Seamless AES Encryption Between JavaScript and Java
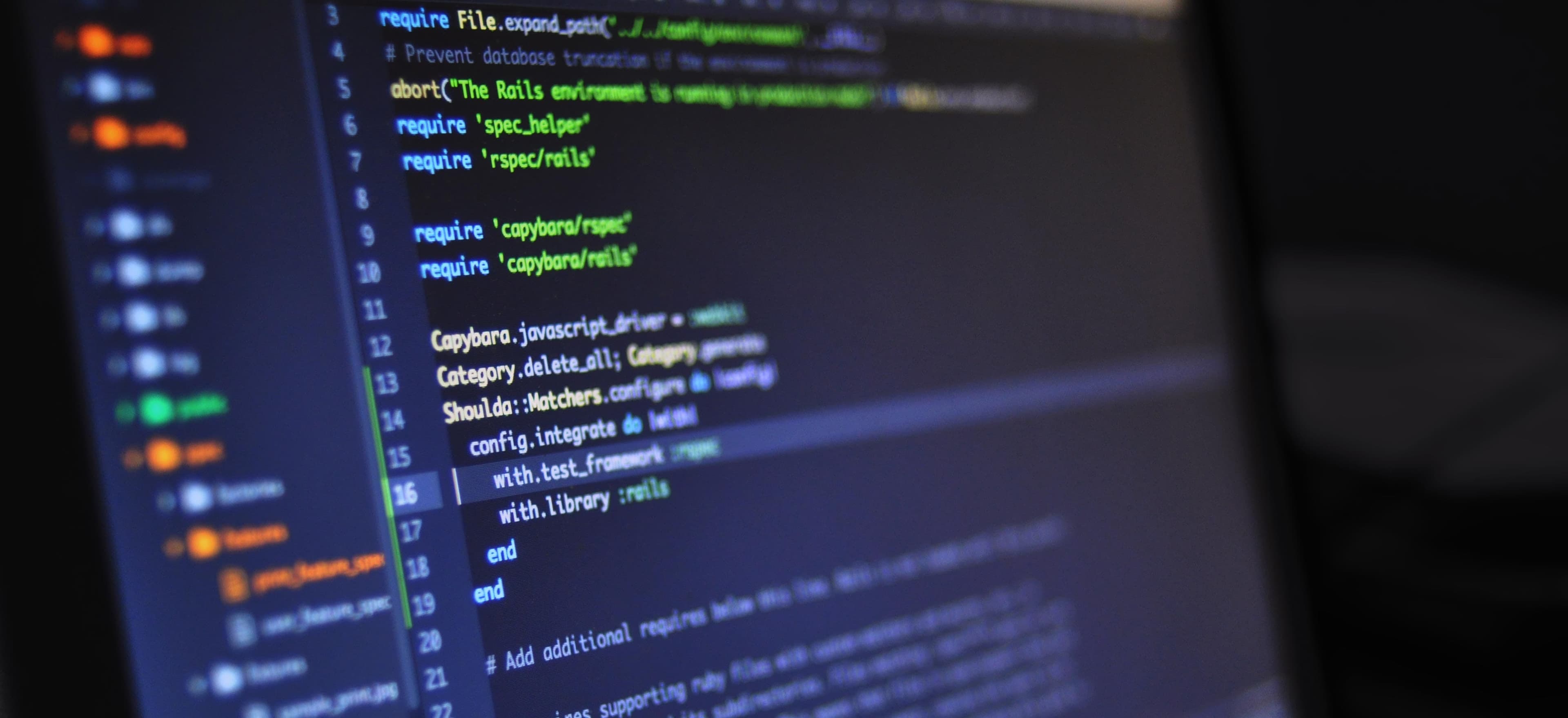
- Published on
Seamless AES Encryption Between JavaScript and Java
In today's tech-driven world, the need for secure data transmission is paramount. One robust method for ensuring confidentiality is Advanced Encryption Standard (AES). This post will guide you through the process of achieving seamless AES encryption between JavaScript and Java. By using synchronous code snippets and in-depth explanations, you'll learn how to encrypt and decrypt data effectively between the two languages.
Understanding AES Encryption
AES is a symmetric encryption algorithm, meaning it uses the same key for both encryption and decryption. It is widely used due to its speed and security. In this article, you will understand how to implement AES in both Java and JavaScript, ensuring that the two can communicate securely.
Prerequisites
Before proceeding, ensure you have:
- Java Development Kit (JDK) installed on your machine.
- A JavaScript runtime environment (Node.js or a browser) to test JavaScript code.
- Basic understanding of Java and JavaScript.
Setting Up the Java Side
Maven Dependencies
To leverage AES in Java, add the following dependency to your pom.xml
if you're using Maven:
<dependency>
<groupId>javax.crypto</groupId>
<artifactId>javax.crypto-api</artifactId>
<version>1.0.1</version>
</dependency>
AES Encryption and Decryption in Java
Here's how to create a Java class that encrypts and decrypts data using AES:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESEncryption {
private static final String ALGORITHM = "AES";
// Generates a new AES key
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance(ALGORITHM);
keyGen.init(128); // You can also use 192 or 256 bits
return keyGen.generateKey();
}
// Encrypts plain text
public static String encrypt(String data, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedBytes = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
// Decrypts encrypted text
public static String decrypt(String encryptedData, SecretKey key) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedData));
return new String(decryptedBytes);
}
public static void main(String[] args) throws Exception {
SecretKey secretKey = generateKey();
String originalData = "Hello AES Encryption!";
// Encrypt the data
String encryptedData = encrypt(originalData, secretKey);
System.out.println("Encrypted: " + encryptedData);
// Decrypt the data
String decryptedData = decrypt(encryptedData, secretKey);
System.out.println("Decrypted: " + decryptedData);
}
}
Code Commentary
-
SecretKey Generation: The
generateKey
method creates a new AES key. The key length can be adjusted to increase security. -
Encryption: In the
encrypt
method, we initialize the cipher in the encryption mode, process the data, and return the Base64-encoded string of the encrypted bytes. -
Decryption: The
decrypt
method reverses the process. It decodes the Base64 string first before decrypting it back to plain text.
By utilizing the Base64
library, we ensure that binary data transmitted is safe from corruption during transport.
Setting Up the JavaScript Side
On the JavaScript side, we can use the crypto-js
library to handle AES encryption and decryption. To install it via npm or include it in your HTML, execute:
npm install crypto-js
AES Encryption and Decryption in JavaScript
Here's a simple implementation using the crypto-js
library:
const CryptoJS = require("crypto-js");
function encrypt(data, key) {
// Encrypting data using AES
return CryptoJS.AES.encrypt(data, key).toString();
}
function decrypt(encryptedData, key) {
// Decrypting AES encrypted data
const bytes = CryptoJS.AES.decrypt(encryptedData, key);
return bytes.toString(CryptoJS.enc.Utf8);
}
const originalData = "Hello AES Encryption!";
const secretKey = "my-secret-key"; // Must match the key used in Java
const encryptedData = encrypt(originalData, secretKey);
console.log("Encrypted:", encryptedData);
const decryptedData = decrypt(encryptedData, secretKey);
console.log("Decrypted:", decryptedData);
Code Commentary
-
Encrypt Function: This method accepts plain text and a key, returning the encrypted text as a string.
-
Decrypt Function: It processes the encrypted string, converting it back to the original text.
-
Secret Key: Ensure that the key used in Java matches the one used in JavaScript. Different key lengths can lead to compatibility issues.
Testing Encryption Between Java and JavaScript
To successfully verify that your encryption is compatible between the two languages, consider the following:
- Use the same secret key, since AES is symmetric.
- Ensure the data format is consistent (Base64 encoding in Java).
- Test various data lengths and types to confirm robustness.
Example Interaction
-
Encrypt data in Java:
- The Java application runs, encrypting data such as "Hello AES Encryption!" with a generated key.
- This encrypted result is then forwarded to the JavaScript environment.
-
Decrypt in JavaScript:
- JavaScript code receives the Base64 string.
- Utilizes the exact same key for decryption.
Important Points to Consider
- Security Risks: Avoid hardcoding secret keys. Use environment variables or secure storage.
- Compatibility: Different library implementations may lead to variations in encryption output. Always test.
- Key Management: Using stronger keys (192-256 bits) increases security but may introduce complexity in key handling.
The Closing Argument
AES encryption seamlessly integrates between Java and JavaScript with relatively straightforward implementations. The key takeaway lies in coordinating the key and format across both platforms for effective security practices.
By understanding how to encrypt and decrypt data seamlessly in these two languages, you take a significant step toward building secure applications. These methodologies can be further expanded to include more layers of security, such as hashing and salted keys suitable for sensitive data storage.
For further reading, you may explore the Java Cryptography Architecture (JCA) for more information on cryptography in Java, and for JavaScript, check out the CryptoJS documentation.
By implementing the code snippets and instructions provided, you will be well-equipped to handle AES encryption efficiently within your applications. Let’s embrace secure coding practices as we advance into a more digitized future!
Checkout our other articles