Why Your Domain Logic Needs Isolation in Software Design
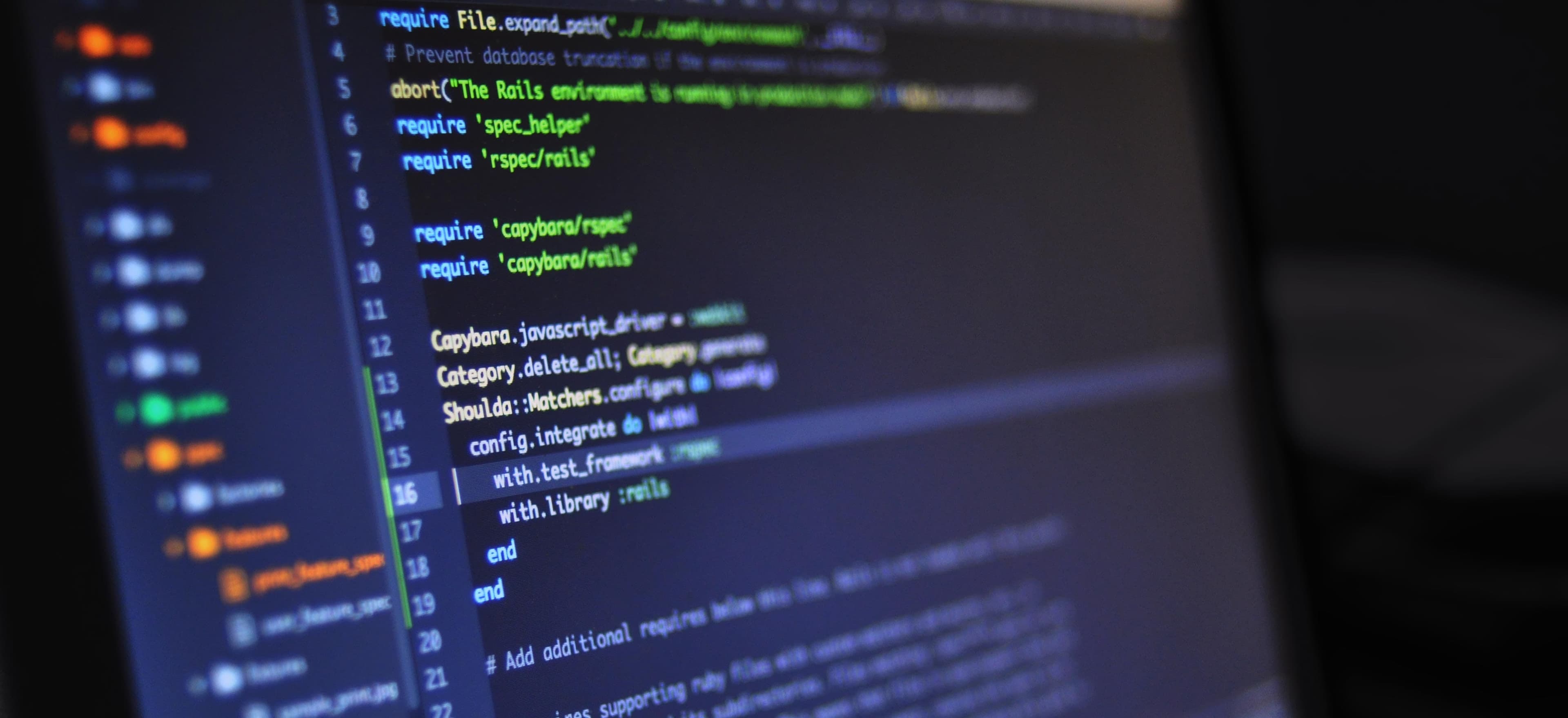
- Published on
Why Your Domain Logic Needs Isolation in Software Design
In software design, particularly in complex systems, your domain logic plays a critical role. It encapsulates the business rules and core functionalities that define what your application does. However, how you organize this logic significantly impacts maintainability, scalability, and testability. One key principle in achieving these objectives is the isolation of your domain logic. In this blog post, we will explore why isolating your domain logic is essential and how to effectively implement it.
Understanding Domain Logic
Before diving into the reasons for isolation, let’s clarify what we mean by domain logic. Domain logic includes the rules, calculations, and workflows that define how your application operates. It answers questions like:
- What happens when an order is placed?
- How are customer discounts calculated?
- What are the criteria for user authentication?
Isolating your domain logic means separating it from other aspects of your application, such as UI and infrastructure concerns. This practice leads to several benefits.
Benefits of Isolation
1. Improved Testability
When domain logic is isolated, it becomes easier to write unit tests. You can create tests specifically for the business rules without worrying about UI or data access complexities. This separation leads to faster feedback loops during development.
Code Example:
Here’s a simple Java example demonstrating isolated domain logic:
public class Order {
private double totalPrice;
private List<Item> items;
public void addItem(Item item) {
items.add(item);
calculateTotalPrice();
}
private void calculateTotalPrice() {
totalPrice = items.stream()
.mapToDouble(Item::getPrice)
.sum();
}
public double getTotalPrice() {
return totalPrice;
}
}
Commentary:
In this code snippet, the Order
class encapsulates the domain logic related to order processing. By keeping this logic within the Order
class, we provide a specific, isolated point for testing, independent of UI or external services.
2. Enhanced Maintainability
When domain logic is mixed with other code, making changes becomes cumbersome. Isolation means that changes needed in business rules are localized and do not inadvertently affect the rest of your application.
Example Scenario:
Suppose you need to change the way discounts are calculated in your e-commerce platform. If discount logic is tangled with other parts of your application, you will need to test all dependencies. However, if it’s isolated, you can simply modify the discount logic without worrying about unrelated code.
3. Clearer Architecture
Isolating domain logic fosters a cleaner architecture. It encourages following best practices such as Domain-Driven Design (DDD), which emphasizes defining boundaries for your business logic and entities.
Key Concept: Creating bounded contexts helps you break down complex domains into manageable pieces.
4. Increased Reusability
When designed properly, isolated domain logic can be reused across different parts of an application or even in different applications. This is particularly beneficial in microservices architectures where specific services handle distinct business domains.
Strategies for Isolating Domain Logic
Now that we understand the benefits, let’s look at some effective strategies to isolate domain logic.
1. Use Layers in Your Architecture
Implement a layered architecture, often referred to as the n-tier architecture. The common layers include:
- Presentation Layer: Handles user interactions and UI.
- Application Layer: Coordinates the flow between UI and domain logic.
- Domain Layer: Contains business logic and domain entities.
- Infrastructure Layer: Manages data access and external services.
Visual Representation:
+---------------------+
| Presentation |
| Layer |
+---------------------+
| Application |
| Layer |
+---------------------+
| Domain |
| Layer |
+---------------------+
| Infrastructure |
| Layer |
+---------------------+
This separation ensures that your domain logic remains independent of how the data is presented to users or retrieved from databases.
2. Employ Domain Models
Incorporate Domain Models that represent the core concepts of your business. These are rich objects that include both data and behavior relevant to the domain.
Code Example:
public class Customer {
private String name;
private List<Order> orders;
public void placeOrder(Order order) {
orders.add(order);
// Additional business rules can be applied here.
}
// other methods...
}
Commentary:
In this Customer
class, we encapsulate actions related to customer behavior. This keeps our domain logic contained and coherent, making it easier to manage.
3. Dependency Injection
Utilize Dependency Injection (DI) to manage dependencies. DI allows you to inject the required services and components into your domain classes instead of hardcoding them.
Example with Spring Framework:
@Service
public class OrderService {
private final OrderRepository orderRepository;
@Autowired
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
public void processOrder(Order order) {
// Domain logic for processing the order
orderRepository.save(order);
}
}
Commentary:
With DI, the OrderService
is focused on business logic while relying on OrderRepository
for data access. This keeps your domain logic clean and more easily testable.
To Wrap Things Up
Isolating domain logic in software design is not merely a good practice; it's an essential strategy for creating maintainable, testable, and scalable applications. The separation of concerns ensures clarity within your architecture, encourages reuse, and ultimately leads to more robust code.
As you continue to evolve your applications, prioritizing the isolation of your domain logic will pay dividends in the long run. For further reading, consider exploring Domain-Driven Design or reviewing principles of Clean Architecture.
Embrace the practice of keeping your domain logic isolated, and watch your application development process become more efficient, yielding high-quality software solutions tailored for success.
Checkout our other articles