Common Pitfalls When Passing Parameters to JSF Action Methods
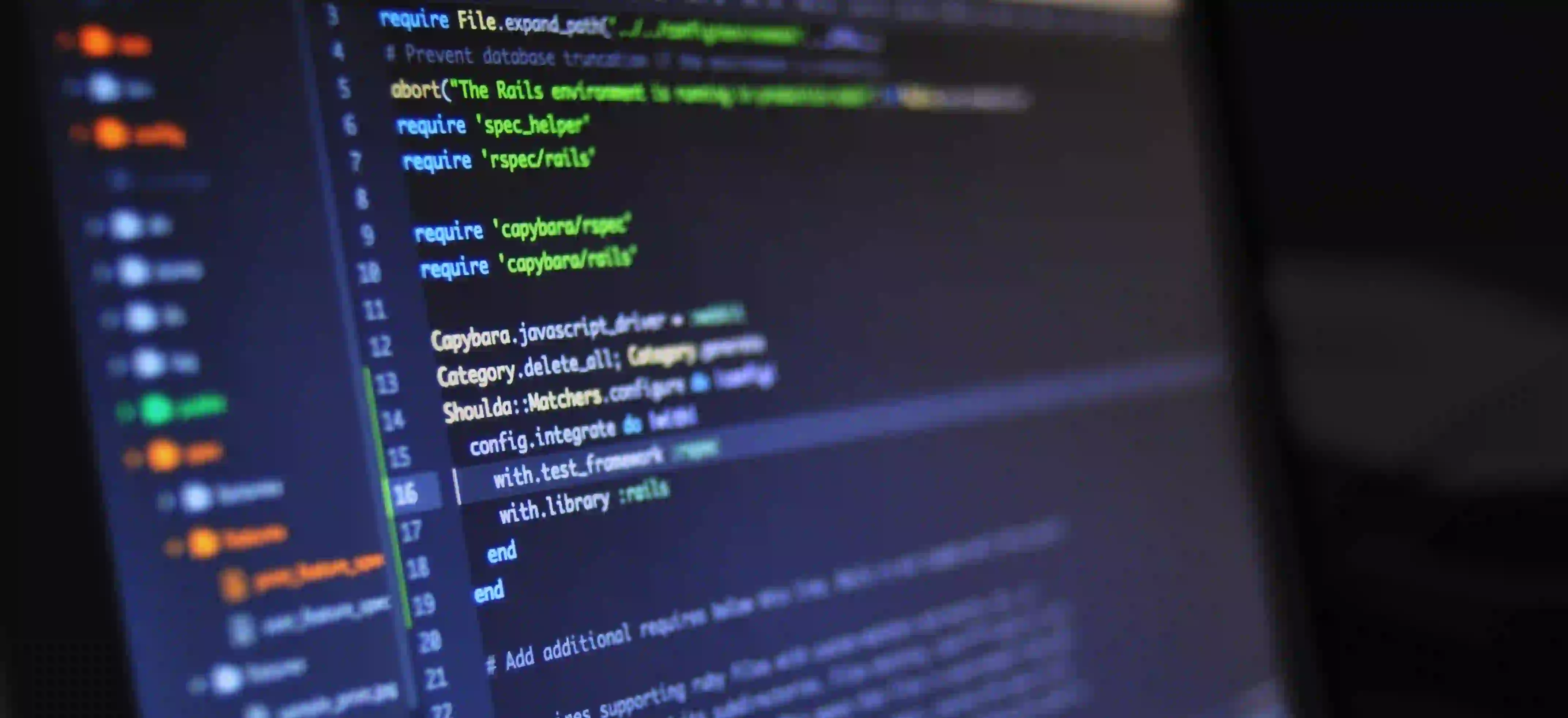
Common Pitfalls When Passing Parameters to JSF Action Methods
JavaServer Faces (JSF) is a robust framework that simplifies the development of user interfaces for Java web applications. However, as with any framework, there are common pitfalls developers encounter, especially when passing parameters to action methods. In this blog post, we’ll go through some of these common issues and how you can avoid them to ensure smooth navigation and robust functionality in your JSF applications.
Understanding JSF Action Methods
Before diving into the pitfalls, let's establish a brief overview of JSF action methods. An action method is a method that is invoked when a user interacts with a JSF component, such as a button. This is typically achieved via an action attribute in a JSF tag.
Here’s a simple example of an action method:
@ManagedBean
@ViewScoped
public class MyBean {
public String myAction() {
// Logic for action
return "success"; // For navigation
}
}
In this example, when a user clicks a button associated with myAction()
, the method is executed, and the user is navigated to the "success" page.
Common Pitfalls
1. Confusing Return Types
A frequent mistake is misunderstanding the return type of an action method. In JSF, the return type is crucial as it determines the navigation outcome.
Example of Incorrect Usage
public String myAction() {
// Some logic here
return null; // This can lead to unexpected results
}
Returning null
from an action method may simply navigate to the current page, not allowing the user to go anywhere. You should aim to return a proper navigation string or a mapped outcome.
2. Not Using the @ViewScoped
Annotation
Another common pitfall is failing to utilize the appropriate scope for your backing beans. The @ViewScoped
annotation allows your bean to maintain its state while the user interacts with the same view.
Example:
@ManagedBean
@ViewScoped
public class MyBean {
private String value;
public void processValue() {
// Handle the logic
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
If you do not specify the scope, the bean might be re-instantiated on every request, losing valuable information. Using @ViewScoped
is vital for keeping data during a user session as they interact with the view.
3. Parameter Binding Issues
When passing parameters to action methods, mishandling parameter binding can lead to issues. Ensure the parameters are defined and bound correctly to the action method.
Incorrect Example
<h:commandButton value="Submit" action="#{myBean.myAction(parameter)}"/>
If parameter
is not defined as a component in your JSF page, this action method will not receive any valid data, leading to a potential NullPointerException
.
Correct Example
Make sure the parameter is defined:
<h:inputText id="parameter" value="#{myBean.parameterValue}" />
<h:commandButton value="Submit" action="#{myBean.myAction(myBean.parameterValue)}"/>
In the above, we bind an input text to the bean, ensuring the action method receives the correct parameter.
4. Ignoring Form Validation and Conversion Errors
JSF automatically handles form validation and conversion, but if you ignore these processes, it can create unexpected results, especially when dealing with non-string input (like dates or numbers).
Example with Validation
<h:inputText id="age" value="#{myBean.age}" required="true" validator="#{myBean.validateAge}"/>
<h:commandButton value="Submit" action="#{myBean.myAction}"/>
In the Java class:
public void validateAge(FacesContext context, UIComponent component, Object value) {
Integer age = (Integer) value;
if (age < 18) {
throw new ValidatorException(new FacesMessage("Age must be 18 or older."));
}
}
Always include validation methods to ensure incoming parameters are valid before processing them.
5. Forgetting to Check for null
and empty
Parameters
It's vital to check for null
or empty parameters in Java action methods, especially if they come from user input. Failing to do so can create exceptions and broken functionality.
Example:
public String myAction(String parameter) {
if (parameter == null || parameter.isEmpty()) {
FacesContext.getCurrentInstance().addMessage(null, new FacesMessage("Parameter cannot be empty"));
return null; // Stay on the same page
}
// Proceed with logical flow
}
This ensures your application gracefully handles incorrect inputs and informs the user of the issue.
6. Improper Use of Navigation Rules
If you are using JSF navigation rules in combination with action methods, ensure that the configured rules address the expected outcomes.
Example of Incorrect Navigation Rule:
<navigation-rule>
<from-view-id>/start.xhtml</from-view-id>
<navigation-case>
<from-outcome>success</from-outcome>
<to-view-id>/success.xhtml</to-view-id>
</navigation-case>
</navigation-rule>
If there’s a mismatch between the action method's return value and what's specified in your navigation rules, users may be directed improperly.
7. Ignoring Exception Handling
Unhandled exceptions during action method invocation can lead to poor user experience. It's critical to implement global exception handling for a smoother user interface.
public String myAction() {
try {
// Action logic here
return "success";
} catch (Exception e) {
FacesContext.getCurrentInstance().addMessage(null,
new FacesMessage(FacesMessage.SEVERITY_ERROR, "Error!", e.getMessage()));
return null; // Stay on the same page with error message
}
}
This provides feedback to users, making the application more resilient.
A Final Look
Passing parameters to JSF action methods can be highly effective if you avoid common pitfalls. By understanding the control flow, scoping, and validation requirements, you can create a seamless user experience. Here are some quick takeaways:
- Always understand the return type.
- Use the correct bean scope (preferably @ViewScoped).
- Bind parameters accurately and check for null or empty values.
- Implement validation rules.
- Use proper navigation rules.
- Implement global exception handling.
By following these best practices, you will ensure that your JSF applications function correctly and provide a better experience for users.
For more in-depth reading on JSF features, you can check out the official JSF documentation and the JSF 2.3 specification.
Feel free to share your own experiences and additional strategies in the comments below!