Overcoming Challenges in Synthetic and Bridge Methodologies
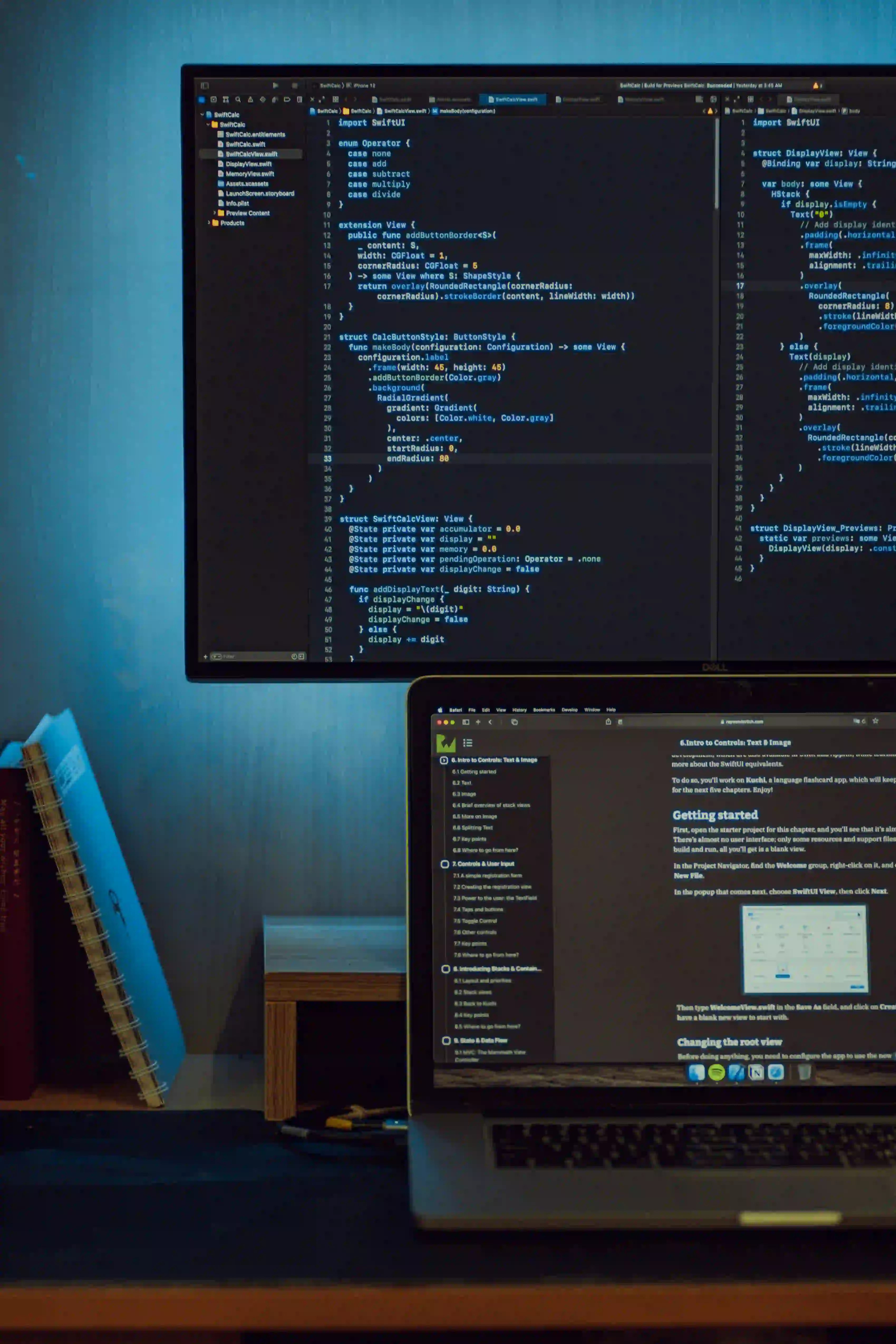
Overcoming Challenges in Synthetic and Bridge Methodologies
Synthetic and bridge methodologies are powerful approaches in various fields, including programming and software development. However, they present certain challenges that can impede progress. In this blog post, we will explore the common hurdles faced when implementing these methodologies and discuss practical solutions to overcome them.
Understanding Synthetic and Bridge Methodologies
Before diving into the challenges, let’s clarify what synthetic and bridge methodologies are.
Synthetic Methodologies
Synthetic methodologies involve combining disparate elements to create a new whole. In programming, this approach can be applied to integrate various frameworks, libraries, or services to build comprehensive solutions.
Bridge Methodologies
In contrast, bridge methodologies act as a conduit between different systems or protocols. In software development, this often refers to creating a middleware layer that facilitates communication between different parts of an architecture.
Common Challenges
1. Complexity in Integration
Challenge: Achieving seamless integration can be daunting due to varying technologies, languages, or standards.
Solution: Implement thorough documentation and utilize standard interfaces where possible. This can drastically reduce misunderstandings and facilitate easier integration.
Example Code: Using REST API for Integration
Here’s a simple example using Java with Spring Boot to integrate a REST API:
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/data")
public ResponseEntity<String> getData() {
// This method fetches data from another service
String data = fetchDataFromService();
return ResponseEntity.ok(data);
}
private String fetchDataFromService() {
// Logic to fetch data from another service
return "Sample Data";
}
}
Why this works: The use of annotations like @RestController
and @GetMapping
makes endpoints clear and manageable, promoting easier integration with front-end systems or other APIs.
2. Lack of Standardization
Challenge: Variation in coding standards, architectural styles, or methodology can lead to inconsistencies.
Solution: Create and enforce coding standards across your team or project, supplemented with style guides and regular code reviews.
Example Code: Code Style Enforcements with Checkstyle
You can integrate Checkstyle in your Maven project:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.2</version>
<configuration>
<configLocation>checkstyle.xml</configLocation>
<failOnViolation>true</failOnViolation>
</configuration>
</plugin>
Why this matters: By enforcing consistent code styles, you reduce friction when integrating code from various developers. It promotes clarity and easier debugging.
3. Performance Bottlenecks
Challenge: Bridging different systems or integrating layers can introduce performance issues.
Solution: Profile your application using tools like JProfiler or VisualVM to identify bottlenecks. Optimizing inefficient code paths is essential.
Example Code: Basic Performance Profiling in Java
Using System.nanoTime()
to measure execution time can help identify sluggish methods:
public void processData() {
long startTime = System.nanoTime();
// Process data
performComplexCalculation();
long endTime = System.nanoTime();
System.out.println("Execution Time: " + (endTime - startTime) + " ns");
}
Why profiling is vital: Continuous profiling allows you to catch performance issues early, which is critical when systems interact, as one slow service can degrade the entire application.
4. Dependency Management
Challenge: Dependencies can become unwieldy and conflict with one another in large projects.
Solution: Utilize dependency management tools like Maven or Gradle. Regular updates and audits of dependencies can prevent issues related to outdated libraries.
Example Code: Managing Dependencies with Maven
Here’s how to specify dependencies in a Maven pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
Why this is effective: Maven simplifies managing versions and helps avoid conflicts through dependency exclusion mechanisms.
5. Testing Challenges
Challenge: Testing synthetic systems or bridges can be complex due to multiple moving parts.
Solution: Implement robust unit tests and integration tests. Use frameworks like JUnit and Mockito to ensure that your components work independently and together.
Example Code: Unit Testing with JUnit
Here’s a simple test case in JUnit:
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class ApiControllerTest {
@Test
public void testGetData() {
ApiController apiController = new ApiController();
ResponseEntity<String> response = apiController.getData();
assertEquals("Sample Data", response.getBody());
}
}
Why testing is crucial: Regular testing ensures that as new features are added or changes are made, the synthetic or bridging functionality remains intact. It builds confidence in the system's reliability.
Wrapping Up
Overcoming challenges in synthetic and bridge methodologies requires a thoughtful approach that combines technical sophistication with effective communication and organization within teams. Embracing best practices like standardization, performance profiling, dependency management, and comprehensive testing can help achieve seamless system integration.
By using tools and techniques highlighted in this post, software developers can navigate the complexities of these methodologies, building robust applications that meet and exceed user expectations.
For further reading on best practices in software development methodologies, check out resources on Agile Development, or enhance your testing skills with JUnit.
By remaining proactive and attentive to these challenges, you can ensure successful implementation, leveraging the full potential of synthetic and bridge methodologies in your projects. Happy coding!