Navigating Java Concurrency: Common Pitfalls to Avoid
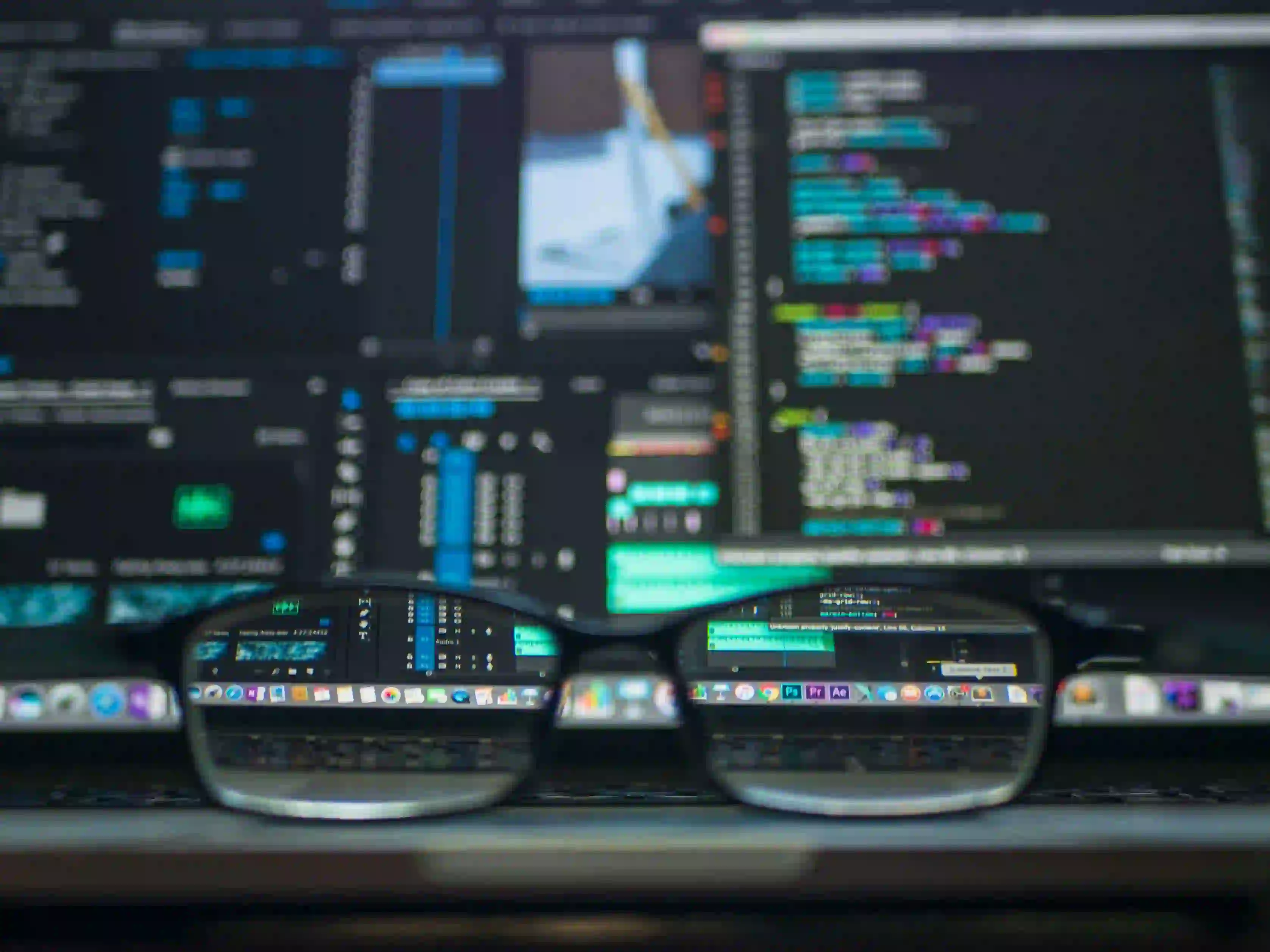
Navigating Java Concurrency: Common Pitfalls to Avoid
Java has long been praised for its powerful concurrency model, allowing developers to create efficient, multi-threaded applications. However, mastering concurrency is not merely about utilizing threads; it's about understanding the subtleties, complexities, and common pitfalls that come with it. In this blog post, we will unpack some of these common pitfalls in Java concurrency, providing you with the knowledge to avoid them and build more robust applications.
Understanding Concurrency
Concurrency in Java allows multiple threads to execute simultaneously, enhancing the performance and responsiveness of applications. Java provides a rich set of features for working with threads through classes like Thread
, Runnable
, and the java.util.concurrent
package.
Why Concurrency Matters
Using concurrency can significantly improve the performance of applications that perform time-consuming tasks, such as network operations or file I/O. However, managing multiple threads can introduce various challenges, including:
- Race Conditions: When two or more threads compete for shared resources, leading to inconsistent data states.
- Deadlocks: A situation where two or more threads are unable to proceed because they are waiting for each other to release resources.
- Thread Safety: Ensuring that shared data structures are manipulated in a way that does not lead to data corruption.
Common Pitfalls and How to Avoid Them
Understanding these common pitfalls will help you make informed decisions when designing and implementing your concurrent applications.
1. Ignoring Thread Safety
One of the biggest mistakes developers make is not ensuring thread safety when accessing shared resources. For example, consider the following code:
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
Why is This an Issue?
The increment
method is not synchronized. If multiple threads call increment
simultaneously, it can lead to incorrect results due to a race condition.
Solution: Use Synchronized Methods
You can resolve this issue by synchronizing the method:
public synchronized void increment() {
count++;
}
This ensures that only one thread can execute the increment()
method at a time, preventing race conditions.
2. Using Thread.run()
Instead of Thread.start()
Many developers mistakenly call the run()
method directly, expecting it to execute the thread. However, doing this does not create a new thread; it simply runs the method in the current thread.
public class MyRunnable implements Runnable {
public void run() {
System.out.println("Running in: " + Thread.currentThread().getName());
}
}
// Incorrect usage
MyRunnable runnable = new MyRunnable();
runnable.run(); // Executes in the current thread, not a new one.
// Correct usage
Thread thread = new Thread(runnable);
thread.start(); // Executes in a new thread.
Why is This an Issue?
Not using start()
means you miss out on the benefits of multithreading, and you may inadvertently create performance bottlenecks.
3. Deadlock Scenarios
Deadlocks occur when two or more threads wait indefinitely for each other to release resources. Consider the following example:
public class A {
synchronized void methodA(B b) {
b.last();
}
}
public class B {
synchronized void last() {}
}
If one thread holds a lock on an instance of A
and is waiting for B
, while another holds a lock on B
and is waiting for A
, you have a classic deadlock scenario.
How to Avoid Deadlocks
You can prevent deadlocks by:
- Lock Ordering: Always acquire locks in a consistent order.
- Timeouts: Implement lock timeouts so threads don’t wait indefinitely.
4. Misusing Volatile
The volatile
keyword in Java is often misunderstood. While it ensures visibility of changes to variables across threads, it does not guarantee atomicity.
public class VolatileExample {
private volatile boolean running = true;
public void stop() {
running = false; // Changes will be visible to other threads
}
public void doWork() {
while (running) {
// Work is done here
}
}
}
Why is This an Issue?
Although running
will be visible across threads, operations on other variables or related states may not be atomic or visible, leading to unexpected behaviors.
5. Blocking I/O Operations
Blocking I/O can be a major performance bottleneck. Traditional I/O operations can block the thread, stopping execution until the operation completes.
Use Asynchronous I/O
Consider using non-blocking I/O (NIO) or asynchronous processing methods to retain responsiveness.
import java.nio.channels.*;
import java.nio.*;
import java.io.*;
public class NonBlockingExample {
public void readData(ReadableByteChannel channel) throws IOException {
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (channel.read(buffer) > 0) {
buffer.flip();
// Process data
buffer.clear();
}
}
}
6. Overusing Synchronization
While it’s important to control access to shared resources, excessive synchronization can lead to performance issues, blocking threads unnecessarily.
Use Concurrent Utilities
Using classes from java.util.concurrent
, such as ConcurrentHashMap
, Lock
implementations, and other concurrent collections, can mitigate the need for excessive synchronization:
import java.util.concurrent.ConcurrentHashMap;
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key", 1); // Thread-safe put operation
Best Practices for Java Concurrency
- Use High-Level Concurrency Utilities: Whenever possible, leverage Java’s built-in concurrent utilities which handle many common concerns efficiently.
- Always Profile Your Application: Performance can vary widely between concurrent implementations. Profiling your application can help identify bottlenecks and inefficiencies.
- Understand the Java Memory Model: A clear understanding of how threads interact with memory can prevent many issues related to inconsistent data states.
Wrapping Up
Concurrency in Java can lead to significant performance enhancements, but it requires careful implementation to avoid common pitfalls. By understanding the complexities of thread management, employing correct synchronization mechanisms, and utilizing Java's concurrency utilities, you can create applications that are not only fast but also reliable.
For further reading, consider checking out the official Java documentation on Concurrency and explore more advanced topics, including Executor Services.
If you have any questions or want to share your experiences related to concurrency challenges in Java, feel free to leave a comment below. Happy coding!