down the possibility of accidental null dereferencing and prepares your code to handle complex data structures elegantly.
Complete Example
Here is a more comprehensive code snippet that demonstrates these concepts:
public class User {
private String username;
public User(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
public class Main {
public static void main(String[] args) {
User user = new User(null); // For testing
// Creating the Optional instance
Optional<String> optionalUsername = Optional.ofNullable(user.getUsername());
// Fetching username with a default value if null
String displayName = optionalUsername.orElse("Guest");
System.out.println("Display Name: " + displayName);
}
}
Breakdown of the Code
-
The
Optional.ofNullable()
method creates anOptional
object. It will hold a non-null username if one exists—otherwise, it'll handle null gracefully. -
The
orElse("Guest")
method specifies the fallback value if the username is absent. This prevents null-related errors. -
Finally, when printing the display name, even if the username is null, it shows "Guest".
This code is cleaner, more maintainable, and protects you from common pitfalls associated with null values.
Real-World Scenarios for Using Elvis and Optional
The Elvis Operator in a Conditionally Detailed Way
Although the Elvis operator's direct use is limited to a select few scenarios, you can still see its varied applications in concise, simple checks. Consider a function returning a user’s role in your application:
public String getUserRole(User user) {
return user.getRole() != null ? user.getRole() : "No Role Assigned";
}
You can express it in one line with the Elvis operator into Groovy, but in Java, always favor clarity and maintainability.
When to Use Optional
The Optional
class shines when you intend to handle potentially missing data:
-
Return Types: When a method may not find a result, use Optional as a return type.
-
Chained Methods: Optionals allow you to chain methods elegantly, minimizing the risk of null pointer exceptions.
Avoiding Nested Optionals
A common mistake is creating nested Optionals. Always flatten your structures where possible:
// Bad Practice
Optional<Optional<String>> user = getUserNameAsOptional(); // Avoid this
// Good Practice
Optional<String> user = getUserNameAsOptional().flatMap(Optional::ofNullable);
In this context, use map
or flatMap
only when necessary, promoting cleaner code.
Closing the Chapter
The Elvis operator offers a shorthand way of handling null values but is often misapplied by Java developers, potentially leading to confusion and decreased code clarity. Instead, prioritizing the usage of Optional
in Java 8 helps you manage null prevention better.
Utilizing the Optional
class enables more robust and elegant code. Remember to write not just for functionality, but also for readability and maintainability. This promotes clearer communication among developers and aids long-term project success.
For more insights on effective null handling in Java, check out the Java documentation on Optional.
With this knowledge, go forth and master your handling of null values in Java. Happy coding!