Unlocking Hidden Gems: 7 Java Answers You Overlooked
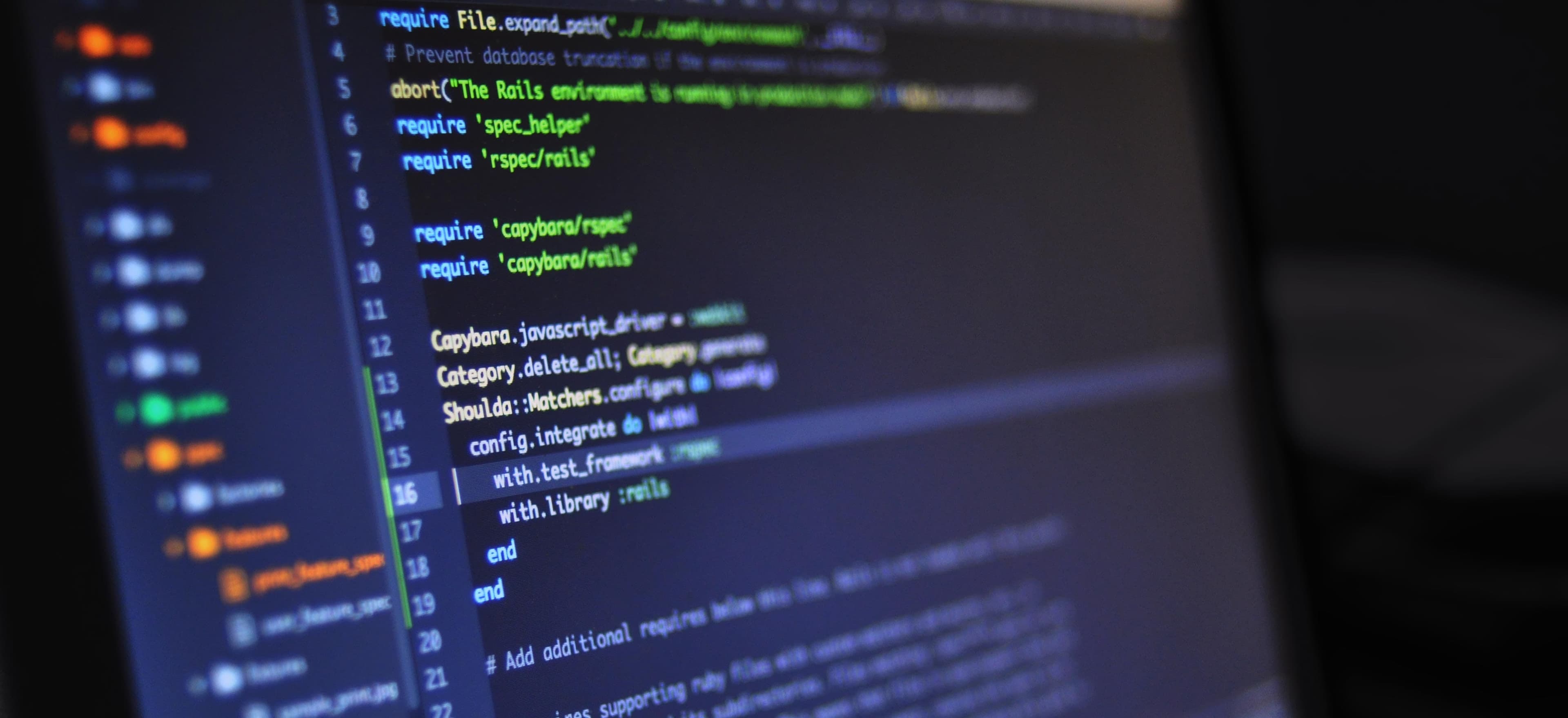
- Published on
Unlocking Hidden Gems: 7 Java Answers You Overlooked
Java, a perennial favorite in the programming world, brings more than just object-oriented programming to the table. Its rich ecosystem is filled with tools, libraries, and features that, while incredibly powerful, often remain untouched by many developers. This blog post explores seven such hidden gems of Java. Whether you're a novice or a seasoned developer, these features can sharpen your coding skills and enhance your applications.
1. Streams API
Introduced in Java 8, the Streams API transforms how we handle collections in Java. It allows you to process sequences of elements, such as lists and sets, efficiently and expressively.
Why Use Streams?
- Concise Code: Streams provide a fluent interface that reduces boilerplate code.
- Parallel Processing: Easily switch to parallel streams for performance gains on large data sets.
Example Code
import java.util.List;
import java.util.Arrays;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using streams to filter, square and collect results
List<Integer> squaredNumbers = numbers.stream()
.filter(n -> n % 2 == 0) // Filter even numbers
.map(n -> n * n) // Square the numbers
.toList(); // Collect results into a new List
System.out.println(squaredNumbers); // Output: [4, 16]
}
}
In this code, note how we chain methods to create a pipeline. This not only improves readability but also allows for complex data processing without the need for.verbose for
loops.
2. Optional Class
The Optional
class in Java provides a way to express that a value may or may not be present. This is particularly useful for dealing with null
values that can lead to NullPointerExceptions
.
Why Use Optional?
- Clear Intent: It communicates the possibility of absence clearly.
- Safer Code: Encourages you to consider the absence of a value.
Example Code
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> name = getNameById(1);
// Use ifPresent to execute logic only if a value is present
name.ifPresent(n -> System.out.println("Name found: " + n));
// Provide default value if not present
String defaultName = name.orElse("Default Name");
System.out.println("Hello, " + defaultName);
}
private static Optional<String> getNameById(int id) {
return (id == 1) ? Optional.of("Alice") : Optional.empty();
}
}
In this example, you'll see how Optional
streamlines checking for nulls, providing a more fluent way to manage absence. This can significantly reduce error rates in your code.
3. var Keyword
Java 10 introduced the var
keyword, enabling type inference for local variables. This allows you to define variables without explicitly stating their types.
Why Use var?
- Reduced Verbosity: Especially helpful for complex types.
- Improved Readability: Makes the code cleaner without sacrificing clarity.
Example Code
import java.util.List;
public class VarExample {
public static void main(String[] args) {
var numbers = List.of(1, 2, 3, 4, 5);
var sum = numbers.stream()
.mapToInt(Integer::intValue)
.sum();
System.out.println("Sum: " + sum);
}
}
Here, the var
keyword allows us to focus on the logic without getting bogged down by data types. However, it's essential to use it judiciously, as too much reliance on var
can obscure the understanding of the code.
4. Records
Java 14 introduced the concept of records, which provide a concise way to create data-carrying classes. They essentially reduce boilerplate code and improve immutability.
Why Use Records?
- Less Boilerplate: Automatically generates constructors, accessors, equals, hashCode, and toString methods.
- Immutable Data holders: Ensures that instances of records are immutable.
Example Code
public record Person(String name, int age) { }
public class RecordExample {
public static void main(String[] args) {
var alice = new Person("Alice", 30);
System.out.println(alice.name()); // Output: Alice
System.out.println(alice.age()); // Output: 30
}
}
As you can see, defining a data class like Person
is straightforward and elegant. This approach not only saves time but also reduces the likelihood of errors typically associated with manually writing boilerplate code.
5. Pattern Matching for instanceof
Introduced as a preview feature in Java 14, pattern matching for instanceof
simplifies the common pattern of type checking and casting.
Why Use Pattern Matching?
- Cleaner Code: Reduces boilerplate associated with type checks.
- Increased Safety: Helps prevent class cast exceptions by providing a safer way to perform casts.
Example Code
public class PatternMatchingExample {
public static void main(String[] args) {
Object obj = "Hello, World!";
if (obj instanceof String s) { // Pattern matching introduced here
System.out.println(s.toUpperCase()); // Output: HELLO, WORLD!
}
}
}
Pattern matching enables developers to express logic more directly, enhancing readability and maintainability.
6. CompletableFuture
The CompletableFuture
class in Java is an enhancement to the Future
interface that allows non-blocking asynchronous programming.
Why Use CompletableFuture?
- Asynchronous Programming: Facilitates concurrent programming.
- Fluent API: Provides methods to handle computations when they complete.
Example Code
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(2000); // Simulating a long-running task
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return 42; // Simulated result
});
// Non-blocking get for the result
future.thenAccept(result -> System.out.println("Result: " + result));
System.out.println("Doing other stuff while waiting...");
}
}
This code shows how CompletableFuture
helps manage tasks without blocking the current thread, leading to a responsive application.
7. Enhanced Switch Expressions
Introduced in Java 12, switch expressions offer a more powerful and flexible way to use switch statements. They allow for more concise syntax and have extended capabilities.
Why Use Enhanced Switch Expressions?
- More Compact Code: Reduces the potential for fall-through bugs.
- Return Values: Switches can now return values seamlessly.
Example Code
public class SwitchExpressionExample {
public static void main(String[] args) {
var dayType = switch (6) {
case 1, 2, 3, 4, 5 -> "Weekday";
case 6, 7 -> "Weekend";
default -> "Invalid day";
};
System.out.println("Day type: " + dayType); // Output: Day type: Weekend
}
}
This elegant handling of multiple cases enhances code readability and maintains intent clearly.
In Conclusion, Here is What Matters
Java is a deep language packed with features that can vastly improve your coding efficiency and the robustness of your applications. From the Streams API to enhanced switch expressions, leveraging these hidden gems can lead to cleaner, more efficient, and more enjoyable coding experiences.
As you embark on your coding journey or continue honing your craft, make sure you familiarize yourself with these features to unlock the full potential of Java. Whether you're building enterprise-level applications or simple tools, these Java capabilities will elevate your programming standards and enhance your software development practices.
For more on Java best practices, check out Oracle's official documentation or the latest Java features on Baeldung. Happy coding!