How the Memento Pattern Can Fix Data Management Woes
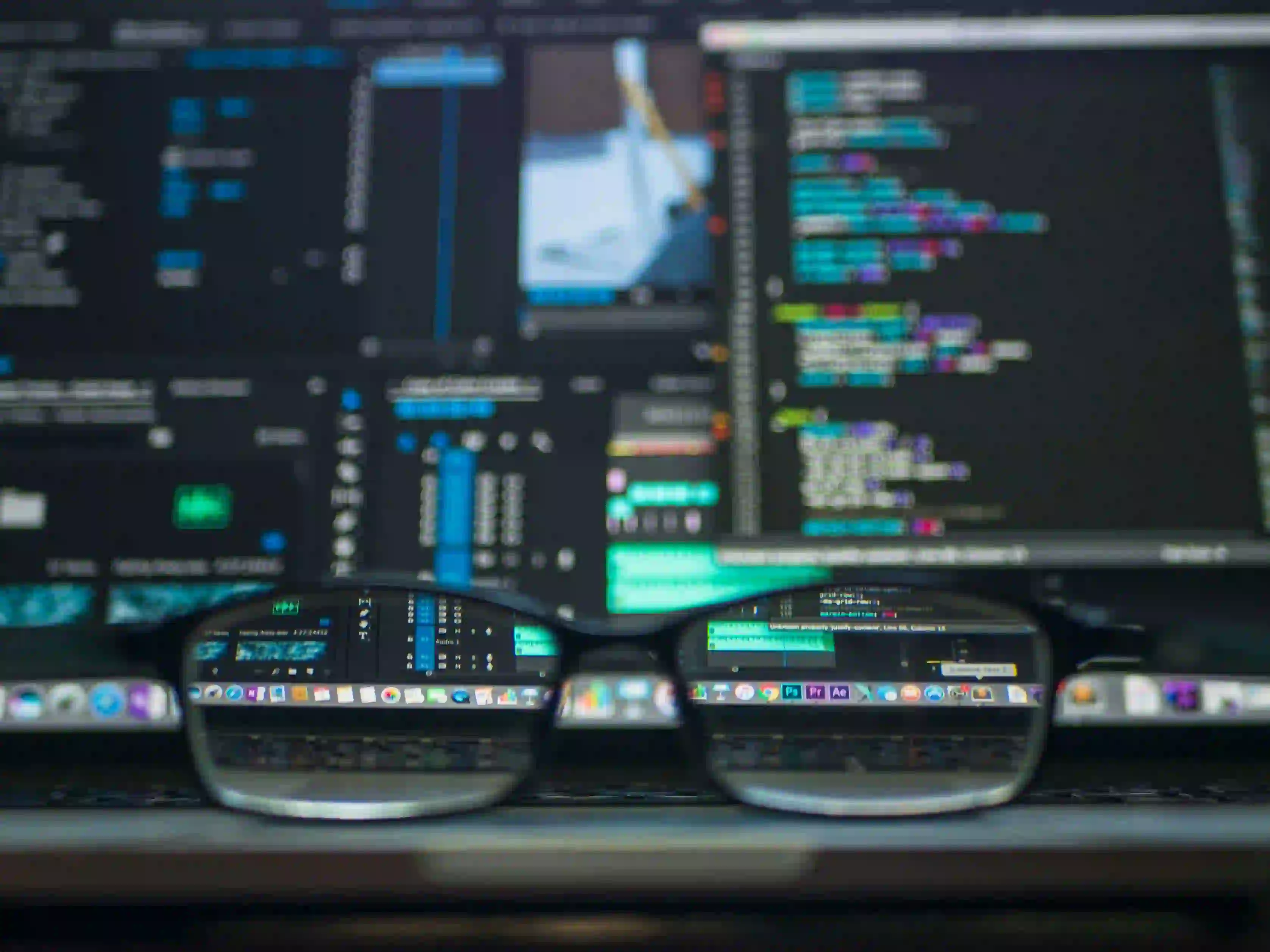
How the Memento Pattern Can Fix Data Management Woes
Data management is a core aspect of software development. With each passing day, applications become more complex, and the need for a reliable way to manage the state of an application becomes increasingly crucial. One design pattern that stands out for its effectiveness in this realm is the Memento Pattern.
In this blog post, we will explore what the Memento Pattern is, how it operates, and how it can help developers manage data more efficiently, while also ensuring that the behavior of our applications remains clean and comprehensible.
What is the Memento Pattern?
The Memento Pattern is a behavioral design pattern that allows an object to capture its internal state at a specific time, allowing the object to revert back to this state later. This is particularly useful for applications that require undo/redo functionality, or need to manage complex states without exposing their internal representation.
Key Components of the Memento Pattern
- Originator: This is the object that holds the internal state.
- Memento: This encapsulates the state of the Originator and stores it. Importantly, it should expose only the data necessary for the Originator to restore its state, thereby safeguarding against unwanted data exposure.
- Caretaker: This maintains the history of Mementos for the Originator. It can request a Memento from the Originator and returns it whenever needed.
Why Use the Memento Pattern?
The Memento Pattern is beneficial for various reasons:
- Encapsulation: It hides the details of the Originator's state. This means a client can save and restore states without needing to understand the inner workings of that state.
- Undo/Redo Operations: It simplifies the implementation of undo/redo functionalities. Users can easily step back to previous states, enhancing the usability of applications.
- Complex State Management: In many applications, an object might have complex states that need to switch back and forth. The Memento Pattern facilitates this process without cluttering the object’s code.
Implementing the Memento Pattern in Java
Let’s delve into a practical Java implementation of the Memento Pattern. For this example, consider a simple text editor that can save its state and revert back to it.
Step 1: Create the Memento Class
The Memento class stores the state of the Originator. This class is simple and intentionally has a limited interface to prevent access to its state from outside.
public class Memento {
private final String state;
public Memento(String state) {
this.state = state;
}
public String getState() {
return this.state;
}
}
Why? Encapsulation of the state ensures that other classes cannot directly modify it; they can only retrieve it through a well-defined interface.
Step 2: Create the Originator Class
The Originator is the object whose state we need to save and restore. Here is how it would look:
public class Originator {
private String state;
public void setState(String state) {
System.out.println("Setting state to: " + state);
this.state = state;
}
public String getState() {
return this.state;
}
public Memento saveStateToMemento() {
System.out.println("Saving state: " + state);
return new Memento(state);
}
public void getStateFromMemento(Memento memento) {
state = memento.getState();
System.out.println("State restored to: " + state);
}
}
Why? The Originator class allows us to both set an internal state and manage Memento creation and restoration. This provides a clean separation of responsibilities.
Step 3: Create the Caretaker Class
The Caretaker manages the Memento. It saves and retrieves Mementos without knowing the specifics of the Originator's state.
import java.util.ArrayList;
import java.util.List;
public class Caretaker {
private List<Memento> mementoList = new ArrayList<>();
public void add(Memento state) {
mementoList.add(state);
}
public Memento get(int index) {
return mementoList.get(index);
}
}
Why? List management here provides a straightforward way to keep track of various states over time, enabling Undo operations by leveraging indexed access.
Step 4: Demo the Memento Pattern
Now let's see this pattern in action in a main
method.
public class Main {
public static void main(String[] args) {
Originator originator = new Originator();
Caretaker caretaker = new Caretaker();
originator.setState("State #1");
caretaker.add(originator.saveStateToMemento());
originator.setState("State #2");
caretaker.add(originator.saveStateToMemento());
originator.setState("State #3");
System.out.println("Current State: " + originator.getState());
// Restore to last saved state
originator.getStateFromMemento(caretaker.get(1));
System.out.println("Restored to: " + originator.getState());
}
}
Output
When you run the above code, it will show the output like:
Setting state to: State #1
Saving state: State #1
Setting state to: State #2
Saving state: State #2
Setting state to: State #3
Current State: State #3
State restored to: State #2
Restored to: State #2
Why? This demonstrates the core functionality of the Memento Pattern, facilitating state management in our text editor scenario effectively.
Real-World Applications
The Memento Pattern can be found in various applications, including:
- Text Editors: As illustrated, editors often need to provide undo/redo function.
- Game Development: Saving the status of player positions, scores, and other variables allows for a rollback to a prior game state.
- Version Control Systems: Such as Git, where commits can be viewed and reverted.
Final Considerations
The Memento Pattern offers a powerful solution to data management woes by allowing for effective state preservation and restoration. It retains encapsulation, supports complex state management, and simplifies the implementation of the undo/redo features.
Incorporating this pattern into your software design can lead to more maintainable and scalable applications. Understanding when and how to use it is crucial for a developer's toolkit; after all, managing complexity is part of the challenge we face in building robust software.
For those looking to dive deeper into design patterns, consider checking out related Java design patterns like the Observer Pattern and the Command Pattern, which also offer valuable solutions for complex system interactions.
By embracing established design patterns such as the Memento, we can tackle data management challenges with confidence, and create applications that are not only functional but also elegant.