How to Resolve Stuck Threads in WebLogic Efficiently
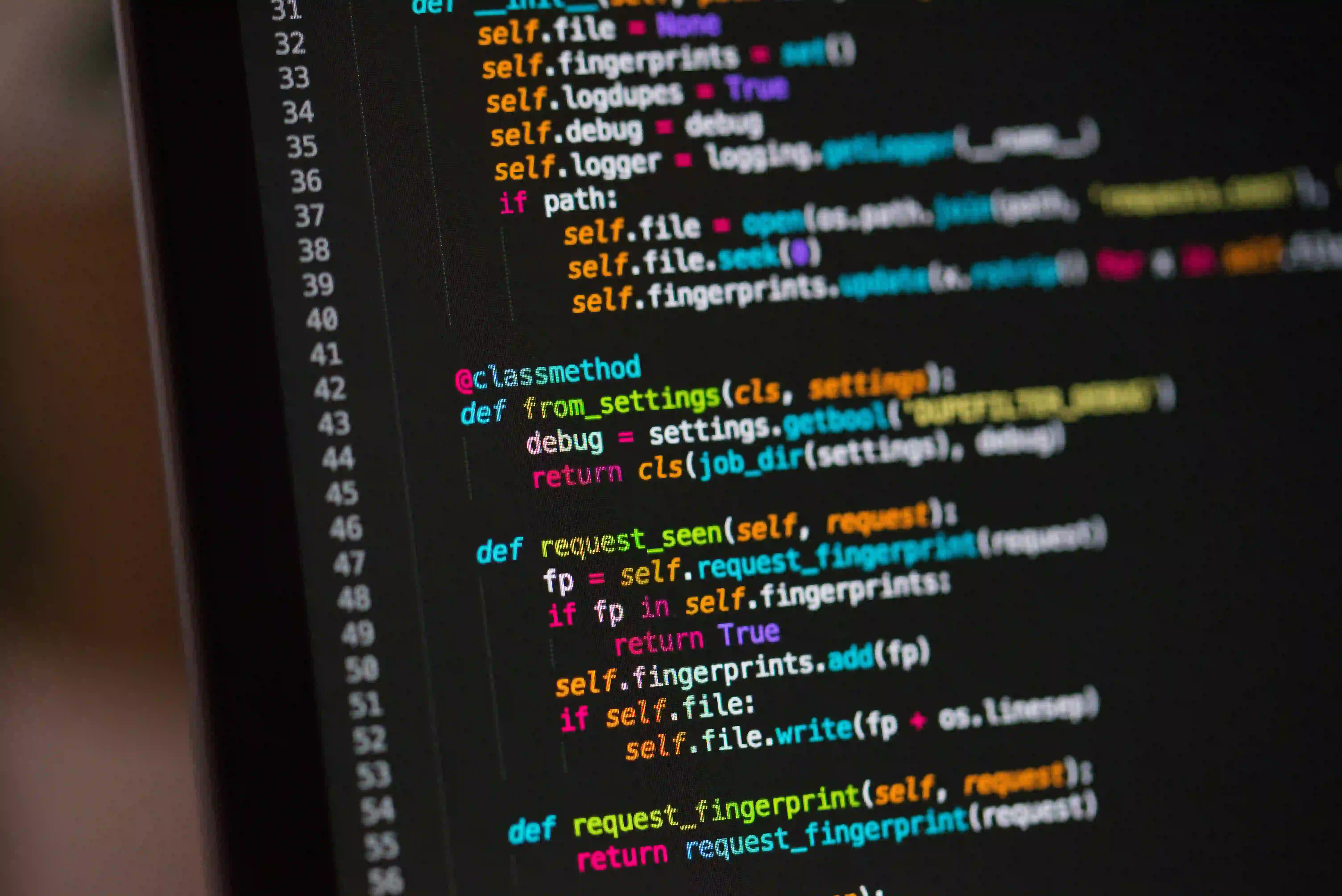
How to Resolve Stuck Threads in WebLogic Efficiently
WebLogic Server is a powerful and widely-used application server that provides a robust platform for running enterprise-level applications. However, like any complex system, it can be plagued by issues, one of which is the phenomenon of "stuck threads." Stuck threads occur when threads in the server are unable to complete their tasks within a specified time frame, potentially causing performance degradation and other system problems. In this blog post, we will explore what stuck threads are, how to identify them, analyze their causes, and implement efficient solutions.
What are Stuck Threads?
In Java-based applications, threads are essential for handling concurrent tasks. A "stuck thread" can be defined as a thread that exceeds a predetermined threshold of execution time without completing its work. In WebLogic, a stuck thread can lead to several problems:
- Application Slowdown: If threads are stuck, application responsiveness can diminish.
- Resource Blockage: The server may begin consuming resources inefficiently.
- Increased Load Times: Users may experience longer load times due to delays in request handling.
Identifying Stuck Threads
Before resolving stuck threads, it is crucial to identify them. WebLogic provides several tools for monitoring thread status:
-
WebLogic Console: In the WebLogic Administration Console, navigate to "Environment" and then "Servers." By selecting a server and clicking on the "Monitoring" tab, you can access thread information.
-
JVisualVM: This monitoring tool allows you to visualize Java threads running within the JVM.
-
Thread Dumps: You can generate thread dumps using
jstack
or within WebLogic itself. Command-line access gives you detailed insights into thread states.
How to Generate a Thread Dump
To generate a thread dump for a running Java application, you can execute the following command:
jstack <pid>
Here, <pid>
is the process ID of your Java application. Generating a thread dump at intervals during peak loads can provide valuable insights into thread states.
Analyzing Thread Dumps
Once you have the thread dump, the next step is to analyze it. Look for threads in the BLOCKED
or RUNNABLE
states. You might encounter code snippets that look something like this:
// A visual representation of a thread stack in a thread dump
"Thread-1" #12 prio=5 os_prio=0 tid=0x00007f4a34c99800 nid=0x1b7e waiting for monitor entry (a java.lang.Object)
java.lang.Thread.State: BLOCKED (on object monitor)
at com.example.MyClass.mySynchronizedMethod(MyClass.java:50)
- waiting to lock <0x00000000c03c2898> (a java.lang.Object)
at com.example.MyServlet.doGet(MyServlet.java:30)
...
Key Indicators to Look For
- BLOCKED State: Indicates that the thread is waiting for a lock held by another thread.
- RUNNABLE State: The thread is active but doesn't make forward progress because it might be busy waiting or encountering other issues.
Common Causes of Stuck Threads
-
Synchronized Methods: Overuse of synchronized blocks can cause threads to wait unnecessarily.
-
Infinite Loops: Threads caught in infinite loops may never reach completion.
-
Deadlocks: Occurs when two or more threads are waiting on each other to release resources.
-
Long-Running Tasks: Tasks that are expected to run quickly but take longer than anticipated due to heavy processing or external service calls.
Resolving Stuck Threads
1. Code Optimization
Always look to refine the blocks of code that might be blocking threads. For example, consider using Java Concurrency API or async/await patterns where suitable instead of relying on synchronized methods.
// Using a ReentrantLock instead of synchronized for better control
ReentrantLock lock = new ReentrantLock();
public void safeMethod() {
lock.lock();
try {
// critical section
} finally {
lock.unlock();
}
}
Why Use ReentrantLock?
- It allows more flexibility and can be controlled better compared to synchronization.
2. Timeout Settings
WebLogic allows you to set timeouts for thread execution. Adjusting these configurations can help recover threads stuck in lengthy operations.
<timeout>
<web-service>
<operation-timeout>10000</operation-timeout> <!-- Timeout set to 10 seconds -->
</web-service>
</timeout>
3. Asynchronous Processing
For long-running operations, consider utilizing asynchronous processing techniques. This will enable threads to be freed for other tasks while waiting for high-latency operations to complete.
public CompletableFuture<String> asyncProcess() {
return CompletableFuture.supplyAsync(() -> {
// Simulate long processing
return "Processed";
});
}
Why Use CompletableFuture?
- It allows non-blocking operations and enhances performance by using pool threads effectively.
4. Regular Monitoring
Through JMX (Java Management Extensions) and monitoring tools, maintain vigilance over your application. Tools like Prometheus and Grafana can be integrated to keep metrics in check, alerting when numbers deviate from normal thresholds.
Closing the Chapter
Resolving stuck threads in WebLogic involves a comprehensive strategy that includes monitoring, optimizing code, and adopting best practices. The key is to be proactive in identifying thread issues early and employing solutions like proper thread management, code optimizations, and timely responses to monitor alerts.
By following these guidelines, you can maintain a durable and responsive WebLogic application environment.
References:
Staying informed and ahead of these issues will not only improve your application's performance but also enhance user satisfaction. Happy coding!