Mastering Java 14's Switch Statement: Common Pitfalls to Avoid
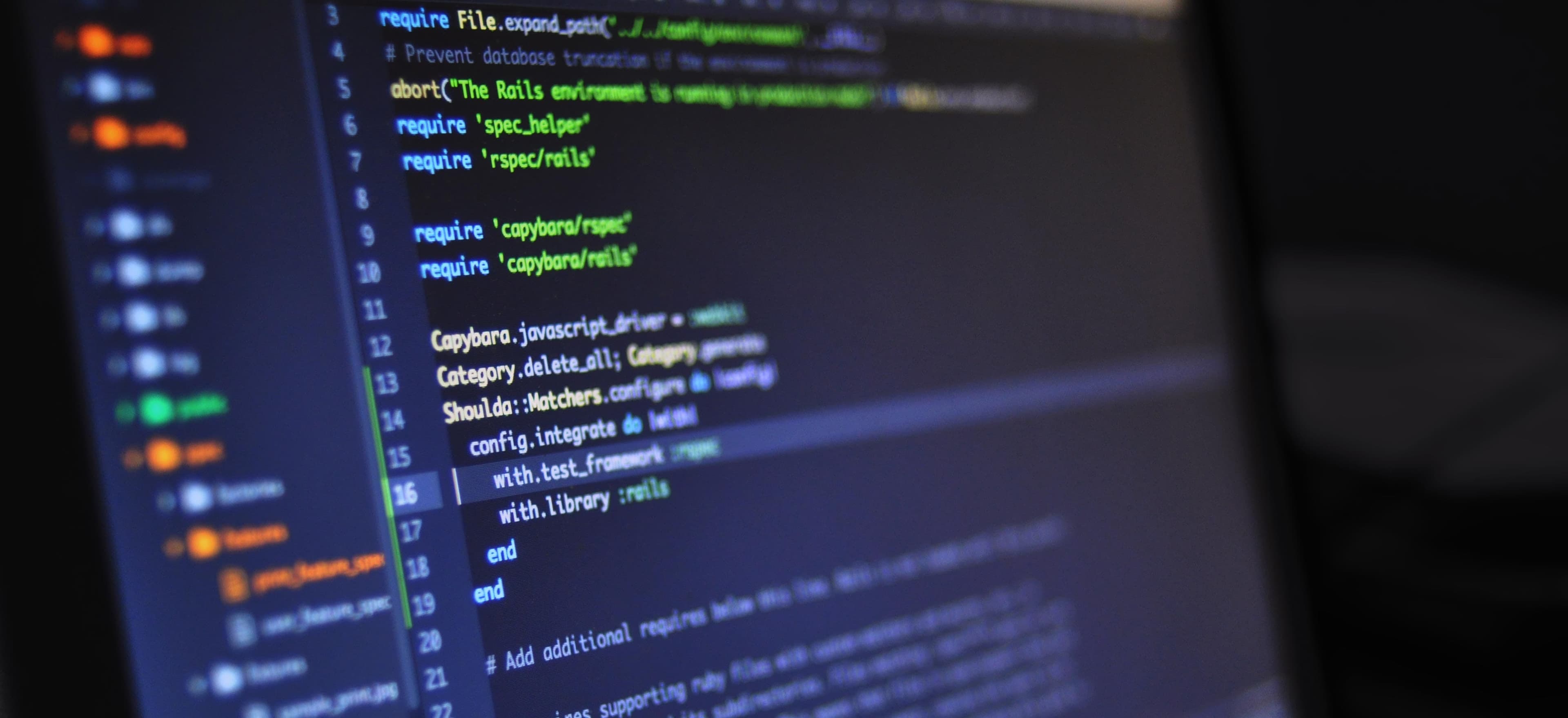
- Published on
Mastering Java 14's Switch Statement: Common Pitfalls to Avoid
Java has evolved significantly over the years, and each version brings enhancements that simplify code writing and increase clarity. Java 14, introduced in March 2020, offered some noteworthy enhancements to the switch statement. These enhancements aimed to streamline coding practices and minimize common pitfalls that developers encounter.
In this blog post, we will explore these enhancements to the switch statement in Java 14, identify some typical pitfalls, and illustrate best practices. By the end, you'll be well-equipped to use the switch statement efficiently in your Java applications.
Understanding Java 14's Switch Statement Enhancements
The New Switch Expression
One of the most significant changes in Java 14 is the introduction of swich expressions. Unlike previous versions, which treated the switch statement purely as a control statement, Java 14 allows developers to use switches as expressions that return a value.
Here’s an example:
int dayOfWeek = 6;
String dayType = switch (dayOfWeek) {
case 1, 2, 3, 4, 5 -> "Weekday";
case 6, 7 -> "Weekend";
default -> throw new IllegalArgumentException("Invalid day: " + dayOfWeek);
};
System.out.println(dayType);
Why Use Switch Expressions?
- Clarity and Readability: The arrow (
->
) notations make the code clearer and easier to read compared to the traditional colon syntax. - Multiline Blocks: Switch expressions can now handle multiple statements by using curly braces, improving the organization of code.
Here's a quick example leveraging multiline cases:
String result = switch (dayOfWeek) {
case 1, 2, 3, 4, 5 -> {
System.out.println("Processing Weekday...");
yield "Weekday"; // Using yield to return a value from a block
}
case 6, 7 -> {
System.out.println("Processing Weekend...");
yield "Weekend";
}
default -> throw new IllegalArgumentException("Invalid day: " + dayOfWeek);
};
System.out.println(result);
Key Benefits
- **
yield
Keyword:** This keyword allows you to return a value from within a block. It’s less error-prone and makes your intentions clear. - Less Boilerplate: You no longer need to use the
break
statement, reducing the chances of forgetting it and causing fall-through errors.
Common Pitfalls to Avoid with Java 14's Switch Statement
While Java 14's enhancements make the switch statement more powerful and readable, a few common pitfalls still exist that developers should be aware of:
1. Forgetting the Default Case
One of the most commonly encountered pitfalls is neglecting to handle the default case. Omitting it can lead to unhandled cases, which can cause IllegalArgumentException
or return null
.
Example:
int number = 10; // A value not handled in the switch
String numberType = switch (number) {
case 1 -> "One";
case 2 -> "Two";
// No default case
};
To avoid this, always include a default case:
String numberType = switch (number) {
case 1 -> "One";
case 2 -> "Two";
default -> "Unknown Number";
};
2. Using Incorrect Case Types
Another pitfall in using switch expressions is the mismatch of data types. Switch expressions require that all cases match the type of the switch variable.
Example:
String weather = "Sunny";
switch (weather) {
case "Sunny", "Cloudy" -> System.out.println("Good weather.");
case 1 -> System.out.println("Bad weather."); // Error: type mismatch
}
Ensure that all case expressions match the type of the switch variable.
3. Overlooking Fall-Through Logic
While traditional switch statements benefitted from fall-through behavior, realizing it might not always be desirable. In expressive cases, fall-through can lead to unintended consequences.
Example of an unintended fall-through:
String fruit = "Apple";
switch (fruit) {
case "Apple":
case "Banana":
System.out.println("This is a fruit.");
case "Carrot": // Missing break statement
System.out.println("It's a vegetable too.");
break;
}
To prevent this from happening in your code, always ensure that every case is properly handled with break statements or utilize switch expressions for clear control.
4. Misuse of Yield Outside Switch Context
When using the yield
keyword within switch expressions, it must not be confused with typical assignments. Misplacing yield outside a switch can result in a syntax error.
Example of incorrect usage:
int day = 3;
String type;
if (day < 6) {
type = yield "Weekday"; // Error: yield used outside of switch expression
}
Recommended Practices
To make the most of Java 14's switch statement and expressions, consider the following best practices:
- Always Include Default Cases: Default cases safeguard against unexpected inputs.
- Prioritize Readability: Use switch expressions whenever possible for clarity.
- Avoid Unnecessary Complexity: If a case can be managed outside of switch, consider using simpler structures (like if-else).
- Use Descriptive Labels: Clearly label each case for better understanding and maintainability.
In Conclusion, Here is What Matters
Java 14's switch statement improvements offer powerful tools for cleaner and more efficient code writing. Still, developers should remain aware of common pitfalls that accompany these enhancements. By avoiding mistakes like neglecting default cases and allowing fall-through, developers can leverage the full capabilities of switch expressions.
To further explore switch statements or Java in general, consider reaching out to Java documentation here for comprehensive guides and best practices.
Armed with this knowledge, embark on your Java programming journey with renewed confidence, ready to outsmart the pitfalls that often accompany the switch statement.
Checkout our other articles