Boosting Logging Performance: Key Strategies Revealed
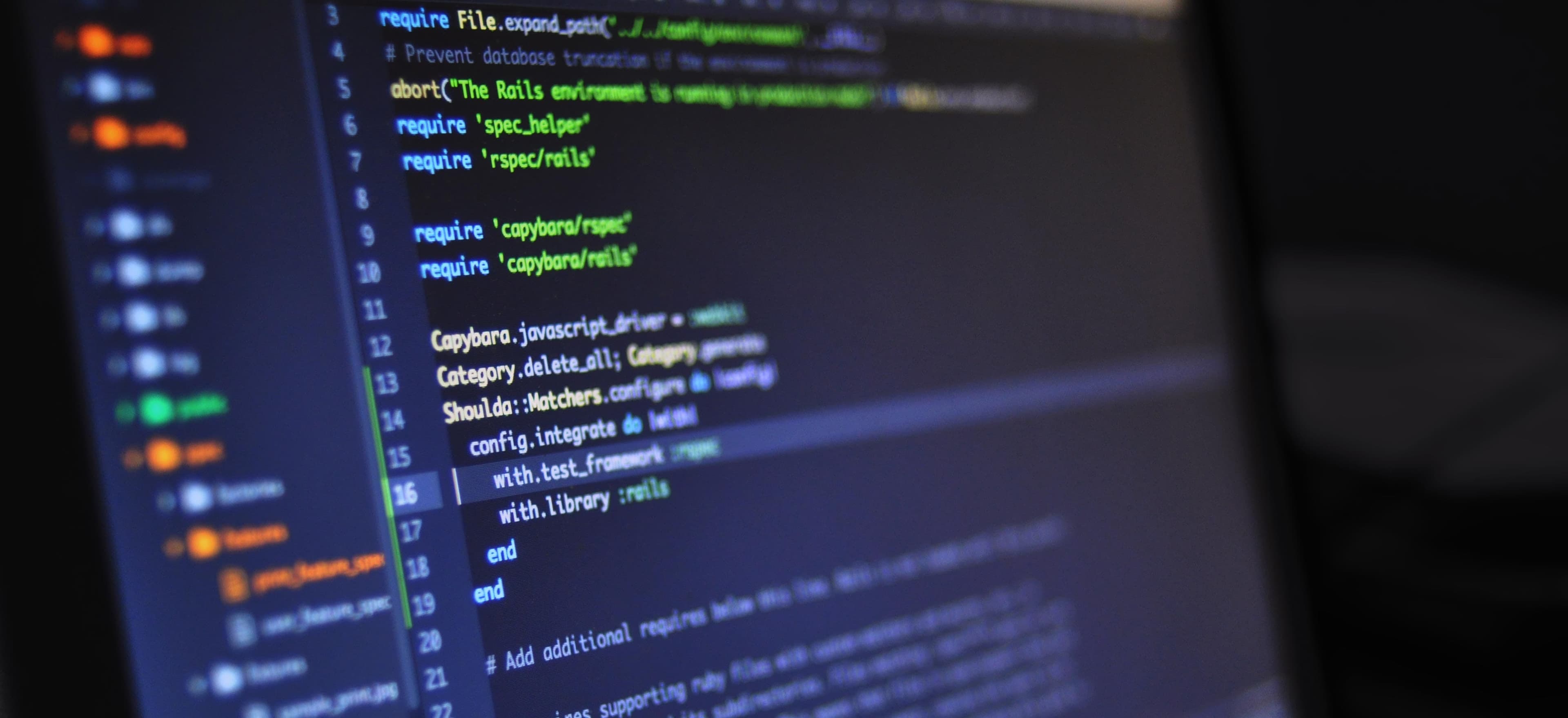
- Published on
Boosting Logging Performance: Key Strategies Revealed
In the ever-evolving world of software development, logging has become an essential part of maintaining, debugging, and analyzing applications. Efficient logging not only helps developers identify issues quickly but also improves application performance. In this blog post, we will explore strategies for optimizing logging performance in Java applications, provide code snippets, and delve into the reasons behind these practices.
Understanding Logging in Java
Java offers several built-in logging frameworks, such as Java Util Logging, Log4j, and SLF4J, to help manage application logs effectively. Each has its own strengths; however, what unites them is their potential to impact performance negatively when not used appropriately.
Why Optimize Logging?
Before diving into performance-boosting strategies, let's understand why optimizing logging is crucial:
- Application Performance: Excessive or poorly managed logging can result in excessive CPU and I/O resource consumption.
- Maintainability: Logs need to be readable and structured for easy analysis.
- Error Diagnosis: Rapid retrieval of logs can significantly expedite issue resolution.
Now that we've established the relevance of optimizing logging in Java, let's explore practical strategies.
1. Use Asynchronous Logging
One of the most effective ways to enhance logging performance is to implement asynchronous logging. This method allows log messages to be written in a separate thread, reducing the time your primary application threads spend waiting for logging operations to complete.
Example of Asynchronous Logging Using Log4j 2
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.core.async.AsyncLogger;
public class AsyncLoggingExample {
private static final Logger logger = LogManager.getLogger(AsyncLoggingExample.class);
public void performTask() {
logger.info("Task started.");
// Task implementation
logger.info("Task completed.");
}
}
Why Asynchronous Logging?
Using AsyncLogger
from Log4j 2 effectively decouples log writing from the application’s main execution flow. This reduces the lag and improves throughput, especially under heavy loads.
2. Log Only What You Need
It's tempting to log every bit of information you can, especially during development. However, excessive logging can lead to performance degradation. Focus on logging what is necessary to trace application behavior and diagnose errors.
Example of Conditional Logging
public void processTransaction(Transaction transaction) {
if (logger.isDebugEnabled()) {
logger.debug("Processing transaction: {}", transaction.getId());
}
// Transaction processing logic
}
Why Conditional Logging?
By checking if debug logging is enabled before logging detailed information, you prevent unnecessary computation in cases where debug logs are not needed, conserving system resources.
3. Implement Logging Levels
Leverage different logging levels to control what gets logged. Java logging frameworks typically support levels such as TRACE, DEBUG, INFO, WARN, ERROR, and FATAL. By setting an appropriate logging level, you can filter out less critical logs in production environments.
Example of Setting Logging Levels
# log4j2.xml configuration example
<Configuration>
<Loggers>
<Root level="ERROR">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Why Use Logging Levels?
Logging levels allow for fine-grained control over what gets output. In a production environment, you might only want errors, while debug information is crucial during development. This flexibility prevents clutter and enhances performance significantly.
4. Buffering Log Outputs
Buffering log outputs can also improve logging performance. By temporarily holding log messages before writing them to disk, you reduce the frequency of I/O operations, which can be a bottleneck.
Example of Buffering
import org.apache.logging.log4j.core.Appender;
import org.apache.logging.log4j.core.ConsoleAppender;
import org.apache.logging.log4j.core.layout.PatternLayout;
public class BufferedLogging {
private static final Appender appender = ConsoleAppender.createAppender(
PatternLayout.newBuilder().withPattern("%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n").build(),
null, null, false, null
);
static {
appender.start();
((LoggerContext) LogManager.getContext(false)).getConfiguration().addAppender(appender);
}
public void logBuffered() {
logger.info("Buffered logging output.");
}
}
Why Buffering?
Buffering reduces disk I/O overhead by consolidating log messages into larger chunks before writing them out. This approach significantly boosts performance, especially for applications with high logging frequency.
5. Configure Log Rotation and Compression
Log files can grow significantly over time, consuming I/O bandwidth. Configuring log rotation and compression ensures old logs are archived efficiently, maintaining optimal performance.
Example of Log Rotation
# log4j2.xml rotation configuration
<Appenders>
<RollingFile name="RollingFile" fileName="logs/app.log"
filePattern="logs/app-%d{yyyy-MM-dd}-%i.log.gz">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
<Policies>
<TimeBasedTriggeringPolicy interval="1" modulate="true"/>
<SizeBasedTriggeringPolicy size="100MB"/>
</Policies>
</RollingFile>
</Appenders>
Why Rotate and Compress Logs?
Log rotation and compression save disk space and maintain application performance. They also make it easier to manage logs over time, so you can investigate historical data without overwhelming your system's resources.
6. Use a Logging Framework
While it's possible to create custom logging solutions, using established logging frameworks can save time and often provides features out-of-the-box that may enhance performance significantly.
Popular Java Logging Frameworks
- Log4j 2: An improvement over Log4j offering asynchronous logging capabilities.
- SLF4J: A simple facade that can support various logging frameworks including Log4j.
- Logback: A robust solution and successor to Log4j offering additional features.
By leveraging these frameworks, you can also take advantage of community support and findings to streamline logging practices in your applications.
Final Thoughts
Optimizing logging performance is crucial for any Java application, extending beyond mere log management into overall application efficiency. By implementing asynchronous logging, conditional logging, using logging levels, buffering outputs, configuring log rotation, and utilizing established logging frameworks, you can significantly enhance performance and maintainability.
As logging continues to play a crucial role in software development, it's essential to adopt optimization strategies that suit your application's specific needs. For further reading, you may explore the official Log4j documentation and best practices for logging in Java.
Embrace these practices, and see remarkable strides in the performance of your Java applications!