Enhancing Java Web Apps: Clickable DataTable Rows Explained
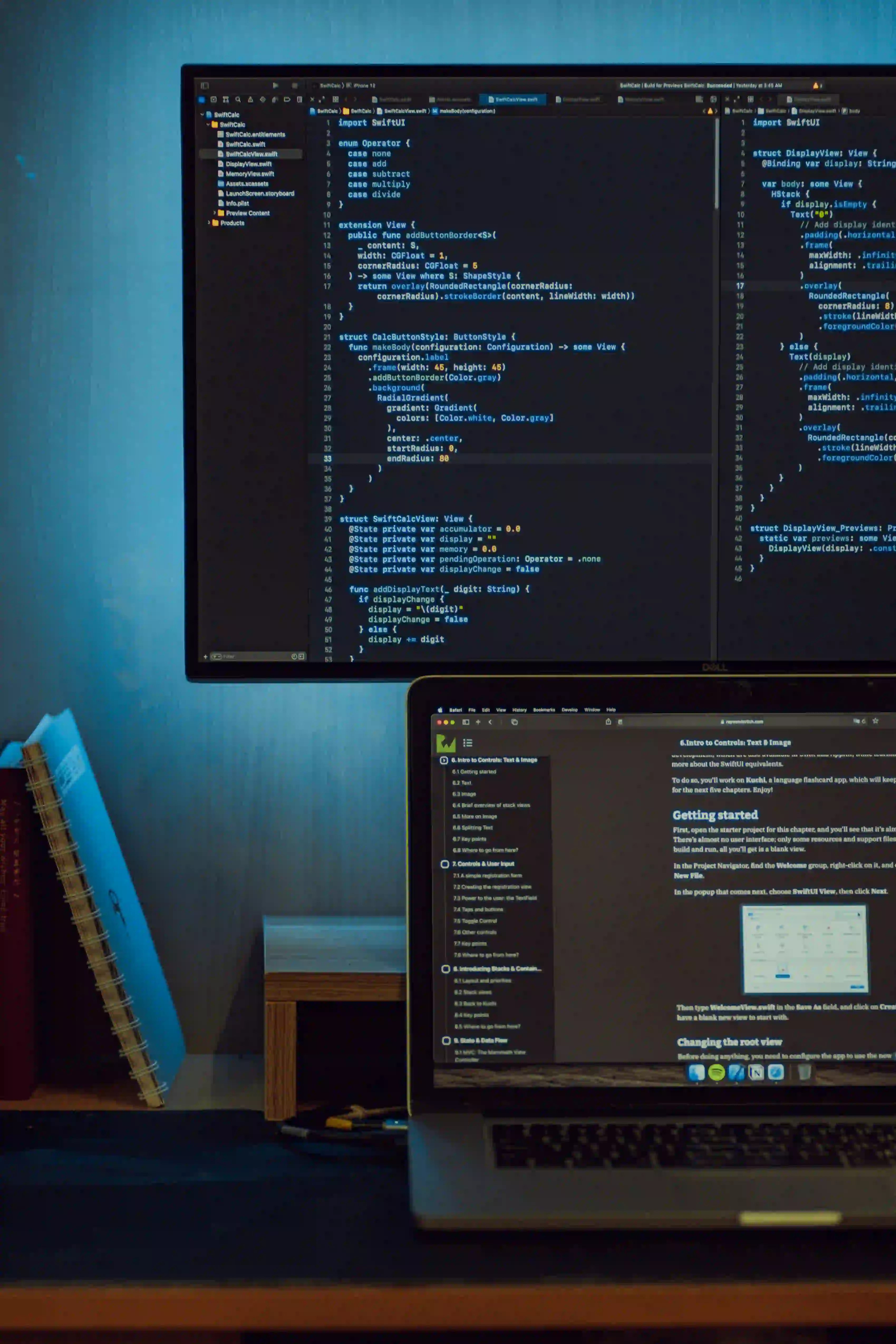
Enhancing Java Web Apps: Clickable DataTable Rows Explained
In the world of modern web applications, user experience (UX) is more than just a buzzword. It's fundamental to creating applications that people enjoy using. As web developers, we constantly seek ways to make our applications easier and more intuitive for users. One effective technique? Making DataTable rows clickable. In this blog post, we'll delve into how to implement this functionality in Java web applications, enhancing your application's interactivity and overall UX.
Understanding the Importance of Clickable Rows
Clickable rows in a DataTable allow users to interact with the data presented easily. This interaction can lead to a variety of actions:
- Viewing Detailed Information: Clicking a row can redirect users to a detailed view of the data.
- Editing Data: Enable users to modify entries seamlessly.
- Additional Actions: Trigger various functions like delete, share, etc.
By incorporating clickable rows, you streamline the user experience and make it more engaging. This concept closely relates to the article Boost UX: Make ASP.NET DataGrid Rows Clickable!, which discusses similar enhancements in ASP.NET. Although the technology varies, the core principle remains: improving UX through interactivity.
Setting Up Your Development Environment
Before we dive into code, ensure you have the following set up:
- A Java EE web server (like Apache Tomcat or Jetty).
- An integrated development environment (IDE) such as IntelliJ IDEA or Eclipse.
- Dependency management tools like Maven or Gradle.
Now, let's get started with our DataTable implementation.
Building the Web Application
Step 1: Include Necessary Dependencies
For this demonstration, we will utilize Servlets and JSP (JavaServer Pages). Make sure to include at least the following dependencies in your pom.xml
if you are using Maven:
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>javax.servlet.jsp-api</artifactId>
<version>2.3.3</version>
<scope>provided</scope>
</dependency>
</dependencies>
Step 2: Create a Simple Data Model
We'll create a simple model to represent our data. Letβs define a User
class that contains basic user information.
public class User {
private int id;
private String name;
private String email;
// Constructor
public User(int id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
// Getters
public int getId() { return id; }
public String getName() { return name; }
public String getEmail() { return email; }
}
Step 3: Building the Data Access Layer
Next, weβll create a simple service that mimics data retrieval. In a real-world scenario, this would connect to a database.
import java.util.ArrayList;
import java.util.List;
public class UserService {
public List<User> getAllUsers() {
List<User> users = new ArrayList<>();
users.add(new User(1, "John Doe", "john@example.com"));
users.add(new User(2, "Jane Smith", "jane@example.com"));
return users;
}
}
Step 4: Creating the Servlet to Handle Requests
We'll create a servlet to manage our web requests and pass the users' list to the JSP page.
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.List;
@WebServlet("/users")
public class UserServlet extends HttpServlet {
private UserService userService = new UserService();
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<User> users = userService.getAllUsers();
request.setAttribute("users", users);
request.getRequestDispatcher("/users.jsp").forward(request, response);
}
}
Step 5: Designing the JSP Page
Now, we will craft the JSP page, users.jsp
, to display the data in a table format and make rows clickable.
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ page import="java.util.List" %>
<%@ page import="yourpackage.User" %>
<html>
<head>
<title>User List</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
}
tr:hover {
background-color: #f1f1f1;
cursor: pointer; /* Change cursor to pointer when hovering */
}
</style>
<script>
function rowClicked(userId) {
window.location.href = 'userDetails.jsp?id=' + userId;
}
</script>
</head>
<body>
<h2>User List</h2>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<%
List<User> users = (List<User>) request.getAttribute("users");
for (User user : users) {
%>
<tr onclick="rowClicked(<%= user.getId() %>)">
<td><%= user.getId() %></td>
<td><%= user.getName() %></td>
<td><%= user.getEmail() %></td>
</tr>
<%
}
%>
</tbody>
</table>
</body>
</html>
Explanation:
- Table Design: We create a simple table to display the user data. CSS is used to style the table, including hover effects.
- JavaScript Function: The
rowClicked()
function handles the click event. It constructs a URL that points to another JSP page, allowing you to view user details. - Dynamic Population: The user data is dynamically populated using JSP scriptlets.
Step 6: Create the User Details Page
Finally, we need to implement the userDetails.jsp
page, which displays detailed information about the users.
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ page import="yourpackage.User" %>
<%@ page import="java.util.List" %>
<%@ page import="yourpackage.UserService" %>
<%
int userId = Integer.parseInt(request.getParameter("id"));
UserService userService = new UserService();
List<User> users = userService.getAllUsers();
User selectedUser = null;
for (User user : users) {
if (user.getId() == userId) {
selectedUser = user;
break;
}
}
%>
<html>
<head>
<title>User Details</title>
</head>
<body>
<h1>User Details</h1>
<%
if (selectedUser != null) {
%>
<p>ID: <%= selectedUser.getId() %></p>
<p>Name: <%= selectedUser.getName() %></p>
<p>Email: <%= selectedUser.getEmail() %></p>
<%
} else {
%>
<p>User not found.</p>
<%
}
%>
</body>
</html>
Highlighting the 'Why':
- This page pulls the selected user's ID from the query parameters and fetches relevant data to display.
- Using a loop, it checks which user matches the provided ID.
Closing the Chapter
Making rows in a DataTable clickable can significantly improve the user experience of your Java web application. The example above illustrates a simple yet effective way to implement clickable rows using Servlets and JSP. By enabling users to easily access detailed information and perform actions, you contribute to a more interactive and enjoyable interface.
If you want to explore further, consider using Java frameworks like Spring Boot or JavaServer Faces (JSF) for a more sophisticated, scalable approach. You can draw inspiration from other platforms too, such as the techniques discussed in the article Boost UX: Make ASP.NET DataGrid Rows Clickable!.
By continuously enhancing web applications and focusing on user interactivity, you are building applications that not only function well but are also a pleasure to use. Happy coding!