Why Underestimating Complexity Leads to Project Failure
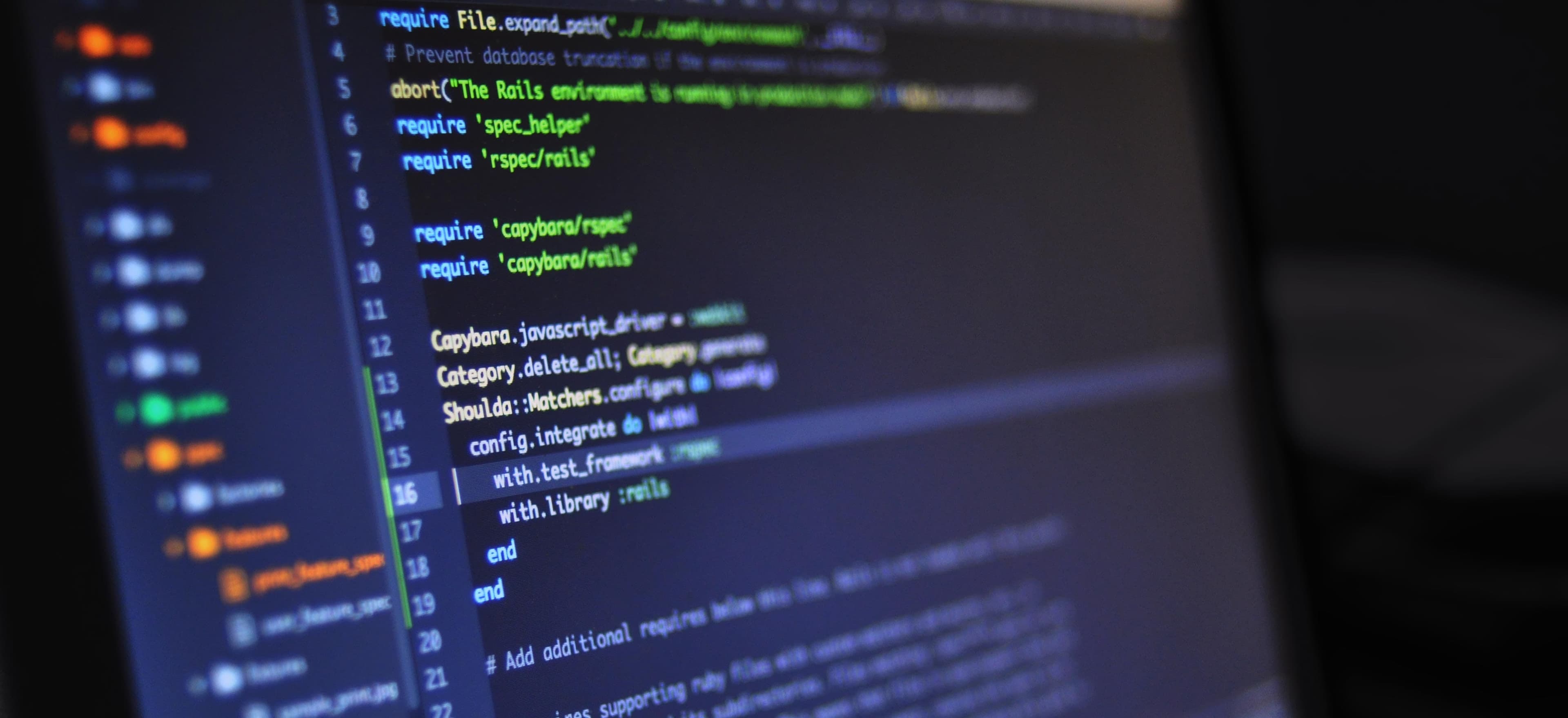
- Published on
Why Underestimating Complexity Leads to Project Failure
In today's fast-paced technological landscape, software development projects often encounter unforeseen challenges. Among them, underestimating complexity stands out as a critical yet frequently overlooked factor in project failures. Understanding the reasons behind this issue is vital for enhancing project management practices, improving team collaboration, and ultimately delivering successful outcomes. This blog post will delve into the intricacies of complexity and its potential pitfalls, especially in Java development.
What is Complexity in Software Projects?
Complexity in software development refers to the challenges that arise from the intricate relationships between components, dependencies, and user requirements. It's not limited to just the codebase; complexity can stem from various sources, including:
- Technical Complexity: How the code is structured and interacts with different components.
- Domain Complexity: The nature of the problem being solved, which might require deep domain knowledge.
- Interpersonal Complexity: The dynamics among team members and their collaboration.
Ignoring the multifaceted nature of complexity can lead to significant pitfalls in project planning and execution.
The Dangers of Underestimating Complexity
Underestimating complexity can cause several issues, including:
- Mismanaged Expectations: Stakeholders may have unrealistic expectations for timelines and deliverables.
- Bottlenecks in Development: Developers may face unexpected roadblocks that impede progress.
- Quality Assurance Issues: A rushed quality assurance process can lead to defects in the product.
- Increased Costs and Resources: Predicting costs and resource allocation becomes challenging when complexity is not accurately assessed.
Example: Code Structure and Dependencies
Consider a Java project where the architecture is not sufficiently designed to handle the expected growth in features. Here’s a simplified code snippet demonstrating a poorly structured dependency:
public class UserService {
private EmailService emailService;
public UserService() {
this.emailService = new EmailService(); // Tight coupling
}
public void registerUser(String email) {
// Registration logic
emailService.sendConfirmation(email); // Direct dependency
}
}
class EmailService {
public void sendConfirmation(String email) {
// Logic to send email
}
}
Why is This a Problem?
The tight coupling between UserService
and EmailService
creates issues if you want to change how emails are sent or mock the service for unit tests.
Improving the Design
By using dependency injection, you increase flexibility and reduce tight coupling. Here’s a revised version:
public class UserService {
private EmailService emailService;
// Dependency injection via constructor
public UserService(EmailService emailService) {
this.emailService = emailService;
}
public void registerUser(String email) {
// Registration logic
emailService.sendConfirmation(email);
}
}
// Now you can inject any implementation of EmailService
Why is This Design Better?
- Flexibility: You can easily swap out
EmailService
with a different implementation. - Testability: It is easier to mock
EmailService
when writing unit tests. - Separation of Concerns: Each class has its own responsibility, making the code easier to understand and maintain.
How to Manage and Mitigate Complexity
To manage complexity effectively and prevent project failure, consider the following strategies:
1. Thorough Planning and Requirement Gathering
Identify the critical requirements and potential challenges early in the project lifecycle. Include all stakeholders in the discussion to form a comprehensive understanding of the project's complexities.
2. Incremental Development and Agile Methodologies
Adopt agile methodologies that emphasize incremental development cycles. This allows you to adapt and respond to challenges as they arise, rather than waiting until the end of the project lifecycle to address them.
3. Regular Code Reviews
Encourage regular code reviews among team members. A second set of eyes can identify issues related to complexity that one individual may overlook, fostering better understanding and collaboration among team members.
4. Training and Skill Development
Invest in training for your team to improve their domain knowledge and technical skills. A well-informed team can better anticipate complexities and devise effective strategies to manage them.
5. Utilizing Tools and Frameworks
Leverage modern Java frameworks and tools (e.g., Spring, Hibernate) that promote best practices in code structure and reduce the inherent complexity of development. For more insights, check out Spring Framework Documentation and how it can facilitate better project management.
Case Studies: Real-World Impact of Underestimating Complexity
Several high-profile software project failures demonstrate the repercussions of underestimating complexity.
Example: Healthcare.gov Launch
The initial launch of Healthcare.gov in 2013 was plagued with technical issues. One of the main reasons for its failure was the underestimation of the complexities involved in creating a platform that integrated multiple federal agencies and provided a seamless user experience. The challenges experienced resulted in significant delays and cost overruns.
Example: The Boeing 737 Max
While not a software project in the traditional sense, the Boeing 737 Max crisis highlighted issues stemming from complexity underestimations. The software meant to control the plane’s flight was not properly integrated and tested alongside hardware systems, leading to fatal consequences. This situation serves as a reminder of the real-world implications that can arise from poor complexity assessment.
The Closing Argument
Underestimating complexity can have dire consequences in software development projects, particularly in Java programming. It’s essential to recognize the various forms of complexity—technical, domain, and interpersonal—and address them through meticulous planning, incremental development, and ongoing training.
By adopting best practices that encourage flexibility and adaptability, you can better navigate the labyrinth of software complexity. This approach not only enhances project outcomes but also fosters a culture of continuous learning and improvement.
For further reading on managing complexity in projects, consider resources such as the Agile Alliance or software project management literature. As we advance in our technological capabilities, understanding and mitigating complexity is a fundamental skill for successful project management in any domain.
By fostering awareness and implementing effective strategies, you can significantly reduce the likelihood of project failure due to underestimated complexity.