Common Pitfalls in Startup Self-Testing for Continuous Delivery
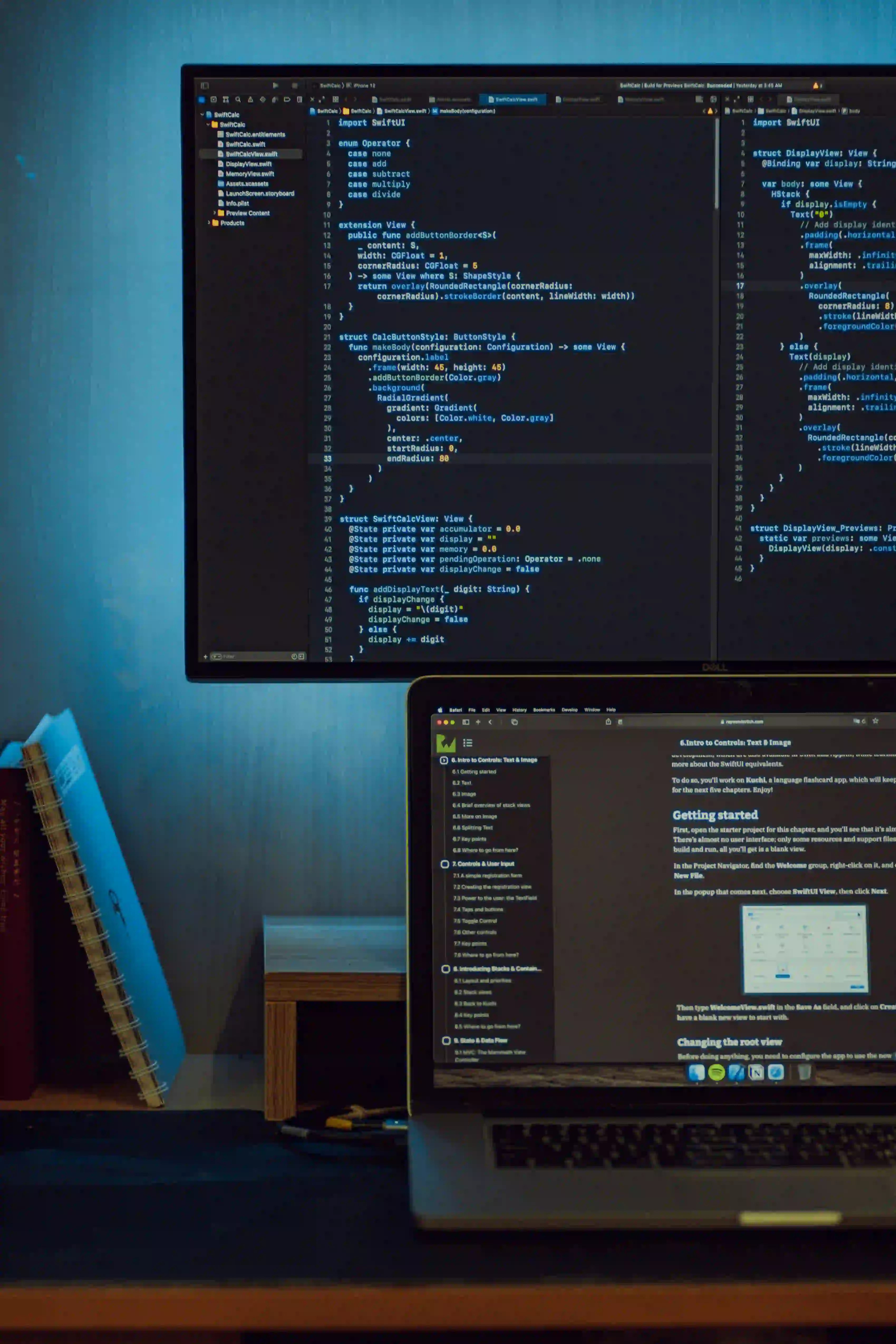
Common Pitfalls in Startup Self-Testing for Continuous Delivery
In the fast-paced world of startups, Continuous Delivery (CD) provides an avenue for rapid product iteration, allowing businesses to deploy new features and fixes quickly. However, how startups approach self-testing during this process can significantly impact their product quality and overall success. This blog post will delve into common pitfalls that startups encounter in self-testing and outline strategies to avoid them, ensuring a more robust Continuous Delivery pipeline.
Understanding Continuous Delivery
Before we dive into self-testing pitfalls, let’s clarify what Continuous Delivery entails. Continuous Delivery is an engineering practice where code changes are automatically prepared for a release to production. It involves:
- Automated Testing: Ensuring code quality through a suite of automated tests.
- Continuous Integration: Merging code changes into a shared repository frequently.
- Deployment Automation: Streamlining the process of deploying the code to various environments.
Continuous Delivery empowers startups to remain agile and responsive, but it does not eliminate the need for thorough testing.
Pitfall 1: Insufficient Test Coverage
One of the most frequent mistakes startups make is neglecting adequate test coverage. Many teams achieve initial success by focusing only on critical paths and high-impact features, overlooking the importance of comprehensive testing.
Why It Matters
Lack of thorough test coverage can lead to undetected bugs that might surface later in the development cycle or, worse, in production. This can erode user trust and lead to costly rollbacks.
Strategy to Avoid This Pitfall
-
Adopt a Coverage Metric: Start tracking test coverage using tools like JaCoCo or Cobertura. Aim for a minimum coverage percentage and gradually increase it.
☕snippet.java// Example: Calculating coverage with JaCoCo // Add JaCoCo plugin in your build.gradle file plugins { id 'jacoco' } jacoco { toolVersion = "0.8.5" } task jacocoTestReport(type: JacocoReport) { reports { xml.enabled true html.enabled true } // Specify source files and classes sourceDirectories.setFrom(files("src/main/java")) classDirectories.setFrom(files("build/classes/java/main")) executionData.setFrom(fileTree(dir: 'build', includes: ['**/*.exec'])) }
-
Ensure Comprehensive Testing: Include unit tests, integration tests, and end-to-end tests to validate all components and their interactions.
Pitfall 2: Ignoring Automated Testing
Automated testing is a cornerstone of successful Continuous Delivery. Startups often opt for manual testing due to perceived time constraints, believing that it is faster in the short term.
Why It Matters
Manual testing can quickly lead to inconsistencies, human errors, and oversight. Automated tests, on the other hand, are scalable and repeatable, enabling faster development cycles.
Strategy to Avoid This Pitfall
-
Invest in Testing Frameworks: Frameworks like JUnit and TestNG simplify writing and organizing tests.
☕snippet.javaimport org.junit.Test; import static org.junit.Assert.assertEquals; public class CalculatorTest { @Test public void testAddition() { Calculator calc = new Calculator(); assertEquals(5, calc.add(2, 3)); } }
-
Integrate Tests into CI/CD Pipelines: Ensure that all tests run automatically during the CI process. Tools like Jenkins or GitHub Actions can help you automate this.
⚙️snippet.yml# Example GitHub Actions workflow for testing name: CI on: push: branches: [ main ] jobs: test: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up JDK 11 uses: actions/setup-java@v1 with: java-version: '11' - name: Run tests run: ./gradlew test
Pitfall 3: Overlooking Non-Functional Requirements
While functional testing is essential, overlooking non-functional requirements like performance, security, and usability testing can have dire consequences. Startups often focus exclusively on functionality, ignoring these aspects.
Why It Matters
Non-functional issues may not be apparent through standard testing but can lead to performance bottlenecks, security vulnerabilities, and poor user experiences in production.
Strategy to Avoid This Pitfall
-
Incorporate Load Testing: Use tools like Apache JMeter or Gatling to simulate heavy loads and identify breaking points.
-
Security Testing: Integrate static and dynamic analysis tools like SonarQube or OWASP ZAP to detect vulnerabilities early.
Pitfall 4: Failing to Update Tests Along with Code Changes
As your application evolves, keeping tests updated is crucial. Startups often treat tests as secondary, leading to obsolete tests that are no longer relevant.
Why It Matters
Outdated tests can provide false confidence, leading to untested code paths and increasing the risk of bugs making their way to production.
Strategy to Avoid This Pitfall
-
Establish Test Review Process: Include test cases as part of your code review process. Reviewers should evaluate not just code changes, but also the corresponding tests.
-
Leverage Test-Driven Development (TDD): Encourage writing tests before the actual code. This practice will ensure that changes in the code are consistently reflected in tests.
Pitfall 5: Ignoring Feedback from Test Results
Many teams get caught up in testing and fail to genuinely analyze and act on test results. This reactive approach can stifle growth and improvement.
Why It Matters
Ignoring feedback leads to repeated mistakes and hinders awareness about potential issues in the test cases or the code itself.
Strategy to Avoid This Pitfall
-
Regularly Review Test Results: Schedule time for analyzing test results with your team to identify patterns and recurring issues.
-
Implement Continuous Monitoring: Utilize application performance monitoring (APM) tools such as New Relic or Prometheus to continuously gather feedback on system performance and stability.
A Final Look
Avoiding self-testing pitfalls is crucial for startups aiming to maintain a seamless Continuous Delivery process. By ensuring adequate test coverage, leveraging automated testing, addressing non-functional requirements, keeping tests updated, and analyzing feedback, your startup can confidently push new features and updates without compromising on quality.
To explore more about testing and automated processes, check out Martin Fowler's thoughts on Continuous Delivery and test-driven development. These resources can provide additional insights into improving your development practices.
Remember, the goal of Continuous Delivery is not just to ship code quickly, but to do so reliably and consistently. Your testing strategy plays a pivotal role in achieving that objective. Engage your team, embrace automation, and turn testing from a chore into a cornerstone of your development process.