Boost Cache Efficiency with Last-Modified and ETag Headers
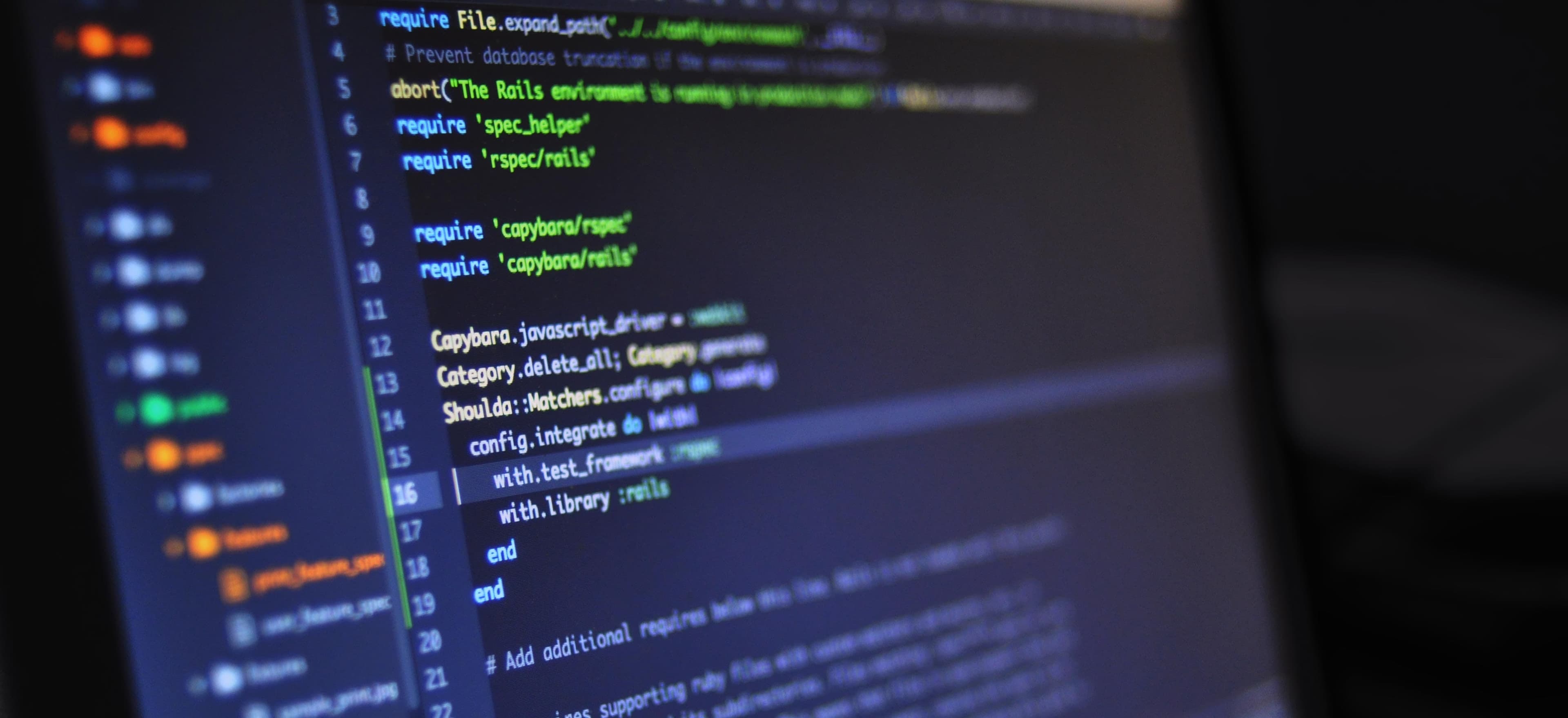
- Published on
Boost Cache Efficiency with Last-Modified and ETag Headers
Caching is a critical component of web development and performance optimization. Understanding how to manage and use cache effectively can lead to improved load times and a better overall user experience. While there are various caching strategies available, two powerful HTTP headers that are often overlooked are Last-Modified
and ETag
headers. In this post, we will delve into these headers, how they work, and how they can help you boost cache efficiency in your applications.
What are Last-Modified and ETag Headers?
Last-Modified Header
The Last-Modified
header is a response header used to indicate the last time a resource was changed. When a client requests a resource, the server sends this header along with the content. The client can then store this information and use it in subsequent requests to the server.
ETag Header
The ETag
header, short for "entity tag," is a unique identifier assigned by the server to a specific version of a resource. Whenever the resource changes, the server updates the ETag. This allows clients to check the validity of their cached version without needing to download the entire resource again.
How Do They Improve Cache Efficiency?
-
Reduced Bandwidth Usage: By using these headers, clients can validate their cached resources with the server rather than retrieving everything anew. This reduces the amount of data sent over the network.
-
Improved Load Times: Since clients can skip downloading unchanged resources, they experience faster load times, which enhances user experience.
-
Effective Cache Management: Both headers allow developers to manage cache more efficiently by only re-validating resources when necessary.
Implementing Last-Modified and ETag in Your Application
Last-Modified Example
To implement the Last-Modified
header, you first need to know when a resource was last updated. For example:
import javax.servlet.http.HttpServletResponse;
import java.time.Instant;
public void serveResource(HttpServletResponse response) {
// Get the last modified time of the resource
Instant lastModifiedTime = Instant.now(); // Example: fetch from database or file metadata
// Set Last-Modified header
response.setHeader("Last-Modified", lastModifiedTime.toString());
// Serve the resource
response.getWriter().write("Your resource content!");
}
Why Use Last-Modified? When a client receives this header, it stores the timestamp of the last modification. In subsequent requests, the client can send an If-Modified-Since
header to check if the resource has changed since that date. If the server responds with a 304 Not Modified
, the client can use its cached version.
ETag Example
To use ETags, you first need to generate a unique tag for the resource:
import javax.servlet.http.HttpServletResponse;
public void serveResource(HttpServletResponse response) {
// Generate an ETag. In real-world scenarios, this could be a hash of the content.
String etag = generateEtagForResource(); // Replace with actual logic here
// Set ETag header
response.setHeader("ETag", etag);
// Serve the resource
response.getWriter().write("Your resource content!");
}
private String generateEtagForResource() {
// Mock implementation; informally hash your resource
return String.valueOf(System.currentTimeMillis()); // Simple current timestamp as ETag
}
Why Use ETag? When the client sends an If-None-Match
header with its stored ETag, the server can compare it against the current ETag of the resource. If they match, the server responds with 304 Not Modified
, allowing the client to use the cached version.
Comparing Last-Modified and ETag
While both Last-Modified
and ETag
serve the purpose of cache validation, they come with different advantages and limitations:
| Feature | Last-Modified | ETag | |---------------------------------|-----------------------------------|-----------------------------------| | Format | Timestamp | Unique identifier, often a hash | | Granularity | Can be coarse (last update only) | Can be very fine (specific content variations) | | Response Time | Requires checking timestamp | Fast, checks the ETag only |
Use Cases and Best Practices
1. Static Resources
For static resources like images, CSS, and JavaScript files, using ETags is often the best choice as it provides a precise way of identifying changes to a file.
2. Dynamic Content
For dynamic content, such as HTML pages that may be updated frequently, the Last-Modified
header can help reduce unnecessary loads while still giving a reasonable update frequency.
3. Combine Headers
In many cases, it is beneficial to use both headers together. This gives clients multiple ways to validate their cache while allowing more flexibility in caching strategies.
4. Testing and Monitoring
Make sure to monitor the responses when implementing these headers. Use tools like Google PageSpeed Insights to track performance improvements. Test the cache behavior with developer tools in browsers like Chrome or Firefox, checking the Network
tab for responses.
The Last Word
Implementing Last-Modified
and ETag
headers can significantly enhance your web application’s cache efficiency, leading to improved load times and reduced bandwidth usage. By understanding and effectively utilizing these headers, you can ensure that your users experience the web application at its best.
For additional reading, the Mozilla Developer Network (MDN) on HTTP caching provides a thorough explanation of these mechanisms, offering in-depth understanding for developers at any level.
By taking these principles to heart and implementing them diligently, you will not only optimize your application but also improve the user experience you provide.
Additional Resources
- Understanding HTTP Caching
- Caching Best Practices
Happy coding!