Avoiding the Pitfalls of Double-Checked Locking in Java
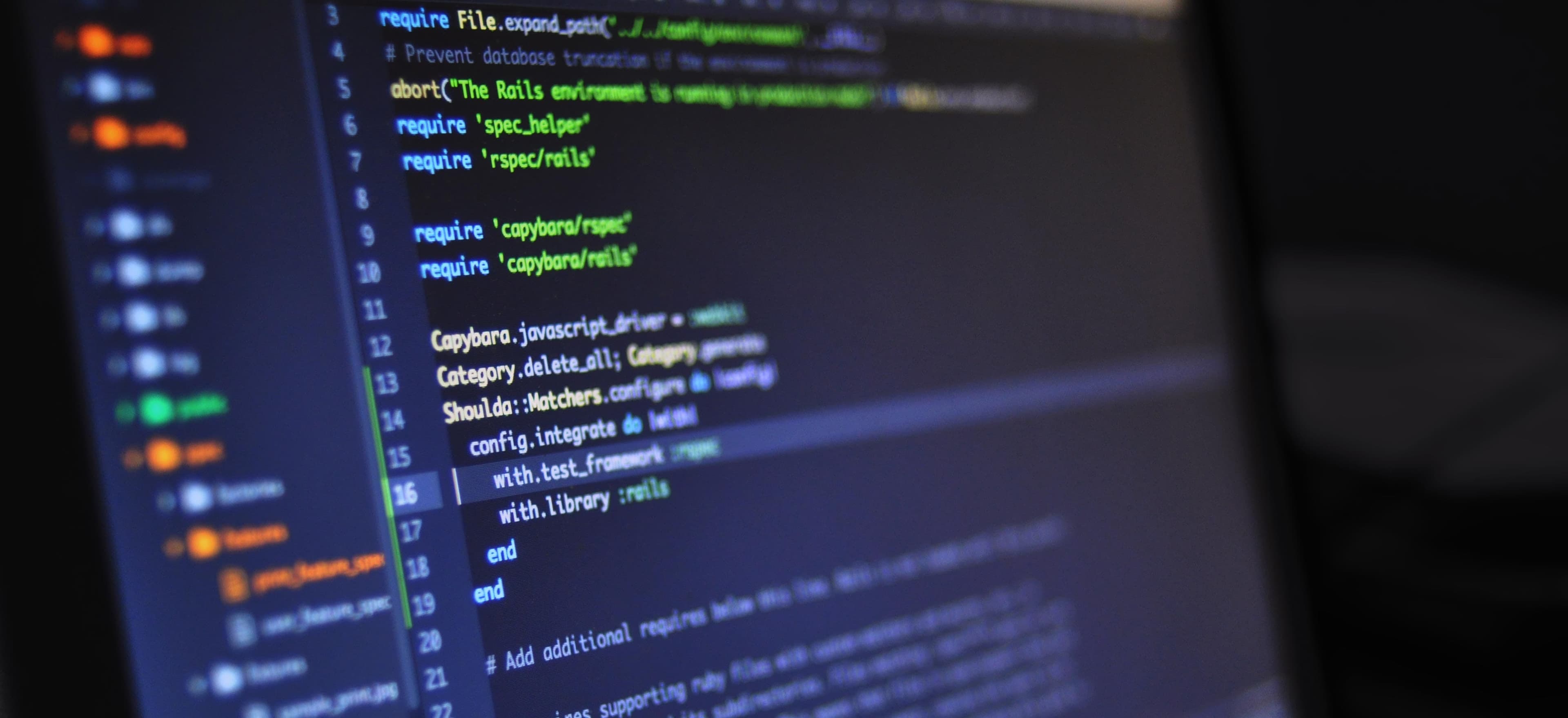
- Published on
Avoiding the Pitfalls of Double-Checked Locking in Java
When it comes to creating singleton classes in Java, many developers rush to implement the double-checked locking mechanism. Although it appears to be an efficient way to control access to a shared resource, it often introduces subtle bugs and complexities that can lead to unexpected behaviors in multithreaded environments.
In this blog post, we'll explore the issues surrounding double-checked locking, why they matter, and how to use alternatives to ensure thread safety. We'll dive into clear examples, practice discussions, best practices, and effective solutions within Java.
Understanding Double-Checked Locking
Double-checked locking means checking if a resource has already been initialized before entering a synchronized block. If the resource is not initialized, it locks the resource to initialize it.
public class Singleton {
private static volatile Singleton instance;
private Singleton() {
// private constructor
}
public static Singleton getInstance() {
if (instance == null) { // First check (no synchronization)
synchronized (Singleton.class) {
if (instance == null) { // Second check (synchronized)
instance = new Singleton(); // Create instance
}
}
}
return instance;
}
}
Why Double-Checked Locking?
-
Performance: Reduces the overhead of acquiring a lock by allowing threads to bypass synchronization when the instance is already initialized.
-
Memory Use: Optimizes resource usage. The locking mechanism is only engaged when it's absolutely necessary.
The Problem with Double-Checked Locking
The primary issue with using double-checked locking arises from how Java's memory model handles object initialization. Specifically, when a thread allocates memory for an object and invokes its constructor, other threads may see a partially constructed object before the constructor completes.
This can lead to situations where a thread retrieves a non-null value from a singleton instance, but crucial fields may not have been initialized yet. Consider the following problematic scenario:
public class Singleton {
private static volatile Singleton instance;
private String value;
private Singleton() {
value = "Initialized Value";
}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton(); // Object reference may be visible before constructor finishes
}
}
}
return instance;
}
public String getValue() {
return value; // Can return null or a default value if not completely initialized
}
}
In this example, if a thread invokes getValue()
too early, it may obtain a default value instead of the properly initialized one.
Alternatives to Double-Checked Locking
1. Use Enum Singleton
One of the simplest and safest ways to implement a singleton in Java is through the use of an enum. This approach provides built-in serialization and guarantees that only one instance is created, regardless of how many times the singleton is referenced.
public enum Singleton {
INSTANCE;
private String value = "Initialized Value";
public String getValue() {
return value;
}
}
This method ensures that the INSTANCE
is created just once when the enum class is loaded, guaranteeing thread safety without any additional synchronization.
2. Initialization-on-Demand Holder Idiom
Another effective approach is to utilize the Initialization-on-Demand Holder Idiom. This pattern leverages the class loader mechanism to ensure thread-safe instantiation without requiring synchronization.
public class Singleton {
private Singleton() {
// private constructor
}
private static class Holder {
private static final Singleton INSTANCE = new Singleton();
}
public static Singleton getInstance() {
return Holder.INSTANCE;
}
}
In this pattern, the inner static class Holder
contains the singleton instance. The instance is only created when the getInstance()
method is called, enabling efficient lazy initialization while remaining thread-safe.
Key Takeaways
-
Avoid the Complexity of Double-Checked Locking: While it promises performance gains, its downsides far outweigh the benefits. The potential for subtle bugs makes it less desirable than straightforward methods.
-
Favor Immutable Objects: Immutable singletons, such as those created with enums, are inherently simpler and safer.
-
Initialization-on-Demand Holder Idiom: This idiom offers both lazy initialization and thread safety, making it an excellent alternative.
-
Know the Java Memory Model: Understand how the Java Memory Model manages object visibility. Knowing this helps avoid pitfalls in multithreading environments.
The Last Word
Double-checked locking is a common pattern that developers might resort to when creating singleton instances in Java. Unfortunately, it could lead to unexpected behaviors and difficulties in thread safety. By using enums or the initialization-on-demand holder idiom, you can create thread-safe singletons without the pitfalls inherent in double-checked locking.
To dive deeper into the nuances of Java's concurrency mechanisms and related topics, check out Java Concurrency in Practice for more comprehensive insights or visit Baeldung for practical examples.
Embrace straightforward patterns! Your code will not only be cleaner; it will also be more robust and maintainable. Happy coding!
Checkout our other articles