Mastering Delayed Queues: Tackling Common Implementation Issues
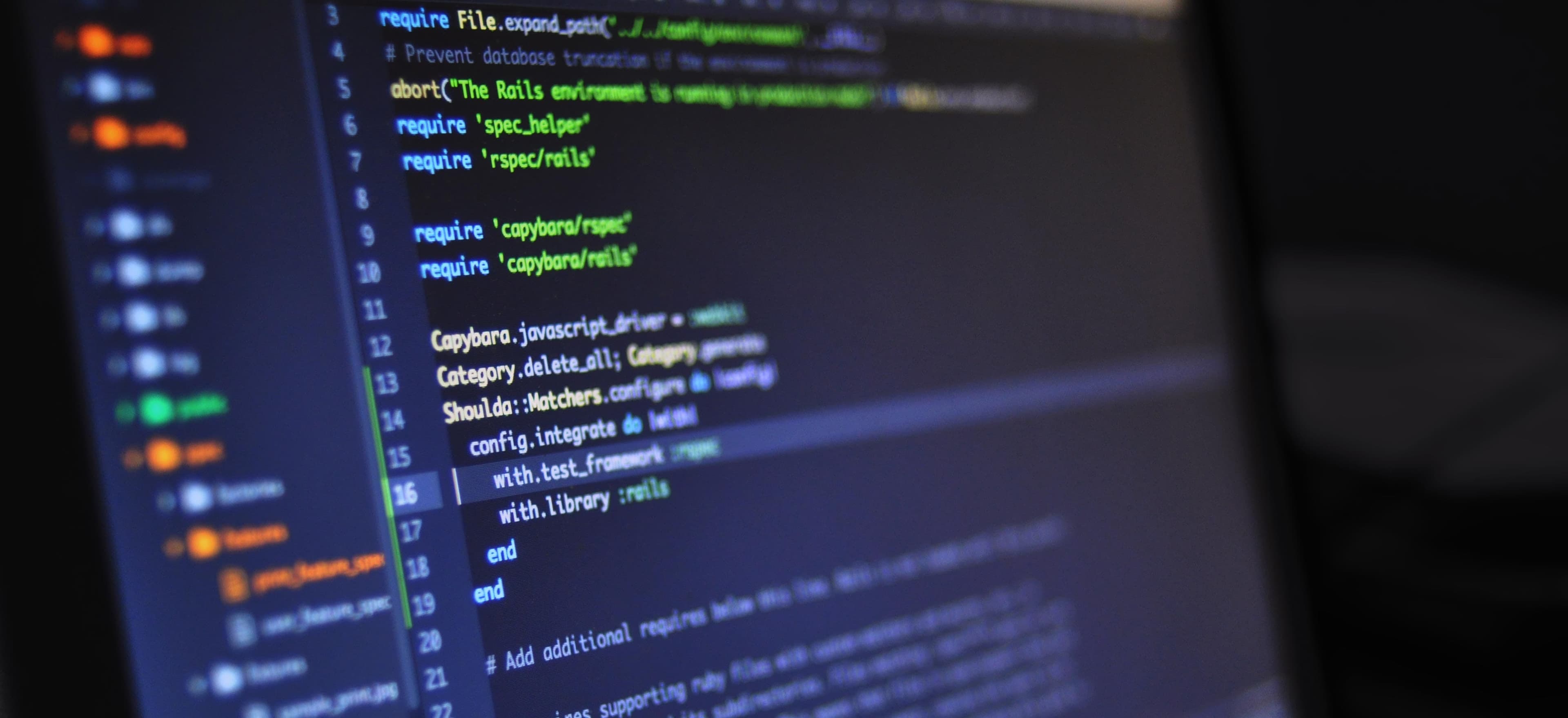
- Published on
Mastering Delayed Queues: Tackling Common Implementation Issues
In today's fast-paced software development landscape, the demand for efficient message processing has increased significantly. One of the essential components in asynchronous processing systems is the delayed queue. This blog post delves into the intricacies of delayed queues in Java, touching on common implementation issues and providing practical solutions.
Understanding Delayed Queues
A delayed queue is essentially a messaging system designed to hold messages for a specified amount of time before making them available for consumption. This is particularly useful for use cases like task scheduling, where certain tasks should only execute after a designated delay.
Why Use Delayed Queues?
- Task Scheduling: Allows developers to schedule tasks that must occur after a specific timeframe.
- Rate Limiting: Helps control the rate at which messages are processed, reducing the risk of overwhelming downstream systems.
- Retry Mechanisms: Provides control over how often a message is retried after a failure.
Java provides several libraries and frameworks that support delayed queues, including the Java Concurrent Library and external libraries like Apache Kafka and RabbitMQ.
The Java Concurrent Library: DelayedQueue
The DelayQueue
class from Java's java.util.concurrent
package is commonly used to implement delayed queues. Here's a simple overview of how it works:
import java.util.concurrent.DelayQueue;
import java.util.concurrent.Delayed;
import java.util.concurrent.TimeUnit;
class DelayedTask implements Delayed {
private final long delayTime;
private final long creationTime;
private final String taskName;
public DelayedTask(long delay, String taskName) {
this.delayTime = delay;
this.creationTime = System.currentTimeMillis();
this.taskName = taskName;
}
@Override
public long getDelay(TimeUnit unit) {
long diff = creationTime + delayTime - System.currentTimeMillis();
return unit.convert(diff, TimeUnit.MILLISECONDS);
}
@Override
public int compareTo(Delayed o) {
if (this.getDelay(TimeUnit.MILLISECONDS) < o.getDelay(TimeUnit.MILLISECONDS)) {
return -1;
}
if (this.getDelay(TimeUnit.MILLISECONDS) > o.getDelay(TimeUnit.MILLISECONDS)) {
return 1;
}
return 0;
}
@Override
public String toString() {
return "Task: " + taskName;
}
}
Code Explanation
-
Delayed Interface: By implementing the
Delayed
interface, theDelayedTask
class can specify delay logic. -
getDelay Method: This method calculates the delay remaining until the task can be executed. It's crucial for determining when to release the task from the queue.
-
compareTo Method: This is used to define the order of elements in the queue based on their delay.
-
Basic Setup: To add a task to
DelayQueue
, you can do so as follows:
DelayQueue<DelayedTask> delayQueue = new DelayQueue<>();
delayQueue.offer(new DelayedTask(5000, "Task 1")); // 5 seconds delay
Using DelayedQueue
Here’s how you can setup a consumer that will process tasks from the DelayQueue
:
public class DelayedQueueExample {
public static void main(String[] args) {
DelayQueue<DelayedTask> delayQueue = new DelayQueue<>();
// Adding tasks with different delays
delayQueue.offer(new DelayedTask(3000, "Task 1")); // 3 seconds delay
delayQueue.offer(new DelayedTask(1000, "Task 2")); // 1 second delay
delayQueue.offer(new DelayedTask(2000, "Task 3")); // 2 seconds delay
// Consumer that processes tasks
new Thread(() -> {
try {
while (true) {
DelayedTask task = delayQueue.take();
System.out.println("Processing: " + task);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
}
}
Important Notes
-
Blocking Behavior: The
take()
method will block until a task becomes available, making it an effective design for a message-consuming thread. -
Thread Safety:
DelayQueue
is thread-safe. Multiple threads can safely insert and remove objects simultaneously.
Common Implementation Issues
Implementing delayed queues in Java is not without its challenges. Here are some common issues and their solutions.
1. Task Timeout and Precision
Java’s system clock can result in imprecision. If tasks do not execute precisely after their intended delay, it could confuse your scheduling logic.
Solution
Consider using scheduling frameworks like Spring's @Scheduled
annotations or Quartz
, which offer more control and reliability over timing:
@Scheduled(fixedDelay = 1000)
public void scheduledTask() {
// Logic to execute periodically
}
2. Memory Leaks
Large volumes of tasks can lead to memory issues if they aren't handled correctly.
Solution
Always ensure that tasks are removed from the queue after processing. If a task fails, implement a retry policy that removes them after N retries, preventing them from accumulating.
3. Scaling Issues
As the volume of messages increases, a single-threaded consumer might become a bottleneck.
Solution
You can use a Thread Pool Executor to manage multiple consumer threads more effectively:
ExecutorService executor = Executors.newFixedThreadPool(5);
while (!delayQueue.isEmpty()) {
executor.submit(() -> {
try {
DelayedTask task = delayQueue.take();
System.out.println("Processing: " + task);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
Best Practices
- Monitor and Log: Regularly log the performance and health of your queue system.
- Avoid Long-Running Tasks: The consumer thread should not perform long-running tasks to ensure responsiveness.
- Configure Delays Carefully: Use a sensible granularity to configure your delays to avoid timer precision issues.
Closing Remarks
Mastering delayed queues in Java can significantly enhance your application’s messaging capabilities. By understanding the underlying mechanisms and common pitfalls, you can build robust systems that handle asynchronous processing seamlessly.
For further reading, consider checking out:
Feel free to share your own experiences or challenges you've faced with delayed queues in Java in the comments below! Happy coding!