Top 5 Java 7 Features You Might Not Be Using
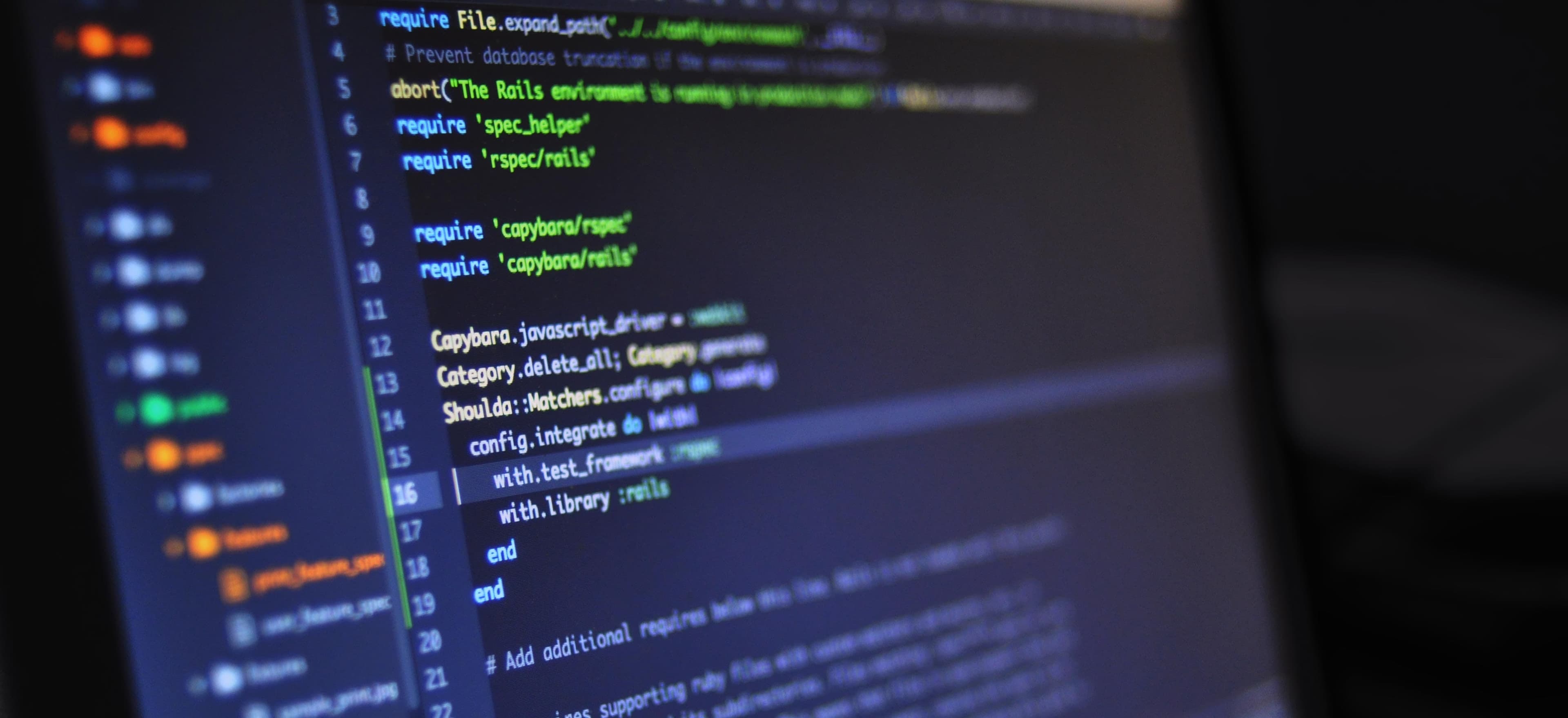
- Published on
Top 5 Java 7 Features You Might Not Be Using
Java 7, officially released in July 2011, introduced several features aimed at improving productivity, maintainability, and performance. Although many developers are aware of Java 7's major enhancements, several powerful features often remain underutilized. In this blog post, we will delve into the top five features of Java 7 that you might not be using but could significantly enhance your coding experience.
1. The Diamond Operator
What is it?
The diamond operator, < >
, simplifies the instantiation of generic classes. Prior to Java 7, developers were required to specify the generic type when declaring and instantiating a new object, leading to verbose code.
Example:
List<String> list = new ArrayList<String>();
Why Use It?
In Java 7, the diamond operator allows you to omit the type during instantiation, leading to cleaner and more readable code.
Revised Example:
List<String> list = new ArrayList<>();
By using the diamond operator, the compiler infers the type from the variable declaration, making your code less cluttered and easier to understand.
2. Try-with-Resources Statement
What is it?
The try-with-resources statement simplifies the management of resources such as files or sockets. Resources that implement the AutoCloseable
interface can be automatically closed after use.
Example:
Here is the traditional way of handling a BufferedReader
:
BufferedReader br = new BufferedReader(new FileReader("file.txt"));
try {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} finally {
br.close();
}
Why Use It?
With Java 7, you can utilize try-with-resources to enhance maintainability:
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} // br automatically closed here
This pattern eliminates the boilerplate code for resource management, drastically reducing the potential for resource leaks.
3. String in switch Statement
What is it?
Prior to Java 7, switch statements only supported primitive data types and enumerated types. With Java 7, you can now use Strings, which can simplify your logic and improve readability.
Example:
Consider the standard approach to branching based on string values with if-else statements:
String animal = "Dog";
if (animal.equals("Dog")) {
System.out.println("It's a dog.");
} else if (animal.equals("Cat")) {
System.out.println("It's a cat.");
} else {
System.out.println("Unknown animal.");
}
Why Use It?
Switch statements provide improved readability and performance in some cases:
String animal = "Dog";
switch (animal) {
case "Dog":
System.out.println("It's a dog.");
break;
case "Cat":
System.out.println("It's a cat.");
break;
default:
System.out.println("Unknown animal.");
}
Using a switch statement allows you to more easily visually parse your code, especially when dealing with multiple conditions.
4. New File I/O (NIO.2) APIs
What is it?
Java 7 introduced a new NIO.2 API to improve the way developers handle file I/O operations. This API provides a more comprehensive interface for file and directory manipulation, making it easier to work with files.
Example:
Using the classic File
methods:
File file = new File("example.txt");
if (!file.exists()) {
file.createNewFile();
}
Why Use It?
With NIO.2, file management becomes a lot more contained and readable:
import java.nio.file.*;
Path path = Paths.get("example.txt");
if (Files.notExists(path)) {
Files.createFile(path);
}
The new API also comes with several other methods to handle file attributes, directory walking, and file copying. You can learn more about it in the Java NIO.2 documentation.
5. Type Inference for Generic Instance Creation
What is it?
Type inference for generic instance creation allows you to avoid repeating type parameters, simplifying code that uses generics.
Example:
Here's a less concise way of declaring a list:
Map<String, List<String>> map = new HashMap<String, List<String>>();
Why Use It?
In Java 7, you can declare it more clearly:
Map<String, List<String>> map = new HashMap<>();
This not only saves time but also makes your code easier to read and maintain.
Lessons Learned
Java 7 brought significant improvements to the Java programming language. While many developers have adopted the basics from the release, the above features often remain underused despite their ability to enhance code quality. By integrating the diamond operator, try-with-resources statements, string switch cases, the NIO.2 API, and type inference into your everyday coding practices, you can create cleaner, more efficient, and maintainable Java code.
For more in-depth features and techniques related to Java development, you might find the following resources helpful:
- Official Java Documentation
- Oracle's Java Tutorials
- Java SE 7 Features Overview
Embrace these powerful enhancements to refine your skills and build better applications with Java. Happy coding!
Checkout our other articles