Understanding String Memory Management in Programming
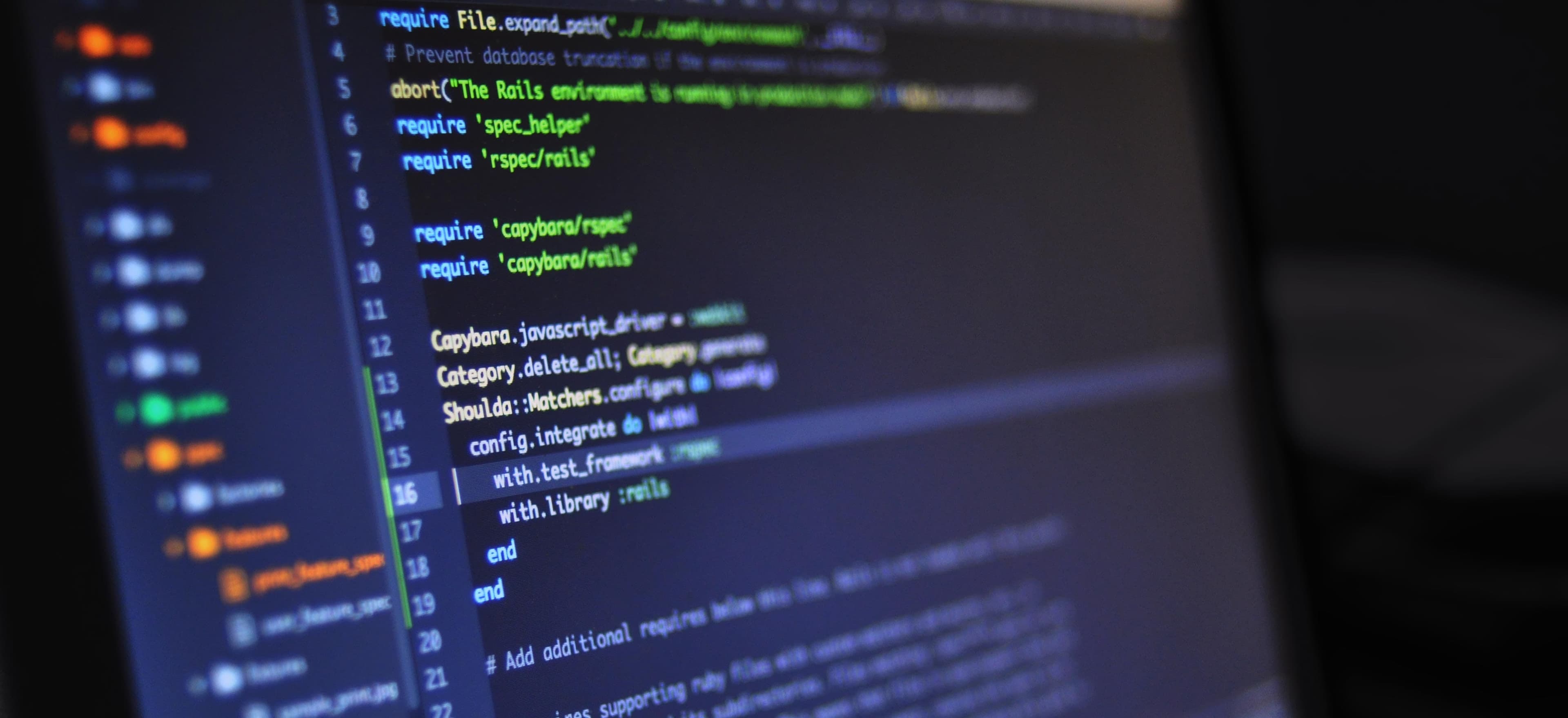
- Published on
Understanding String Memory Management in Programming
In programming, effective string handling and memory management are critical components that determine the performance and reliability of applications. As a widely-used programming language, Java offers robust tools for managing strings, yet there are nuances to be aware of, especially regarding memory allocation, immutability, and performance considerations. In this blog post, we will delve into the intricacies of string memory management in Java, explore the differences between string types, and offer practical code examples to illustrate these concepts.
What is a String in Java?
In Java, a string is a sequence of characters. It is represented by the String
class that provides various methods to manipulate the sequence. Strings in Java are immutable, meaning that once a String
object is created, it cannot be changed. Despite this immutability, new string instances can be generated based on existing strings.
The Immutability of Strings
The immutability characteristic ensures that strings are thread-safe and can be safely shared between multiple threads without jumping into synchronization issues. This design choice can lead to efficient memory management within the Java Virtual Machine (JVM).
Here's a simple example demonstrating string immutability:
public class StringImmutability {
public static void main(String[] args) {
String original = "Hello, World!";
String modified = original.replace("World", "Java");
System.out.println("Original String: " + original); // Outputs "Hello, World!"
System.out.println("Modified String: " + modified); // Outputs "Hello, Java!"
}
}
In this code snippet, the original string remains unchanged after the replace operation. Instead, a new string instance is created. This highlights a fundamental aspect of Java strings: they do not modify the original instance, ensuring stability and safety in string operations.
String Pool and Memory Management
Java utilizes a special pool known as the String Pool to optimize memory use. When a string literal is created, Java checks the string pool:
- If the string already exists, it reuses the existing string reference.
- If not, it adds the new string to the pool.
This can be illustrated with the following code snippet:
public class StringPoolExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Hello"; // Reuses the reference from String Pool
System.out.println(str1 == str2); // Outputs true
String str3 = new String("Hello"); // Creates a new memory allocation
System.out.println(str1 == str3); // Outputs false
}
}
Explanation of the Code
In this example:
str1
andstr2
point to the same memory reference in the string pool.str3
, however, is a distinct object in the heap memory due to thenew
keyword.
Understanding this behavior is crucial for optimizing memory management and performance in Java applications.
Differences Between String, StringBuilder, and StringBuffer
While String
is immutable, StringBuilder
and StringBuffer
provide mutable alternatives. They allow modification of strings without creating new instances, which can enhance performance when performing multiple string operations.
StringBuilder
StringBuilder
is faster than StringBuffer
because it is not synchronized, making it suitable for single-threaded scenarios:
public class StringBuilderExample {
public static void main(String[] args) {
StringBuilder stringBuilder = new StringBuilder("Hello");
stringBuilder.append(", World!");
System.out.println(stringBuilder.toString()); // Outputs "Hello, World!"
}
}
StringBuffer
On the other hand, StringBuffer
is synchronized and thread-safe, making it the better option when multiple threads access a string concurrently:
public class StringBufferExample {
public static void main(String[] args) {
StringBuffer stringBuffer = new StringBuffer("Hello");
stringBuffer.append(", World!");
System.out.println(stringBuffer.toString()); // Outputs "Hello, World!"
}
}
When to Use Each Type
- Use
String
for constant strings or when you do not require many modifications. - Use
StringBuilder
for single-threaded environments where performance is a priority. - Use
StringBuffer
when thread safety is essential.
Memory Considerations and Garbage Collection
In terms of memory management, Java automatically handles memory through Garbager Collection (GC). Unused string objects become eligible for garbage collection, which allows the JVM to reclaim memory. However, understanding how objects enter into memory can help you write more efficient code.
For example, avoid unnecessary string concatenations in a loop:
public class InefficientStringConcatenation {
public static void main(String[] args) {
String result = "";
for (int i = 0; i < 1000; i++) {
result += i; // Creates new String objects repeatedly
}
System.out.println(result);
}
}
In the example above, frequent concatenation leads to excessive memory allocation. Instead, prefer using StringBuilder
:
public class EfficientStringConcatenation {
public static void main(String[] args) {
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append(i); // Modifies existing memory
}
System.out.println(result.toString());
}
}
The Last Word
Understanding string memory management in Java is essential for optimizing performance and preventing memory leaks. The immutability of the String
class offers safety and efficiency in multi-threaded contexts, while StringBuilder
and StringBuffer
cater to different threading needs. Understanding string pooling and effective use of garbage collection can further enhance your application's efficiency.
For more in-depth information on Java memory management, consider visiting the Oracle Java Documentation and Java Performance Tuning.
By applying these concepts thoughtfully, you can improve both the reliability and performance of your Java applications. Keep exploring and optimizing your code, and you'll find that memory management can both simplify and enhance your programming experience.
Checkout our other articles