Overcoming Common Missteps in Prototype Design Patterns
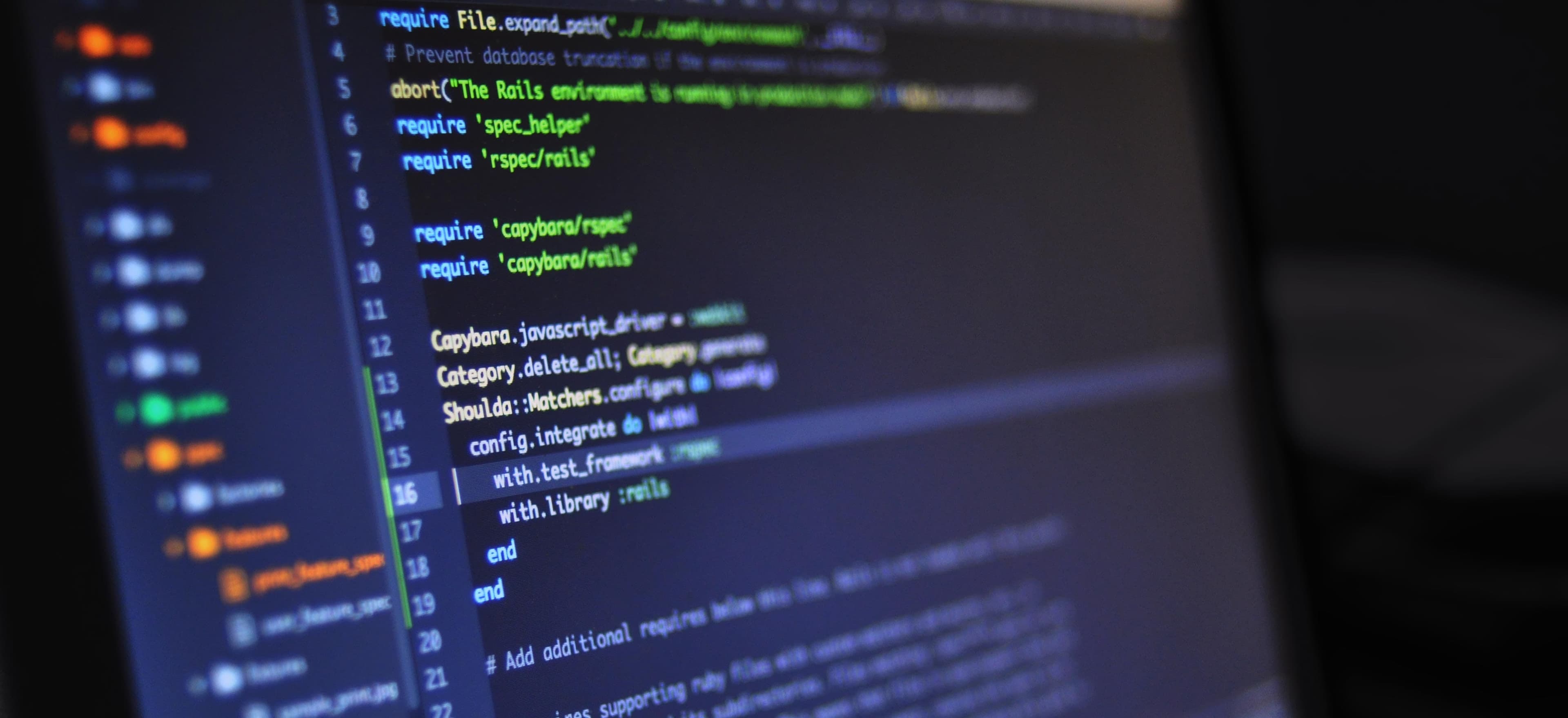
- Published on
Overcoming Common Missteps in Prototype Design Patterns
The Prototype design pattern is a creational pattern that enables object creation based on a template of an existing object. By cloning an instance of a class, you can produce new objects without the overhead of creating them from scratch. However, missteps often occur, leading to inefficient or error-prone designs. In this blog post, we’ll uncover common pitfalls when implementing the Prototype design pattern and offer strategies to overcome them.
Table of Contents
- Understanding the Prototype Pattern
- Common Missteps in Prototype Design Patterns
- Misstep 1: Improper Cloning
- Misstep 2: Forgetting to Implement
Cloneable
- Misstep 3: Ignoring Deep vs. Shallow Copies
- Misstep 4: Overusing Prototypes
- Best Practices for Implementing Prototype
- Conclusion
- References
Understanding the Prototype Pattern
Before we delve into the missteps, it's essential to understand the Prototype design pattern. This pattern is especially useful when:
- Object creation is costly.
- You have numerous similar objects.
- You aim to minimize dependencies between classes.
The central concept is that a class specifies the kinds of objects to create using a prototypical instance, which will be cloned to produce new objects.
Here’s a simple Java implementation of the Prototype pattern:
// Prototype interface
public interface Prototype {
Prototype clone();
}
// Concrete prototype class
public class ConcretePrototype implements Prototype {
private String name;
public ConcretePrototype(String name) {
this.name = name;
}
@Override
public Prototype clone() {
return new ConcretePrototype(this.name);
}
public String getName() {
return name;
}
}
In this implementation, ConcretePrototype
implements the Prototype
interface and defines the clone
method. By calling clone
, you create a new instance that shares the same name
property.
Common Missteps in Prototype Design Patterns
Misstep 1: Improper Cloning
Not all clone implementations are equal. One common mistake is failing to copy all relevant fields or object states.
Solution: Ensure you clone all necessary data fields. Review the object state, and consider all attributes when implementing the clone
method.
@Override
public Prototype clone() {
// Avoiding improper cloning
ConcretePrototype clone = new ConcretePrototype(this.name);
// Clone deep or shallow as needed based on object's properties
return clone;
}
Misstep 2: Forgetting to Implement Cloneable
Many developers assume that the Prototype pattern automatically handles cloning. However, you must ensure that the class implements the Cloneable
interface.
Solution: Always declare your class to be Cloneable
. Not implementing it can lead to runtime exceptions.
public class ConcretePrototype implements Prototype, Cloneable {
// Implementation...
}
Misstep 3: Ignoring Deep vs. Shallow Copies
A common oversight is not distinguishing between deep and shallow copies. A shallow copy duplicates the immediate fields of an object but does not copy the objects referenced by those fields. Contrastingly, a deep copy duplicates everything recursively.
Solution: Determine the needs of your application. If your object contains mutable references, utilize deep copying.
@Override
public Prototype clone() {
ConcretePrototype clone = new ConcretePrototype(this.name);
// Assume we need a deep copy of mutable fields
clone.mutableField = this.mutableField.clone(); // Example of deep copying
return clone;
}
Misstep 4: Overusing Prototypes
Some developers become overly reliant on the Prototype pattern. While it’s beneficial in many cases, using it indiscriminately can introduce complexity.
Solution: Use prototypes when you truly need to duplicate instances. Evaluate whether the burden of managing cloned objects outweighs the benefits.
Best Practices for Implementing Prototype
To ensure the effective use of the Prototype Design Pattern, consider these best practices:
-
Keep Cloning Logic Simple: Your clone method should be easy to understand, avoiding unnecessary complexity.
-
Prototype Management: Provide a central location for managing prototypes, making it easy to create specific instances.
-
Testing: Always test cloned objects extensively. Ensure they behave as expected when modified independently.
-
Documentation and Comments: Always document your methods, especially
clone
, to explain the cloning strategy used. -
Consider Alternatives: Be open to alternatives like the Factory pattern when the Prototype pattern doesn't fit.
In Conclusion, Here is What Matters
The Prototype design pattern offers unique advantages for object instantiation, particularly when object creation is costly. However, common missteps can undermine its effectiveness. By staying mindful of cloning correctly, implementing Cloneable
, distinguishing between deep and shallow copies, and judiciously applying the pattern, you'll position yourself to harness its true potential.
In an evolving software landscape, continuous learning is key. Engage with various design patterns like Singleton and Factory to enrich your understanding and application of best practices in software design.
For more insights into design patterns and effective programming practices, explore resources such as Effective Java or Design Patterns: Elements of Reusable Object-Oriented Software.
Happy coding!
Checkout our other articles