Solving Hurdles in Generic Text Comparison with LCS
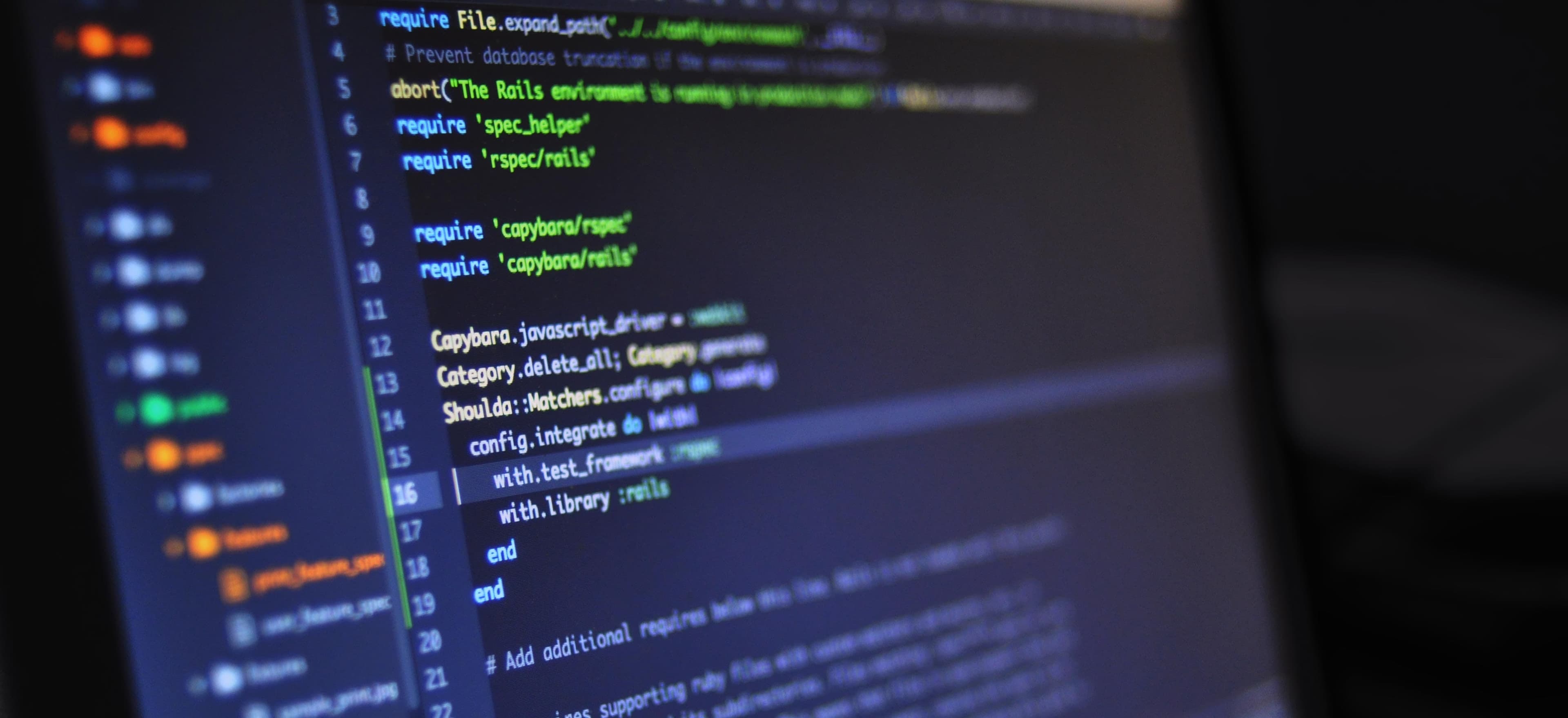
- Published on
Solving Hurdles in Generic Text Comparison with LCS
When it comes to text comparison, developers often face challenges, especially when dealing with large datasets. One of the most effective algorithms for this task is the Longest Common Subsequence (LCS). In this blog, we will explore what LCS is, how it works, its advantages, and provide Java code snippets to illustrate its implementation.
Understanding LCS
The Longest Common Subsequence (LCS) is a classic algorithm used to find the longest subsequence that appears in the same relative order in two sequences. Unlike substrings, the characters of a subsequence do not need to be adjacent.
For example:
- For the sequences "ABCBDAB" and "BDCAB", the LCS is "BCAB" or "BDAB", both of which have a length of 4.
Why Use the LCS Algorithm?
- Efficiency in Comparison: LCS can be used to find similarities between two texts efficiently without requiring a full comparison character by character.
- Versatile Applications: It's applied in various fields such as version control systems, bioinformatics, and data comparison tools.
- Error Detection: LCS helps in identifying changes and discrepancies in data, crucial for error detection in data storage.
The Basics of LCS Algorithm
LCS is typically implemented using dynamic programming due to its optimal substructure property. This means that the solution to the LCS problem can be built from solutions to smaller subproblems.
Dynamic Programming Table
The key idea is to create a two-dimensional table where each cell (i, j)
holds the length of the LCS of the two substrings s1[0..i-1]
and s2[0..j-1]
.
The Algorithm Steps
- Create a table of size
(m+1) x (n+1)
, wherem
andn
are the lengths of the two strings. - Initialize the first row and the first column with 0s.
- Compare each character of both strings and fill the table according to the rules:
- If characters match, increment the diagonal value.
- If they don’t, take the maximum value from the left or above.
- The value in the bottom-right corner of the table represents the length of the LCS.
Java Implementation of LCS
Let's delve into a Java implementation of the LCS algorithm.
public class LCS {
// Function to find LCS of two strings
public static int findLCS(String s1, String s2) {
int m = s1.length();
int n = s2.length();
// Create a table to store lengths of longest common subsequence.
int[][] dp = new int[m + 1][n + 1];
// Build the lookup table in bottom up fashion
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (s1.charAt(i - 1) == s2.charAt(j - 1)) {
// If characters match, increment the matching count.
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
// Take the maximum of left or top cell
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
// The length of the LCS is in the bottom right cell.
return dp[m][n];
}
public static void main(String[] args) {
String s1 = "ABCBDAB";
String s2 = "BDCAB";
// Calling the LCS function
int lcsLength = findLCS(s1, s2);
System.out.println("Length of LCS is: " + lcsLength);
}
}
Code Commentary
In the above code:
-
Table Initialization: We create a 2D array
dp
to store lengths of the longest common subsequences computed at various steps. This step is crucial as it allows the algorithm to refer back to previously computed results. -
Inner Loop Logic: The nested loop iterates through each character of both strings. If characters match, the corresponding cell in the table is updated to
dp[i-1][j-1] + 1
. This effectively counts the length of the matching subsequence up to that point. -
Return Value: Finally, the length of the LCS is returned from the bottom-right corner of the table, giving the result quickly and effectively.
Time Complexity
The time complexity of the LCS algorithm is O(m * n), where m and n are the lengths of the two inputs. This is efficient for many practical use cases.
Further Optimizations
Space Efficiency
While the above implementation has a time complexity of O(m * n), it can be optimized to use only O(min(m, n)) space. This can be achieved by storing only the current and previous row of the DP table.
public static int findLCSOptimized(String s1, String s2) {
int m = s1.length();
int n = s2.length();
// To optimize space, we can use only two rows
int[] prev = new int[n + 1];
int[] curr = new int[n + 1];
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (s1.charAt(i - 1) == s2.charAt(j - 1)) {
curr[j] = prev[j - 1] + 1;
} else {
curr[j] = Math.max(prev[j], curr[j - 1]);
}
}
// Move current to previous for the next iteration
int[] temp = prev;
prev = curr;
curr = temp;
}
// The length of the LCS is in the last cell of previous row
return prev[n];
}
Code Explanation
- The optimized version uses two 1D arrays,
prev
andcurr
, which significantly reduces the space complexity while maintaining the same time complexity.
Closing the Chapter
The Longest Common Subsequence algorithm is a powerful tool for text comparison, especially in scenarios demanding efficiency and accuracy. By leveraging dynamic programming, you can implement this algorithm in Java effectively, allowing for various applications from data comparison to error detection.
For further reading and a deeper understanding of dynamic programming, consider checking out GeeksforGeeks on Dynamic Programming or the classic introduction in the book Introduction to Algorithms.
The versatility of LCS proves invaluable for developers and researchers alike, making it an essential algorithm in the realm of programming. Understanding and implementing LCS is a stepping stone toward mastering various complex text analysis tasks. Happy coding!
Checkout our other articles